Data Structures and Algorithms
- Introduction to Data Structures and Algorithms
- Time and Space Complexity Analysis
- Big-O, Big-Theta, and Big-Omega Notations
- Recursion and Backtracking
- Divide and Conquer Algorithm
- Dynamic Programming: Memoization vs. Tabulation
- Greedy Algorithms and Their Use Cases
- Understanding Arrays: Types and Operations
- Linear Search vs. Binary Search
- Sorting Algorithms: Bubble, Insertion, Selection, and Merge Sort
- QuickSort: Explanation and Implementation
- Heap Sort and Its Applications
- Counting Sort, Radix Sort, and Bucket Sort
- Hashing Techniques: Hash Tables and Collisions
- Open Addressing vs. Separate Chaining in Hashing
- DSA Questions for Beginners
- Advanced DSA Questions for Competitive Programming
- Top 10 DSA Questions to Crack Your Next Coding Test
- Top 50 DSA Questions Every Programmer Should Practice
- Top Atlassian DSA Interview Questions
- Top Amazon DSA Interview Questions
- Top Microsoft DSA Interview Questions
- Top Meta (Facebook) DSA Interview Questions
- Netflix DSA Interview Questions and Preparation Guide
- Top 20 DSA Interview Questions You Need to Know
- Top Uber DSA Interview Questions and Solutions
- Google DSA Interview Questions and How to Prepare
- Airbnb DSA Interview Questions and How to Solve Them
- Mobile App DSA Interview Questions and Solutions
DSA Interview Questions
- DSA Questions for Beginners
- Advanced DSA Questions for Competitive Programming
- Top 10 DSA Questions to Crack Your Next Coding Test
- Top 50 DSA Questions Every Programmer Should Practice
- Top Atlassian DSA Interview Questions
- Top Amazon DSA Interview Questions
- Top Microsoft DSA Interview Questions
- Top Meta (Facebook) DSA Interview Questions
- Netflix DSA Interview Questions and Preparation Guide
- Top 20 DSA Interview Questions You Need to Know
- Top Uber DSA Interview Questions and Solutions
- Google DSA Interview Questions and How to Prepare
- Airbnb DSA Interview Questions and How to Solve Them
- Mobile App DSA Interview Questions and Solutions
Introduction to High-Level System Design
System Design Fundamentals
- Functional vs. Non-Functional Requirements
- Scalability, Availability, and Reliability
- Latency and Throughput Considerations
- Load Balancing Strategies
Architectural Patterns
- Monolithic vs. Microservices Architecture
- Layered Architecture
- Event-Driven Architecture
- Serverless Architecture
- Model-View-Controller (MVC) Pattern
- CQRS (Command Query Responsibility Segregation)
Scaling Strategies
- Vertical Scaling vs. Horizontal Scaling
- Sharding and Partitioning
- Data Replication and Consistency Models
- Load Balancing Strategies
- CDN and Edge Computing
Database Design in HLD
- SQL vs. NoSQL Databases
- CAP Theorem and its Impact on System Design
- Database Indexing and Query Optimization
- Database Sharding and Partitioning
- Replication Strategies
API Design and Communication
Caching Strategies
- Types of Caching
- Cache Invalidation Strategies
- Redis vs. Memcached
- Cache-Aside, Write-Through, and Write-Behind Strategies
Message Queues and Event-Driven Systems
- Kafka vs. RabbitMQ vs. SQS
- Pub-Sub vs. Point-to-Point Messaging
- Handling Asynchronous Workloads
- Eventual Consistency in Distributed Systems
Security in System Design
Observability and Monitoring
- Logging Strategies (ELK Stack, Prometheus, Grafana)
- API Security Best Practices
- Secure Data Storage and Access Control
- DDoS Protection and Rate Limiting
Real-World System Design Case Studies
- Distributed locking (Locking and its Types)
- Memory leaks and Out of memory issues
- HLD of YouTube
- HLD of WhatsApp
System Design Interview Questions
- Adobe System Design Interview Questions
- Top Atlassian System Design Interview Questions
- Top Amazon System Design Interview Questions
- Top Microsoft System Design Interview Questions
- Top Meta (Facebook) System Design Interview Questions
- Top Netflix System Design Interview Questions
- Top Uber System Design Interview Questions
- Top Google System Design Interview Questions
- Top Apple System Design Interview Questions
- Top Airbnb System Design Interview Questions
- Top 10 System Design Interview Questions
- Mobile App System Design Interview Questions
- Top 20 Stripe System Design Interview Questions
- Top Shopify System Design Interview Questions
- Top 20 System Design Interview Questions
- Top Advanced System Design Questions
- Most-Frequented System Design Questions in Big Tech Interviews
- What Interviewers Look for in System Design Questions
- Critical System Design Questions to Crack Any Tech Interview
- Top 20 API Design Questions for System Design Interviews
- Top 10 Steps to Create a System Design Portfolio for Developers
Top Microsoft DSA Interview Questions and Tips for Success
Preparing for a Microsoft Data Structures and Algorithms (DSA) interview can feel overwhelming, but with the right strategy, you can stand out. Did you know that over 70% of candidates struggle with optimizing their code during technical interviews? To help you prepare, we’ve compiled the top Microsoft DSA interview questions and success strategies. Don’t forget to sign up for free course updates to stay ahead in your preparation!
