Data Structures and Algorithms
- Introduction to Data Structures and Algorithms
- Time and Space Complexity Analysis
- Big-O, Big-Theta, and Big-Omega Notations
- Recursion and Backtracking
- Divide and Conquer Algorithm
- Dynamic Programming: Memoization vs. Tabulation
- Greedy Algorithms and Their Use Cases
- Understanding Arrays: Types and Operations
- Linear Search vs. Binary Search
- Sorting Algorithms: Bubble, Insertion, Selection, and Merge Sort
- QuickSort: Explanation and Implementation
- Heap Sort and Its Applications
- Counting Sort, Radix Sort, and Bucket Sort
- Hashing Techniques: Hash Tables and Collisions
- Open Addressing vs. Separate Chaining in Hashing
- DSA Questions for Beginners
- Advanced DSA Questions for Competitive Programming
- Top 10 DSA Questions to Crack Your Next Coding Test
- Top 50 DSA Questions Every Programmer Should Practice
- Top Atlassian DSA Interview Questions
- Top Amazon DSA Interview Questions
- Top Microsoft DSA Interview Questions
- Top Meta (Facebook) DSA Interview Questions
- Netflix DSA Interview Questions and Preparation Guide
- Top 20 DSA Interview Questions You Need to Know
- Top Uber DSA Interview Questions and Solutions
- Google DSA Interview Questions and How to Prepare
- Airbnb DSA Interview Questions and How to Solve Them
- Mobile App DSA Interview Questions and Solutions
DSA Interview Questions
- DSA Questions for Beginners
- Advanced DSA Questions for Competitive Programming
- Top 10 DSA Questions to Crack Your Next Coding Test
- Top 50 DSA Questions Every Programmer Should Practice
- Top Atlassian DSA Interview Questions
- Top Amazon DSA Interview Questions
- Top Microsoft DSA Interview Questions
- Top Meta (Facebook) DSA Interview Questions
- Netflix DSA Interview Questions and Preparation Guide
- Top 20 DSA Interview Questions You Need to Know
- Top Uber DSA Interview Questions and Solutions
- Google DSA Interview Questions and How to Prepare
- Airbnb DSA Interview Questions and How to Solve Them
- Mobile App DSA Interview Questions and Solutions
Introduction to High-Level System Design
System Design Fundamentals
- Functional vs. Non-Functional Requirements
- Scalability, Availability, and Reliability
- Latency and Throughput Considerations
- Load Balancing Strategies
Architectural Patterns
- Monolithic vs. Microservices Architecture
- Layered Architecture
- Event-Driven Architecture
- Serverless Architecture
- Model-View-Controller (MVC) Pattern
- CQRS (Command Query Responsibility Segregation)
Scaling Strategies
- Vertical Scaling vs. Horizontal Scaling
- Sharding and Partitioning
- Data Replication and Consistency Models
- Load Balancing Strategies
- CDN and Edge Computing
Database Design in HLD
- SQL vs. NoSQL Databases
- CAP Theorem and its Impact on System Design
- Database Indexing and Query Optimization
- Database Sharding and Partitioning
- Replication Strategies
API Design and Communication
Caching Strategies
- Types of Caching
- Cache Invalidation Strategies
- Redis vs. Memcached
- Cache-Aside, Write-Through, and Write-Behind Strategies
Message Queues and Event-Driven Systems
- Kafka vs. RabbitMQ vs. SQS
- Pub-Sub vs. Point-to-Point Messaging
- Handling Asynchronous Workloads
- Eventual Consistency in Distributed Systems
Security in System Design
Observability and Monitoring
- Logging Strategies (ELK Stack, Prometheus, Grafana)
- API Security Best Practices
- Secure Data Storage and Access Control
- DDoS Protection and Rate Limiting
Real-World System Design Case Studies
- Distributed locking (Locking and its Types)
- Memory leaks and Out of memory issues
- HLD of YouTube
- HLD of WhatsApp
System Design Interview Questions
- Adobe System Design Interview Questions
- Top Atlassian System Design Interview Questions
- Top Amazon System Design Interview Questions
- Top Microsoft System Design Interview Questions
- Top Meta (Facebook) System Design Interview Questions
- Top Netflix System Design Interview Questions
- Top Uber System Design Interview Questions
- Top Google System Design Interview Questions
- Top Apple System Design Interview Questions
- Top Airbnb System Design Interview Questions
- Top 10 System Design Interview Questions
- Mobile App System Design Interview Questions
- Top 20 Stripe System Design Interview Questions
- Top Shopify System Design Interview Questions
- Top 20 System Design Interview Questions
- Top Advanced System Design Questions
- Most-Frequented System Design Questions in Big Tech Interviews
- What Interviewers Look for in System Design Questions
- Critical System Design Questions to Crack Any Tech Interview
- Top 20 API Design Questions for System Design Interviews
- Top 10 Steps to Create a System Design Portfolio for Developers
Longest Consecutive Subsequence: O(n) Algorithm Optimization
Problem Statement
Given an unsorted array of integers, the task is to return the length of the longest consecutive elements sequence while ensuring an algorithm with O(n) time complexity. The goal is to calculate the longest chain of consecutive numbers present in the array.
