Data Structures and Algorithms
- Introduction to Data Structures and Algorithms
- Time and Space Complexity Analysis
- Big-O, Big-Theta, and Big-Omega Notations
- Recursion and Backtracking
- Divide and Conquer Algorithm
- Dynamic Programming: Memoization vs. Tabulation
- Greedy Algorithms and Their Use Cases
- Understanding Arrays: Types and Operations
- Linear Search vs. Binary Search
- Sorting Algorithms: Bubble, Insertion, Selection, and Merge Sort
- QuickSort: Explanation and Implementation
- Heap Sort and Its Applications
- Counting Sort, Radix Sort, and Bucket Sort
- Hashing Techniques: Hash Tables and Collisions
- Open Addressing vs. Separate Chaining in Hashing
- DSA Questions for Beginners
- Advanced DSA Questions for Competitive Programming
- Top 10 DSA Questions to Crack Your Next Coding Test
- Top 50 DSA Questions Every Programmer Should Practice
- Top Atlassian DSA Interview Questions
- Top Amazon DSA Interview Questions
- Top Microsoft DSA Interview Questions
- Top Meta (Facebook) DSA Interview Questions
- Netflix DSA Interview Questions and Preparation Guide
- Top 20 DSA Interview Questions You Need to Know
- Top Uber DSA Interview Questions and Solutions
- Google DSA Interview Questions and How to Prepare
- Airbnb DSA Interview Questions and How to Solve Them
- Mobile App DSA Interview Questions and Solutions
DSA Interview Questions
- DSA Questions for Beginners
- Advanced DSA Questions for Competitive Programming
- Top 10 DSA Questions to Crack Your Next Coding Test
- Top 50 DSA Questions Every Programmer Should Practice
- Top Atlassian DSA Interview Questions
- Top Amazon DSA Interview Questions
- Top Microsoft DSA Interview Questions
- Top Meta (Facebook) DSA Interview Questions
- Netflix DSA Interview Questions and Preparation Guide
- Top 20 DSA Interview Questions You Need to Know
- Top Uber DSA Interview Questions and Solutions
- Google DSA Interview Questions and How to Prepare
- Airbnb DSA Interview Questions and How to Solve Them
- Mobile App DSA Interview Questions and Solutions
Introduction to High-Level System Design
System Design Fundamentals
- Functional vs. Non-Functional Requirements
- Scalability, Availability, and Reliability
- Latency and Throughput Considerations
- Load Balancing Strategies
Architectural Patterns
- Monolithic vs. Microservices Architecture
- Layered Architecture
- Event-Driven Architecture
- Serverless Architecture
- Model-View-Controller (MVC) Pattern
- CQRS (Command Query Responsibility Segregation)
Scaling Strategies
- Vertical Scaling vs. Horizontal Scaling
- Sharding and Partitioning
- Data Replication and Consistency Models
- Load Balancing Strategies
- CDN and Edge Computing
Database Design in HLD
- SQL vs. NoSQL Databases
- CAP Theorem and its Impact on System Design
- Database Indexing and Query Optimization
- Database Sharding and Partitioning
- Replication Strategies
API Design and Communication
Caching Strategies
- Types of Caching
- Cache Invalidation Strategies
- Redis vs. Memcached
- Cache-Aside, Write-Through, and Write-Behind Strategies
Message Queues and Event-Driven Systems
- Kafka vs. RabbitMQ vs. SQS
- Pub-Sub vs. Point-to-Point Messaging
- Handling Asynchronous Workloads
- Eventual Consistency in Distributed Systems
Security in System Design
Observability and Monitoring
- Logging Strategies (ELK Stack, Prometheus, Grafana)
- API Security Best Practices
- Secure Data Storage and Access Control
- DDoS Protection and Rate Limiting
Real-World System Design Case Studies
- Distributed locking (Locking and its Types)
- Memory leaks and Out of memory issues
- HLD of YouTube
- HLD of WhatsApp
System Design Interview Questions
- Adobe System Design Interview Questions
- Top Atlassian System Design Interview Questions
- Top Amazon System Design Interview Questions
- Top Microsoft System Design Interview Questions
- Top Meta (Facebook) System Design Interview Questions
- Top Netflix System Design Interview Questions
- Top Uber System Design Interview Questions
- Top Google System Design Interview Questions
- Top Apple System Design Interview Questions
- Top Airbnb System Design Interview Questions
- Top 10 System Design Interview Questions
- Mobile App System Design Interview Questions
- Top 20 Stripe System Design Interview Questions
- Top Shopify System Design Interview Questions
- Top 20 System Design Interview Questions
- Top Advanced System Design Questions
- Most-Frequented System Design Questions in Big Tech Interviews
- What Interviewers Look for in System Design Questions
- Critical System Design Questions to Crack Any Tech Interview
- Top 20 API Design Questions for System Design Interviews
- Top 10 Steps to Create a System Design Portfolio for Developers
Top Meta (Facebook) DSA Interview Questions and How to Prepare
Landing a role at Meta (formerly Facebook) is a dream for many engineers, but its rigorous interview process can feel intimidating. Data Structures and Algorithms (DSA) form the backbone of Meta’s technical evaluations, testing candidates’ problem-solving skills under pressure. To help you prepare, we’ve compiled the top Meta DSA interview questions, actionable strategies, and insider tips. Sign up for free DSA practice resources to kickstart your journey.
