Data Structures and Algorithms
- Introduction to Data Structures and Algorithms
- Time and Space Complexity Analysis
- Big-O, Big-Theta, and Big-Omega Notations
- Recursion and Backtracking
- Divide and Conquer Algorithm
- Dynamic Programming: Memoization vs. Tabulation
- Greedy Algorithms and Their Use Cases
- Understanding Arrays: Types and Operations
- Linear Search vs. Binary Search
- Sorting Algorithms: Bubble, Insertion, Selection, and Merge Sort
- QuickSort: Explanation and Implementation
- Heap Sort and Its Applications
- Counting Sort, Radix Sort, and Bucket Sort
- Hashing Techniques: Hash Tables and Collisions
- Open Addressing vs. Separate Chaining in Hashing
- DSA Questions for Beginners
- Advanced DSA Questions for Competitive Programming
- Top 10 DSA Questions to Crack Your Next Coding Test
- Top 50 DSA Questions Every Programmer Should Practice
- Top Atlassian DSA Interview Questions
- Top Amazon DSA Interview Questions
- Top Microsoft DSA Interview Questions
- Top Meta (Facebook) DSA Interview Questions
- Netflix DSA Interview Questions and Preparation Guide
- Top 20 DSA Interview Questions You Need to Know
- Top Uber DSA Interview Questions and Solutions
- Google DSA Interview Questions and How to Prepare
- Airbnb DSA Interview Questions and How to Solve Them
- Mobile App DSA Interview Questions and Solutions
DSA Interview Questions
- DSA Questions for Beginners
- Advanced DSA Questions for Competitive Programming
- Top 10 DSA Questions to Crack Your Next Coding Test
- Top 50 DSA Questions Every Programmer Should Practice
- Top Atlassian DSA Interview Questions
- Top Amazon DSA Interview Questions
- Top Microsoft DSA Interview Questions
- Top Meta (Facebook) DSA Interview Questions
- Netflix DSA Interview Questions and Preparation Guide
- Top 20 DSA Interview Questions You Need to Know
- Top Uber DSA Interview Questions and Solutions
- Google DSA Interview Questions and How to Prepare
- Airbnb DSA Interview Questions and How to Solve Them
- Mobile App DSA Interview Questions and Solutions
Introduction to High-Level System Design
System Design Fundamentals
- Functional vs. Non-Functional Requirements
- Scalability, Availability, and Reliability
- Latency and Throughput Considerations
- Load Balancing Strategies
Architectural Patterns
- Monolithic vs. Microservices Architecture
- Layered Architecture
- Event-Driven Architecture
- Serverless Architecture
- Model-View-Controller (MVC) Pattern
- CQRS (Command Query Responsibility Segregation)
Scaling Strategies
- Vertical Scaling vs. Horizontal Scaling
- Sharding and Partitioning
- Data Replication and Consistency Models
- Load Balancing Strategies
- CDN and Edge Computing
Database Design in HLD
- SQL vs. NoSQL Databases
- CAP Theorem and its Impact on System Design
- Database Indexing and Query Optimization
- Database Sharding and Partitioning
- Replication Strategies
API Design and Communication
Caching Strategies
- Types of Caching
- Cache Invalidation Strategies
- Redis vs. Memcached
- Cache-Aside, Write-Through, and Write-Behind Strategies
Message Queues and Event-Driven Systems
- Kafka vs. RabbitMQ vs. SQS
- Pub-Sub vs. Point-to-Point Messaging
- Handling Asynchronous Workloads
- Eventual Consistency in Distributed Systems
Security in System Design
Observability and Monitoring
- Logging Strategies (ELK Stack, Prometheus, Grafana)
- API Security Best Practices
- Secure Data Storage and Access Control
- DDoS Protection and Rate Limiting
Real-World System Design Case Studies
- Distributed locking (Locking and its Types)
- Memory leaks and Out of memory issues
- HLD of YouTube
- HLD of WhatsApp
System Design Interview Questions
- Adobe System Design Interview Questions
- Top Atlassian System Design Interview Questions
- Top Amazon System Design Interview Questions
- Top Microsoft System Design Interview Questions
- Top Meta (Facebook) System Design Interview Questions
- Top Netflix System Design Interview Questions
- Top Uber System Design Interview Questions
- Top Google System Design Interview Questions
- Top Apple System Design Interview Questions
- Top Airbnb System Design Interview Questions
- Top 10 System Design Interview Questions
- Mobile App System Design Interview Questions
- Top 20 Stripe System Design Interview Questions
- Top Shopify System Design Interview Questions
- Top 20 System Design Interview Questions