Key constraints include:
- 0 ≤ nums.length ≤ 10^5
- -10^9 ≤ nums[i] ≤ 10^9
This guide explains various approaches to solving the problem, including naive, sorting, and optimal HashSet-based methods.
Detailed Examples and Explanations
Example 1
Input: nums = [100, 4, 200, 1, 3, 2]
Output: 4
Explanation: The longest consecutive sequence is [1, 2, 3, 4].
Natural question: How do you determine the start of a sequence?
By checking if the preceding number (num – 1) does not exist in the array, we can confirm the beginning of a consecutive chain.
Example 2
Input: nums = [0, 3, 7, 2, 5, 8, 4, 6, 0, 1]
Output: 9
Explanation: Although duplicates exist, they are ignored. The consecutive sequence is effectively [0, 1, 2, 3, 4, 5, 6, 7, 8].
Example 3
Input: nums = [1, 0, 1, 2]
Output: 3
Explanation: The consecutive sequence is [0, 1, 2]. Duplicate entries are not counted more than once.
Approaches and Algorithm Optimization
Naive Approach
The intuitive method involves iterating through each element and checking if the next consecutive element exists in the array. Although straightforward, it has a worst-case time complexity of O(n²) due to repeated searches.
Step-by-Step Process:
- Iterate over each element.
- For each element, check if the next consecutive number exists.
- Count consecutive numbers and update the maximum length.
Issue : Inefficient for large inputs due to repeated searching.
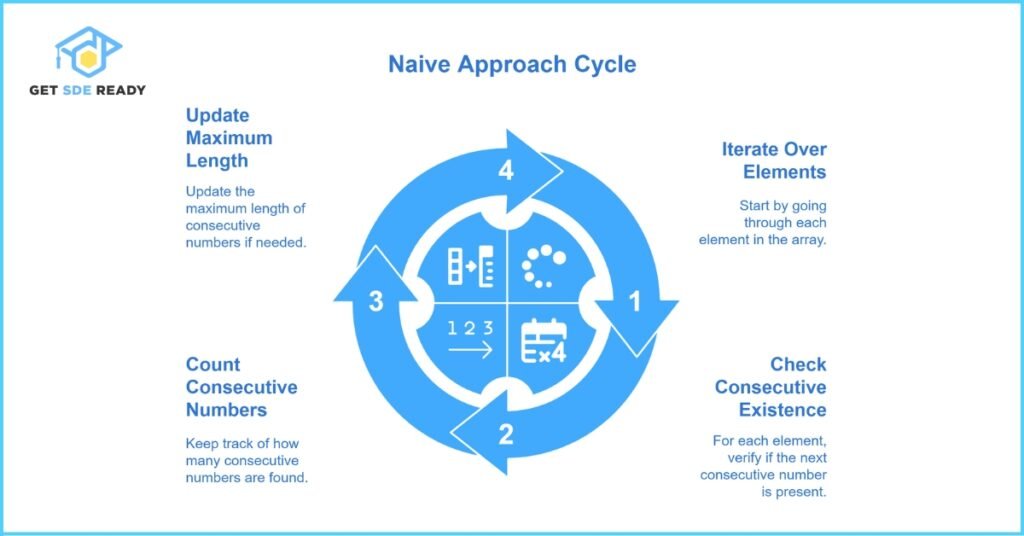
Optimization 1: Sorting (O(n log n))
Sorting the array can significantly reduce the number of lookups required.