Understanding Microsoft’s DSA Interview Structure
Microsoft’s DSA interviews test your problem-solving skills, coding efficiency, and ability to handle real-world scenarios. The process typically includes 2–3 coding rounds, each lasting 45–60 minutes.
Types of Questions Asked
Questions range from arrays and strings to advanced graph algorithms. For example:
- Arrays: Rotate matrices, find subarrays with specific sums.
- Trees: Validate BSTs, perform vertical traversals.
- Dynamic Programming: Solve knapsack or longest palindromic substring problems.
Evaluation Criteria
Candidates are graded on:
- Problem-Solving Approach: How logically you break down the problem.
- Code Efficiency: Optimal time and space complexity (e.g., O(n log n)).
- Communication: Explaining your thought process clearly.
Evaluation Aspect | Description | Weightage |
Problem-Solving | Logical breakdown and edge cases | 40% |
Coding Skills | Clean, efficient code | 35% |
Communication | Clarity in explaining solutions | 25% |
A Microsoft hiring manager once said, “We look for candidates who ask clarifying questions before jumping into code.”
Essential Tips for Acing the DSA Interview
Practice Smartly
Focus on high-frequency topics like trees, graphs, and DP. Platforms like LeetCode and CodeSignal offer Microsoft-specific question banks.
Master Time Management
- Spend 10 minutes understanding the problem.
- Write pseudocode before coding.
- Allocate 5–10 minutes for testing edge cases.

Top 20 Microsoft DSA Interview Questions
Arrays and Strings
1. Rotate a Matrix by 90 Degrees
- Approach: Layer-by-layer rotation with in-place swaps.
- Time Complexity: O(n²)
2. Longest Substring Without Repeating Characters
- Approach: Sliding window with hashmap for indices.
- Time Complexity: O(n)
3. Two Sum
- Approach: Hashmap to store complements.
- Time Complexity: O(n)
4. Maximum Subarray (Kadane’s Algorithm)
- Approach: Track current and global maxima.
- Time Complexity: O(n)
5. Merge Intervals
- Approach: Sort intervals and merge overlapping ones.
- Time Complexity: O(n log n)
Linked Lists
6. Reverse a Linked List
- Approach: Iterative three-pointer method.
- Time Complexity: O(n)
7. Detect Cycle in a Linked List
- Approach: Floyd’s Tortoise and Hare algorithm.
- Time Complexity: O(n)
8. Merge Two Sorted Lists
- Approach: Dummy node for streamlined merging.
- Time Complexity: O(n + m)
9. Remove Nth Node From End
- Approach: Two pointers with a gap of n nodes.
- Time Complexity: O(n)
Trees and Graphs
10. Validate a Binary Search Tree (BST)
- Approach: In-order traversal with bounds checking.
