DSA Interview Questions
- DSA Questions for Beginners
- Advanced DSA Questions for Competitive Programming
- Top 10 DSA Questions to Crack Your Next Coding Test
- Top 50 DSA Questions Every Programmer Should Practice
- Top Atlassian DSA Interview Questions
- Top Amazon DSA Interview Questions
- Top Microsoft DSA Interview Questions
- Top Meta (Facebook) DSA Interview Questions
- Netflix DSA Interview Questions and Preparation Guide
- Top 20 DSA Interview Questions You Need to Know
- Top Uber DSA Interview Questions and Solutions
- Google DSA Interview Questions and How to Prepare
- Airbnb DSA Interview Questions and How to Solve Them
- Mobile App DSA Interview Questions and Solutions
System Design Interview Questions
- Adobe System Design Interview Questions
- Top Atlassian System Design Interview Questions
- Top Amazon System Design Interview Questions
- Top Microsoft System Design Interview Questions
- Top Meta (Facebook) System Design Interview Questions
- Top Netflix System Design Interview Questions
- Top Uber System Design Interview Questions
- Top Google System Design Interview Questions
- Top Apple System Design Interview Questions
- Top Airbnb System Design Interview Questions
- Top 10 System Design Interview Questions
- Mobile App System Design Interview Questions
- Top 20 Stripe System Design Interview Questions
- Top Shopify System Design Interview Questions
- Top 20 System Design Interview Questions
- Top Advanced System Design Questions
- Most-Frequented System Design Questions in Big Tech Interviews
- What Interviewers Look for in System Design Questions
- Critical System Design Questions to Crack Any Tech Interview
- Top 20 API Design Questions for System Design Interviews
- Top 10 Steps to Create a System Design Portfolio for Developers
Introduction to High-Level System Design
System Design Fundamentals
- Functional vs. Non-Functional Requirements
- Scalability, Availability, and Reliability
- Latency and Throughput Considerations
- Load Balancing Strategies
Architectural Patterns
- Monolithic vs. Microservices Architecture
- Layered Architecture
- Event-Driven Architecture
- Serverless Architecture
- Model-View-Controller (MVC) Pattern
- CQRS (Command Query Responsibility Segregation)
Scaling Strategies
- Vertical Scaling vs. Horizontal Scaling
- Sharding and Partitioning
- Data Replication and Consistency Models
- Load Balancing Strategies
- CDN and Edge Computing
Database Design in HLD
- SQL vs. NoSQL Databases
- CAP Theorem and its Impact on System Design
- Database Indexing and Query Optimization
- Database Sharding and Partitioning
- Replication Strategies
API Design and Communication
Caching Strategies
- Types of Caching
- Cache Invalidation Strategies
- Redis vs. Memcached
- Cache-Aside, Write-Through, and Write-Behind Strategies
Message Queues and Event-Driven Systems
- Kafka vs. RabbitMQ vs. SQS
- Pub-Sub vs. Point-to-Point Messaging
- Handling Asynchronous Workloads
- Eventual Consistency in Distributed Systems
Security in System Design
Observability and Monitoring
- Logging Strategies (ELK Stack, Prometheus, Grafana)
- API Security Best Practices
- Secure Data Storage and Access Control
- DDoS Protection and Rate Limiting
Real-World System Design Case Studies
- Distributed locking (Locking and its Types)
- Memory leaks and Out of memory issues
- HLD of YouTube
- HLD of WhatsApp
Top 10 DSA Questions to Crack Your Next Coding Test
In today’s competitive coding environment, preparing for coding tests means mastering Data Structures and Algorithms (DSA). Whether you’re a student, a professional, or simply passionate about coding, understanding DSA is a must for cracking technical interviews and tests. If you’re looking for free courses or the latest course updates, check out our lead capture form here. In this article, we will cover the top 10 DSA questions along with effective strategies and common pitfalls so you can be confident in your coding abilities. The content below is designed in a clear, engaging way that even a fifth grader can follow while still providing detailed insights and practical tips.
