Data Structures and Algorithms
- Introduction to Data Structures and Algorithms
- Time and Space Complexity Analysis
- Big-O, Big-Theta, and Big-Omega Notations
- Recursion and Backtracking
- Divide and Conquer Algorithm
- Dynamic Programming: Memoization vs. Tabulation
- Greedy Algorithms and Their Use Cases
- Understanding Arrays: Types and Operations
- Linear Search vs. Binary Search
- Sorting Algorithms: Bubble, Insertion, Selection, and Merge Sort
- QuickSort: Explanation and Implementation
- Heap Sort and Its Applications
- Counting Sort, Radix Sort, and Bucket Sort
- Hashing Techniques: Hash Tables and Collisions
- Open Addressing vs. Separate Chaining in Hashing
- DSA Questions for Beginners
- Advanced DSA Questions for Competitive Programming
- Top 10 DSA Questions to Crack Your Next Coding Test
- Top 50 DSA Questions Every Programmer Should Practice
- Top Atlassian DSA Interview Questions
- Top Amazon DSA Interview Questions
- Top Microsoft DSA Interview Questions
- Top Meta (Facebook) DSA Interview Questions
- Netflix DSA Interview Questions and Preparation Guide
- Top 20 DSA Interview Questions You Need to Know
- Top Uber DSA Interview Questions and Solutions
- Google DSA Interview Questions and How to Prepare
- Airbnb DSA Interview Questions and How to Solve Them
- Mobile App DSA Interview Questions and Solutions
DSA Interview Questions
- DSA Questions for Beginners
- Advanced DSA Questions for Competitive Programming
- Top 10 DSA Questions to Crack Your Next Coding Test
- Top 50 DSA Questions Every Programmer Should Practice
- Top Atlassian DSA Interview Questions
- Top Amazon DSA Interview Questions
- Top Microsoft DSA Interview Questions
- Top Meta (Facebook) DSA Interview Questions
- Netflix DSA Interview Questions and Preparation Guide
- Top 20 DSA Interview Questions You Need to Know
- Top Uber DSA Interview Questions and Solutions
- Google DSA Interview Questions and How to Prepare
- Airbnb DSA Interview Questions and How to Solve Them
- Mobile App DSA Interview Questions and Solutions
Introduction to High-Level System Design
System Design Fundamentals
- Functional vs. Non-Functional Requirements
- Scalability, Availability, and Reliability
- Latency and Throughput Considerations
- Load Balancing Strategies
Architectural Patterns
- Monolithic vs. Microservices Architecture
- Layered Architecture
- Event-Driven Architecture
- Serverless Architecture
- Model-View-Controller (MVC) Pattern
- CQRS (Command Query Responsibility Segregation)
Scaling Strategies
- Vertical Scaling vs. Horizontal Scaling
- Sharding and Partitioning
- Data Replication and Consistency Models
- Load Balancing Strategies
- CDN and Edge Computing
Database Design in HLD
- SQL vs. NoSQL Databases
- CAP Theorem and its Impact on System Design
- Database Indexing and Query Optimization
- Database Sharding and Partitioning
- Replication Strategies
API Design and Communication
Caching Strategies
- Types of Caching
- Cache Invalidation Strategies
- Redis vs. Memcached
- Cache-Aside, Write-Through, and Write-Behind Strategies
Message Queues and Event-Driven Systems
- Kafka vs. RabbitMQ vs. SQS
- Pub-Sub vs. Point-to-Point Messaging
- Handling Asynchronous Workloads
- Eventual Consistency in Distributed Systems
Security in System Design
Observability and Monitoring
- Logging Strategies (ELK Stack, Prometheus, Grafana)
- API Security Best Practices
- Secure Data Storage and Access Control
- DDoS Protection and Rate Limiting
Real-World System Design Case Studies
- Distributed locking (Locking and its Types)
- Memory leaks and Out of memory issues
- HLD of YouTube
- HLD of WhatsApp
System Design Interview Questions
- Adobe System Design Interview Questions
- Top Atlassian System Design Interview Questions
- Top Amazon System Design Interview Questions
- Top Microsoft System Design Interview Questions
- Top Meta (Facebook) System Design Interview Questions
- Top Netflix System Design Interview Questions
- Top Uber System Design Interview Questions
- Top Google System Design Interview Questions
- Top Apple System Design Interview Questions
- Top Airbnb System Design Interview Questions
- Top 10 System Design Interview Questions
- Mobile App System Design Interview Questions
- Top 20 Stripe System Design Interview Questions
- Top Shopify System Design Interview Questions
- Top 20 System Design Interview Questions
- Top Advanced System Design Questions
- Most-Frequented System Design Questions in Big Tech Interviews
- What Interviewers Look for in System Design Questions
- Critical System Design Questions to Crack Any Tech Interview
- Top 20 API Design Questions for System Design Interviews
- Top 10 Steps to Create a System Design Portfolio for Developers
Mastering XOR: Tricks and Applications in Programming
Discover the power of bitwise operations with XOR in this comprehensive guide. Learn how XOR enhances programming efficiency, supports cryptography, and enables robust error detection through innovative techniques and optimized algorithms.
