Data Structures and Algorithms
- Introduction to Data Structures and Algorithms
- Time and Space Complexity Analysis
- Big-O, Big-Theta, and Big-Omega Notations
- Recursion and Backtracking
- Divide and Conquer Algorithm
- Dynamic Programming: Memoization vs. Tabulation
- Greedy Algorithms and Their Use Cases
- Understanding Arrays: Types and Operations
- Linear Search vs. Binary Search
- Sorting Algorithms: Bubble, Insertion, Selection, and Merge Sort
- QuickSort: Explanation and Implementation
- Heap Sort and Its Applications
- Counting Sort, Radix Sort, and Bucket Sort
- Hashing Techniques: Hash Tables and Collisions
- Open Addressing vs. Separate Chaining in Hashing
- DSA Questions for Beginners
- Advanced DSA Questions for Competitive Programming
- Top 10 DSA Questions to Crack Your Next Coding Test
- Top 50 DSA Questions Every Programmer Should Practice
- Top Atlassian DSA Interview Questions
- Top Amazon DSA Interview Questions
- Top Microsoft DSA Interview Questions
- Top Meta (Facebook) DSA Interview Questions
- Netflix DSA Interview Questions and Preparation Guide
- Top 20 DSA Interview Questions You Need to Know
- Top Uber DSA Interview Questions and Solutions
- Google DSA Interview Questions and How to Prepare
- Airbnb DSA Interview Questions and How to Solve Them
- Mobile App DSA Interview Questions and Solutions
DSA Interview Questions
- DSA Questions for Beginners
- Advanced DSA Questions for Competitive Programming
- Top 10 DSA Questions to Crack Your Next Coding Test
- Top 50 DSA Questions Every Programmer Should Practice
- Top Atlassian DSA Interview Questions
- Top Amazon DSA Interview Questions
- Top Microsoft DSA Interview Questions
- Top Meta (Facebook) DSA Interview Questions
- Netflix DSA Interview Questions and Preparation Guide
- Top 20 DSA Interview Questions You Need to Know
- Top Uber DSA Interview Questions and Solutions
- Google DSA Interview Questions and How to Prepare
- Airbnb DSA Interview Questions and How to Solve Them
- Mobile App DSA Interview Questions and Solutions
Introduction to High-Level System Design
System Design Fundamentals
- Functional vs. Non-Functional Requirements
- Scalability, Availability, and Reliability
- Latency and Throughput Considerations
- Load Balancing Strategies
Architectural Patterns
- Monolithic vs. Microservices Architecture
- Layered Architecture
- Event-Driven Architecture
- Serverless Architecture
- Model-View-Controller (MVC) Pattern
- CQRS (Command Query Responsibility Segregation)
Scaling Strategies
- Vertical Scaling vs. Horizontal Scaling
- Sharding and Partitioning
- Data Replication and Consistency Models
- Load Balancing Strategies
- CDN and Edge Computing
Database Design in HLD
- SQL vs. NoSQL Databases
- CAP Theorem and its Impact on System Design
- Database Indexing and Query Optimization
- Database Sharding and Partitioning
- Replication Strategies
API Design and Communication
Caching Strategies
- Types of Caching
- Cache Invalidation Strategies
- Redis vs. Memcached
- Cache-Aside, Write-Through, and Write-Behind Strategies
Message Queues and Event-Driven Systems
- Kafka vs. RabbitMQ vs. SQS
- Pub-Sub vs. Point-to-Point Messaging
- Handling Asynchronous Workloads
- Eventual Consistency in Distributed Systems
Security in System Design
Observability and Monitoring
- Logging Strategies (ELK Stack, Prometheus, Grafana)
- API Security Best Practices
- Secure Data Storage and Access Control
- DDoS Protection and Rate Limiting
Real-World System Design Case Studies
- Distributed locking (Locking and its Types)
- Memory leaks and Out of memory issues
- HLD of YouTube
- HLD of WhatsApp
System Design Interview Questions
- Adobe System Design Interview Questions
- Top Atlassian System Design Interview Questions
- Top Amazon System Design Interview Questions
- Top Microsoft System Design Interview Questions
- Top Meta (Facebook) System Design Interview Questions
- Top Netflix System Design Interview Questions
- Top Uber System Design Interview Questions
- Top Google System Design Interview Questions
- Top Apple System Design Interview Questions
- Top Airbnb System Design Interview Questions
- Top 10 System Design Interview Questions
- Mobile App System Design Interview Questions
- Top 20 Stripe System Design Interview Questions
- Top Shopify System Design Interview Questions
- Top 20 System Design Interview Questions
- Top Advanced System Design Questions
- Most-Frequented System Design Questions in Big Tech Interviews
- What Interviewers Look for in System Design Questions
- Critical System Design Questions to Crack Any Tech Interview
- Top 20 API Design Questions for System Design Interviews
- Top 10 Steps to Create a System Design Portfolio for Developers
Greedy Algorithms and Their Use Cases
If you’re eager to master problem-solving techniques that power everything from GPS navigation to data compression, you’re in the right place. Greedy algorithms are a cornerstone of efficient coding, and understanding them can elevate your programming skills. For free resources and course updates to sharpen your expertise, join our community of learners today.