Why DSA is Crucial for Coding Tests
Understanding data structures and algorithms is key to solving problems quickly and efficiently during coding tests. Many technical interviews focus on these topics because they measure your problem-solving skills and the efficiency of your code. In addition, mastering DSA not only improves your coding abilities but also lays the foundation for a successful career in software development.
Learning DSA helps in:
- Enhancing problem-solving skills by breaking down complex problems into smaller, manageable parts.
- Improving your coding efficiency through optimized solutions.
- Building a strong foundation for advanced topics like system design and optimization.
Many industry experts note that proficiency in DSA can increase your chances of landing a job by 30% or more. According to a study by HackerRank, candidates who perform well in DSA questions are 2.5 times more likely to succeed in technical interviews.
Additionally, quotes from top tech leaders, such as the famous statement by Bill Gates, “The ability to solve problems is more important than knowing all the answers,” resonate with the importance of continuous learning and practice in DSA.
Beyond the technical benefits, the confidence gained through mastering these questions is invaluable. A focused approach to DSA can lead to more successful outcomes in competitive coding tests and real-world programming challenges.
Also Read: Low-Level Design of YouTube Recommendations
In-Depth Analysis of Top 10 DSA Questions
This section dives into the top 10 DSA questions that are commonly asked during coding tests. Each question is explained with its core idea, approaches to solve it, and sample code or pseudocode where applicable. Below, you will find detailed explanations under H3 headers for each question.
1. Reverse a Linked List
Reversing a linked list is a classic problem that tests your understanding of pointer manipulation. The idea is to change the direction of the linked list so that the head becomes the tail and vice versa.
- Approach:
Use iterative or recursive methods to change the pointers. The iterative method involves three pointers: previous, current, and next. - Tips:
Ensure you handle edge cases, such as an empty list or a list with only one node.
Key Points:
- Time Complexity: O(n)
- Space Complexity: O(1)
Example Table:
Approach | Time Complexity | Space Complexity |
Iterative | O(n) | O(1) |
Recursive | O(n) | O(n) |
Also Read: Low-Level Design of WhatsApp Messaging