Time Complexity: O(n)
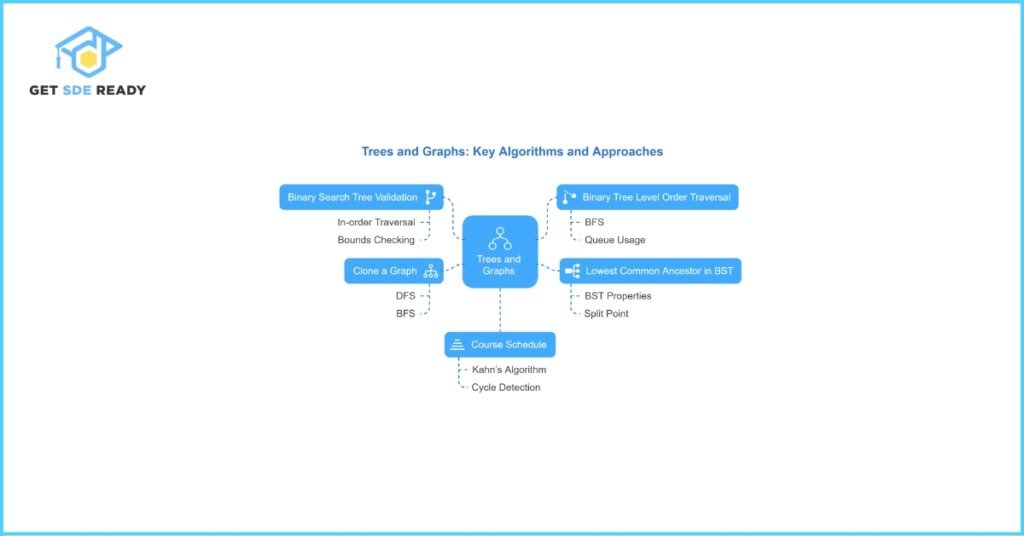
11. Binary Tree Level Order Traversal
- Approach: BFS using a queue.
- Time Complexity: O(n)
12. Lowest Common Ancestor (LCA) in a BST
- Approach: Exploit BST properties to find split point.
- Time Complexity: O(h)
13. Clone a Graph (DFS/BFS)
- Approach: Hashmap to map original and cloned nodes.
- Time Complexity: O(n)
14. Course Schedule (Topological Sorting)
- Approach: Detect cycles using Kahn’s algorithm.
- Time Complexity: O(n + e)
Dynamic Programming (DP)
15. 0/1 Knapsack Problem
- Approach: DP table with weight-value pairs.
- Time Complexity: O(nW)
16. Longest Palindromic Subsequence
- Approach: DP with substring expansion.
- Time Complexity: O(n²)
17. Climbing Stairs (Fibonacci)
- Approach: Bottom-up DP with memoization.
- Time Complexity: O(n)
18. Coin Change Problem
- Approach: DP array for minimum coins per amount.
- Time Complexity: O(n × amount)
Hash Tables and Heaps
19. Subarray Sum Equals K
- Approach: Prefix sum with hashmap.
- Time Complexity: O(n)
20. Find the Kth Largest Element
- Approach: Min-heap of size k.
- Time Complexity: O(n log k)
Common Mistakes to Avoid
Ignoring Edge Cases
Forgetting to test empty inputs or large datasets can cost you. For example, always check if a tree is empty before traversal.

Overcomplicating Solutions
Aim for simplicity. If your code has nested loops, ask: “Can this be done with a hashmap or two pointers?”
Resources for Preparation
Online Platforms
- LeetCode: Filter questions by Microsoft tag.
- GeeksforGeeks: Detailed explanations for 500+ DSA problems.
Books
- Cracking the Coding Interview by Gayle Laakmann McDowell.
- Introduction to Algorithms by Cormen (for theory).
Comparison of Approaches for Key Problems
Problem | Optimal Approach | Time Complexity |
Two Sum | Hashmap | O(n) |
Detect Cycle in Linked List | Floyd’s Algorithm | O(n) |
Validate BST | In-order Traversal | O(n) |
Coin Change | Dynamic Programming | O(n × amount) |
FAQ Section
Which topics are most frequent in Microsoft DSA interviews?
Arrays, strings, trees, and dynamic programming appear in 80% of interviews. Strengthen these areas with our DSA & Web Dev Combined Course.
How important is code optimization?
Critical! Microsoft evaluates solutions for O(n) or O(n log n) time. Practice optimizing nested loops.
Should I focus on theory or coding?
Balance both. Understand Big-O notation but prioritize writing clean code. Use our Master DSA, Web Dev & System Design for end-to-end prep.