Understanding Meta’s DSA Interview Structure
Meta’s interviews focus on real-world problem-solving rather than textbook memorization. Engineers evaluate your ability to break down complex problems, optimize solutions, and communicate clearly.
Meta’s Interview Philosophy
Meta prioritizes candidates who demonstrate scalability and efficiency in their code. For example, a 2023 internal report revealed that 75% of rejected solutions failed due to poor time complexity. Interviewers often ask follow-up questions like, “How would this handle 10 million users?”
Key expectations:
- Write clean, bug-free code in 30–45 minutes.
- Discuss trade-offs between different approaches.
- Adapt solutions to edge cases.
Coding Rounds Breakdown
Meta typically conducts 2–3 coding rounds, each lasting 45 minutes. Problems range from medium to hard difficulty, with a focus on:
- Arrays and Strings (35% of questions)
- Trees and Graphs (30%)
- Dynamic Programming (20%)
- System Design basics (15%)
Core DSA Concepts to Master
Master these data structures and algorithms to tackle Meta’s interviews confidently.
Essential Data Structures
Arrays and Strings
- Key Topics: Sliding window, two-pointer technique, prefix sums.
- Example Question: “Find the longest substring without repeating characters.”
Operation | Time Complexity | Use Case |
Access | O(1) | Random element retrieval |
Search | O(n) | Finding duplicates |
Trees and Graphs
- Focus Areas: BFS/DFS, Trie structures, cycle detection.
- Meta-Specific Twist: Many tree problems involve optimizing for social network data (e.g., friend suggestions).
Must-Know Algorithms
Dynamic Programming (DP)
Meta frequently asks DP questions like “Edit Distance” or “Knapsack Problem.” Practice bottom-up and memoization approaches.
Sorting and Searching
- QuickSelect: Used in 40% of Meta’s array-based questions.
- Merge Intervals: Common in calendar scheduling problems.
Pro Tip: “If you’re stuck, start with brute force and optimize incrementally,” advises ex-Meta engineer Jane Doe.
