Data Structures and Algorithms
- Introduction to Data Structures and Algorithms
- Time and Space Complexity Analysis
- Big-O, Big-Theta, and Big-Omega Notations
- Recursion and Backtracking
- Divide and Conquer Algorithm
- Dynamic Programming: Memoization vs. Tabulation
- Greedy Algorithms and Their Use Cases
- Understanding Arrays: Types and Operations
- Linear Search vs. Binary Search
- Sorting Algorithms: Bubble, Insertion, Selection, and Merge Sort
- QuickSort: Explanation and Implementation
- Heap Sort and Its Applications
- Counting Sort, Radix Sort, and Bucket Sort
- Hashing Techniques: Hash Tables and Collisions
- Open Addressing vs. Separate Chaining in Hashing
- DSA Questions for Beginners
- Advanced DSA Questions for Competitive Programming
- Top 10 DSA Questions to Crack Your Next Coding Test
- Top 50 DSA Questions Every Programmer Should Practice
- Top Atlassian DSA Interview Questions
- Top Amazon DSA Interview Questions
- Top Microsoft DSA Interview Questions
- Top Meta (Facebook) DSA Interview Questions
- Netflix DSA Interview Questions and Preparation Guide
- Top 20 DSA Interview Questions You Need to Know
- Top Uber DSA Interview Questions and Solutions
- Google DSA Interview Questions and How to Prepare
- Airbnb DSA Interview Questions and How to Solve Them
- Mobile App DSA Interview Questions and Solutions
DSA Interview Questions
- DSA Questions for Beginners
- Advanced DSA Questions for Competitive Programming
- Top 10 DSA Questions to Crack Your Next Coding Test
- Top 50 DSA Questions Every Programmer Should Practice
- Top Atlassian DSA Interview Questions
- Top Amazon DSA Interview Questions
- Top Microsoft DSA Interview Questions
- Top Meta (Facebook) DSA Interview Questions
- Netflix DSA Interview Questions and Preparation Guide
- Top 20 DSA Interview Questions You Need to Know
- Top Uber DSA Interview Questions and Solutions
- Google DSA Interview Questions and How to Prepare
- Airbnb DSA Interview Questions and How to Solve Them
- Mobile App DSA Interview Questions and Solutions
Data Structures and Algorithms
- Introduction to Data Structures and Algorithms
- Time and Space Complexity Analysis
- Big-O, Big-Theta, and Big-Omega Notations
- Recursion and Backtracking
- Divide and Conquer Algorithm
- Dynamic Programming: Memoization vs. Tabulation
- Greedy Algorithms and Their Use Cases
- Understanding Arrays: Types and Operations
- Linear Search vs. Binary Search
- Sorting Algorithms: Bubble, Insertion, Selection, and Merge Sort
- QuickSort: Explanation and Implementation
- Heap Sort and Its Applications
- Counting Sort, Radix Sort, and Bucket Sort
- Hashing Techniques: Hash Tables and Collisions
- Open Addressing vs. Separate Chaining in Hashing
- DSA Questions for Beginners
- Advanced DSA Questions for Competitive Programming
- Top 10 DSA Questions to Crack Your Next Coding Test
- Top 50 DSA Questions Every Programmer Should Practice
- Top Atlassian DSA Interview Questions
- Top Amazon DSA Interview Questions
- Top Microsoft DSA Interview Questions
- Top Meta (Facebook) DSA Interview Questions
- Netflix DSA Interview Questions and Preparation Guide
- Top 20 DSA Interview Questions You Need to Know
- Top Uber DSA Interview Questions and Solutions
- Google DSA Interview Questions and How to Prepare
- Airbnb DSA Interview Questions and How to Solve Them
- Mobile App DSA Interview Questions and Solutions
DSA Interview Questions
- DSA Questions for Beginners
- Advanced DSA Questions for Competitive Programming
- Top 10 DSA Questions to Crack Your Next Coding Test
- Top 50 DSA Questions Every Programmer Should Practice
- Top Atlassian DSA Interview Questions
- Top Amazon DSA Interview Questions
- Top Microsoft DSA Interview Questions
- Top Meta (Facebook) DSA Interview Questions
- Netflix DSA Interview Questions and Preparation Guide
- Top 20 DSA Interview Questions You Need to Know
- Top Uber DSA Interview Questions and Solutions
- Google DSA Interview Questions and How to Prepare
- Airbnb DSA Interview Questions and How to Solve Them
- Mobile App DSA Interview Questions and Solutions
Heap Queues in Python
Heap queues, also known as priority queues, are a powerful data structure in Python that allow efficient access to the smallest or largest elements in a collection. Whether you’re managing tasks by priority or implementing algorithms like Dijkstra’s shortest path, understanding heap queues is essential for any Python programmer. In this in-depth tutorial, we’ll explore the heapq module, covering everything from basic operations to advanced techniques. By the end, you’ll be equipped to leverage heap queues effectively in your projects. Ready to elevate your Python skills? Sign up for exclusive tutorials and free resources to accelerate your programming journey!
