Introduction to High-Level System Design
System Design Fundamentals
- Functional vs. Non-Functional Requirements
- Scalability, Availability, and Reliability
- Latency and Throughput Considerations
- Load Balancing Strategies
Architectural Patterns
- Monolithic vs. Microservices Architecture
- Layered Architecture
- Event-Driven Architecture
- Serverless Architecture
- Model-View-Controller (MVC) Pattern
- CQRS (Command Query Responsibility Segregation)
Scaling Strategies
- Vertical Scaling vs. Horizontal Scaling
- Sharding and Partitioning
- Data Replication and Consistency Models
- Load Balancing Strategies
- CDN and Edge Computing
Database Design in HLD
- SQL vs. NoSQL Databases
- CAP Theorem and its Impact on System Design
- Database Indexing and Query Optimization
- Database Sharding and Partitioning
- Replication Strategies
API Design and Communication
Caching Strategies
- Types of Caching
- Cache Invalidation Strategies
- Redis vs. Memcached
- Cache-Aside, Write-Through, and Write-Behind Strategies
Message Queues and Event-Driven Systems
- Kafka vs. RabbitMQ vs. SQS
- Pub-Sub vs. Point-to-Point Messaging
- Handling Asynchronous Workloads
- Eventual Consistency in Distributed Systems
Security in System Design
Observability and Monitoring
- Logging Strategies (ELK Stack, Prometheus, Grafana)
- API Security Best Practices
- Secure Data Storage and Access Control
- DDoS Protection and Rate Limiting
Real-World System Design Case Studies
- Distributed locking (Locking and its Types)
- Memory leaks and Out of memory issues
- HLD of YouTube
- HLD of WhatsApp
System Design Interview Questions
- Adobe System Design Interview Questions
- Top Atlassian System Design Interview Questions
- Top Amazon System Design Interview Questions
- Top Microsoft System Design Interview Questions
- Top Meta (Facebook) System Design Interview Questions
- Top Netflix System Design Interview Questions
- Top Uber System Design Interview Questions
- Top Google System Design Interview Questions
- Top Apple System Design Interview Questions
- Top Airbnb System Design Interview Questions
- Top 10 System Design Interview Questions
- Mobile App System Design Interview Questions
- Top 20 Stripe System Design Interview Questions
- Top Shopify System Design Interview Questions
- Top 20 System Design Interview Questions
- Top Advanced System Design Questions
- Most-Frequented System Design Questions in Big Tech Interviews
- What Interviewers Look for in System Design Questions
- Critical System Design Questions to Crack Any Tech Interview
- Top 20 API Design Questions for System Design Interviews
- Top 10 Steps to Create a System Design Portfolio for Developers
Introduction to High-Level System Design
System Design Fundamentals
- Functional vs. Non-Functional Requirements
- Scalability, Availability, and Reliability
- Latency and Throughput Considerations
- Load Balancing Strategies
Architectural Patterns
- Monolithic vs. Microservices Architecture
- Layered Architecture
- Event-Driven Architecture
- Serverless Architecture
- Model-View-Controller (MVC) Pattern
- CQRS (Command Query Responsibility Segregation)
Scaling Strategies
- Vertical Scaling vs. Horizontal Scaling
- Sharding and Partitioning
- Data Replication and Consistency Models
- Load Balancing Strategies
- CDN and Edge Computing
Database Design in HLD
- SQL vs. NoSQL Databases
- CAP Theorem and its Impact on System Design
- Database Indexing and Query Optimization
- Database Sharding and Partitioning
- Replication Strategies
API Design and Communication
Caching Strategies
- Types of Caching
- Cache Invalidation Strategies
- Redis vs. Memcached
- Cache-Aside, Write-Through, and Write-Behind Strategies
Message Queues and Event-Driven Systems
- Kafka vs. RabbitMQ vs. SQS
- Pub-Sub vs. Point-to-Point Messaging
- Handling Asynchronous Workloads
- Eventual Consistency in Distributed Systems
Security in System Design
Observability and Monitoring
- Logging Strategies (ELK Stack, Prometheus, Grafana)
- API Security Best Practices
- Secure Data Storage and Access Control
- DDoS Protection and Rate Limiting
Real-World System Design Case Studies
- Distributed locking (Locking and its Types)
- Memory leaks and Out of memory issues
- HLD of YouTube
- HLD of WhatsApp
System Design Interview Questions
- Adobe System Design Interview Questions
- Top Atlassian System Design Interview Questions
- Top Amazon System Design Interview Questions
- Top Microsoft System Design Interview Questions
- Top Meta (Facebook) System Design Interview Questions
- Top Netflix System Design Interview Questions
- Top Uber System Design Interview Questions