Understanding XOR: Basics, Properties, and Benefits
What is XOR and How Does It Work?
XOR (Exclusive OR) is a fundamental bitwise operation that compares two bits. It returns 1 if the bits differ and 0 if they are the same. In most programming languages, XOR is represented by the ^ symbol. This operation is crucial in cryptography, data structures, and error detection techniques.
Key XOR Properties
The table below summarizes the core properties of XOR:
Property | Formula | Example |
Identity | A XOR 0 = A | 5 XOR 0 = 5 |
Self-Inverse | A XOR A = 0 | 5 XOR 5 = 0 |
Commutative | A XOR B = B XOR A | 5 XOR 3 = 3 XOR 5 |
Associative | (A XOR B) XOR C = A XOR (B XOR C) | (5 XOR 3) XOR 2 = 5 XOR (3 XOR 2) |
Involution | If X = A XOR B then: X XOR B = A X XOR A = B | For a = 7 and b = 4: 7 XOR 4 = 3 3 XOR 4 = 7 3 XOR 7 = 4 |
Visualizing XOR in Binary
Understanding XOR at the bit level is essential. Consider these examples:
Example 1: 5 XOR 3
Number | Binary Representation |
5 | 0101 |
3 | 0011 |
Result | 0110 (6) |
Example 2: 8 XOR 2
Number | Binary Representation |
8 | 1000 |
2 | 0010 |
Result | 1010 (10) |
Advanced XOR Programming Tricks and Practical Applications
Swapping Two Numbers Without a Temporary Variable
Utilize XOR to swap values without extra space:
void swap(int a, int b) {
    a = a ^ b;
    b = a ^ b;
    a = a ^ b;
    System.out.println(“Swapped values: a = ” + a + “, b = ” + b);
}
Step-by-step execution:
Step | Operation | a (Binary) | b (Binary) |
Initial | a = 5, b = 3 | 0101 | 0011 |
Step 1 | a = a ^ b | 0110 (6) | 0011 |
Step 2 | b = a ^ b | 0110 | 0101 (5) |
Step 3 | a = a ^ b | 0011 (3) | 0101 |
This method works because XOR-ing twice with the same value cancels out the effect, efficiently swapping the values.
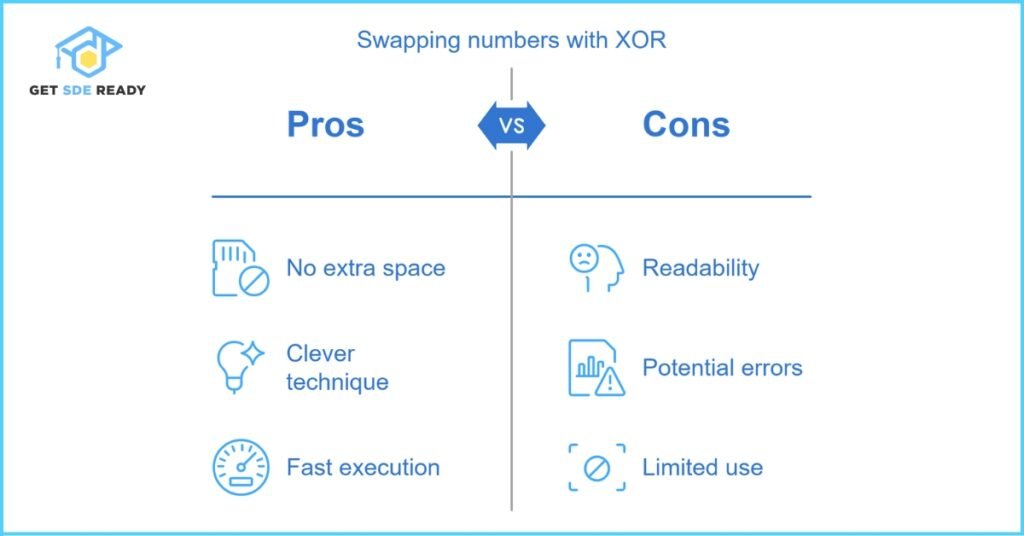
Finding the Unique Element in an Array
Identify the single unique element in an array where all other elements appear twice:
int findUnique(int[] arr) {
int unique = 0;
for (int num : arr) {
unique ^= num;
}
return unique;
}
Example with Array [2, 3, 2, 4, 4]:
Operation | Calculation | Result |
Start | unique = 0 | 0 |
0 XOR 2 | 0 ^ 2 | 2 |
2 XOR 3 | 2 ^ 3 | 1 |
1 XOR 2 | 1 ^ 2 | 3 |
3 XOR 4 | 3 ^ 4 | 7 |
7 XOR 4 | 7 ^ 4 | 3 |
The duplicate elements cancel out, leaving the unique element (3).