- Top Advanced System Design Questions
- Most-Frequented System Design Questions in Big Tech Interviews
- What Interviewers Look for in System Design Questions
- Critical System Design Questions to Crack Any Tech Interview
- Top 20 API Design Questions for System Design Interviews
- Top 10 Steps to Create a System Design Portfolio for Developers
Mastering the Stack Data Structure: A Complete Guide
The stack data structure is a cornerstone of programming, offering a simple yet powerful way to manage data. Whether you’re reversing a string, handling browser history, or managing function calls, stacks are essential. In this guide, we’ll explore everything you need to know about stacks—from their core principles to real-world applications and implementations. By the end, you’ll have a deep understanding of how stacks work and why they’re so vital in computer science.
Ready to level up your coding skills and ace your next interview? Stay ahead of the game by signing up for the latest updates on our cutting-edge courses and resources!
What is a Stack Data Structure?
A stack is a linear data structure that organizes elements in a specific order: Last In, First Out (LIFO). This means the last element added to the stack is the first one to be removed. Think of it like a stack of plates—you add and remove plates from the top.
In programming, stacks are used to manage data where the order of operations matters. They’re crucial for tasks like reversing sequences, temporarily holding data, and managing function calls in virtually every programming language.

Real-World Example of a Stack
Consider a web browser’s back button. Each time you visit a new page, its URL is added (or “pushed”) to a stack. When you click “back,” the most recent URL is removed (or “popped”) from the stack, taking you to the previous page. This LIFO approach ensures you always return to the last page you visited first.
Discover more practical examples in our comprehensive DSA course.
Understanding the LIFO Principle in Stacks
The Last In, First Out (LIFO) principle is the foundation of how stacks operate. It dictates that the most recently added element is always the first to be removed. This principle is enforced through two primary operations:
- Push: Adds an element to the top of the stack.
- Pop: Removes the top element from the stack.
These operations ensure that only the most recent data is accessible, making stacks ideal for scenarios where the order of operations is critical.
For a deeper dive into LIFO and other ordering principles, check out this study on data structure principles.
Uses and Applications of Stacks in Data Structures
Stacks are versatile and find applications across various domains in computer science:
1. Function Call Management
Stacks manage function calls in programming languages, tracking the order of function execution. Each function call adds a frame to the stack, and when the function completes, its frame is removed.
2. Expression Evaluation
Stacks are used to evaluate arithmetic expressions, especially those with operators and parentheses. Algorithms like the Shunting Yard algorithm rely on stacks to convert and evaluate expressions.
3. Backtracking Algorithms
In search and pathfinding algorithms, stacks help remember the current path or state. If a dead end is reached, the algorithm backtracks by removing elements from the stack.
4. Undo Mechanisms
Text editors and software applications use stacks to implement undo features (e.g., Ctrl+Z). Each action is added to a stack, and undoing removes the most recent action.
5. Web Browser History
As mentioned earlier, stacks manage page navigation in browsers, allowing users to go back to previous pages in the order they were visited.
6. Syntax Checking in Compilers
Compilers use stacks to check for balanced symbols (e.g., {}, (), []) in code, ensuring proper nesting and syntax.
7. Memory Management
Stacks help manage memory allocation and deallocation, ensuring that the most recently allocated memory block is freed first.
Looking to sharpen your skills for top tech interviews? Our prep guide for Amazon DSA interview questions dives into memory optimization and more.
Stack Operations With Examples
Stacks support several key operations that are essential for managing data:
1. Push
Adds an element to the top of the stack.