- Top Google System Design Interview Questions
- Top Apple System Design Interview Questions
- Top Airbnb System Design Interview Questions
- Top 10 System Design Interview Questions
- Mobile App System Design Interview Questions
- Top 20 Stripe System Design Interview Questions
- Top Shopify System Design Interview Questions
- Top 20 System Design Interview Questions
- Top Advanced System Design Questions
- Most-Frequented System Design Questions in Big Tech Interviews
- What Interviewers Look for in System Design Questions
- Critical System Design Questions to Crack Any Tech Interview
- Top 20 API Design Questions for System Design Interviews
- Top 10 Steps to Create a System Design Portfolio for Developers
Top Google DSA Interview Questions and How to Prepare
Introduction
In today’s competitive tech landscape, cracking a Google DSA interview can open doors to exciting opportunities. Many candidates begin their preparation by exploring free resources and updates – for instance, you can check out our free course updates to stay ahead. Data Structures and Algorithms (DSA) form the backbone of technical interviews, and understanding them is crucial to success. A strong foundation in DSA not only improves problem-solving skills but also builds the confidence needed during high-pressure interviews.
The Google interview process is rigorous and multifaceted, with DSA questions being a central component. Candidates must be adept at tackling challenges that range from basic array manipulation to complex graph problems. Emphasizing clarity and logical reasoning during the interview is just as important as coding skills. This article delves deep into top DSA interview questions at Google and offers step-by-step guidance on how to prepare effectively.
Understanding Google DSA Interviews
Google is known for its intensive technical interview process, which evaluates not only coding ability but also problem-solving acumen. Interviews typically include whiteboard sessions, coding on a computer, and discussions around various algorithms and data structures. According to industry surveys, a candidate’s performance in DSA rounds can determine up to 60% of the overall interview score.
Candidates should expect questions that cover arrays, linked lists, trees, graphs, and more advanced topics like dynamic programming and greedy algorithms. The interview format is designed to assess both theoretical knowledge and the practical application of these concepts. Many interviewers emphasize clarity in explanation and an ability to optimize solutions under time constraints. This blend of theory and practice is what sets Google interviews apart.
Key DSA Interview Questions Explored
Google interviewers focus on questions that test core data structures and algorithmic thinking. In this section, we explore several key questions that candidates often encounter during the interview process.
Common Interview Questions
- Arrays and Strings:
- How do you find the maximum subarray sum?
- Explain two-pointer techniques for solving string problems.
- Linked Lists:
- How do you reverse a linked list?
- What are the differences between singly and doubly linked lists?
- Trees and Graphs:
- How do you perform a level-order traversal of a binary tree?
- Explain depth-first search (DFS) and breadth-first search (BFS) in graphs.
- Dynamic Programming and Greedy Algorithms:
- Solve the classic knapsack problem.
- How do you optimize coin change problems using dynamic programming?
These questions not only evaluate your technical knowledge but also test your analytical skills and ability to communicate solutions clearly.
Sample DSA Question Breakdown
Consider the classic problem of reversing a linked list. Here’s how you might approach it:
- Understanding the Problem:
- Read and re-read the problem statement.
- Clarify any ambiguous requirements with the interviewer.
- Outlining the Approach:
- Decide whether to use an iterative or recursive solution.
- Consider edge cases such as an empty list or a list with a single node.
- Implementing the Code:
- Write clean, well-documented code on a whiteboard or IDE.
- Test the solution with sample inputs to validate correctness.
A bullet point summary for such problems includes:
- Understand the problem requirements.
- Outline a step-by-step plan.
- Write and test your solution thoroughly.
Detailed Analysis of DSA Problems
A detailed breakdown of DSA problems is essential for understanding the nuances of each topic. Here, we analyze various categories of DSA problems and discuss common pitfalls along with best practices.
