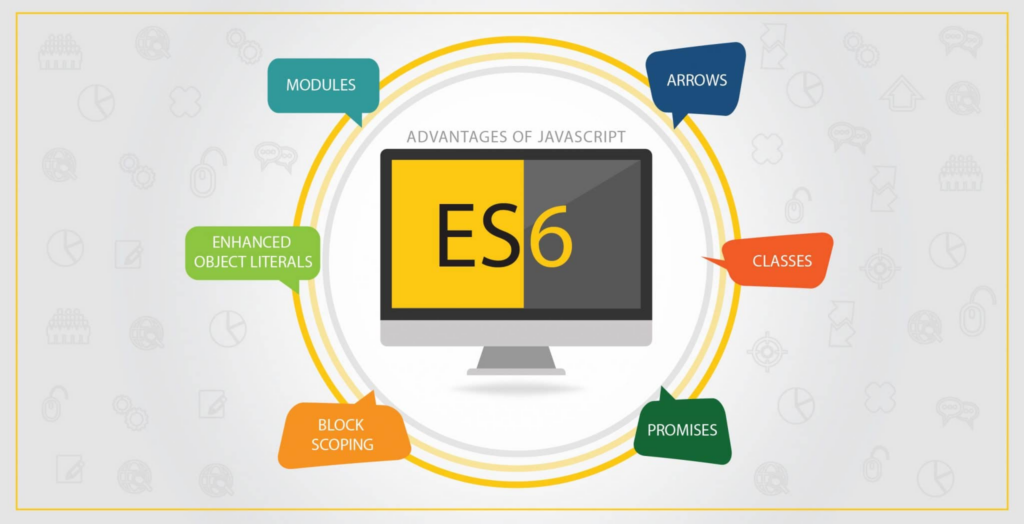
JavaScript has come a long way since its inception, evolving through various iterations to become a powerful and versatile language. One of the most significant updates in its history is the introduction of ECMAScript 2015, commonly known as ES6. This version brought a multitude of new features and improvements, making JavaScript more efficient, readable, and developer-friendly. Let’s explore the key features and benefits of ES6 that have transformed the landscape of modern JavaScript development.
1. Block-Scoped Variables: Let and Const
Before ES6, JavaScript had only function-scoped variables using var
. ES6 introduced let
and const
for block-scoped variables, providing more control over variable scope and preventing issues like variable hoisting and redeclaration.
{
let x = 10;
const y = 20;
console.log(x, y); // Output: 10, 20
}
console.log(x); // ReferenceError: x is not defined
console.log(y); // ReferenceError: y is not defined
2. Arrow Functions
Arrow functions offer a more concise syntax for writing function expressions and lexically bind the this
value, making them especially useful for callbacks and methods.
const add = (a, b) => a + b;
console.log(add(2, 3)); // Output: 5
3. Template Literals
Template literals provide a convenient way to create multi-line strings and include expressions within strings using backticks (`
) and ${}
syntax.
const name = 'Alice';
const greeting = `Hello, ${name}!`;
console.log(greeting); // Output: Hello, Alice!
4. Default Parameters
ES6 allows functions to have default parameter values, making it easier to handle optional parameters.
function greet(name = 'Guest') {
return `Hello, ${name}!`;
}
console.log(greet()); // Output: Hello, Guest!
console.log(greet('Bob')); // Output: Hello, Bob!
5. Destructuring Assignment
Destructuring assignment enables the extraction of values from arrays or properties from objects into distinct variables in a more readable and concise manner.
const person = { name: 'Alice', age: 25 };
const { name, age } = person;
console.log(name, age); // Output: Alice 25
const numbers = [1, 2, 3];
const [first, second] = numbers;
console.log(first, second); // Output: 1 2
6. Spread and Rest Operators
The spread operator (...
) allows the expansion of iterable elements (like arrays) into individual elements, while the rest operator collects multiple elements into an array.
const arr1 = [1, 2, 3];
const arr2 = [...arr1, 4, 5];
console.log(arr2); // Output: [1, 2, 3, 4, 5]
function sum(...numbers) {
return numbers.reduce((acc, num) => acc + num, 0);
}
console.log(sum(1, 2, 3)); // Output: 6
7. Classes
ES6 introduced a more intuitive syntax for creating and managing classes, making object-oriented programming more straightforward in JavaScript.
class Person {
constructor(name, age) {
this.name = name;
this.age = age;
}
greet() {
return `Hello, my name is ${this.name} and I am ${this.age} years old.`;
}
}
const alice = new Person('Alice', 25);
console.log(alice.greet()); // Output: Hello, my name is Alice and I am 25 years old.
8. Promises
Promises provide a better way to handle asynchronous operations compared to traditional callback functions, allowing for more readable and maintainable code.
const fetchData = () => {
return new Promise((resolve, reject) => {
setTimeout(() => resolve('Data fetched'), 1000);
});
};
fetchData()
.then(data => console.log(data)) // Output: Data fetched
.catch(error => console.error(error));
9. Modules
ES6 modules allow for the modularization of code by providing a standardized way to export and import functions, objects, or values between different files.
// module.js
export const greet = name => `Hello, ${name}!`;
// main.js
import { greet } from './module.js';
console.log(greet('Alice')); // Output: Hello, Alice!
10. Enhanced Object Literals
ES6 enhances object literals by allowing shorthand property names, method definitions, and computed property names.
const name = 'Alice';
const person = {
name,
greet() {
return `Hello, my name is ${this.name}.`;
},
['age' + 'Years']: 25
};
console.log(person.greet()); // Output: Hello, my name is Alice.
console.log(person.ageYears); // Output: 25
Whether you’re a seasoned JavaScript developer or just starting, mastering ES6 is crucial for staying up-to-date with the latest trends and best practices in the ever-evolving world of web development.
Author
Nishant Singhal