What is a Heap Queue in Python?
A heap queue (or priority queue) is a data structure that maintains a collection of elements, allowing quick access to the smallest (min-heap) or largest (max-heap) element. In Python, the heapq module implements a min-heap by default, where the smallest element is always at the root of the heap. This makes it ideal for scenarios where you need to repeatedly retrieve the smallest item efficiently.
Key Features of heapq
- Efficient Operations: Adding and removing elements is done in O(log n) time.
- Space-Efficient: Stored as a list, making it memory-friendly.
- Versatile: Can be used to implement priority queues, heaps, and more.
- Part of Standard Library: No need for external installations—it’s built into Python.
Heap queues are particularly useful in applications like task scheduling, event simulations, and graph algorithms. To dive deeper into data structures, explore our Data Structures and Algorithms course.
Creating a Heap Queue
To use a heap queue in Python, start by importing the heapq module. You can then convert a list into a heap using the heapify() function, which rearranges the list in-place to satisfy the heap property.
Example: Creating a Heap Queue
import heapq
# Create a list
data = [10, 20, 15, 30, 40]
# Convert the list into a heap
heapq.heapify(data)
print("Heap queue:", data)
Output:
Heap queue: [10, 20, 15, 30, 40]
In this example, heapify() transforms the list into a valid min-heap, where the smallest element (10) is at the root (index 0).
Basic Operations on Heap Queues
Heap queues support several fundamental operations that make them efficient for priority-based tasks.
1. Adding Elements with heappush
To add an element to the heap while maintaining the heap property, use heappush().
import heapq
# Initialize a heap
heap = [10, 20, 15, 30, 40]
heapq.heapify(heap)
# Add a new element
heapq.heappush(heap, 5)
print("Heap after push:", heap)
Output:
Heap after push: [5, 10, 15, 30, 40, 20]
The new element (5) is added, and the heap is adjusted to keep the smallest element at the root.
2. Removing the Smallest Element with heappop
To remove and return the smallest element, use heappop().
smallest = heapq.heappop(heap)
print("Smallest element:", smallest)
print("Heap after pop:", heap)
Output:
Smallest element: 5
Heap after pop: [10, 20, 15, 30, 40]
After removing 5, the next smallest element (10) becomes the root.
3. Peeking at the Smallest Element
To view the smallest element without removing it, simply access the first element of the list (heap[0]).
print("Smallest element:", heap[0])
Output:
Smallest element: 10
These operations form the core of working with heap queues. For more coding practice, check out our Crash Course.
Advanced Operations on Heap Queues
The heapq module also provides advanced functions for more complex tasks, enhancing its versatility.
1. Simultaneous Push and Pop with heappushpop
The heappushpop() function allows you to add a new element and remove the smallest element in a single operation, which is more efficient than performing both actions separately.
# Push 25 and pop the smallest element
smallest = heapq.heappushpop(heap, 25)
print("Popped element:", smallest)
print("Heap after pushpop:", heap)
Output:
Popped element: 10
Heap after pushpop: [15, 20, 25, 30, 40]
Here, 25 is added, and the smallest element (10) is removed simultaneously.
2. Finding n Largest and Smallest Elements
Use nlargest() and nsmallest() to retrieve the n largest or smallest elements from the heap.