Problem Categories and Their Nuances
Category | Common Problem Types | Key Considerations |
Arrays & Strings | Subarray problems, sliding window techniques, string manipulation | Time complexity, edge cases, space optimization |
Linked Lists | Reversal, cycle detection, merging sorted lists | Pointer management, memory efficiency |
Trees & Graphs | Tree traversals, graph connectivity, shortest path problems | Recursive vs iterative solutions, stack overflow risks |
Dynamic Programming | Knapsack, Fibonacci series, coin change problems | Overlapping subproblems, memoization techniques |
Greedy Algorithms | Activity selection, interval scheduling | Local optimum vs global optimum, proof of correctness |
In-Depth Example: Dynamic Programming
Dynamic Programming (DP) questions are among the most challenging. Here’s an overview of how to approach a DP problem:
- Problem Identification:
Recognize that the problem has overlapping subproblems and an optimal substructure. - Memoization vs Tabulation:
Decide whether to use a top-down (memoization) or bottom-up (tabulation) approach. - Edge Cases:
Always test with minimal and maximal inputs to ensure robustness.
Using these steps, candidates can dissect complex DP problems and build reliable solutions.
Strategies to Prepare for DSA Interviews
Preparation for Google DSA interviews requires a structured plan and consistent practice. Here are some strategies to help you get ready for the challenge.
Study Plan and Daily Practice
- Create a Study Schedule:
Allocate specific hours each day to practice coding problems. - Use Online Platforms:
Websites like LeetCode, HackerRank, and CodeSignal offer a variety of problems that simulate real interview scenarios. - Review Concepts Regularly:
Make time for revisiting core DSA concepts, even if you feel confident in them.
Practice Techniques
- Mock Interviews:
Engage in mock interviews to simulate the real experience. - Peer Programming:
Work with study partners to review and improve your coding approaches. - Timed Sessions:
Practice solving problems within a time limit to build speed and accuracy.
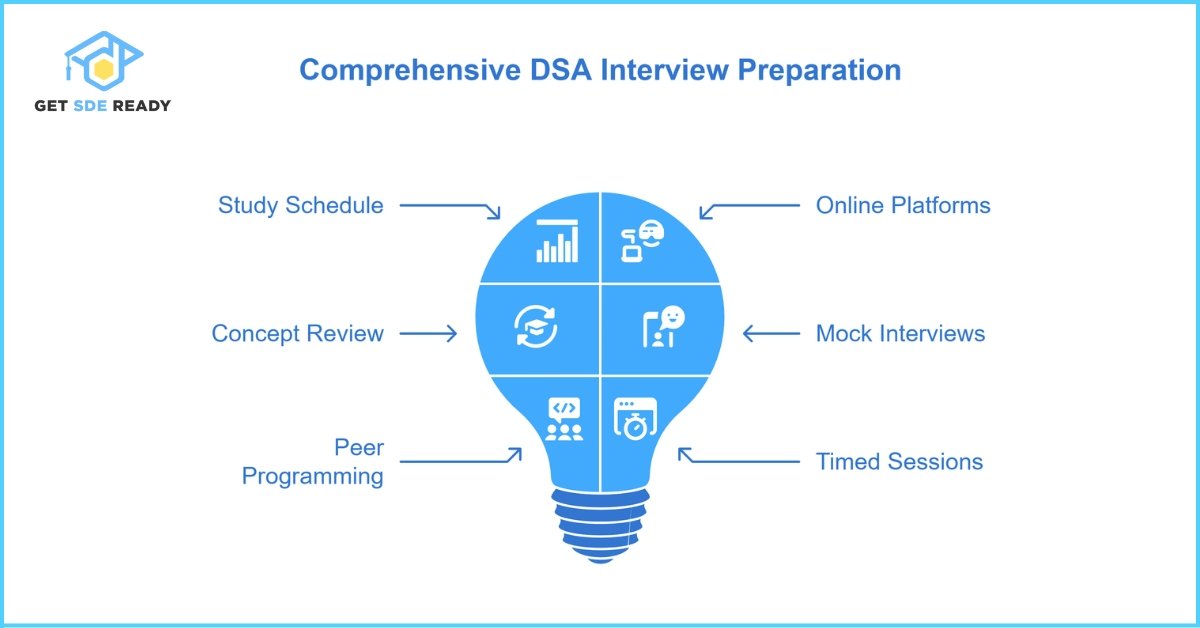
Key preparation tips in bullet form:
- Develop a consistent daily study routine.