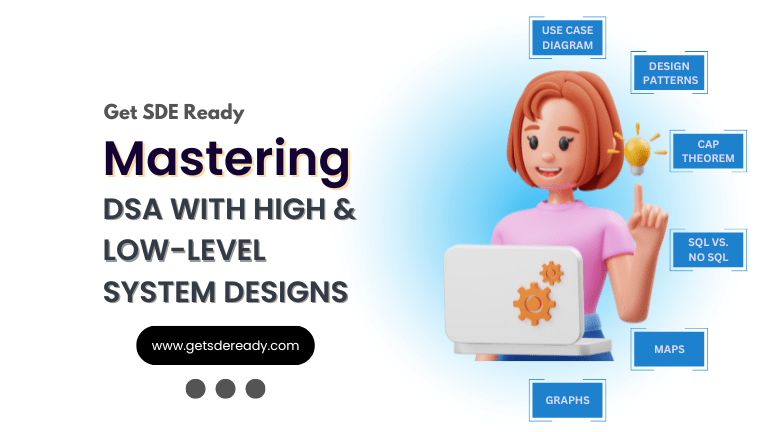
DSA, High & Low Level System Designs
- 85+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 400+ DSA Practice Questions
- Comprehensive Notes
- HackerRank Tests & Quizzes
- Topic-wise Quizzes
- Case Studies
- Access to Global Peer Community
Buy for 60% OFF
₹25,000.00 ₹9,999.00
Accelerate your Path to a Product based Career
Boost your career or get hired at top product-based companies by joining our expertly crafted courses. Gain practical skills and real-world knowledge to help you succeed.
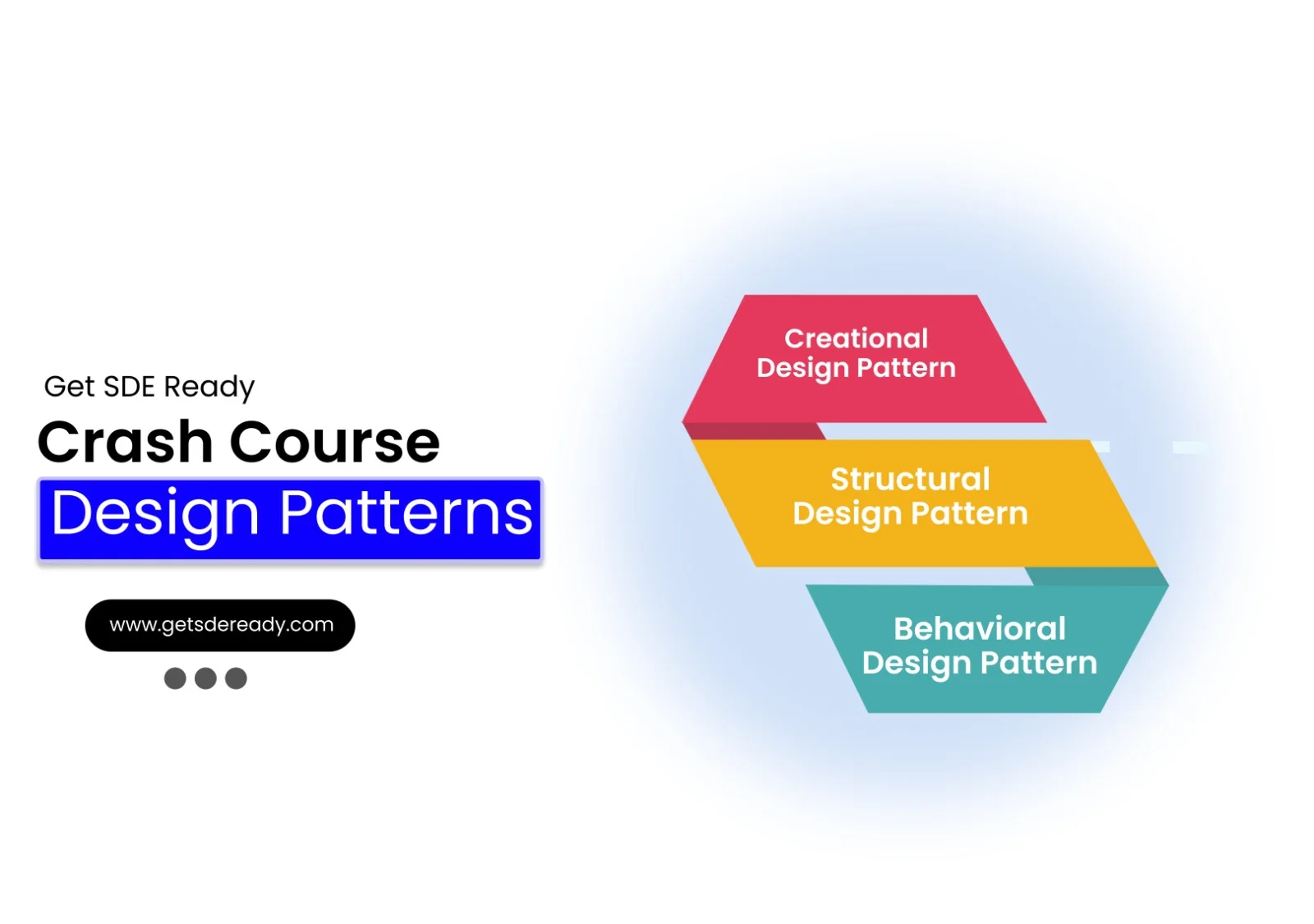
Design Patterns Bootcamp
- Live Classes & Recordings
- 24/7 Live Doubt Support