Example in Python:
stack = []
stack.append(10) # Push 10 onto the stack
stack.append(20) # Push 20 onto the stack
print(stack) # Output: [10, 20]
2. Pop
Removes and returns the top element from the stack.
Example in Python:
stack = [10, 20]
top_element = stack.pop() # Pop the top element (20)
print(top_element) # Output: 20
print(stack) # Output: [10]

3. Peek (or Top)
Retrieves the top element without removing it.
Example in Python:
stack = [10, 20]
top_element = stack[-1] # Get the top element (20)
print(top_element) # Output: 20

4. IsEmpty
Checks if the stack is empty.
Example in Python:
stack = []
is_empty = len(stack) == 0 # True if stack is empty
print(is_empty) # Output: True

5. IsFull
Checks if the stack has reached its maximum capacity (relevant for fixed-size stacks).
Example in Python:
def is_full(stack, capacity):
return len(stack) == capacity
stack = [10, 20]
print(is_full(stack, 2)) # Output: True

For a comprehensive learning path, explore our essential DSA and web dev courses.
Stack Implementation in Programming Languages
Stacks can be implemented in various programming languages using arrays or linked lists. Below are examples of stack implementations in Python, C, C++, and Java.
Stack Implementation in Python
Python’s list can be used to implement a stack efficiently.
def create_stack():
return []
def check_empty(stack):
return len(stack) == 0
def push(stack, item):
stack.append(item)
print(f"Pushed item: {item}")
def pop(stack):
if check_empty(stack):
return "Stack is empty"
return stack.pop()
stack = create_stack()
push(stack, "1")
push(stack, "2")
print(f"Popped item: {pop(stack)}")
print(f"Stack after popping: {stack}")
Stack Implementation in C
In C, stacks are typically implemented using arrays with a fixed size.
#include
#define MAX 10
typedef struct {
int items[MAX];
int top;
} Stack;
void initStack(Stack *s) {
s->top = -1;
}
int isEmpty(Stack *s) {
return s->top == -1;
}
int isFull(Stack *s) {
return s->top == MAX - 1;
}
void push(Stack *s, int item) {
if (isFull(s)) {
printf("Stack is full\n");
} else {
s->items[++s->top] = item;
printf("Pushed item: %d\n", item);
}
}
int pop(Stack *s) {
if (isEmpty(s)) {
printf("Stack is empty\n");
return -1;
} else {
return s->items[s->top--];
}
}
int main() {
Stack s;
initStack(&s);
push(&s, 10);
push(&s, 20);
printf("Popped item: %d\n", pop(&s));
return 0;
}
Stack Implementation in C++
C++ allows for a more object-oriented approach to stack implementation.
#include
#define MAX 10
class Stack {
private:
int top;
int items[MAX];
public:
Stack() : top(-1) {}
bool isEmpty() { return top == -1; }
bool isFull() { return top == MAX - 1; }
void push(int item) {
if (isFull()) {
std::cout << "Stack is full\n";
} else {
items[++top] = item;
std::cout << "Pushed item: " << item << std::endl;
}
}
int pop() {
if (isEmpty()) {
std::cout << "Stack is empty\n";
return -1;
} else {
return items[top--];
}
}
int peek() {
if (!isEmpty()) {
return items[top];
} else {
std::cout << "Stack is empty\n";
return -1;
}
}
};
int main() {
Stack s;
s.push(10);
s.push(20);
std::cout << "Popped item: " << s.pop() << std::endl;
std::cout << "Top item now: " << s.peek() << std::endl;
return 0;
}
Stack Implementation in Java
Java’s class-based structure makes stack implementation straightforward.
public class Stack {
private int[] items;
private int top;
private int capacity;
public Stack(int size) {
items = new int[size];
capacity = size;
top = -1;
}
public void push(int item) {
if (isFull()) {
throw new RuntimeException("Stack is full");
}
items[++top] = item;
System.out.println("Pushed item: " + item);
}
public int pop() {
if (isEmpty()) {
throw new RuntimeException("Stack is empty");
}
return items[top--];
}
public int peek() {
if (isEmpty()) {
throw new RuntimeException("Stack is empty");
}
return items[top];
}
public boolean isEmpty() {
return top == -1;
}
public boolean isFull() {
return top == capacity - 1;
}
public static void main(String[] args) {
Stack stack = new Stack(3);
stack.push(10);
stack.push(20);
System.out.println("Popped item: " + stack.pop());
System.out.println("Top item now: " + stack.peek());
}
}
Advantages and Disadvantages of Stacks
Like any data structure, stacks come with their own set of strengths and limitations.