- Leverage online coding platforms.
- Engage in regular mock interviews.
- Focus on problem areas and revisit core concepts.
Best Practices for DSA Interview Success
Adopting best practices is crucial to excel during DSA interviews. This section outlines habits and strategies that can help you perform at your best.
Communication and Problem-Solving
Clear communication is as important as coding skills. When solving a problem:
- Talk Through Your Thought Process:
Explain your reasoning and approach as you code. - Ask Clarifying Questions:
If a problem statement is unclear, ask questions to avoid assumptions. - Write Clean Code:
Use proper variable names, indentation, and comments.
Comparative Table: Effective Interview Practices
Practice | Why It Matters | How to Implement |
Clear Communication | Ensures interviewers understand your approach | Verbally explain each step while coding |
Regular Practice | Builds confidence and reduces anxiety | Solve a set number of problems daily |
Mock Interviews | Simulates real interview pressure | Schedule weekly practice sessions with peers |
Review & Revise | Identifies and corrects common mistakes | Analyze feedback from every mock session |
Following these best practices will not only prepare you for tough questions but also demonstrate your ability to work through problems logically and efficiently.
Advanced DSA Problem Solving Techniques
For those who are aiming to reach an expert level, mastering advanced problem solving techniques is essential. This section highlights some strategies that can elevate your performance.
Advanced Techniques and Patterns
- Divide and Conquer:
Break complex problems into manageable subproblems and solve them independently. - Memoization:
Optimize recursive solutions by storing previously computed results. - Greedy Algorithms:
Identify and implement strategies that choose the best immediate solution, which may lead to an overall optimal solution.
Example: Incorporating Python Libraries
Many candidates find that leveraging modern libraries can help in testing and understanding algorithm behavior. Consider these popular Python libraries and frameworks:
- NumPy and Pandas: Great for handling large data sets and performing complex operations efficiently.
- NetworkX: Ideal for graph-based problems and network analysis.
- SciPy: Useful for advanced mathematical computations.
These libraries not only streamline testing but also offer built-in functions that can validate your approach quickly.
Benefits of Advanced Preparation
- Efficiency Gains:
Advanced techniques can reduce time complexity, making solutions more efficient. - Better Problem Insights:
A deep understanding of algorithmic patterns allows for innovative problem-solving approaches. - Competitive Edge:
Mastery of these techniques sets you apart from other candidates during interviews.
Key bullet points:
- Use divide and conquer to simplify problems.
- Implement memoization to optimize recursive calls.
- Embrace greedy strategies where applicable.
Advanced Topics in DSA and System Design
This section bridges the gap between DSA fundamentals and system design—an essential component for many top tech interviews. Understanding how DSA principles integrate with system design can be a game-changer.
Integrating DSA with System Design
System design problems at Google often require you to consider scalability, efficiency, and robustness. For example, designing a real-time messaging system involves choosing the right data structures for fast access and reliability.
Comparison Table: DSA vs. System Design Focus Areas
Focus Area | DSA | System Design |
Data Structures | Arrays, Trees, Graphs, Hash Tables | Scalable storage solutions, caching mechanisms |
Algorithm Efficiency | Time and Space Complexity | Throughput, latency, and fault tolerance |
Problem Solving Approach | Step-by-step coding problems | High-level architectural planning |
Additional Considerations
- Scalability:
Even if a solution works in theory, it must scale to meet real-world demands. - Trade-offs:
Analyze trade-offs between different system components to build balanced solutions. - Design Patterns:
Familiarize yourself with common design patterns such as Singleton, Factory, and Observer, which often complement DSA solutions in system design contexts.
Furthermore, integrating resources such as Top 10 Python Libraries and Frameworks can help you leverage technology to prototype and test your designs efficiently.
