Data Structures and Algorithms
- Introduction to Data Structures and Algorithms
- Time and Space Complexity Analysis
- Big-O, Big-Theta, and Big-Omega Notations
- Recursion and Backtracking
- Divide and Conquer Algorithm
- Dynamic Programming: Memoization vs. Tabulation
- Greedy Algorithms and Their Use Cases
- Understanding Arrays: Types and Operations
- Linear Search vs. Binary Search
- Sorting Algorithms: Bubble, Insertion, Selection, and Merge Sort
- QuickSort: Explanation and Implementation
- Heap Sort and Its Applications
- Counting Sort, Radix Sort, and Bucket Sort
- Hashing Techniques: Hash Tables and Collisions
- Open Addressing vs. Separate Chaining in Hashing
- DSA Questions for Beginners
- Advanced DSA Questions for Competitive Programming
- Top 10 DSA Questions to Crack Your Next Coding Test
- Top 50 DSA Questions Every Programmer Should Practice
- Top Atlassian DSA Interview Questions
- Top Amazon DSA Interview Questions
- Top Microsoft DSA Interview Questions
- Top Meta (Facebook) DSA Interview Questions
- Netflix DSA Interview Questions and Preparation Guide
- Top 20 DSA Interview Questions You Need to Know
- Top Uber DSA Interview Questions and Solutions
- Google DSA Interview Questions and How to Prepare
- Airbnb DSA Interview Questions and How to Solve Them
- Mobile App DSA Interview Questions and Solutions
DSA Interview Questions
- DSA Questions for Beginners
- Advanced DSA Questions for Competitive Programming
- Top 10 DSA Questions to Crack Your Next Coding Test
- Top 50 DSA Questions Every Programmer Should Practice
- Top Atlassian DSA Interview Questions
- Top Amazon DSA Interview Questions
- Top Microsoft DSA Interview Questions
- Top Meta (Facebook) DSA Interview Questions
- Netflix DSA Interview Questions and Preparation Guide
- Top 20 DSA Interview Questions You Need to Know
- Top Uber DSA Interview Questions and Solutions
- Google DSA Interview Questions and How to Prepare
- Airbnb DSA Interview Questions and How to Solve Them
- Mobile App DSA Interview Questions and Solutions
Data Structures and Algorithms
- Introduction to Data Structures and Algorithms
- Time and Space Complexity Analysis
- Big-O, Big-Theta, and Big-Omega Notations
- Recursion and Backtracking
- Divide and Conquer Algorithm
- Dynamic Programming: Memoization vs. Tabulation
- Greedy Algorithms and Their Use Cases
- Understanding Arrays: Types and Operations
- Linear Search vs. Binary Search
- Sorting Algorithms: Bubble, Insertion, Selection, and Merge Sort
- QuickSort: Explanation and Implementation
- Heap Sort and Its Applications
- Counting Sort, Radix Sort, and Bucket Sort
- Hashing Techniques: Hash Tables and Collisions
- Open Addressing vs. Separate Chaining in Hashing
- DSA Questions for Beginners
- Advanced DSA Questions for Competitive Programming
- Top 10 DSA Questions to Crack Your Next Coding Test
- Top 50 DSA Questions Every Programmer Should Practice
- Top Atlassian DSA Interview Questions
- Top Amazon DSA Interview Questions
- Top Microsoft DSA Interview Questions
- Top Meta (Facebook) DSA Interview Questions
- Netflix DSA Interview Questions and Preparation Guide
- Top 20 DSA Interview Questions You Need to Know
- Top Uber DSA Interview Questions and Solutions
- Google DSA Interview Questions and How to Prepare
- Airbnb DSA Interview Questions and How to Solve Them
- Mobile App DSA Interview Questions and Solutions
DSA Interview Questions
- DSA Questions for Beginners
- Advanced DSA Questions for Competitive Programming
- Top 10 DSA Questions to Crack Your Next Coding Test
- Top 50 DSA Questions Every Programmer Should Practice
- Top Atlassian DSA Interview Questions
- Top Amazon DSA Interview Questions
- Top Microsoft DSA Interview Questions
- Top Meta (Facebook) DSA Interview Questions
- Netflix DSA Interview Questions and Preparation Guide
- Top 20 DSA Interview Questions You Need to Know
- Top Uber DSA Interview Questions and Solutions
- Google DSA Interview Questions and How to Prepare
- Airbnb DSA Interview Questions and How to Solve Them
- Mobile App DSA Interview Questions and Solutions
Priority Queues in C++ STL
Priority queues are a powerful data structure in the C++ Standard Template Library (STL), enabling efficient management of elements based on their priority. Whether you’re preparing for coding interviews or developing applications that require ordered processing, understanding priority queues is essential. In this comprehensive guide, we’ll explore their syntax, operations, internal workings, and more, complete with examples and practical insights. Want to elevate your skills? Sign up for our free courses or get the latest updates via our lead capture form and start mastering data structures today!