2. Detect a Cycle in a Linked List
Detecting a cycle in a linked list is another fundamental problem that helps evaluate your understanding of pointers and memory.
- Approach:
Use Floyd’s Cycle-Finding Algorithm (Tortoise and Hare), which uses two pointers moving at different speeds. - Tips:
If the fast pointer meets the slow pointer, a cycle exists.
Key Points:
- Time Complexity: O(n)
- Space Complexity: O(1)
Bullet Points:
- Efficient detection of cycles.
- Minimal memory overhead.
- Widely used in interview scenarios.
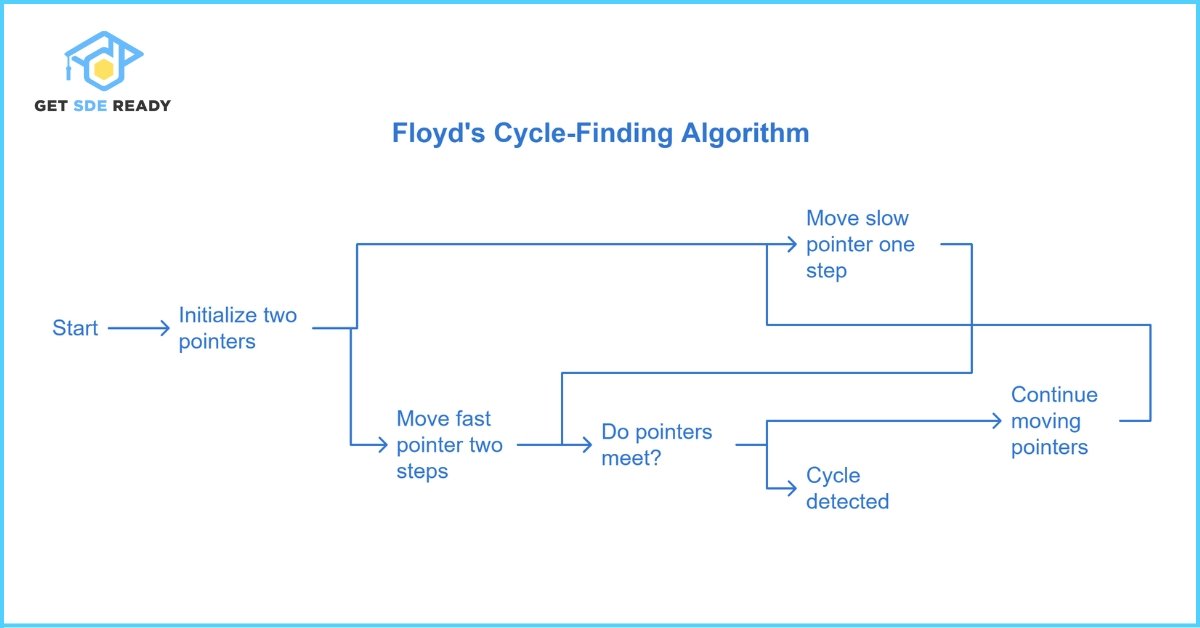
3. Merge Two Sorted Arrays
Merging two sorted arrays tests your ability to handle arrays and the two-pointer technique.
- Approach:
Start pointers at the beginning of both arrays and merge them in a sorted manner. - Tips:
Consider edge cases where arrays are of different sizes.
Key Points:
- Time Complexity: O(n + m)
- Space Complexity: O(n + m) if extra space is used; in-place merge can optimize space usage.
Bullet Points:
- Ideal for practicing the two-pointer technique.
- Common in algorithmic challenges.
- Useful for understanding sorting fundamentals.
4. Binary Search on a Sorted Array
Binary search is a classic example of using a divide-and-conquer approach to reduce the search space.
- Approach:
Continuously split the array into halves until the element is found or the subarray size becomes zero. - Tips:
Always calculate the mid-point carefully to avoid overflow in some programming languages.
Key Points:
- Time Complexity: O(log n)
- Space Complexity: O(1)
Bullet Points:
- Efficient searching in sorted arrays.
- Critical for understanding algorithm efficiency.
- Forms the basis for more advanced search algorithms.
5. Find the Kth Largest Element in an Array
This problem challenges you to efficiently locate the kth largest element, often solved using a heap or quickselect algorithm.
- Approach:
Use a min-heap for a simpler solution or quickselect for an average-case linear time solution. - Tips:
Understand the trade-offs between heaps and partition-based methods.
Key Points:
- Time Complexity: O(n) average for quickselect, O(n log k) using a heap.
- Space Complexity: Varies by method.
Bullet Points:
- Excellent for practicing selection algorithms.
- Demonstrates the concept of partitioning.
- Frequently encountered in competitive programming.
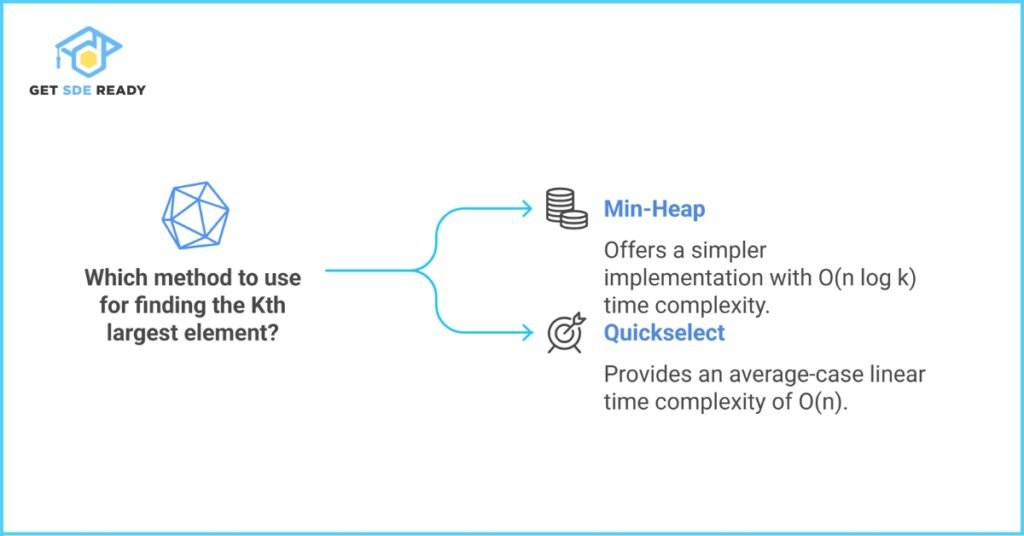
6. Implement a Stack Using Queues
This problem tests your understanding of data structures by implementing one data structure using another.