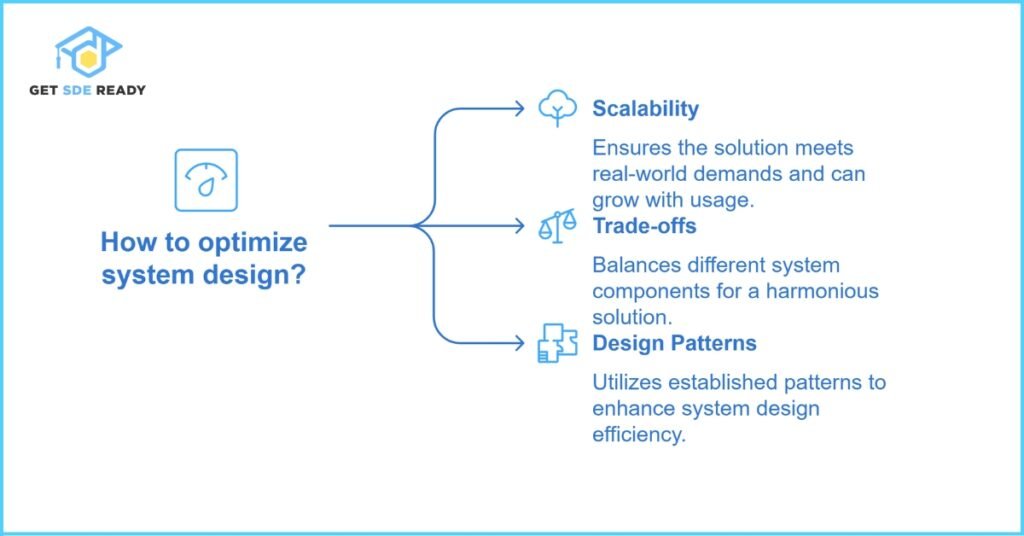
Resources and Study Materials for DSA Preparation
Access to the right resources can significantly accelerate your learning curve. In this section, we outline various study materials and platforms that offer comprehensive DSA practice and insights.
Recommended Resources
- Online Coding Platforms:
Websites like LeetCode, HackerRank, CodeChef, and GeeksforGeeks provide a wide range of problems with varying difficulty. - Books:
- “Cracking the Coding Interview” by Gayle Laakmann McDowell
- “Introduction to Algorithms” by Cormen, Leiserson, Rivest, and Stein
- Video Tutorials:
YouTube channels such as “Tech With Tim,” “CS Dojo,” and “Abdul Bari” offer in-depth tutorials and problem-solving sessions.
Resource Summary Table
Resource Type | Examples | Benefits |
Coding Platforms | LeetCode, HackerRank, CodeChef | Real interview simulation, vast problem repository |
Books | Cracking the Coding Interview, CLRS | Detailed explanations and advanced problem sets |
Video Tutorials | CS Dojo, Tech With Tim, Abdul Bari | Visual learning and step-by-step walkthroughs |
These resources can help you build a robust understanding of DSA concepts and prepare you thoroughly for technical interviews.
Additional Tips and Tricks for Interview Success
Beyond mastering the core concepts, there are numerous additional tips and tricks that can set you apart during your Google interview.
Personal Preparation Strategies
- Time Management:
Develop strategies to manage your time efficiently during both practice sessions and the actual interview. - Mock Interviews:
Schedule regular mock interviews with peers or mentors to build confidence and receive constructive feedback.
Stress Management:
Engage in activities like meditation, exercise, or hobbies to maintain a balanced and calm mindset.
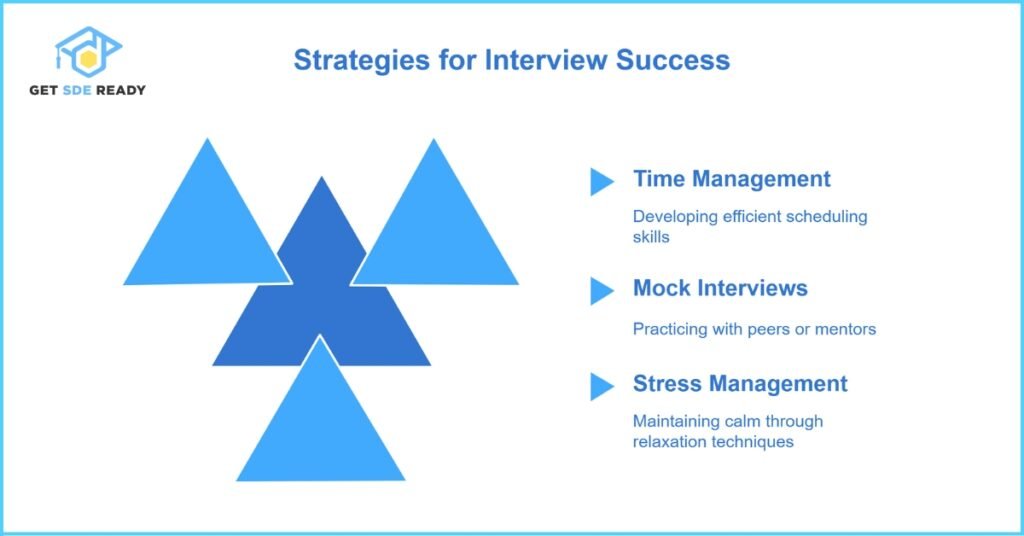
Quick Tips at a Glance
- Review common pitfalls and learn from past mistakes.