Introduction to Greedy Algorithms
Greedy algorithms solve problems by making the best choice at each step, hoping it leads to the optimal solution. Imagine you’re trying to make change with the fewest coins possible—you’d pick the largest coin value first (like 25 cents before 10 cents). This “take what you can now” approach is simple but powerful.
However, greedy algorithms don’t always guarantee the best result. They work only when a problem has two key properties:
Key Properties
Optimal Substructure
Problems solvable by greedy methods can be broken into smaller subproblems. Solving each subproblem optimally ensures the overall solution is optimal.
Greedy Choice Property
Each decision is irreversible and locally optimal. For example, in scheduling jobs for maximum profit, selecting the highest-paying job first might lead to the best outcome.
Structured learning, like a crash course in algorithmic design, can help you recognize these patterns faster.
Characteristics of Greedy Algorithms
Optimal Substructure
Problems solvable by greedy methods can be broken into smaller subproblems. Solving each subproblem optimally ensures the overall solution is optimal.
Greedy Choice Property
Each decision is irreversible and locally optimal. For example, in scheduling jobs for maximum profit, selecting the highest-paying job first might lead to the best outcome.
Key Traits of Greedy Algorithms
- Immediate decision-making
- No reconsideration of past choices
- High efficiency but limited applicability
Mastering these traits is foundational for acing coding interviews, where greedy strategies often appear.

Common Use Cases of Greedy Algorithms
Huffman Coding for Data Compression
Huffman coding assigns shorter codes to frequent characters, reducing file sizes. It’s used in ZIP files and MP3 encoding. This technique is a staple in data science workflows, where efficient data storage is critical.
Dijkstra’s Algorithm for Shortest Path
This algorithm finds the shortest path in graphs with non-negative weights, making it ideal for GPS navigation systems. Developers often encounter this in web development projects involving route optimization for logistics apps.
Minimum Spanning Tree (Kruskal’s and Prim’s Algorithms)
Both algorithms connect nodes in a graph with minimal total edge weight.
Algorithm | Approach | Use Case |
Kruskal’s | Sort edges, add incrementally | Sparse networks |
Prim’s | Grow tree from a start node | Dense networks |
These concepts are covered in depth in advanced DSA courses for programmers aiming to specialize in network optimization.
Activity Selection Problem
Selecting the maximum number of non-overlapping tasks (e.g., scheduling meetings) is a classic greedy problem.
Advantages and Disadvantages
Pros of Greedy Algorithms
- Speed: Often run in linear or logarithmic time.
- Simplicity: Easy to implement with clear logic.
- Efficiency: Minimal memory usage.
Cons of Greedy Algorithms
- Not Always Optimal: Fail without the greedy choice property.
- Short-Sighted: Local choices can lead to poor global results.
For a deeper dive into balancing these trade-offs, consider mastering system design principles to complement algorithmic knowledge.

Greedy vs. Dynamic Programming
Factor | Greedy Algorithms | Dynamic Programming |
Approach | Top-down, immediate choices | Bottom-up, memorization |
Optimality | Sometimes optimal | Always optimal |
Complexity | Low | High |
Steps to Implement a Greedy Algorithm
Problem Analysis
Verify the problem has optimal substructure and greedy choice properties.