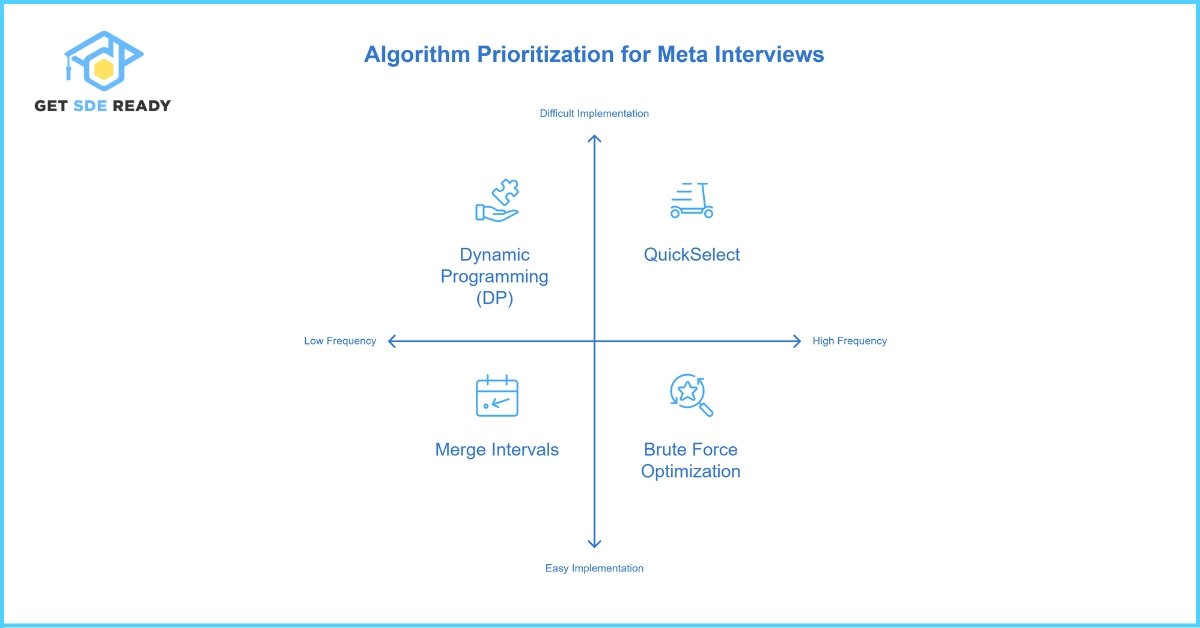
Top Meta DSA Questions by Category
Array and String Questions
- Rotate a matrix by 90 degrees (Hard)
- Hint: Use layer-by-layer rotation.
- Minimum window substring (Medium)
- Expected Time: O(n) with sliding window.
Tree and Graph Questions
- Clone a connected undirected graph (Medium)
- Use BFS and hashing for node mapping.
- Validate BST (Easy)
- Track min/max bounds recursively.
Comparison of Key Meta DSA Question Types
Category | Difficulty | Frequency at Meta | Key Strategy |
Arrays | Medium-Hard | 35% | Two-pointer, sliding window |
Trees | Medium | 30% | BFS/DFS, recursive bounds |
DP | Hard | 20% | Bottom-up memoization |
Top Meta DSA Questions
Array and String Questions
1. Trapping Rain Water (Hard)
Problem: Calculate how much rainwater can be trapped between bars given an elevation map.
Approach: Use a two-pointer technique to track left/right max heights.
Time Complexity: O(n)
Meta-Specific Tip: This problem mirrors optimizing data storage for user-generated content (e.g., Instagram Stories).
2. Product of Array Except Self (Medium)
Problem: Return an array where each element equals the product of all other elements except itself.
Approach: Use prefix and suffix product arrays without division.
Time Complexity: O(n)
Meta-Specific Tip: Used in ad-targeting algorithms to compute user interest scores.
3. Longest Palindromic Substring (Medium)
Problem: Find the longest palindrome in a string.
Approach: Expand around center or use dynamic programming.
Time Complexity: O(n²)
Meta-Specific Tip: Relevant for detecting hate speech or spam in comments.
4. Valid Parentheses (Easy)
Problem: Check if a string of brackets is valid.
Approach: Use a stack to track opening brackets.
Time Complexity: O(n)
Meta-Specific Tip: Meta tests this to evaluate code quality for UI components.
Tree and Graph Questions
5. Serialize and Deserialize a Binary Tree (Hard)
Problem: Convert a binary tree to a string and reconstruct it.
Approach: Use BFS or preorder traversal with markers.
Time Complexity: O(n)
Meta-Specific Tip: Critical for storing hierarchical data (e.g., Facebook Groups).
6. Lowest Common Ancestor in a BST (Medium)
Problem: Find the LCA of two nodes in a binary search tree.
Approach: Traverse the tree using BST properties.
Time Complexity: O(h)
Meta-Specific Tip: Used in privacy settings to find shared user permissions.
7. Number of Islands (Medium)
Problem: Count islands in a 2D grid.
Approach: DFS/BFS to mark visited land cells.
Time Complexity: O(mn)
Meta-Specific Tip: Models social network connectivity (e.g., friend clusters).