Advantages
- Simplicity: Stacks are easy to implement using arrays or linked lists.
- Efficiency: Push, pop, and peek operations are fast, typically O(1) time complexity.
- Memory Management: Stacks require minimal overhead, needing only a pointer to the top.
- Recursion Support: Essential for managing recursive function calls.
- Order Maintenance: Ideal for applications where the most recent element needs to be processed first.
Disadvantages
- Limited Access: Only the top element is accessible, making it unsuitable for applications requiring access to other elements.
- Fixed Size (for arrays): Array-based stacks have a fixed capacity, which can lead to overflow.
- Memory Underutilization: If the stack isn’t fully used, memory can be wasted.
- Potential for Overflow/Underflow: Mismanaging push and pop operations can cause errors.
- Not Scalable: Resizing dynamic stacks (e.g., using linked lists) can be costly.
For more on data structure trade-offs, see this research paper on data structure efficiency.
How is a stack implemented in programming?
Stacks can be implemented using arrays or linked lists. Array-based stacks have a fixed size, while linked list-based stacks can grow dynamically. For hands-on practice, our DSA course provides step-by-step coding exercises.
What is stack overflow and stack underflow?
- Stack Overflow: Occurs when trying to push an element onto a full stack.
- Stack Underflow: Occurs when trying to pop an element from an empty stack.
Master error handling techniques with our web development course.
Can a stack be implemented using queues?
Yes, but it requires two queues to simulate the LIFO behavior of a stack. This advanced technique is explored in our master DSA and system design course.
What is the difference between a stack and a queue?
- Stack: Follows LIFO (Last In, First Out).
- Queue: Follows FIFO (First In, First Out).
Dive into this comparison and more in our master DSA and web dev course.
Are there any limitations of using a stack?
Yes, stacks only allow access to the top element, which can be restrictive for certain applications. They also risk overflow and underflow if not managed properly. Learn to navigate these trade-offs in our data science course.
Preparing for a big interview? Check out our curated list of top 20 DSA interview questions or explore company-specific guides like Netflix, Meta, Amazon, or Atlassian to get a competitive edge.
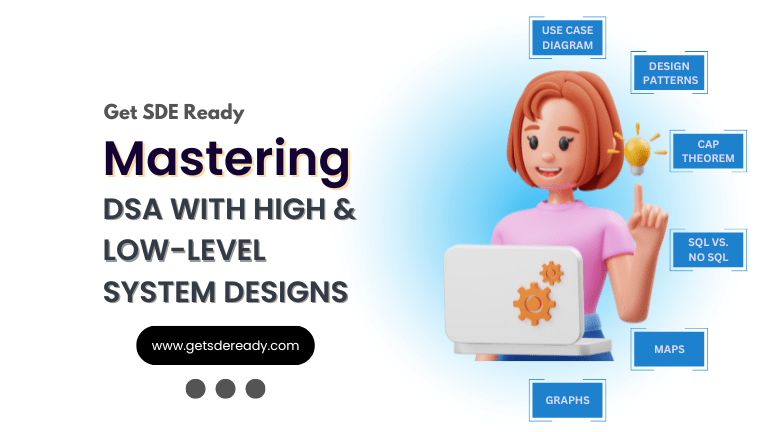
DSA, High & Low Level System Designs
- 85+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 400+ DSA Practice Questions
- Comprehensive Notes
- HackerRank Tests & Quizzes
- Topic-wise Quizzes
- Case Studies
- Access to Global Peer Community
Buy for 60% OFF
₹25,000.00 ₹9,999.00
Accelerate your Path to a Product based Career
Boost your career or get hired at top product-based companies by joining our expertly crafted courses. Gain practical skills and real-world knowledge to help you succeed.