Define the Greedy Choice
Identify the best local choice (e.g., largest coin in change-making).
Prove Optimality
Use mathematical proofs to confirm the algorithm works.
Example Code: Coin Change Problem
def coin_change(coins, amount):
coins.sort(reverse=True)
count = 0
for coin in coins:
while amount >= coin:
amount -= coin
count += 1
return count if amount == 0 else -1
This type of problem is a favorite in top tech company interviews, where candidates must demonstrate both coding skill and strategic thinking.
Real-World Examples of Greedy Algorithms
Telecommunications
Greedy algorithms optimize bandwidth allocation in networks.
Finance
Stock trading strategies often use greedy logic for short-term profit maximization.
Travel and Logistics
Ride-sharing apps like Uber use Dijkstra’s algorithm to calculate fastest routes.
Industry Applications Table
Industry | Application |
Healthcare | MRI scan scheduling |
E-commerce | Inventory management |
Aerospace | Flight path optimization |
For case studies on these applications, explore domain-specific courses that blend algorithm design with industry needs.

What types of problems are best suited for greedy algorithms?
Greedy algorithms excel in optimization problems where local choices lead to global solutions, like the activity selection or Huffman coding. For structured learning, explore our Data Structures & Algorithms Course, which covers these concepts in depth.
Can greedy algorithms be used in web development?
Yes! They optimize tasks like resource loading and API scheduling. To build these skills, enroll in our Web Development Course, which integrates algorithm design with practical projects.
How do I prepare for greedy algorithm interview questions?
Mastering DSA basics is crucial. Our Comprehensive DSA & Web Dev Program includes mock interviews and problem-solving drills tailored for top tech companies like Meta and Netflix.
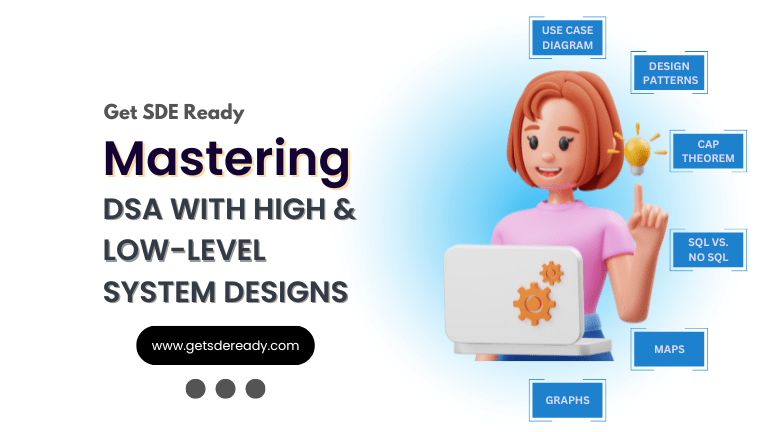
DSA, High & Low Level System Designs
- 85+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 400+ DSA Practice Questions
- Comprehensive Notes
- HackerRank Tests & Quizzes
- Topic-wise Quizzes
- Case Studies
- Access to Global Peer Community
Buy for 60% OFF
₹25,000.00 ₹9,999.00
Accelerate your Path to a Product based Career
Boost your career or get hired at top product-based companies by joining our expertly crafted courses. Gain practical skills and real-world knowledge to help you succeed.