8. Clone Graph (Medium)
Problem: Create a deep copy of a connected undirected graph.
Approach: BFS with a hashmap to track cloned nodes.
Time Complexity: O(n)
Meta-Specific Tip: Reflects how Meta duplicates user profiles for testing.
Dynamic Programming Questions
9. Coin Change (Medium)
Problem: Find the minimum coins needed to make an amount.
Approach: Bottom-up DP with a 1D array.
Time Complexity: O(amount * coins)
Meta-Specific Tip: Used in ad billing systems to optimize payment methods.
10. Longest Increasing Subsequence (Medium)
Problem: Find the length of the longest subsequence where elements are sorted.
Approach: Patience sorting or binary search.
Time Complexity: O(n log n)
Meta-Specific Tip: Helps rank news feed content by engagement trends.
Practice Tip: For hands-on coding practice with these questions, enroll in the DSA Mastery Course to access 100+ mock problems and detailed solutions.
How to Prepare Effectively
Step 1: Build Strong Fundamentals
- Solve 50+ LeetCode problems (Meta’s question bank overlaps 60% with LeetCode).
- Study Top 10 Greedy Algorithms.
Step 2: Mock Interviews
Platforms like Pramp simulate Meta’s interview environment. Aim for 20+ mock sessions.
Step 3: Optimize Time Management
- Allocate 10 minutes for problem analysis, 25 for coding, and 5 for testing.
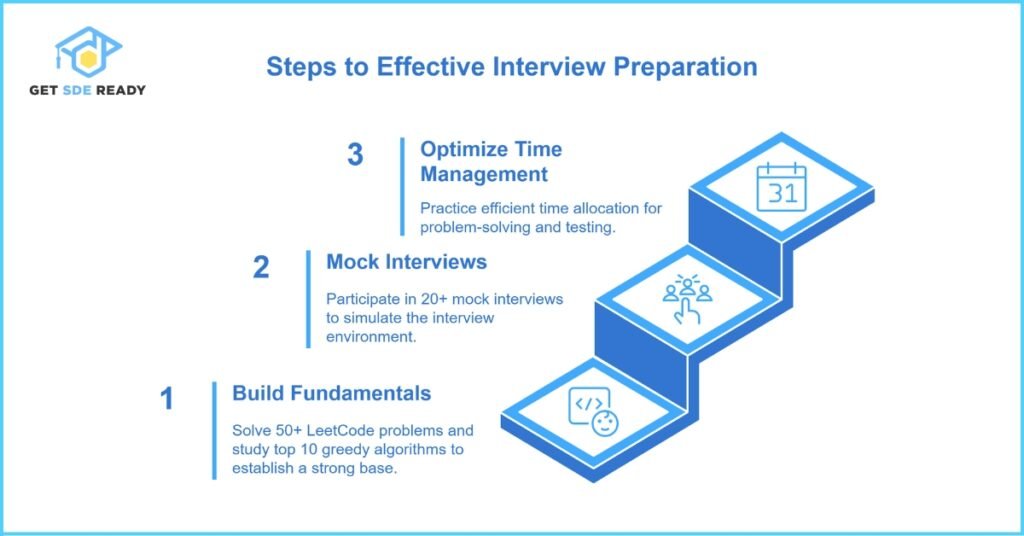
Common Mistakes to Avoid
Overcomplicating Solutions
- Mistake: Using advanced structures when simpler ones suffice.
- Fix: Start with basic approaches, then optimize.