Introduction to Priority Queues
In the world of data structures, priority queues shine by prioritizing elements based on their significance rather than their order of arrival. Unlike a standard queue that adheres to the First-In-First-Out (FIFO) principle, a priority queue ensures the highest-priority element is always processed first. This makes them invaluable in scenarios like task scheduling in operating systems, where urgent tasks take precedence, or in Dijkstra’s algorithm for finding the shortest path.
Understanding Priority Queues in C++ STL
The C++ STL provides std::priority_queue, a container adaptor that efficiently manages prioritized elements. Its syntax is:
std::priority_queue<T, Container, Compare> pq;
- T: The type of elements (e.g., int, double).
- Container: The underlying container, defaulting to std::vector<T>.
- Compare: A comparator defining priority order, defaulting to std::less<T> (max-heap, where the largest element has the highest priority).
Internally, it leverages a binary heap—typically a max-heap—ensuring the top element is the greatest. You can switch to a min-heap using std::greater<T> or define a custom comparator for unique priority schemes. For an in-depth exploration, check out our DSA course.
Types of Heaps
- Max-Heap: Largest element at the top (default).
- Min-Heap: Smallest element at the top (with std::greater<T>).
Declaring and Initializing Priority Queues
Priority queues offer flexible declaration and initialization options:
Creating an Empty Priority Queue
#include
#include
int main() {
std::priority_queue pq;
return 0;
}
Inserting Elements
pq.push(5);
pq.push(1);
pq.push(10); // Top element becomes 10
Initializing from Another Container
#include
std::vector vec = {3, 1, 4, 1, 5};
std::priority_queue pq(vec.begin(), vec.end()); // Top element is 5
Using a Custom Comparator (Min-Heap)
std::priority_queue, std::greater> min_pq;
min_pq.push(5);
min_pq.push(1);
min_pq.push(10); // Top element is 1
Inserting Elements (push)
The push method adds an element and adjusts the heap.
pq.push(7); // O(log n)
Accessing the Top Element (top)
The top method retrieves the highest-priority element.
int highest = pq.top(); // O(1), returns 10 if 10 is the largest
Deleting Elements (pop)
The pop method removes the top element.
pq.pop(); // O(log n), removes 10, next highest becomes top
Pseudo Traversal
To access all elements, copy the queue and pop iteratively:
pq.pop(); // O(log n), removes 10, next highest becomes top
Pseudo Traversal
To access all elements, copy the queue and pop iteratively:
std::priority_queue temp = pq;
while (!temp.empty()) {
std::cout << temp.top() << " "; // Prints elements in priority order
temp.pop();
}
Changing Priority Order
Create a min-heap or use a custom comparator:
std::priority_queue, std::greater> min_pq;
Master these operations with our Crash Course.
Time Complexity of Operations
Operation | Time Complexity |
push (insert) | O(log n) |
pop (delete) | O(log n) |
top (access) | O(1) |
size | O(1) |
empty | O(1) |
Initialization | O(n) |
The logarithmic complexity arises from heap rebalancing. Dive deeper into complexities with our Data Science course.
Other Common Operations
Finding the Size
size_t num = pq.size(); // O(1)
Checking if Empty
if (pq.empty()) std::cout << "Empty\n"; // O(1)
Reversing Priority
Switch comparators (e.g., std::less to std::greater).
Priority Queue of Tuples
using Tuple = std::tuple;
auto comp = [](const Tuple& a, const Tuple& b) { return std::get<0>(a) < std::get<0>(b); };
std::priority_queue, decltype(comp)> tuple_pq(comp);
Custom Priority Criteria
Define your own comparator for complex logic.