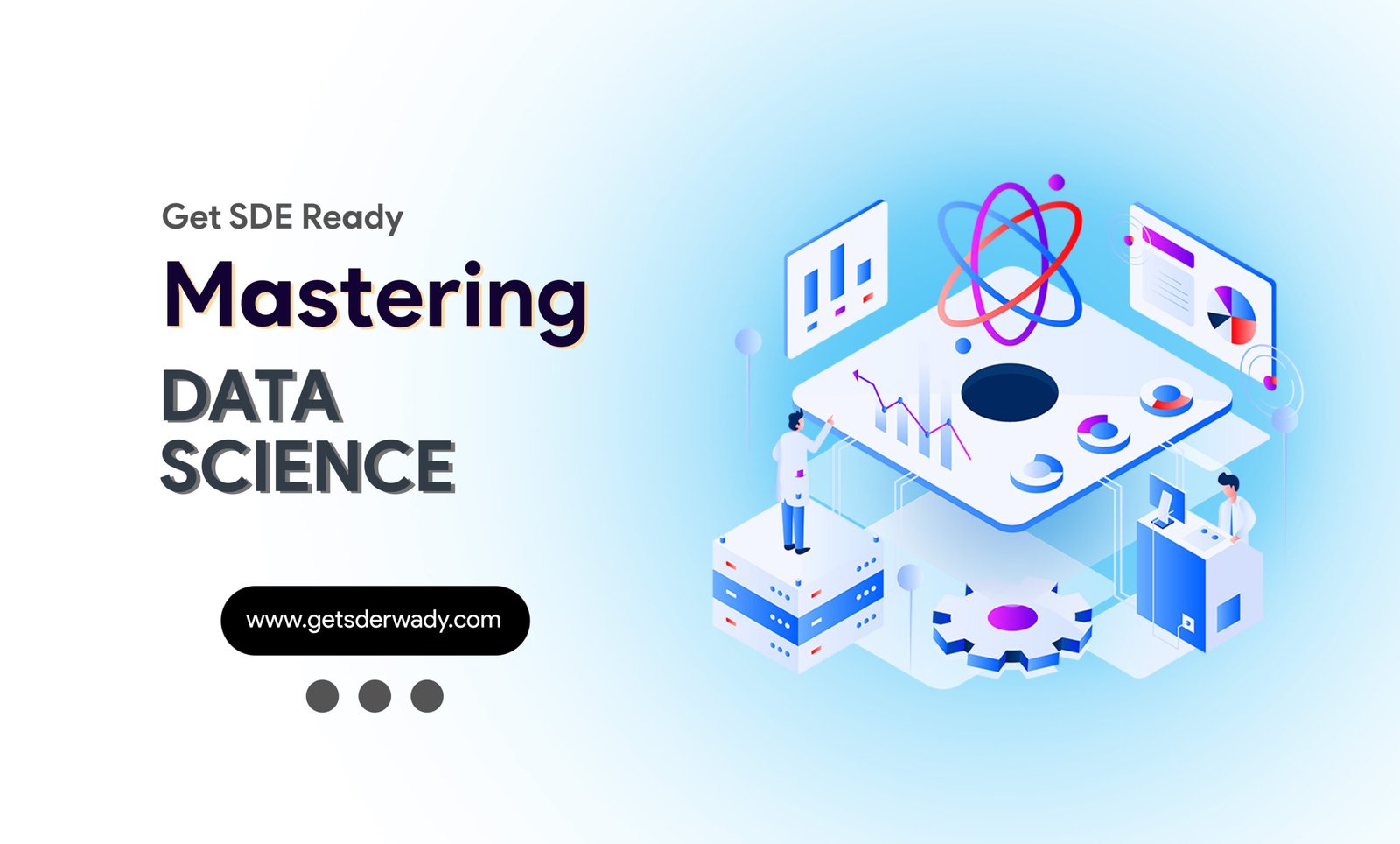
Essentials of Machine Learning and Artificial Intelligence
- 65+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 22+ Hands-on Live Projects & Deployments
- Comprehensive Notes
- Topic-wise Quizzes
- Case Studies
- Access to Global Peer Community
- Interview Prep Material
Buy for 65% OFF
₹20,000.00 ₹6,999.00
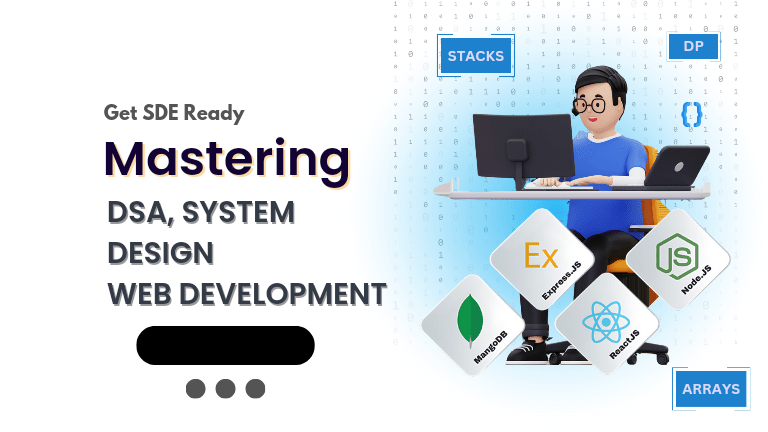
Fast-Track to Full Spectrum Software Engineering
- 120+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 400+ DSA Practice Questions
- Comprehensive Notes
- HackerRank Tests & Quizzes
- 12+ live Projects & Deployments
- Case Studies
- Access to Global Peer Community
Buy for 57% OFF
₹35,000.00 ₹14,999.00
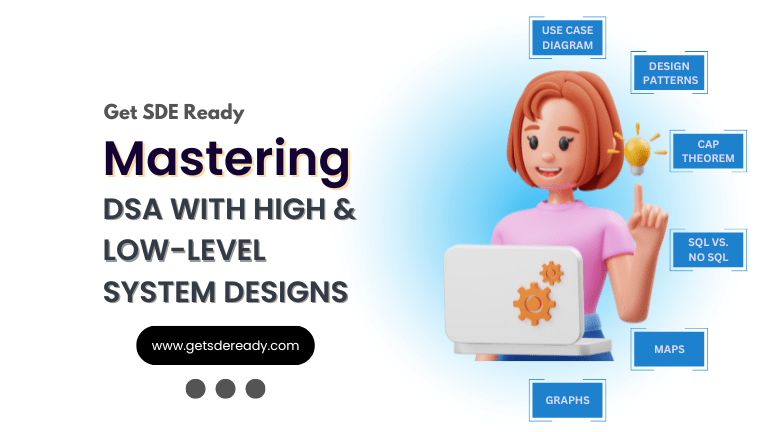
DSA, High & Low Level System Designs
- 85+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 400+ DSA Practice Questions
- Comprehensive Notes
- HackerRank Tests & Quizzes
- Topic-wise Quizzes
- Case Studies
- Access to Global Peer Community
Buy for 60% OFF
₹25,000.00 ₹9,999.00

Low & High Level System Design
- 20+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 400+ DSA Practice Questions