# Find the 3 largest elements
largest = heapq.nlargest(3, heap)
print("3 largest elements:", largest)
# Find the 3 smallest elements
smallest = heapq.nsmallest(3, heap)
print("3 smallest elements:", smallest)
Output:
3 largest elements: [40, 30, 25]
3 smallest elements: [15, 20, 25]
Output:
Processing: High priority task (Priority: 1)
Processing: Low priority task (Priority: 2)
Processing: Medium priority task (Priority: 3)
These functions are efficient for retrieving multiple elements without repeatedly popping.
3. Replacing the Smallest Element with heapreplace
The heapreplace() function removes the smallest element and inserts a new one in a single step.
# Replace the smallest element with 5
replaced = heapq.heapreplace(heap, 5)
print("Replaced element:", replaced)
print("Heap after replace:", heap)
Output:
Replaced element: 15
Heap after replace: [5, 20, 25, 30, 40]
This operation is useful when you need to update the heap by replacing the smallest element.
4. Merging Multiple Heaps with merge
The merge() function combines multiple sorted iterables (including heaps) into a single sorted iterator.
# Create another heap
heap2 = [2, 4, 6, 8]
heapq.heapify(heap2)
# Merge the two heaps
merged = list(heapq.merge(heap, heap2))
print("Merged heap:", merged)
Output:
Merged heap: [2, 4, 5, 6, 8, 20, 25, 30, 40]
This is efficient for combining pre-sorted data while maintaining order.
Advantages and Disadvantages of Heap Queues
Advantages
- Efficiency: Operations like push and pop are O(log n), making them fast for large datasets.
- Space-Efficient: Stored as a list, minimizing memory overhead.
- Easy to Use: The heapq module provides straightforward functions for heap operations.
- Versatile: Can be adapted for various data structures like priority queues and binary trees.
Disadvantages
- Limited Functionality: Lacks support for random access or modifying middle elements.
- No Built-In Sorting: While it maintains order, it doesn’t sort the entire list on demand.
- Not Thread-Safe: Requires additional synchronization in multithreaded environments.
Understanding these pros and cons helps you decide when to use heap queues in your projects.
Conclusion
Heap queues in Python, implemented via the heapq module, are a versatile and efficient way to manage priority-based data. From basic operations like pushing and popping elements to advanced functions like merging heaps, heapq offers a robust toolkit for handling min-heaps. While it has limitations, such as lack of thread safety and random access, its benefits make it indispensable for tasks requiring quick access to the smallest elements. Start incorporating heap queues into your Python projects to optimize performance and streamline your code!
FAQs
What is the difference between a heap queue and a regular queue?
A heap queue prioritizes elements based on their value (smallest first in a min-heap), while a regular queue follows FIFO (First-In-First-Out). Learn more in our Data Structures and Algorithms course.
How do I implement a max-heap in Python?
While heapq provides min-heaps by default, you can simulate a max-heap by negating values or using a custom comparator. Explore practical examples in our Web Development course.
What are some real-world applications of heap queues?
Heap queues are used in task scheduling, event simulations, and algorithms like Dijkstra’s shortest path. Discover more in our Design and DSA Combined course.
How can I prepare for data structure interviews?
Master key concepts and practice problems like those in our Master DSA, Web Dev, and System Design course. Check out top questions from Netflix or Amazon.
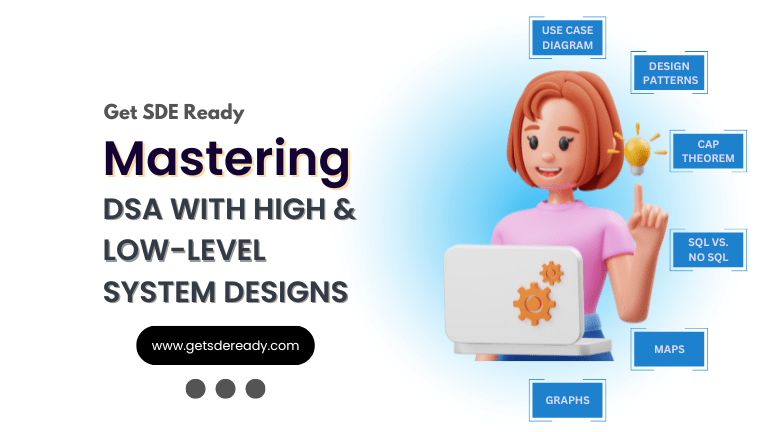
DSA, High & Low Level System Designs
- 85+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 400+ DSA Practice Questions
- Comprehensive Notes
- HackerRank Tests & Quizzes
- Topic-wise Quizzes
- Case Studies
- Access to Global Peer Community
Buy for 60% OFF
₹25,000.00 ₹9,999.00
Accelerate your Path to a Product based Career
Boost your career or get hired at top product-based companies by joining our expertly crafted courses. Gain practical skills and real-world knowledge to help you succeed.