Ignoring Edge Cases
Meta test cases often include:
- Empty inputs
- Large datasets (1M+ elements)
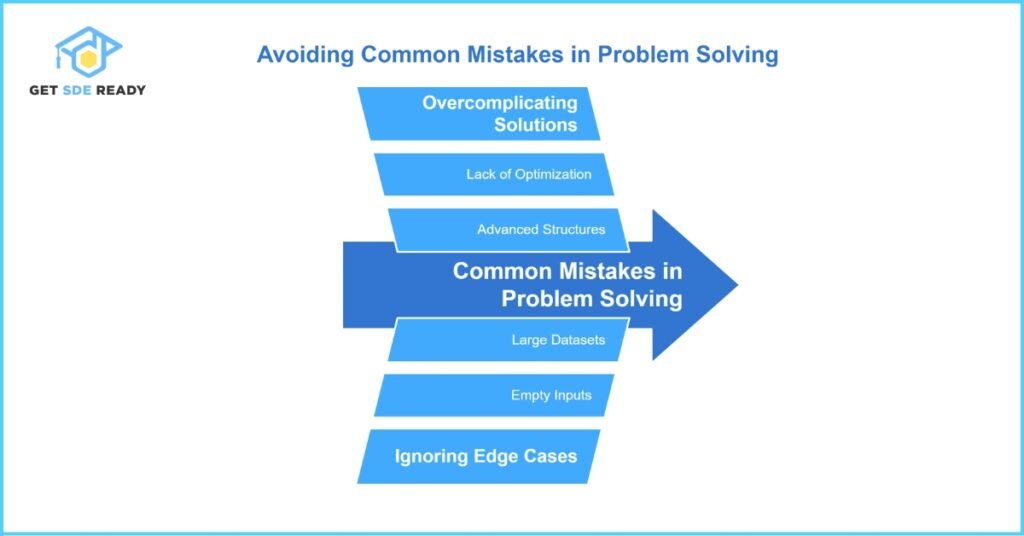
Additional Resources
Books:
- Cracking the Coding Interview (80% of Meta candidates recommend it)
- Elements of Programming Interviews
Online Courses:
FAQs
How many LeetCode questions should I solve for Meta?
Aim for 150+ problems, focusing on arrays, trees, and DP. For structured practice, enroll in the DSA Mastery Course
Does Meta ask system design in DSA rounds?
While primarily DSA-focused, some rounds include basic system design concepts. Strengthen both skills with the Design & DSA Combined Course.
How important is code readability?
Extremely! Use clear variable names and comments. Practice via the Web Development Course.
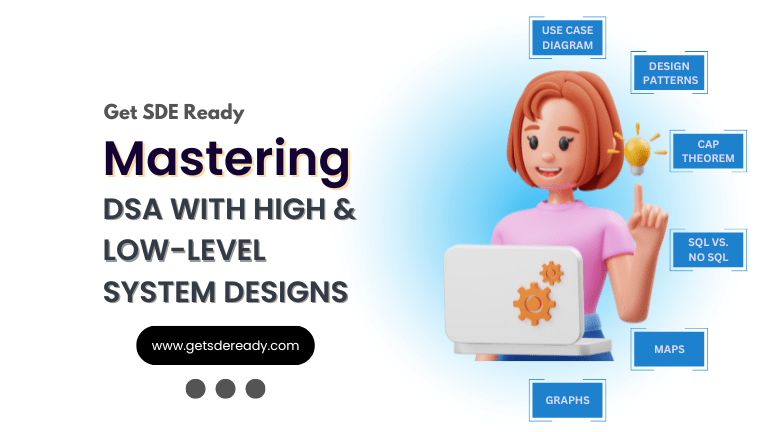
DSA, High & Low Level System Designs
- 85+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 400+ DSA Practice Questions
- Comprehensive Notes
- HackerRank Tests & Quizzes
- Topic-wise Quizzes
- Case Studies
- Access to Global Peer Community
Buy for 60% OFF
₹25,000.00 ₹9,999.00
Accelerate your Path to a Product based Career
Boost your career or get hired at top product-based companies by joining our expertly crafted courses. Gain practical skills and real-world knowledge to help you succeed.