- Approach:
Use two queues to mimic the behavior of a stack. - Tips:
Focus on the push and pop operations to maintain the Last-In-First-Out (LIFO) property.
Key Points:
- Time Complexity: Varies based on implementation.
- Space Complexity: O(n)
Bullet Points:
- Enhances understanding of abstract data types.
- Good exercise in adapting data structure operations.
- Often discussed in system design interviews.
7. Evaluate a Postfix Expression
Evaluating postfix expressions requires understanding of stacks and operator precedence.
- Approach:
Use a stack to push operands and apply operators as they appear. - Tips:
Ensure correct handling of operator precedence and edge cases like division by zero.
Key Points:
- Time Complexity: O(n)
- Space Complexity: O(n)
Bullet Points:
- Simplifies the evaluation of complex expressions.
- Excellent for understanding stack-based algorithms.
- Frequently appears in coding assessments.
8. Find the Intersection of Two Arrays
This problem is useful to test your grasp of arrays and hash-based data structures.
- Approach:
Use hash sets to store elements of one array and then check for common elements. - Tips:
Consider using sorting as an alternative approach if extra space is a concern.
Key Points:
- Time Complexity: O(n + m)
- Space Complexity: O(min(n, m))
Bullet Points:
- Good for learning hash-based algorithms.
- Demonstrates the importance of data structure choice.
- Common in online coding challenges.
9. Implement Queue Using Stacks
Creating a queue using two stacks is a common problem that demonstrates the versatility of stack operations.
- Approach:
Use two stacks: one for enqueue operations and another for dequeue operations. - Tips:
Balance the operations to ensure efficient amortized performance.
Key Points:
- Time Complexity: O(n) worst-case for dequeue, amortized O(1)
- Space Complexity: O(n)
Bullet Points:
- Reinforces understanding of stack operations.
- Demonstrates data structure transformation.
- Practical for interviews focusing on problem-solving skills.
10. Solve the Two Sum Problem
The two sum problem is widely popular and tests both brute-force and optimized approaches using hash maps.
- Approach:
Use a hash map to check for the complement of each element. - Tips:
Handle cases where there are multiple valid answers by clarifying the problem constraints.
Key Points:
- Time Complexity: O(n)
- Space Complexity: O(n)
Bullet Points:
- Foundation for understanding hash-based searches.
- Frequently asked in technical interviews.
- Enhances algorithm optimization skills.
Also Read: Top 10 DSA Questions on Linked Lists and Arrays
Effective Strategies to Solve DSA Problems
Developing a robust strategy for solving DSA problems can make a significant difference during coding tests. By breaking down problems into smaller, manageable parts, you can approach each problem with a clear mind and a step-by-step plan.
Plan Your Approach
Before diving into the code, spend some time planning:
- Understand the Problem:
Read the question carefully and make sure you fully understand the requirements. - Identify the Data Structures:
Determine which data structures (arrays, linked lists, stacks, etc.) are most appropriate. - Outline a Strategy:
Draft a plan or pseudocode to structure your solution.