Approach:
- Sort the Array: This operation takes O(n log n).
Â
- Count Consecutive Sequences: Iterate through the sorted array, count consecutive numbers, and handle duplicates.
Sample C# Code:
public class Solution {
public int LongestConsecutive(int[] nums) {
if (nums.Length == 0) return 0;
Array.Sort(nums); // O(n log n)
int maxLength = 1, count = 1;
for (int i = 1; i < nums.Length; i++) {
if (nums[i] == nums[i - 1])
continue; // Skip duplicates
if (nums[i] == nums[i - 1] + 1)
count++;
else {
maxLength = Math.Max(maxLength, count);
count = 1; // Reset count
}
}
return Math.Max(maxLength, count);
}
}
Optimal Approach: Using HashSet (O(n))
To meet O(n) time complexity, a HashSet is used for quick lookups.
Approach:
- Build a HashSet: Insert all elements for O(1) lookup.
- Identify Sequence Starts: Iterate through each number and start a sequence only if the previous number (num – 1) is not in the HashSet.
- Count Consecutive Elements: For each starting number, count the consecutive sequence length by checking subsequent numbers in the HashSet.
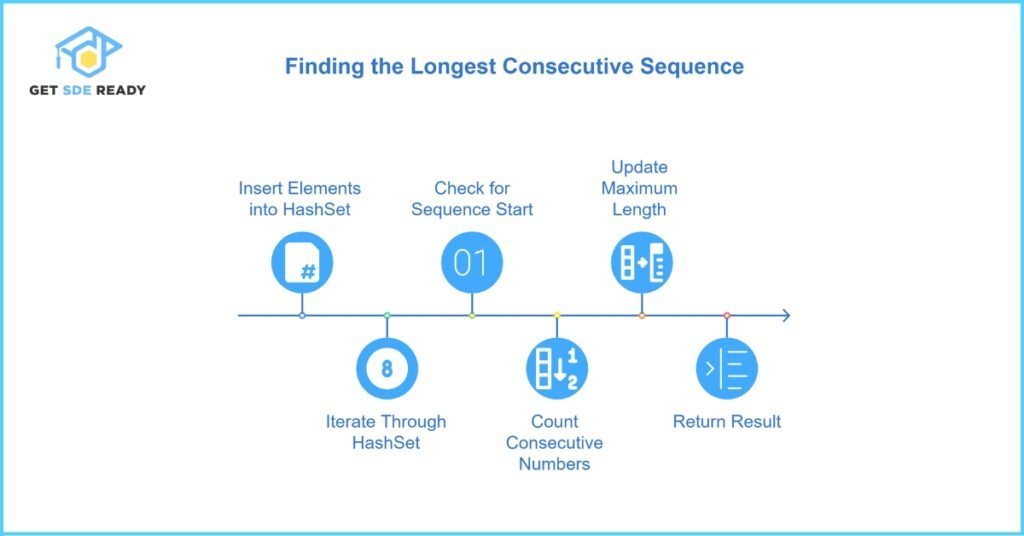
Sample C# Code:
public class Solution {
public int LongestConsecutive(int[] nums) {
if (nums.Length == 0) return 0;
HashSet numSet = new HashSet(nums);
int maxLength = 0;
foreach (int num in numSet) {
if (!numSet.Contains(num - 1)) { // Only start counting if num-1 is not present
int currentNum = num;
int count = 1;
while (numSet.Contains(currentNum + 1)) {
currentNum++;
count++;
}
maxLength = Math.Max(maxLength, count);
}
}
return maxLength;
}
}
Dry Run of the HashSet Approach
For the input nums = [100, 4, 200, 1, 3, 2]:
- Step 1: Insert all elements into a HashSet: {100, 4, 200, 1, 3, 2}.
Â
- Step 2: Iterate through each element.
Â
- For element 1, since 0 is not in the set, begin counting and find the sequence [1, 2, 3, 4].
Â
- Elements that are part of an already counted sequence are skipped.
Â
Result: The longest consecutive sequence is of length 4.