Swapping Priority Queues
pq1.swap(pq2); // O(1)
Explore advanced usage in our Master DSA, Web Dev, and System Design course.
Priority Queue vs. Queue
- Queue (std::queue): FIFO, order-based processing (e.g., BFS).
- Priority Queue: Priority-based, highest priority first (e.g., task scheduling).
For practical examples, see our Top Netflix DSA Interview Questions.
Member Functions of std::priority_queue
Function | Description |
empty() | Checks if the queue is empty. |
size() | Returns the number of elements. |
top() | Accesses the top element. |
push() | Adds an element. |
pop() | Removes the top element. |
swap() | Swaps contents with another queue. |
Details are available in our Essential DSA Web Dev Courses for Programmers.
FAQs
Can I use a priority queue with custom objects?
Yes, define a comparator for your objects. Learn how in our DSA course.
How do I create a min-heap priority queue?
Use std::greater<T>: std::priority_queue<int, std::vector<int>, std::greater<int>>. See our Web Development course.
Is it possible to change an element’s priority after insertion?
Not directly—remove and re-insert it. Explore solutions in our Design and DSA Combined course.
Can I iterate through a priority queue?
No direct iteration; copy and pop elements. Alternatives are in our Master DSA, Web Dev, and System Design course.
What are real-world applications of priority queues?
Task scheduling, Dijkstra’s algorithm, and Huffman coding. Dive into these in our Data Science course.
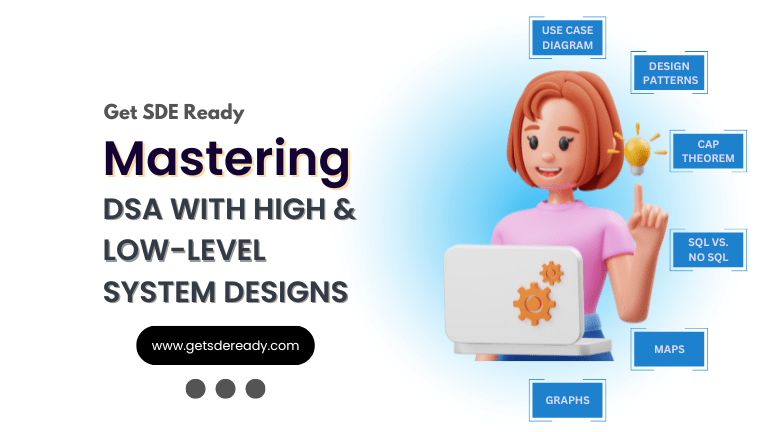
DSA, High & Low Level System Designs
- 85+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 400+ DSA Practice Questions
- Comprehensive Notes
- HackerRank Tests & Quizzes
- Topic-wise Quizzes
- Case Studies
- Access to Global Peer Community
Buy for 60% OFF
₹25,000.00 ₹9,999.00
Accelerate your Path to a Product based Career
Boost your career or get hired at top product-based companies by joining our expertly crafted courses. Gain practical skills and real-world knowledge to help you succeed.