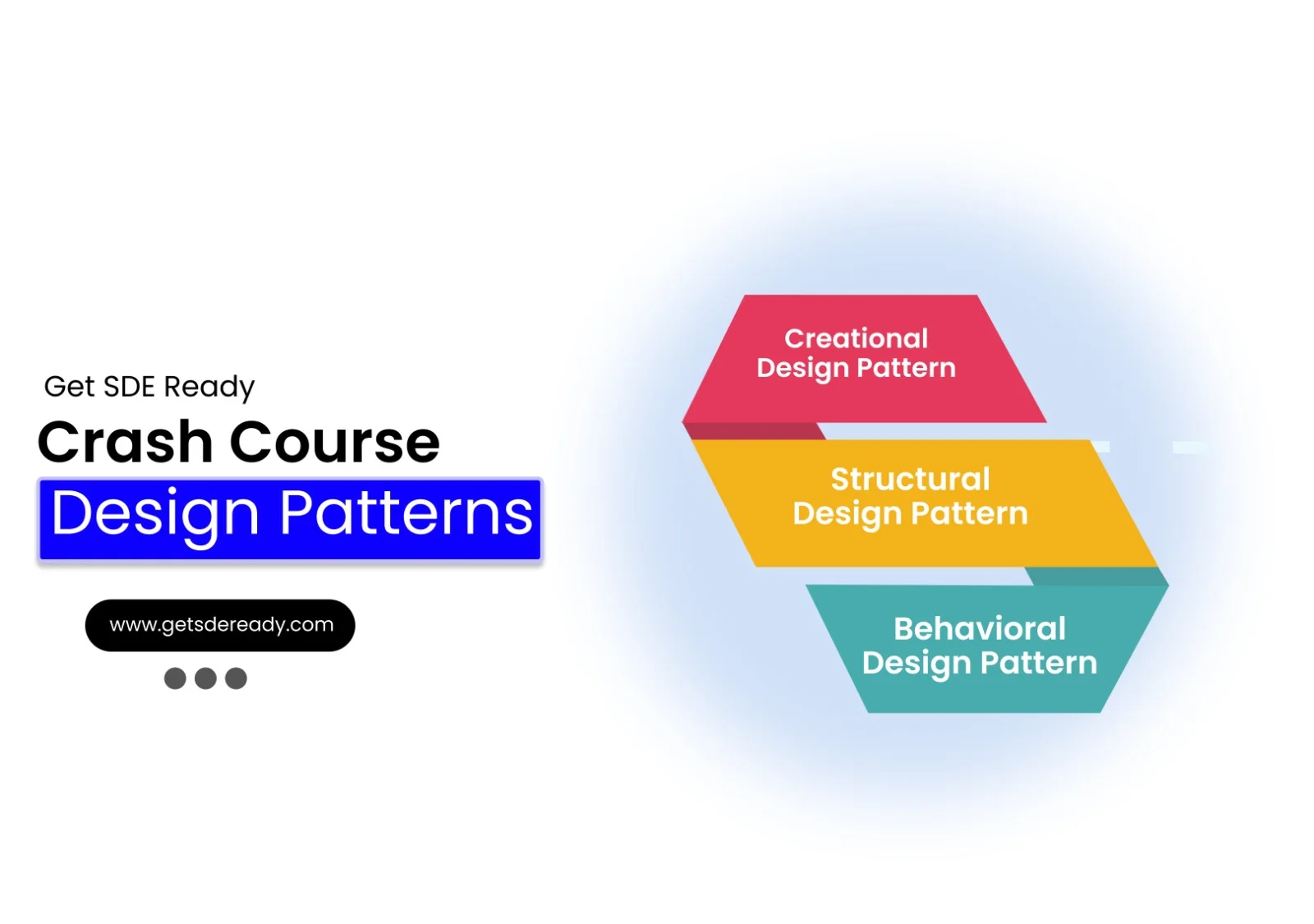
Design Patterns Bootcamp
- Live Classes & Recordings
- 24/7 Live Doubt Support
- Practice Questions
- Case Studies
- Access to Global Peer Community
- Topic wise Quizzes
- Referrals
- Certificate of Completion
Buy for 50% OFF
₹2,000.00 ₹999.00

ML & AI Kickstart
- Live Classes & Recordings
- 24/7 Live Doubt Support
- 2 Live Projects
- Case Studies
- Topic wise Quizzes
- Access to Global Peer Community
- Certificate of Completion
- Referrals
Buy for 50% OFF
₹2,000.00 ₹999.00