- Practice coding on paper or a whiteboard to simulate interview conditions.
- Stay updated with the latest trends in DSA and system design.
Adopting these additional tips can help ensure that you remain focused and confident on the day of your interview.
FAQ
What is the significance of DSA in Google interviews?
DSA is crucial in Google interviews because it forms the basis of problem-solving and logical reasoning required to tackle complex challenges. A strong command over data structures and algorithms not only helps you write efficient code but also demonstrates your ability to optimize solutions under pressure. Candidates who excel in DSA often have an edge in interviews, as these skills are directly related to the practical tasks you’ll face on the job. For further insights on mastering these concepts, consider exploring the DSA course.
How can I effectively prepare for DSA interviews?
Microsoft Azure is ideal for enterprises, especially those already using Microsoft products. Its seamless integration with tools like Office 365 and Windows Server makes it a top choice. For enterprise-level system design skills, check out our Master DSA, Web Dev & System Design Course.
What resources should I use to study DSA for Google interviews?
Studying for DSA interviews should include a mix of interactive coding challenges, comprehensive books, and instructional videos. Online platforms such as LeetCode and HackerRank offer real-time problem simulations, while books like “Cracking the Coding Interview” provide detailed strategies and explanations. Additionally, video tutorials can offer visual guidance and practical walkthroughs. By combining these resources, you can build a solid foundation and prepare effectively. For a combined curriculum that covers multiple aspects, explore the Design DSA Combined course.
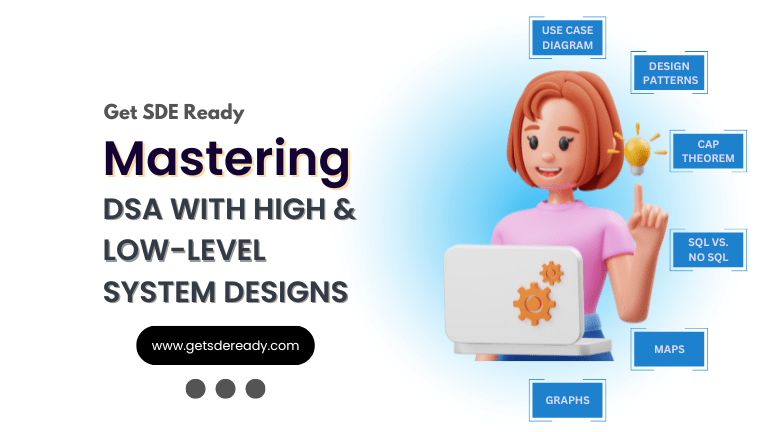
DSA, High & Low Level System Designs
- 85+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 400+ DSA Practice Questions
- Comprehensive Notes
- HackerRank Tests & Quizzes
- Topic-wise Quizzes
- Case Studies
- Access to Global Peer Community
Buy for 60% OFF
₹25,000.00 ₹9,999.00
Accelerate your Path to a Product based Career
Boost your career or get hired at top product-based companies by joining our expertly crafted courses. Gain practical skills and real-world knowledge to help you succeed.