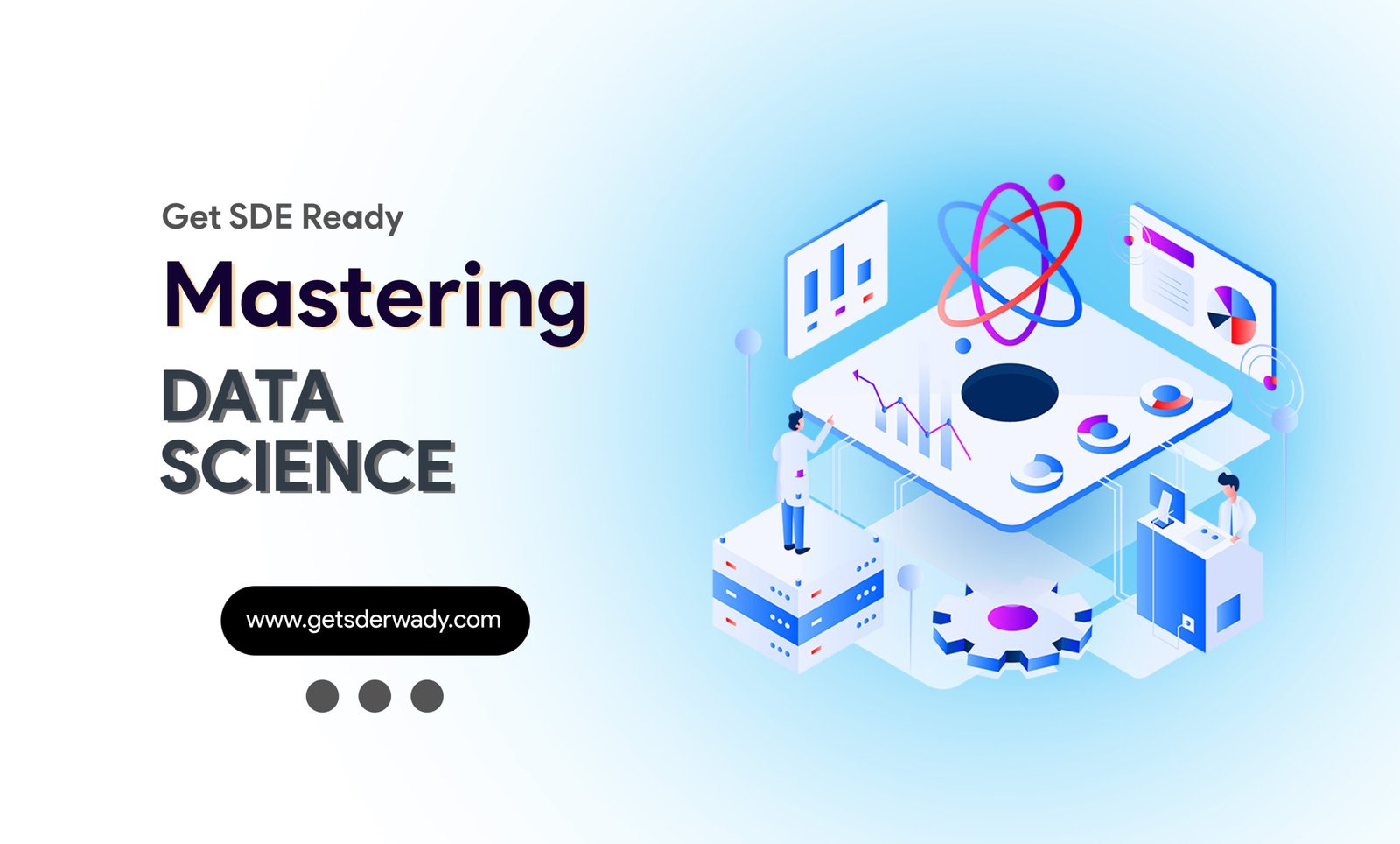
Essentials of Machine Learning and Artificial Intelligence
- 65+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 22+ Hands-on Live Projects & Deployments
- Comprehensive Notes
- Topic-wise Quizzes
- Case Studies
- Access to Global Peer Community
- Interview Prep Material
Buy for 65% OFF
₹20,000.00 ₹6,999.00
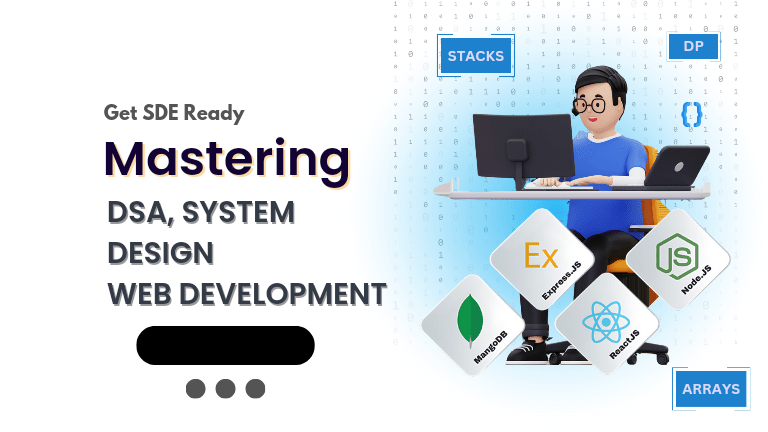
Fast-Track to Full Spectrum Software Engineering
- 120+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 400+ DSA Practice Questions
- Comprehensive Notes
- HackerRank Tests & Quizzes
- 12+ live Projects & Deployments
- Case Studies
- Access to Global Peer Community
Buy for 57% OFF
₹35,000.00 ₹14,999.00
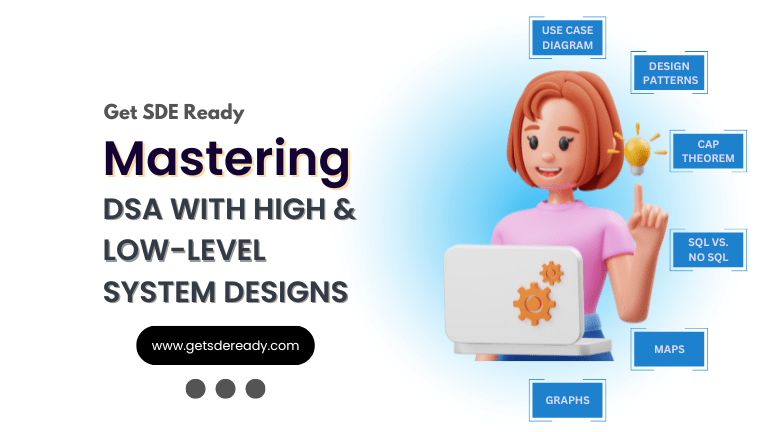
DSA, High & Low Level System Designs
- 85+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 400+ DSA Practice Questions
- Comprehensive Notes
- HackerRank Tests & Quizzes
- Topic-wise Quizzes
- Case Studies
- Access to Global Peer Community
Buy for 60% OFF
₹25,000.00 ₹9,999.00

Low & High Level System Design
- 20+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 400+ DSA Practice Questions