Low & High Level System Design
- 20+ Live Classes & Recordings
- 24*7 Live Doubt Support
- Case Studies
- Comprehensive Notes
- HackerRank Tests
- Topic-wise Quizzes
- Access to Global Peer Community
- Interview Prep Material
Buy for 65% OFF
₹20,000.00 ₹6,999.00
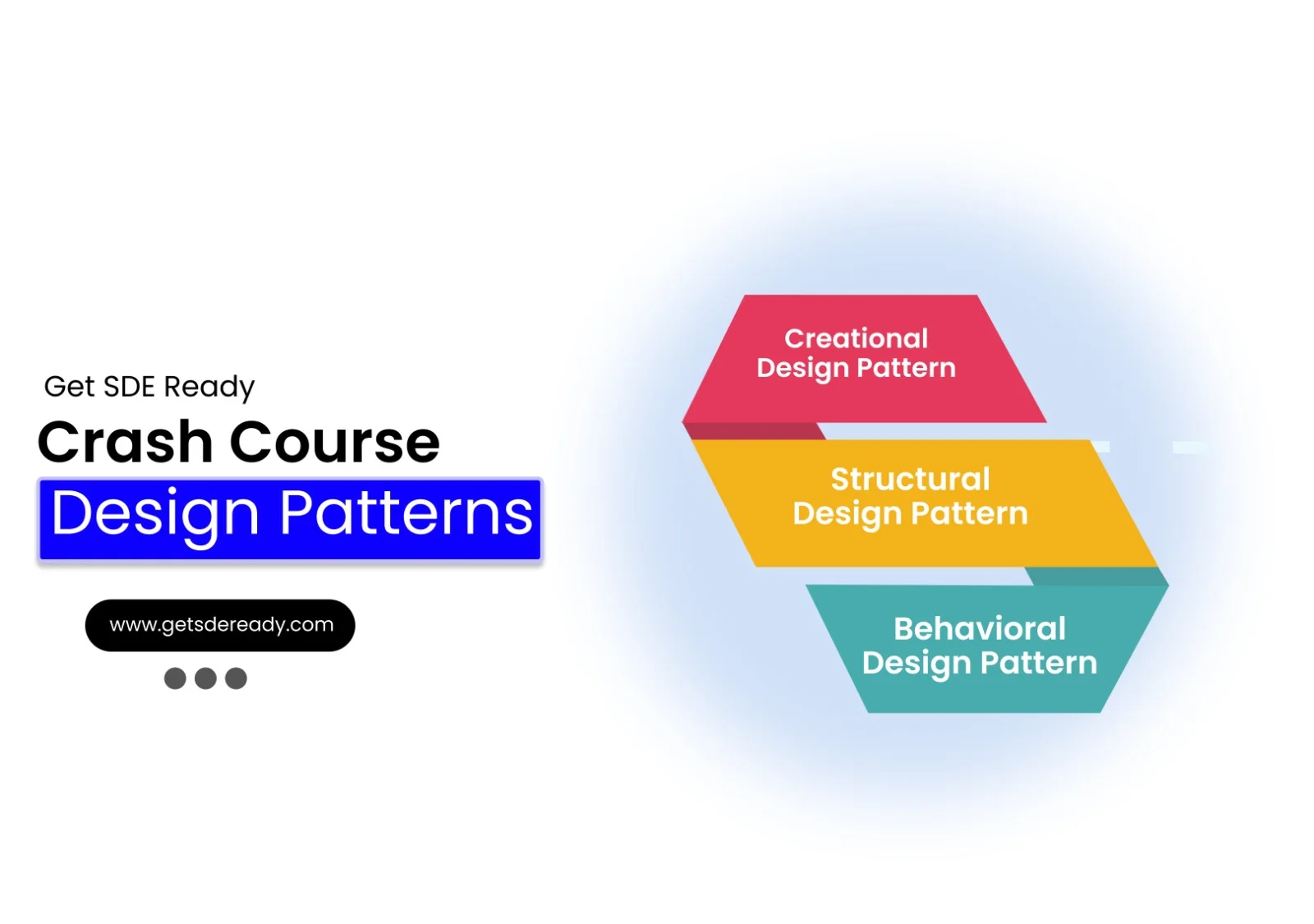
Design Patterns Bootcamp
- Live Classes & Recordings
- 24/7 Live Doubt Support
- Practice Questions
- Case Studies
- Access to Global Peer Community
- Topic wise Quizzes
- Referrals
- Certificate of Completion
Buy for 50% OFF