Practice Regularly
Consistency is key. Regular practice helps you to:
- Improve speed and accuracy:
Regularly solving problems increases your familiarity with common patterns and techniques. - Build intuition:
Over time, you will recognize which approaches work best for different types of problems. - Boost confidence:
Frequent practice reduces anxiety during real coding tests.
Bullet Points for Effective Strategies:
- Break problems into smaller parts.
- Write pseudocode before coding.
- Test edge cases and validate your solution

Table for Strategy Comparison:
Strategy | Benefits | Example Techniques |
Planning and Pseudocode | Clear structure, fewer errors | Flowcharts, step-by-step breakdown |
Consistent Practice | Faster problem recognition | Daily coding challenges, contests |
Peer Reviews and Discussion | Learn alternative approaches | Code reviews, study groups |
These strategies not only improve your ability to solve DSA problems but also prepare you for unexpected challenges during your coding tests.
Also Read: Top 20 Full Stack Developer Web Dev Questions
Common Pitfalls and How to Avoid Them in DSA Interviews
When preparing for DSA interviews, it is important to be aware of common mistakes that can cost you valuable time and points. Avoiding these pitfalls will help ensure that you present a polished, efficient solution during your test.
Typical Pitfalls
- Overcomplicating the Problem:
Many candidates overthink the solution, making it more complex than necessary. Stick to the simplest solution that meets the requirements. - Ignoring Edge Cases:
Failing to consider edge cases, such as empty inputs or very large inputs, can lead to errors. - Poor Time Management:
Spending too much time on one question might leave you with insufficient time for others. It is crucial to allocate your time wisely.
How to Overcome Them
- Simplify Your Approach:
Break down the problem into simpler parts and gradually build the solution. - Practice with a Timer:
Simulate test conditions by practicing under time constraints. - Review and Debug:
After writing the code, test with multiple cases and revise your solution if needed.
Bullet Points for Avoiding Pitfalls:
- Focus on clarity over cleverness.
- Regularly test edge cases.
- Practice solving problems under timed conditions.
Key Fact:
According to a survey on coding interviews by CodeSignal, over 70% of candidates faced challenges primarily due to poor time management and failure to account for edge cases.
Also Read: Top 10 System Design Interview Questions 2025
Resources and Practice Tips for Mastering DSA
Mastering DSA requires the right mix of theory, practice, and continuous feedback. Using a variety of resources can significantly boost your preparation and help you develop a well-rounded approach.
Recommended Resources
- Online Platforms:
Websites like LeetCode, HackerRank, and CodeChef offer a vast array of problems and contests. - Books and Tutorials:
Consider classic texts like “Cracking the Coding Interview” and online tutorials that break down complex topics. - Community Forums:
Join coding communities and discussion groups to exchange ideas and get feedback on your solutions.
Practice Tips
- Set Clear Goals:
Establish daily or weekly targets for solving problems. - Use a Timer:
Simulate real test conditions to improve speed and accuracy. - Peer Review:
Discuss your solutions with peers or mentors to identify areas of improvement.
Table for Resource Comparison:
Resource Type | Pros | Cons |
Online Platforms | Real-time feedback, variety of problems | May require premium subscription |
Books & Tutorials | In-depth theoretical knowledge | Can be outdated in fast-evolving fields |
Community Forums | Peer support and collaboration | Quality of advice may vary |
Remember, consistency and variety in your practice are key to becoming proficient in DSA. Regularly challenging yourself with new problems and engaging with the community will ensure steady improvement.
Also Read: Why System Design Interviews Are Tough
Frequently Asked Questions
What is the importance of DSA in coding tests?
DSA forms the backbone of most coding tests because it teaches you how to approach and solve problems efficiently. Knowing how to manage data and optimize algorithms can significantly reduce the time required to find a solution. Many experts agree that mastery of DSA concepts increases your chances of acing coding interviews. For more insights on courses that enhance these skills, check out our DSA course here.
How should I practice to improve my DSA skills?