- Practice Questions
- Case Studies
- Access to Global Peer Community
- Topic wise Quizzes
- Referrals
- Certificate of Completion
Buy for 50% OFF
₹2,000.00 ₹999.00

ML & AI Kickstart
- Live Classes & Recordings
- 24/7 Live Doubt Support
- 2 Live Projects
- Case Studies
- Topic wise Quizzes
- Access to Global Peer Community
- Certificate of Completion
- Referrals
Buy for 50% OFF
₹2,000.00 ₹999.00
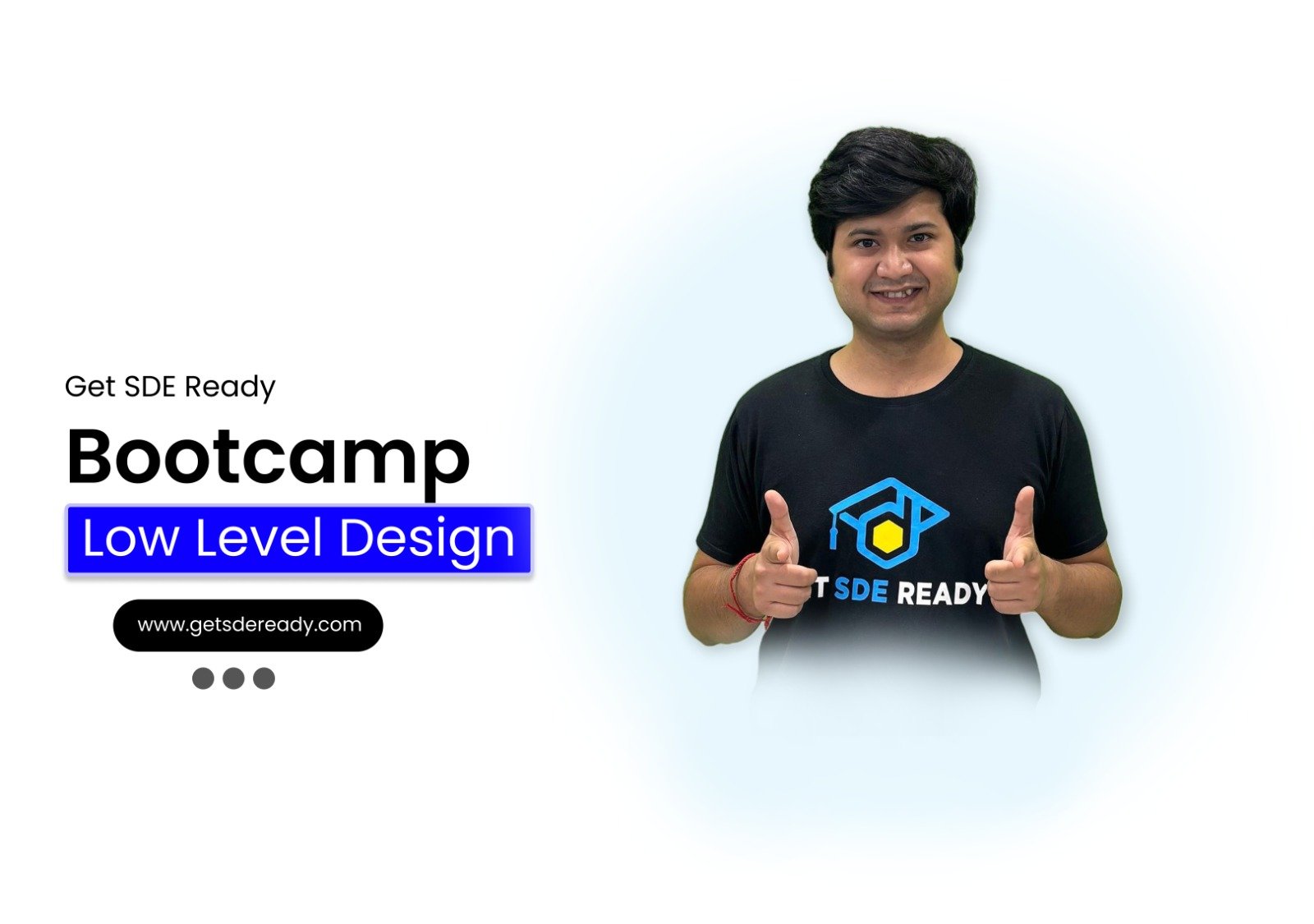
LLD Bootcamp
- 7+ Live Classes & Recordings
- Practice Questions
- 24/7 Live Doubt Support
- Case Studies
- Topic wise Quizzes
- Access to Global Peer Community
- Certificate of Completion
- Referrals
Buy for 50% OFF