Sample C# Code:
Code Implementations in Different Languages
C# Implementation
(See above for detailed C# code examples)
JavaScript Implementation
var longestConsecutive = function(nums) {
if (nums.length === 0) return 0;
let maxLength = 0;
for (let num of nums) {
let currentNum = num;
let count = 1;
while (nums.includes(currentNum + 1)) { // Searching for the next number
currentNum++;
count++;
}
maxLength = Math.max(maxLength, count);
}
return maxLength;
};
Python Implementation
def longestConsecutive(nums):
if not nums:
return 0
max_length = 0
for num in nums:
current_num = num
count = 1
while current_num + 1 in nums: # Searching for the next number
current_num += 1
count += 1
max_length = max(max_length, count)
return max_length
Final Thoughts
This problem illustrates the importance of selecting the right data structure to optimize algorithm performance. The transition from a naive O(n²) approach to a more refined O(n) solution using HashSet shows how minor adjustments can lead to significant improvements in efficiency. This knowledge is beneficial for technical interviews and for understanding practical optimization techniques.
What Should I Focus on for Airbnb DSA Interviews?
When preparing for Airbnb DSA interviews, focus on mastering the fundamentals of data structures and algorithms. This includes arrays, linked lists, trees, graphs, dynamic programming, and sorting techniques. A well-structured study plan that includes hands-on practice with real-world problems will boost your confidence and performance. For a detailed course on DSA fundamentals, check out this course.
How Important Is Code Optimization in DSA Interviews?
Code optimization is crucial during DSA interviews because it reflects your ability to write efficient and scalable solutions. Interviewers are not only interested in getting the correct answer but also in understanding your thought process and how you handle large inputs. Ensuring your solution runs within optimal time and space limits is essential for success. To learn more about optimizing your coding techniques, explore this web development course.
Can Practicing on Paper Improve My Interview Performance?
Absolutely, practicing on paper or a whiteboard can significantly improve your interview performance. It helps simulate the real interview environment where you need to articulate your thought process without the aid of an IDE. Regular practice in this format enables you to organize your ideas better and communicate more effectively under pressure. For more insights on preparing for technical interviews, consider checking out this combined DSA and design course.
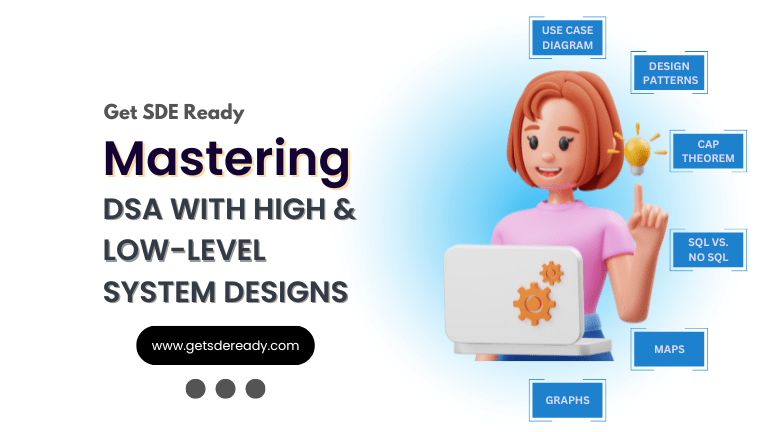
DSA, High & Low Level System Designs
- 85+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 400+ DSA Practice Questions
- Comprehensive Notes
- HackerRank Tests & Quizzes
- Topic-wise Quizzes
- Case Studies
- Access to Global Peer Community
Buy for 60% OFF
₹25,000.00 ₹9,999.00
Accelerate your Path to a Product based Career
Boost your career or get hired at top product-based companies by joining our expertly crafted courses. Gain practical skills and real-world knowledge to help you succeed.