Regular practice is essential for improving your DSA skills. Start by solving problems on reputable online platforms and then gradually increase the difficulty level as you become more confident. It’s also beneficial to review your solutions and understand alternate approaches. Consistent practice paired with peer feedback can accelerate your learning process. If you are interested in comprehensive learning, consider exploring our Web Development course here.
Can mastering DSA really help me in landing a job?
Yes, mastering DSA is one of the most important factors in succeeding in technical interviews. Many top tech companies rely on DSA questions to evaluate problem-solving skills and coding efficiency. Having a solid grasp of these concepts not only boosts your confidence but also makes you a strong candidate in a competitive job market. To dive deeper into mastering DSA alongside other skills, you might want to explore our combined Design and DSA course here.
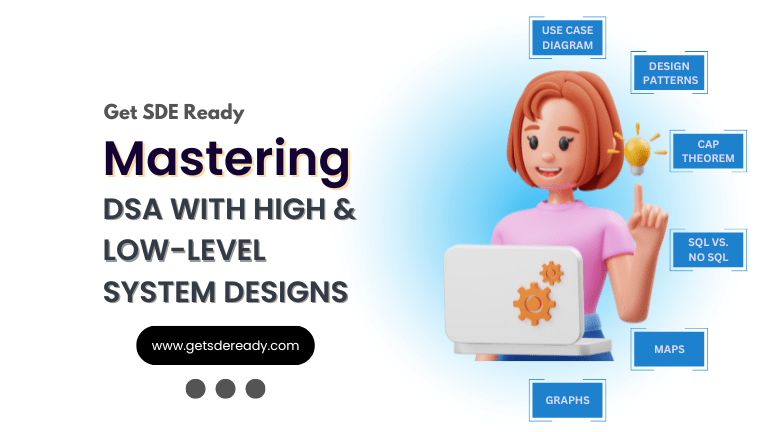
DSA, High & Low Level System Designs
- 85+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 400+ DSA Practice Questions
- Comprehensive Notes
- HackerRank Tests & Quizzes
- Topic-wise Quizzes
- Case Studies
- Access to Global Peer Community