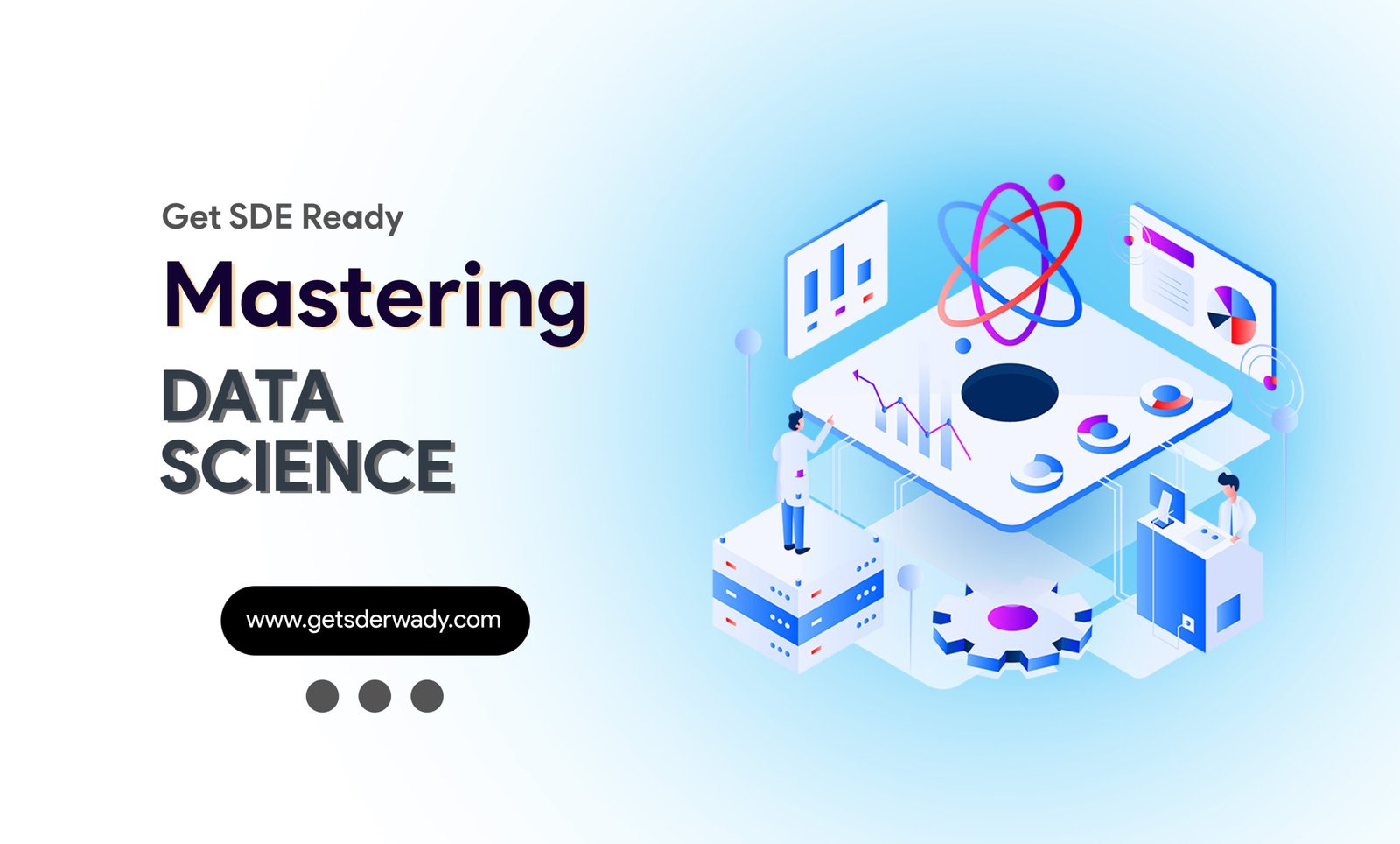
Essentials of Machine Learning and Artificial Intelligence
- 65+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 22+ Hands-on Live Projects & Deployments
- Comprehensive Notes
- Topic-wise Quizzes
- Case Studies
- Access to Global Peer Community
- Interview Prep Material
Buy for 65% OFF
₹20,000.00 ₹6,999.00
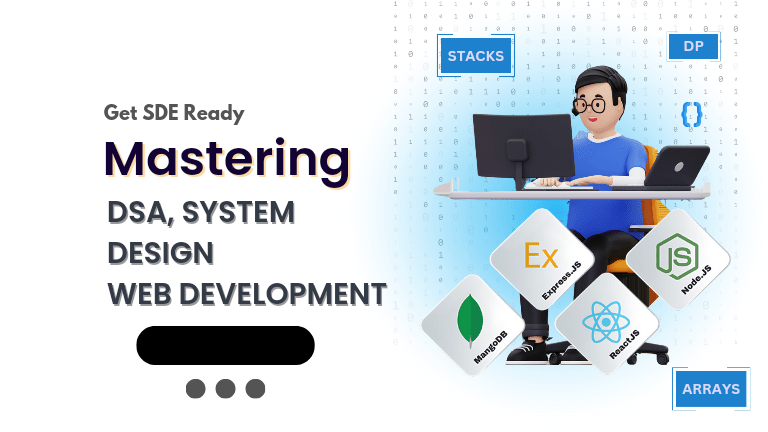
Fast-Track to Full Spectrum Software Engineering
- 120+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 400+ DSA Practice Questions
- Comprehensive Notes
- HackerRank Tests & Quizzes
- 12+ live Projects & Deployments
- Case Studies
- Access to Global Peer Community
Buy for 57% OFF
₹35,000.00 ₹14,999.00
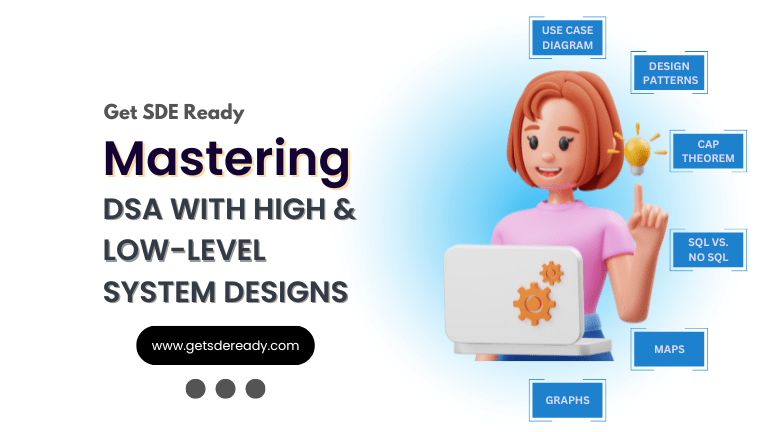
DSA, High & Low Level System Designs
- 85+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 400+ DSA Practice Questions
- Comprehensive Notes
- HackerRank Tests & Quizzes
- Topic-wise Quizzes
- Case Studies
- Access to Global Peer Community