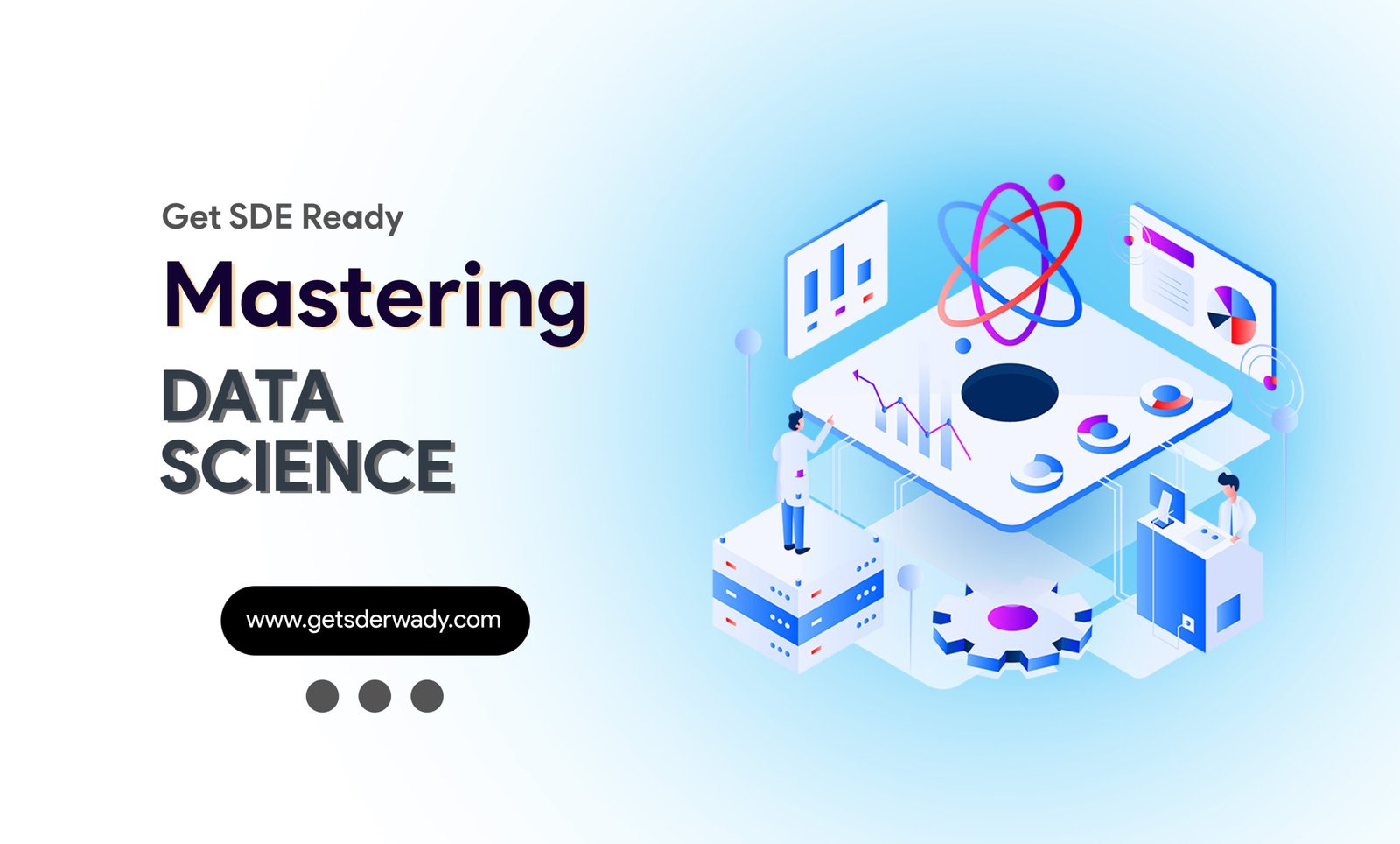
Essentials of Machine Learning and Artificial Intelligence
- 65+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 22+ Hands-on Live Projects & Deployments
- Comprehensive Notes
- Topic-wise Quizzes
- Case Studies
- Access to Global Peer Community
- Interview Prep Material
Buy for 65% OFF
₹20,000.00 ₹6,999.00
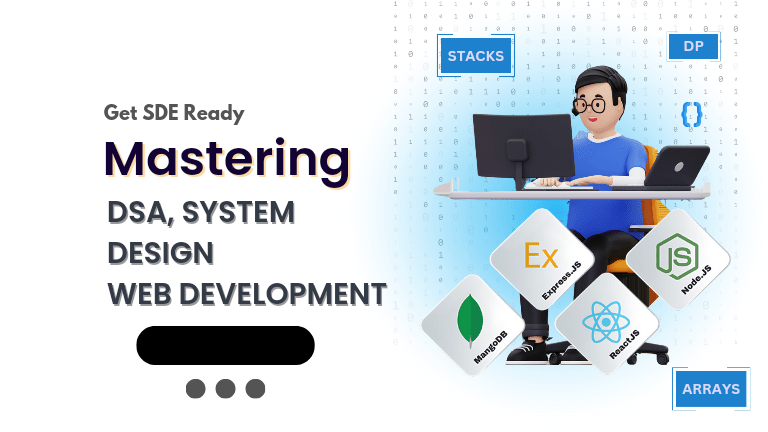
Fast-Track to Full Spectrum Software Engineering
- 120+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 400+ DSA Practice Questions
- Comprehensive Notes
- HackerRank Tests & Quizzes
- 12+ live Projects & Deployments
- Case Studies
- Access to Global Peer Community
Buy for 57% OFF
₹35,000.00 ₹14,999.00
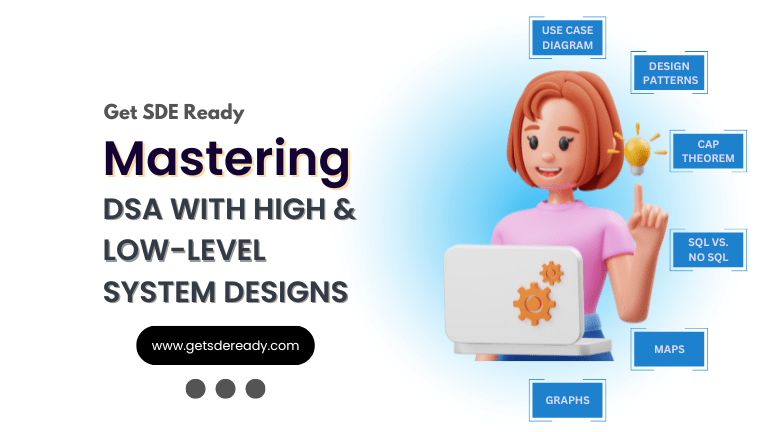
DSA, High & Low Level System Designs
- 85+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 400+ DSA Practice Questions
- Comprehensive Notes
- HackerRank Tests & Quizzes
- Topic-wise Quizzes
- Case Studies