Buy for 60% OFF
₹25,000.00 ₹9,999.00
Accelerate your Path to a Product based Career
Boost your career or get hired at top product-based companies by joining our expertly crafted courses. Gain practical skills and real-world knowledge to help you succeed.
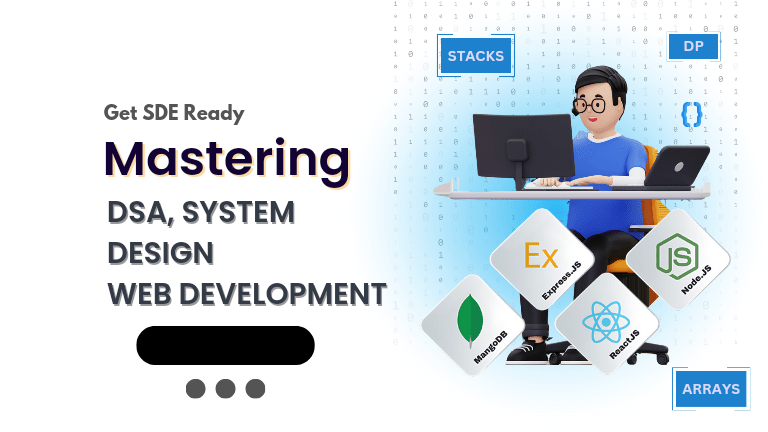
Fast-Track to Full Spectrum Software Engineering
- 120+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 400+ DSA Practice Questions
- Comprehensive Notes
- HackerRank Tests & Quizzes
- 12+ live Projects & Deployments
- Case Studies
- Access to Global Peer Community
Buy for 57% OFF
₹35,000.00 ₹14,999.00
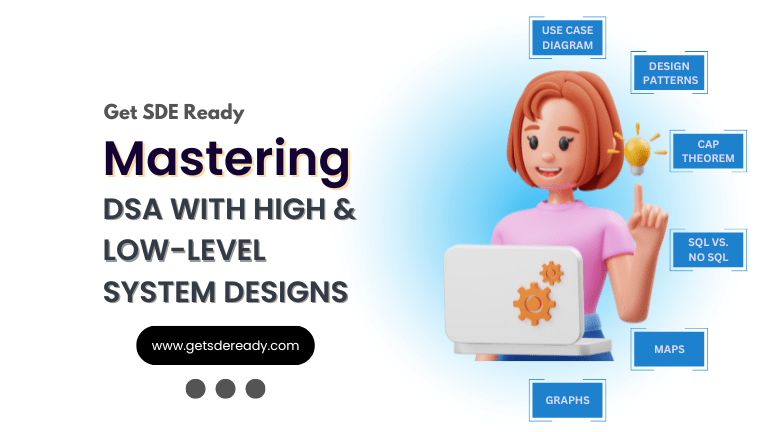
DSA, High & Low Level System Designs
- 85+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 400+ DSA Practice Questions
- Comprehensive Notes
- HackerRank Tests & Quizzes
- Topic-wise Quizzes
- Case Studies
- Access to Global Peer Community
Buy for 60% OFF
₹25,000.00 ₹9,999.00
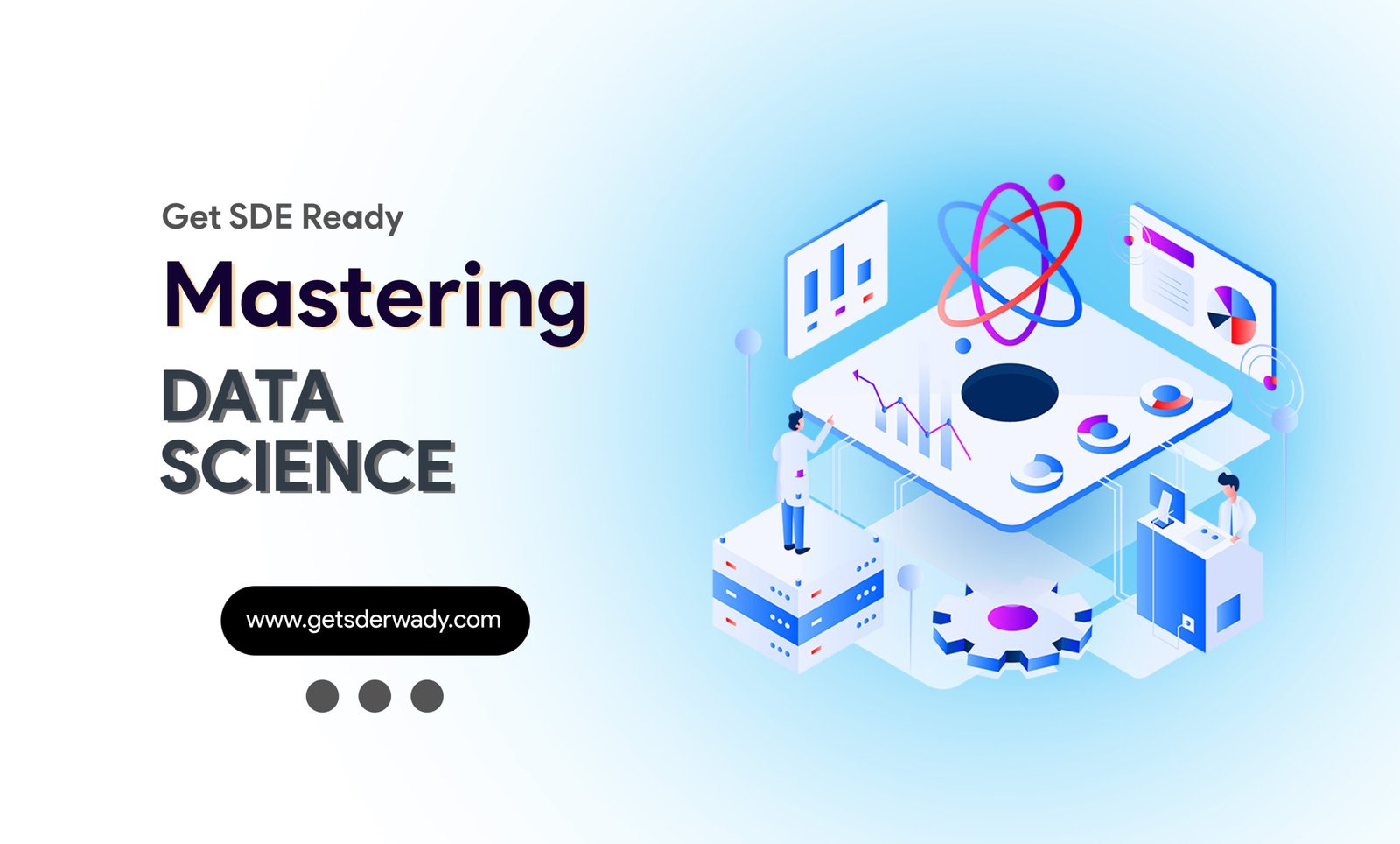
Essentials of Machine Learning and Artificial Intelligence
- 65+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 22+ Hands-on Live Projects & Deployments
- Comprehensive Notes
- Topic-wise Quizzes
- Case Studies
- Access to Global Peer Community
- Interview Prep Material