₹2,000.00 ₹999.00
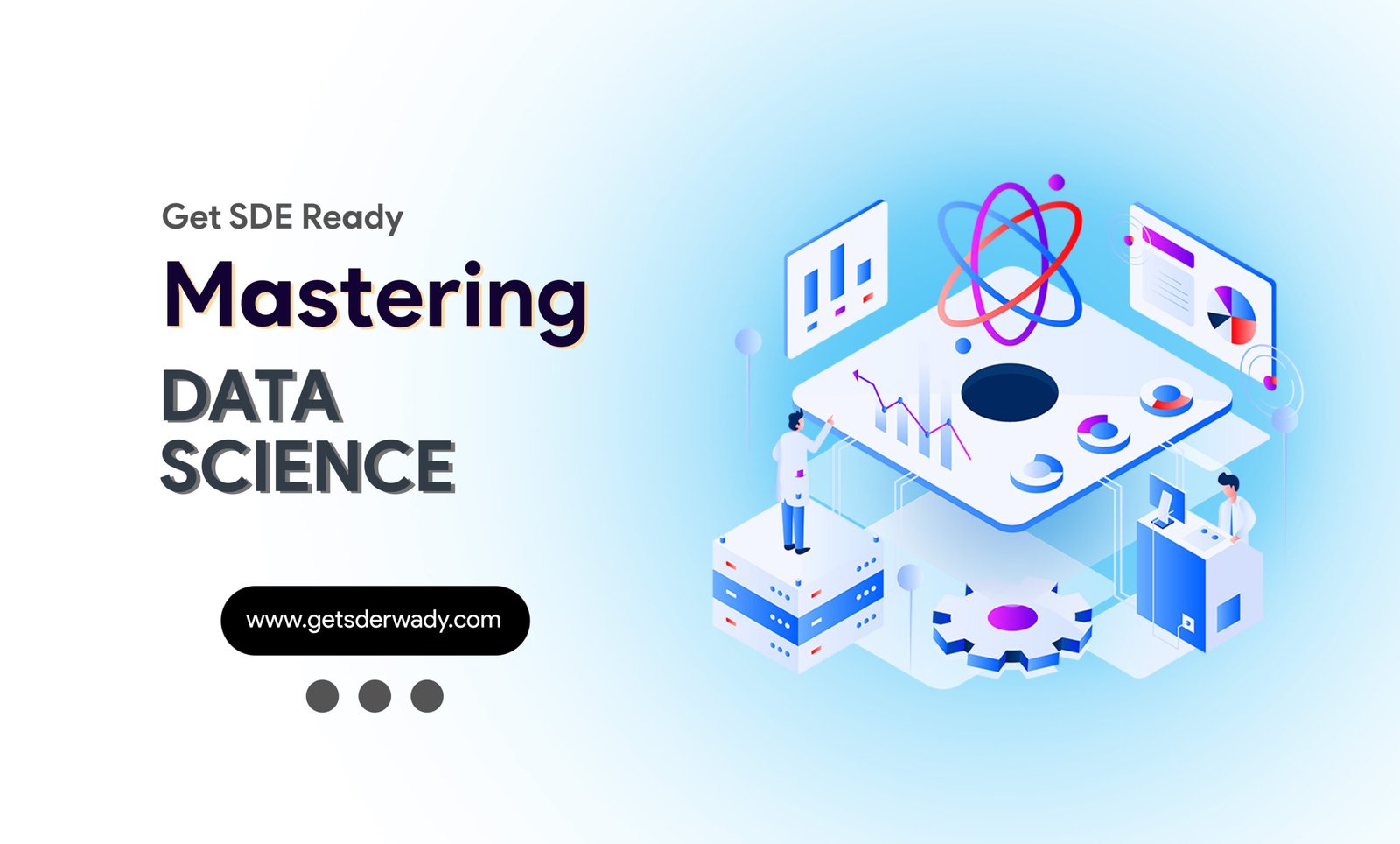
Essentials of Machine Learning and Artificial Intelligence
- 65+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 22+ Hands-on Live Projects & Deployments
- Comprehensive Notes
- Topic-wise Quizzes
- Case Studies
- Access to Global Peer Community
- Interview Prep Material
Buy for 65% OFF
₹20,000.00 ₹6,999.00
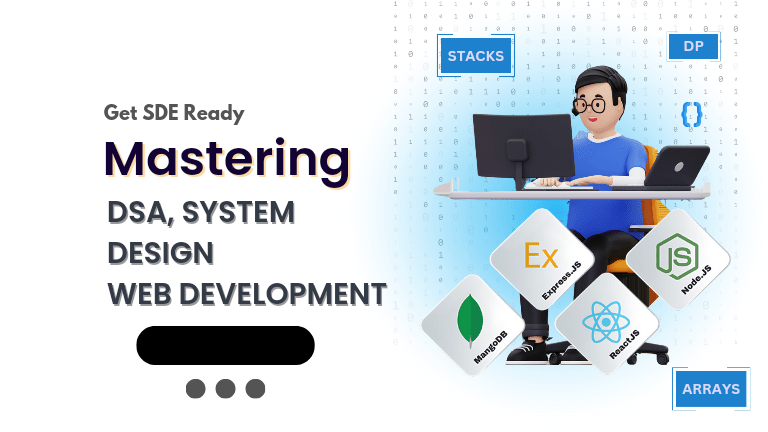
Fast-Track to Full Spectrum Software Engineering
- 120+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 400+ DSA Practice Questions
- Comprehensive Notes
- HackerRank Tests & Quizzes
- 12+ live Projects & Deployments
- Case Studies