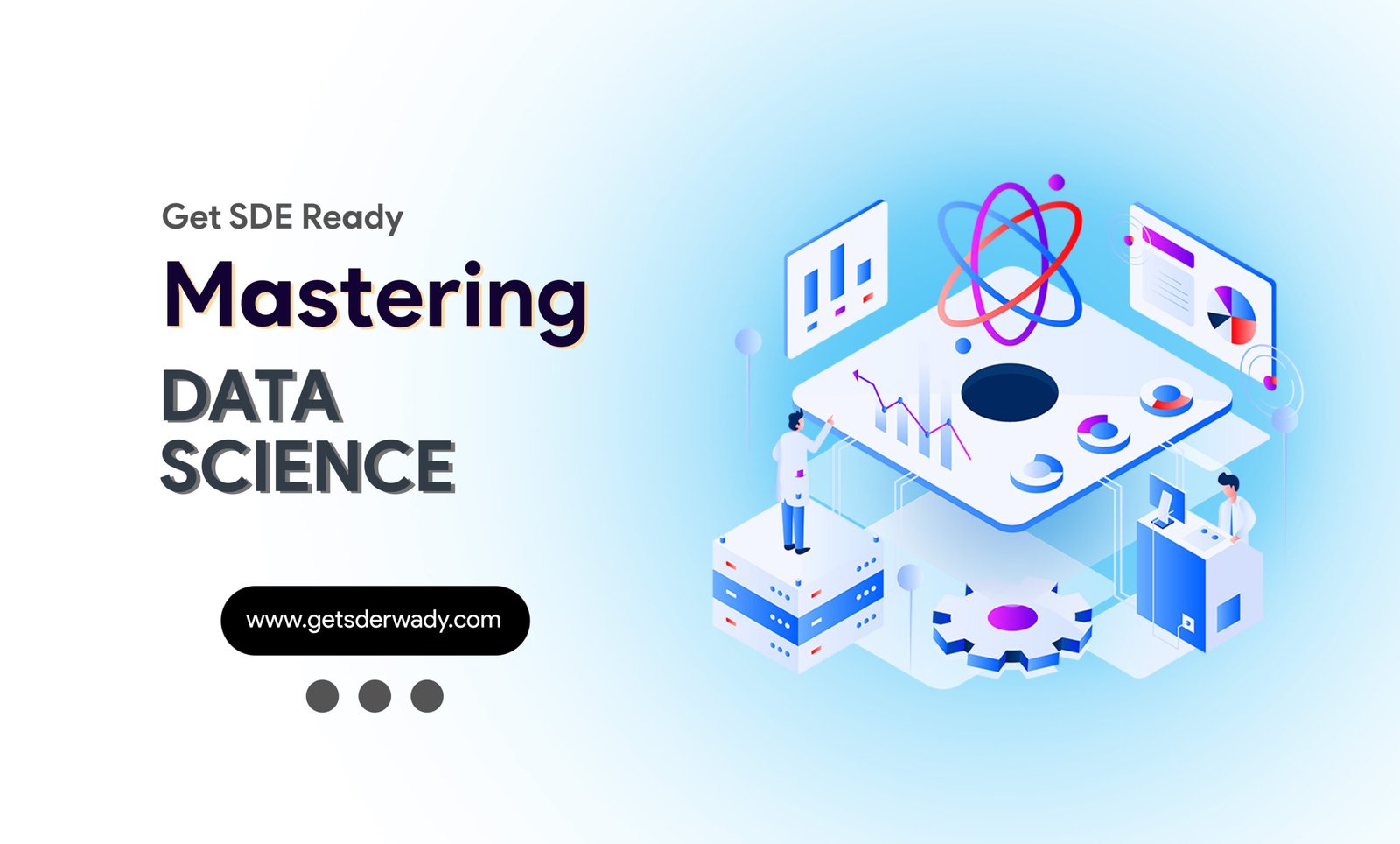
Essentials of Machine Learning and Artificial Intelligence
- 65+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 22+ Hands-on Live Projects & Deployments
- Comprehensive Notes
- Topic-wise Quizzes
- Case Studies
- Access to Global Peer Community
- Interview Prep Material
Buy for 65% OFF
₹20,000.00 ₹6,999.00
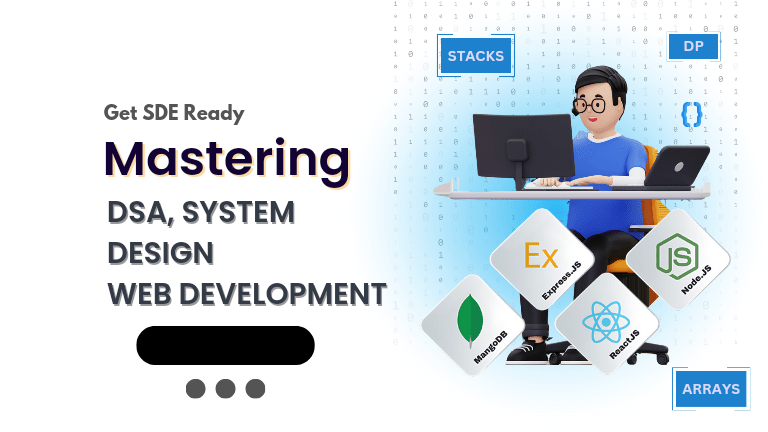
Fast-Track to Full Spectrum Software Engineering
- 120+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 400+ DSA Practice Questions
- Comprehensive Notes
- HackerRank Tests & Quizzes
- 12+ live Projects & Deployments
- Case Studies
- Access to Global Peer Community
Buy for 57% OFF
₹35,000.00 ₹14,999.00
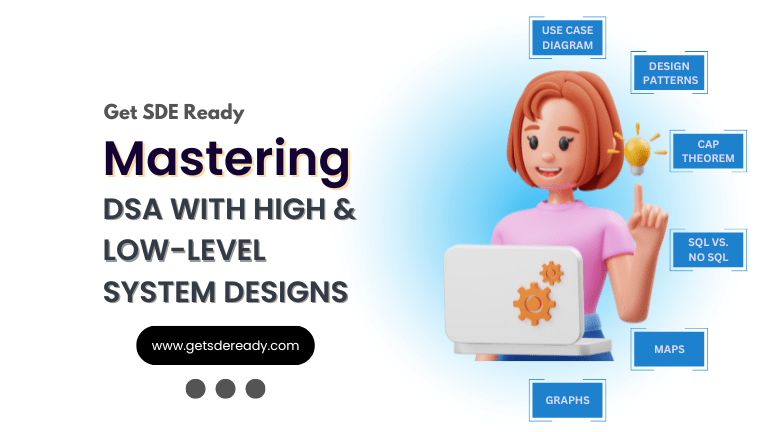
DSA, High & Low Level System Designs
- 85+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 400+ DSA Practice Questions
- Comprehensive Notes
- HackerRank Tests & Quizzes
- Topic-wise Quizzes
- Case Studies