Buy for 65% OFF
₹20,000.00 ₹6,999.00

Low & High Level System Design
- 20+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 400+ DSA Practice Questions
- Comprehensive Notes
- HackerRank Tests
- Topic-wise Quizzes
- Access to Global Peer Community
- Interview Prep Material
Buy for 65% OFF
₹20,000.00 ₹6,999.00
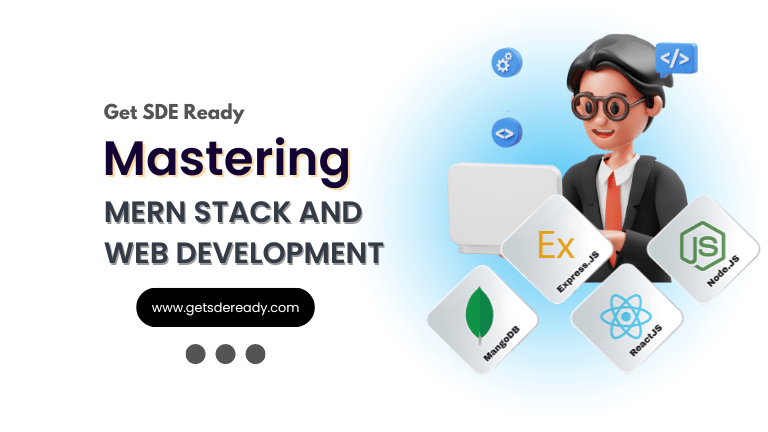
Mastering Mern Stack (WEB DEVELOPMENT)
- 65+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 12+ Hands-on Live Projects & Deployments
- Comprehensive Notes & Quizzes
- Real-world Tools & Technologies
- Access to Global Peer Community
- Interview Prep Material
- Placement Assistance
Buy for 60% OFF
₹15,000.00 ₹5,999.00

Mastering Data Structures & Algorithms
- 65+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 400+ DSA Practice Questions
- Comprehensive Notes
- HackerRank Tests
- Access to Global Peer Community
- Topic-wise Quizzes
- Interview Prep Material
Buy for 50% OFF
₹9,999.00 ₹4,999.00
Reach Out Now
If you have any queries, please fill out this form. We will surely reach out to you.
Contact Email
Reach us at the following email address.
arun@getsdeready.com
Phone Number
You can reach us by phone as well.
+91-97737 28034
Our Location
Rohini, Sector-3, Delhi-110085