Buy for 60% OFF
₹25,000.00 ₹9,999.00

Low & High Level System Design
- 20+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 400+ DSA Practice Questions
- Comprehensive Notes
- HackerRank Tests
- Topic-wise Quizzes
- Access to Global Peer Community
- Interview Prep Material
Buy for 65% OFF
₹20,000.00 ₹6,999.00
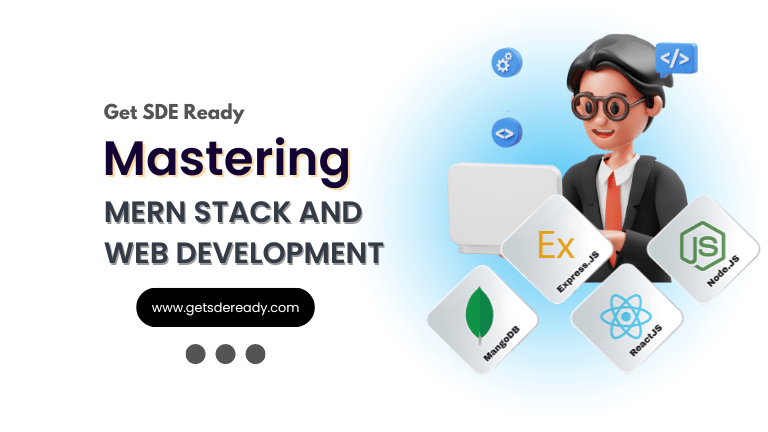
Mastering Mern Stack (WEB DEVELOPMENT)
- 65+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 12+ Hands-on Live Projects & Deployments
- Comprehensive Notes & Quizzes
- Real-world Tools & Technologies
- Access to Global Peer Community
- Interview Prep Material
- Placement Assistance
Buy for 60% OFF
₹15,000.00 ₹5,999.00
Reach Out Now
If you have any queries, please fill out this form. We will surely reach out to you.
Contact Email
Reach us at the following email address.
arun@getsdeready.com
Phone Number
You can reach us by phone as well.
+91-97737 28034
Our Location
Rohini, Sector-3, Delhi-110085