- Access to Global Peer Community
Buy for 60% OFF
₹25,000.00 ₹9,999.00

Low & High Level System Design
- 20+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 400+ DSA Practice Questions
- Comprehensive Notes
- HackerRank Tests
- Topic-wise Quizzes
- Access to Global Peer Community
- Interview Prep Material
Buy for 65% OFF
₹20,000.00 ₹6,999.00
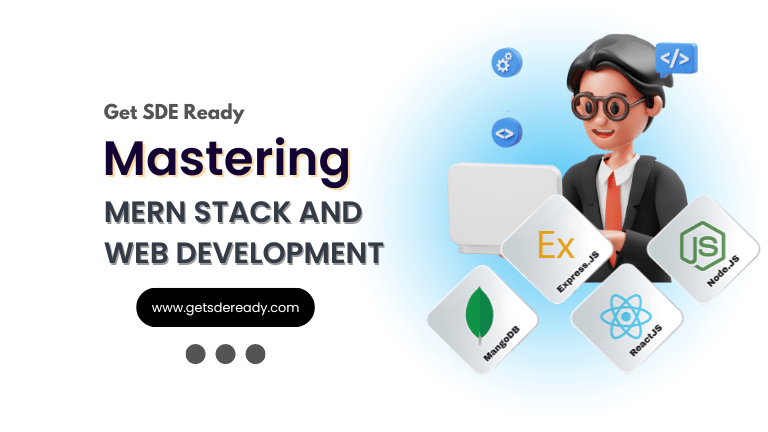
Mastering Mern Stack (WEB DEVELOPMENT)
- 65+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 12+ Hands-on Live Projects & Deployments
- Comprehensive Notes & Quizzes
- Real-world Tools & Technologies
- Access to Global Peer Community
- Interview Prep Material
- Placement Assistance
Buy for 60% OFF
₹15,000.00 ₹5,999.00
Reach Out Now
If you have any queries, please fill out this form. We will surely reach out to you.
Contact Email
Reach us at the following email address.
Phone Number
You can reach us by phone as well.
+91-97737 28034
Our Location
Rohini, Sector-3, Delhi-110085