- Comprehensive Notes
- HackerRank Tests
- Topic-wise Quizzes
- Access to Global Peer Community
- Interview Prep Material
Buy for 65% OFF
₹20,000.00 ₹6,999.00
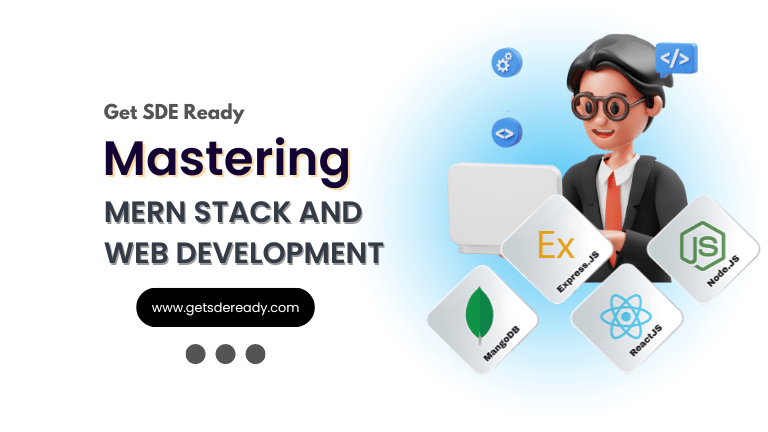
Mastering Mern Stack (WEB DEVELOPMENT)
- 65+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 12+ Hands-on Live Projects & Deployments
- Comprehensive Notes & Quizzes
- Real-world Tools & Technologies
- Access to Global Peer Community
- Interview Prep Material
- Placement Assistance
Buy for 60% OFF
₹15,000.00 ₹5,999.00

Mastering Data Structures & Algorithms
- 65+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 400+ DSA Practice Questions
- Comprehensive Notes
- HackerRank Tests
- Access to Global Peer Community
- Topic-wise Quizzes
- Interview Prep Material
Buy for 50% OFF
₹9,999.00 ₹4,999.00
Reach Out Now
If you have any queries, please fill out this form. We will surely reach out to you.
Contact Email
Reach us at the following email address.
arun@getsdeready.com
Phone Number
You can reach us by phone as well.
+91-97737 28034
Our Location
Rohini, Sector-3, Delhi-110085