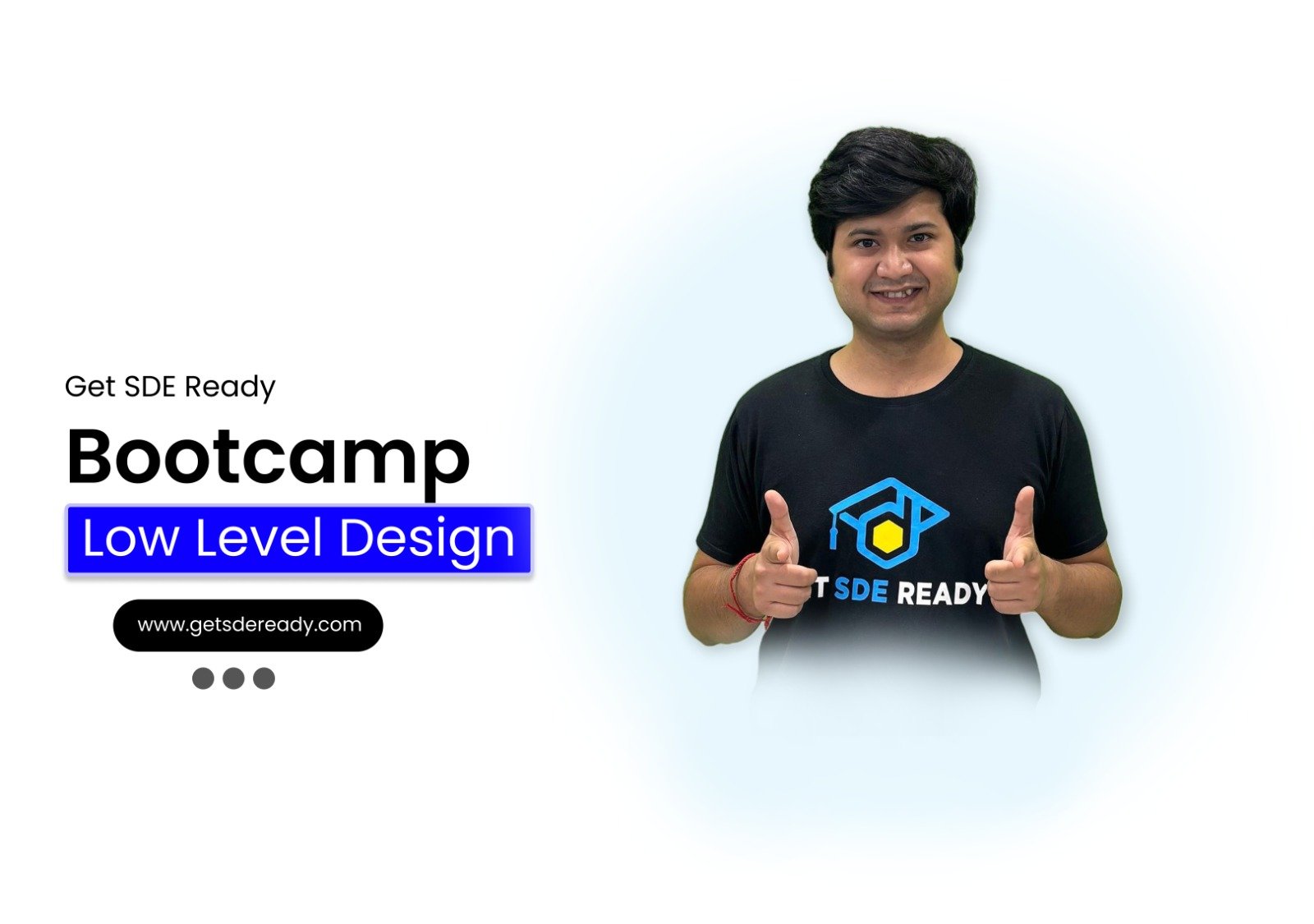
LLD Bootcamp
- 7+ Live Classes & Recordings
- Practice Questions
- 24/7 Live Doubt Support
- Case Studies
- Topic wise Quizzes
- Access to Global Peer Community
- Certificate of Completion
- Referrals
Buy for 50% OFF
₹2,000.00 ₹999.00
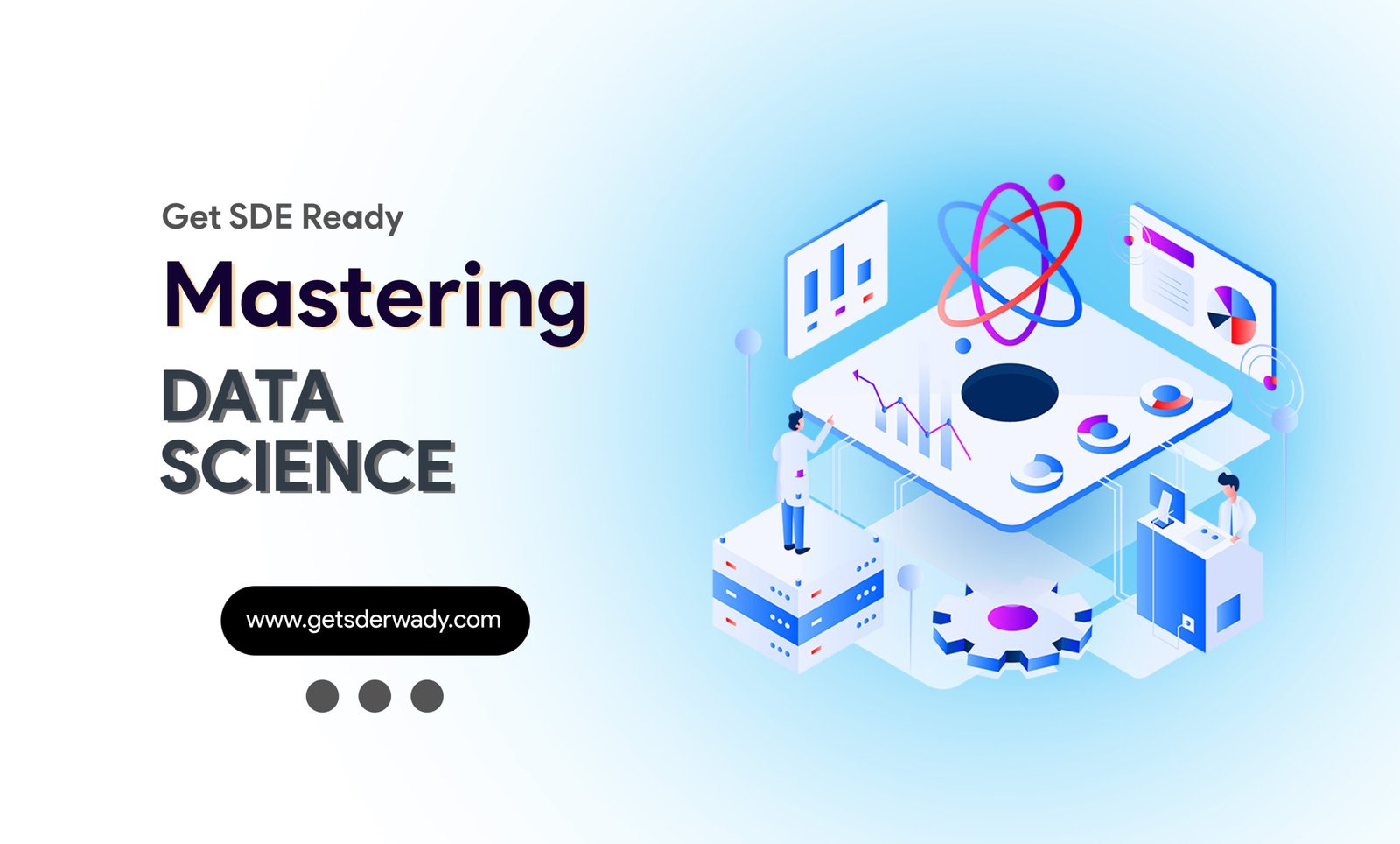
Essentials of Machine Learning and Artificial Intelligence
- 65+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 22+ Hands-on Live Projects & Deployments
- Comprehensive Notes
- Topic-wise Quizzes