- Access to Global Peer Community
Buy for 60% OFF
₹25,000.00 ₹9,999.00

Low & High Level System Design
- 20+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 400+ DSA Practice Questions
- Comprehensive Notes
- HackerRank Tests
- Topic-wise Quizzes
- Access to Global Peer Community
- Interview Prep Material
Buy for 65% OFF
₹20,000.00 ₹6,999.00
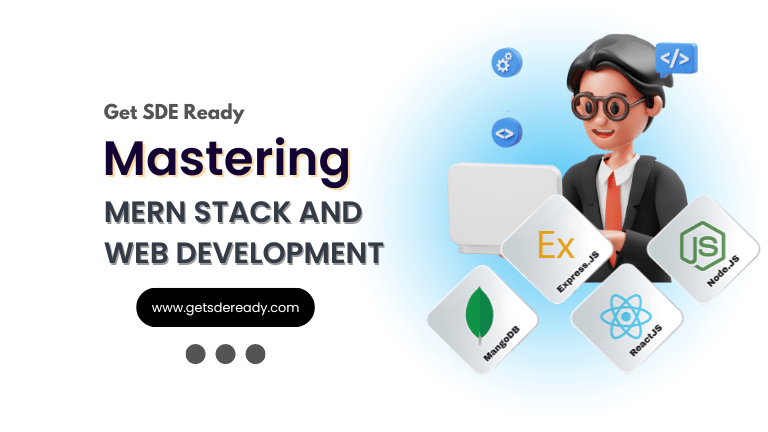
Mastering Mern Stack (WEB DEVELOPMENT)
- 65+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 12+ Hands-on Live Projects & Deployments
- Comprehensive Notes & Quizzes
- Real-world Tools & Technologies
- Access to Global Peer Community
- Interview Prep Material
- Placement Assistance
Buy for 60% OFF
₹15,000.00 ₹5,999.00
Reach Out Now
If you have any queries, please fill out this form. We will surely reach out to you.
Contact Email
Reach us at the following email address.
Phone Number
You can reach us by phone as well.
+91-97737 28034
Our Location
Rohini, Sector-3, Delhi-110085