₹2,000.00 ₹999.00
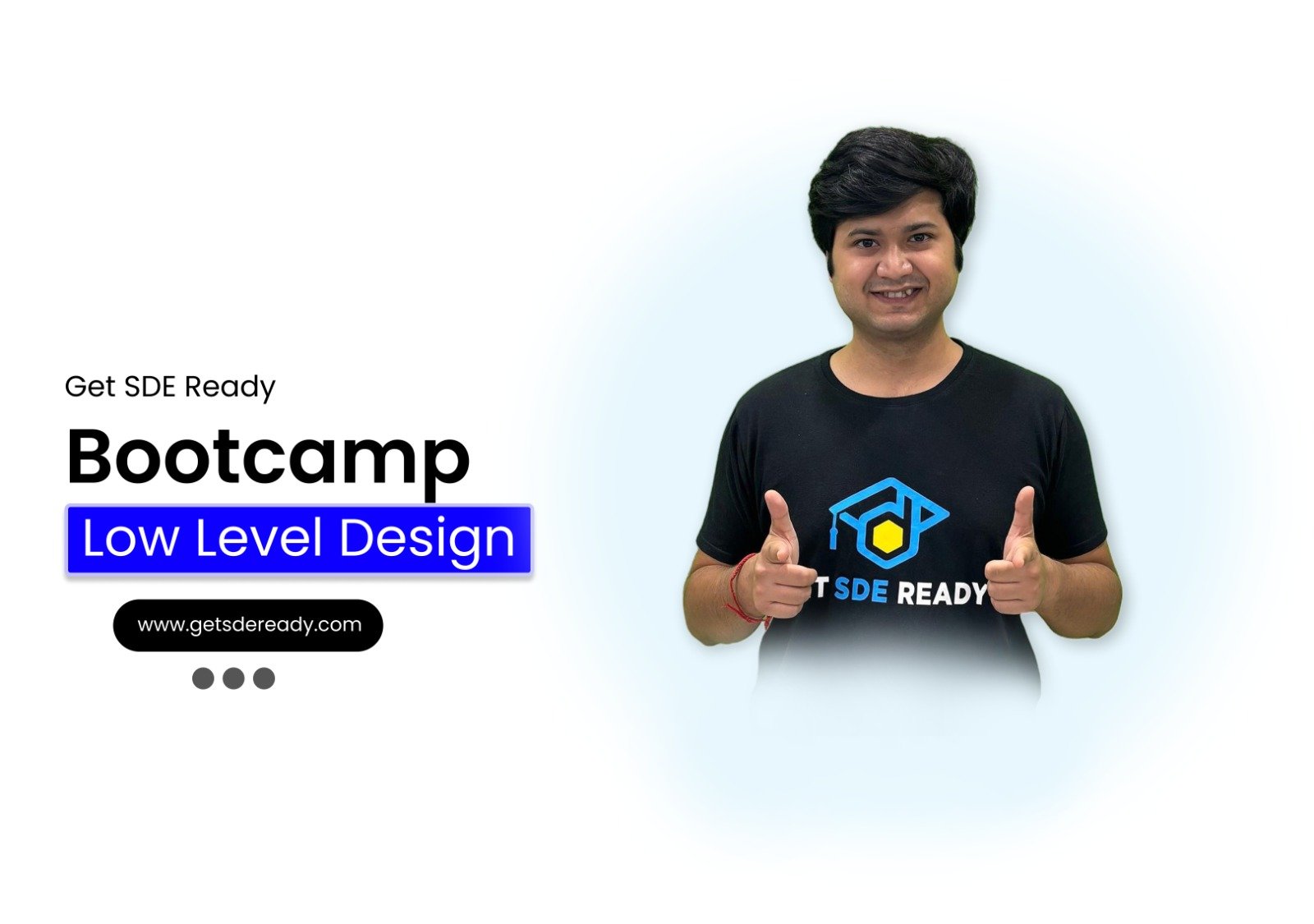
LLD Bootcamp
- 7+ Live Classes & Recordings
- Practice Questions
- 24/7 Live Doubt Support
- Case Studies
- Topic wise Quizzes
- Access to Global Peer Community
- Certificate of Completion
- Referrals
Buy for 50% OFF
₹2,000.00 ₹999.00
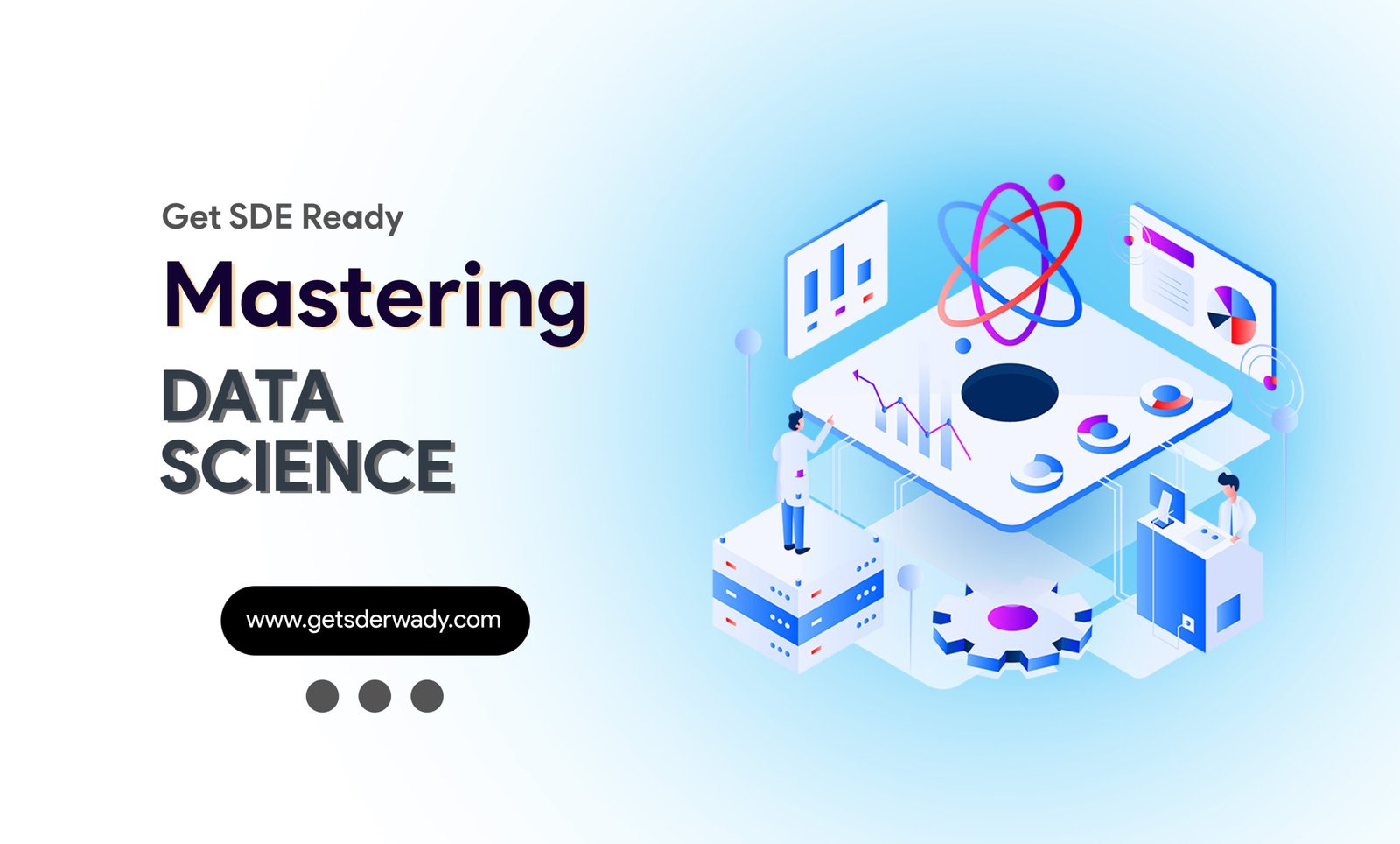
Essentials of Machine Learning and Artificial Intelligence
- 65+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 22+ Hands-on Live Projects & Deployments
- Comprehensive Notes