- Case Studies
- Access to Global Peer Community
- Interview Prep Material
Buy for 65% OFF
₹20,000.00 ₹6,999.00
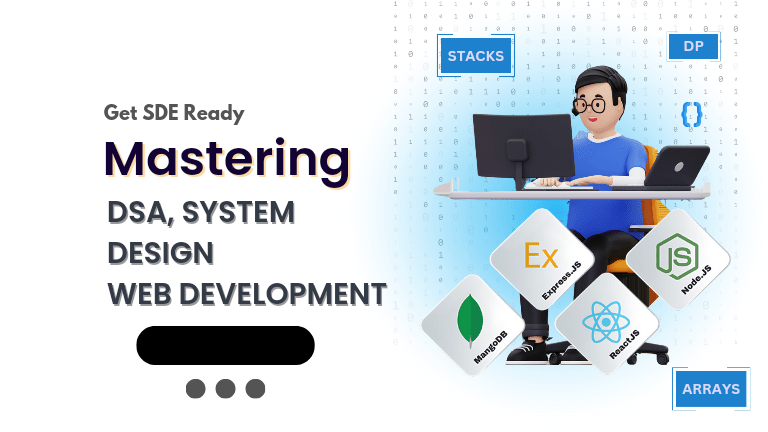
Fast-Track to Full Spectrum Software Engineering
- 120+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 400+ DSA Practice Questions
- Comprehensive Notes
- HackerRank Tests & Quizzes
- 12+ live Projects & Deployments
- Case Studies
- Access to Global Peer Community
Buy for 57% OFF
₹35,000.00 ₹14,999.00
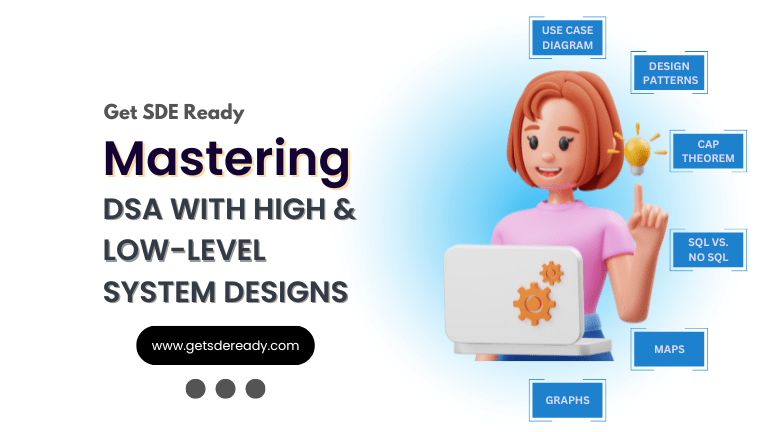
DSA, High & Low Level System Designs
- 85+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 400+ DSA Practice Questions
- Comprehensive Notes
- HackerRank Tests & Quizzes
- Topic-wise Quizzes
- Case Studies
- Access to Global Peer Community
Buy for 60% OFF
₹25,000.00 ₹9,999.00
Reach Out Now
If you have any queries, please fill out this form. We will surely reach out to you.
Contact Email
Reach us at the following email address.
Phone Number
You can reach us by phone as well.
+91-97737 28034
Our Location
Rohini, Sector-3, Delhi-110085