- Comprehensive Notes
- HackerRank Tests
- Topic-wise Quizzes
- Access to Global Peer Community
- Interview Prep Material
Buy for 65% OFF
₹20,000.00 ₹6,999.00
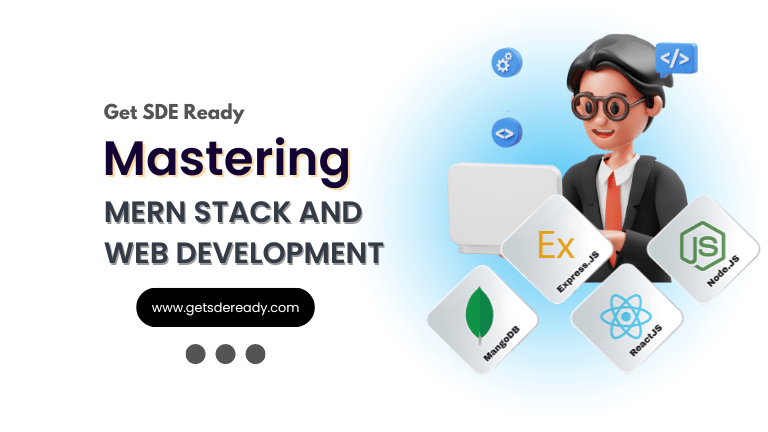
Mastering Mern Stack (WEB DEVELOPMENT)
- 65+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 12+ Hands-on Live Projects & Deployments
- Comprehensive Notes & Quizzes
- Real-world Tools & Technologies
- Access to Global Peer Community
- Interview Prep Material
- Placement Assistance
Buy for 60% OFF
₹15,000.00 ₹5,999.00

Mastering Data Structures & Algorithms
- 65+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 400+ DSA Practice Questions
- Comprehensive Notes
- HackerRank Tests
- Access to Global Peer Community
- Topic-wise Quizzes
- Interview Prep Material
Buy for 50% OFF
₹9,999.00 ₹4,999.00
Reach Out Now
If you have any queries, please fill out this form. We will surely reach out to you.
Contact Email
Reach us at the following email address.
arun@getsdeready.com
Phone Number
You can reach us by phone as well.
+91-97737 28034
Our Location
Rohini, Sector-3, Delhi-110085