- Topic-wise Quizzes
- Case Studies
- Access to Global Peer Community
- Interview Prep Material
Buy for 65% OFF
₹20,000.00 ₹6,999.00
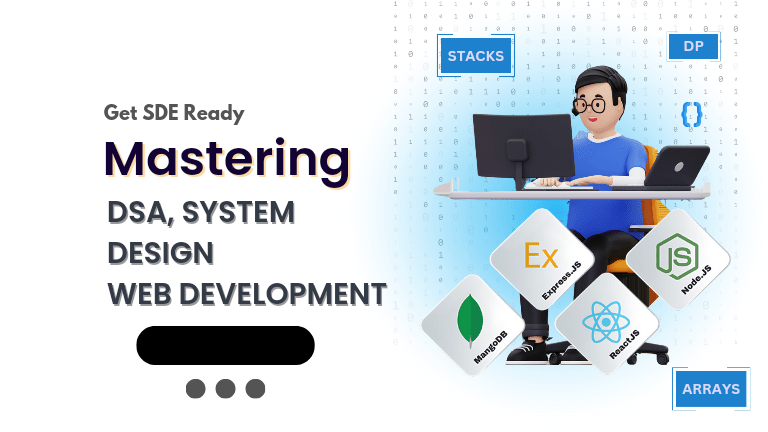
Fast-Track to Full Spectrum Software Engineering
- 120+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 400+ DSA Practice Questions
- Comprehensive Notes
- HackerRank Tests & Quizzes
- 12+ live Projects & Deployments
- Case Studies
- Access to Global Peer Community
Buy for 57% OFF
₹35,000.00 ₹14,999.00
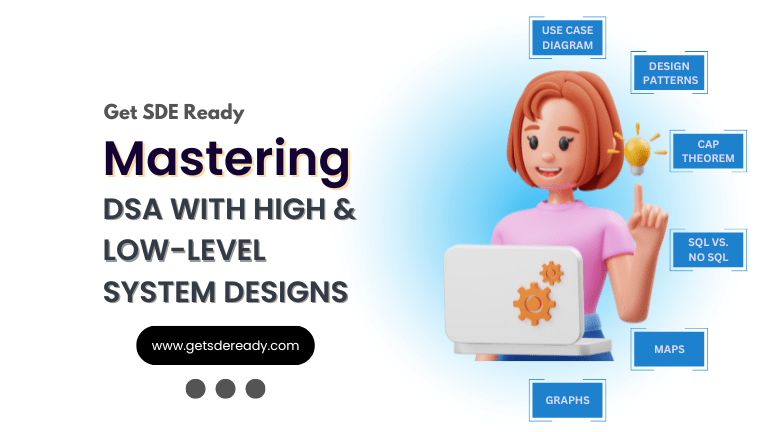
DSA, High & Low Level System Designs
- 85+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 400+ DSA Practice Questions
- Comprehensive Notes
- HackerRank Tests & Quizzes
- Topic-wise Quizzes
- Case Studies
- Access to Global Peer Community
Buy for 60% OFF
₹25,000.00 ₹9,999.00
Reach Out Now
If you have any queries, please fill out this form. We will surely reach out to you.
Contact Email
Reach us at the following email address.
Phone Number
You can reach us by phone as well.
+91-97737 28034
Our Location
Rohini, Sector-3, Delhi-110085