- Access to Global Peer Community
Buy for 57% OFF
₹35,000.00 ₹14,999.00
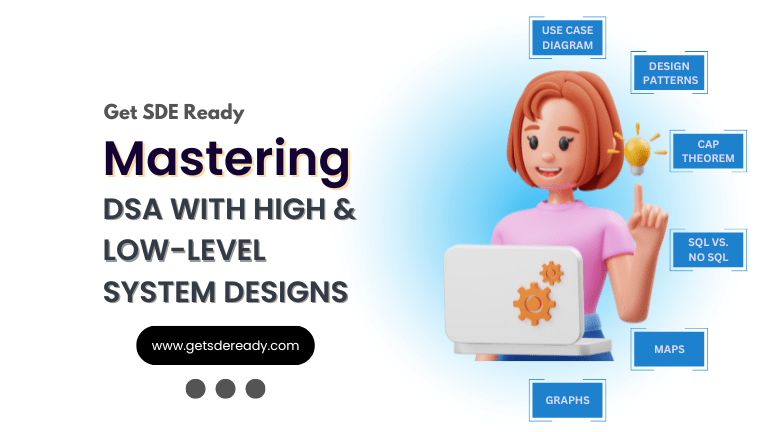
DSA, High & Low Level System Designs
- 85+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 400+ DSA Practice Questions
- Comprehensive Notes
- HackerRank Tests & Quizzes
- Topic-wise Quizzes
- Case Studies
- Access to Global Peer Community
Buy for 60% OFF
₹25,000.00 ₹9,999.00
Reach Out Now
If you have any queries, please fill out this form. We will surely reach out to you.
Contact Email
Reach us at the following email address.
Phone Number
You can reach us by phone as well.
+91-97737 28034
Our Location
Rohini, Sector-3, Delhi-110085