Identifying a Missing Number in a Range
Find the missing number when the array contains numbers from 0 to n with one number missing:
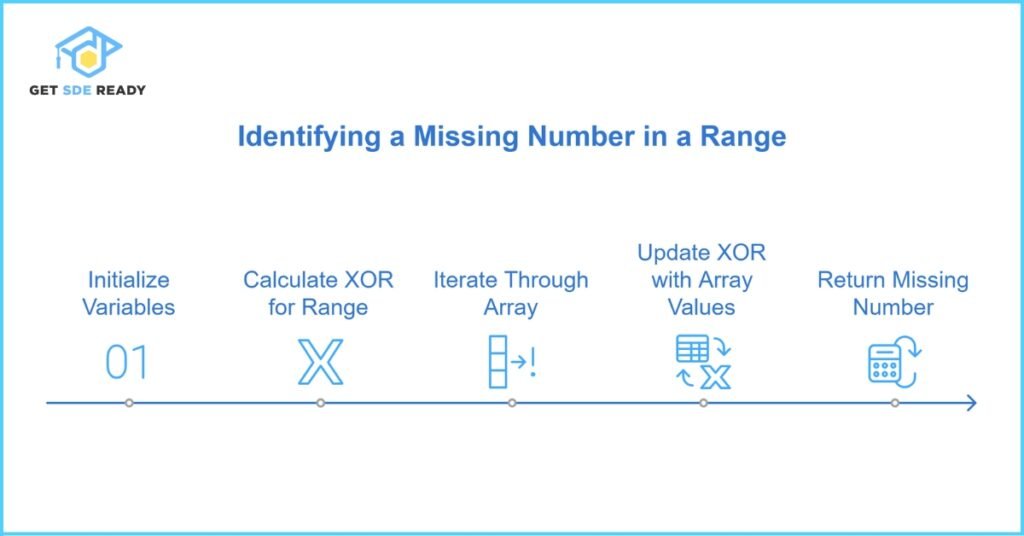
int findMissing(int[] nums) {
int n = nums.length;
int xor = 0;
for (int i = 0; i <= n; i++) {
xor ^= i;
}
for (int num : nums) {
xor ^= num;
}
return xor;
}
Example:
For the array [1, 2, 3, 5] where 4 is missing:
Step | Operation | Intermediate Result |
Compute XOR of full range [1,2,3,4,5] | 1 XOR 2, then XOR 3, 4, 5 | 1 |
Compute XOR of array [1,2,3,5] | 1 XOR 2, then XOR 3, 5 | 5 |
Final step | 1 XOR 5 | 4 (Missing Number) |
XOR operations reveal that the missing number is 4.
Checking for Opposite Signs in Two Numbers
Determine if two numbers have opposite signs without using conditional statements:
boolean haveOppositeSigns(int x, int y) {
return (x ^ y) < 0;
}
A negative result from the XOR operation indicates that the numbers have opposite signs.
Simple Encryption and Decryption Using XOR
Leverage XOR for basic encryption and decryption processes:
String xorEncryptDecrypt(String input, char key) {
StringBuilder output = new StringBuilder();
for (char ch : input.toCharArray()) {
output.append((char)(ch ^ key));
}
return output.toString();
}
- Encrypt a plaintext like “HELLO” by XOR-ing each character with a key.
- Reapplying the XOR operation with the same key decrypts the message back to its original form.
Practical Applications of XOR in Programming
XOR’s versatility is evident in many programming scenarios:
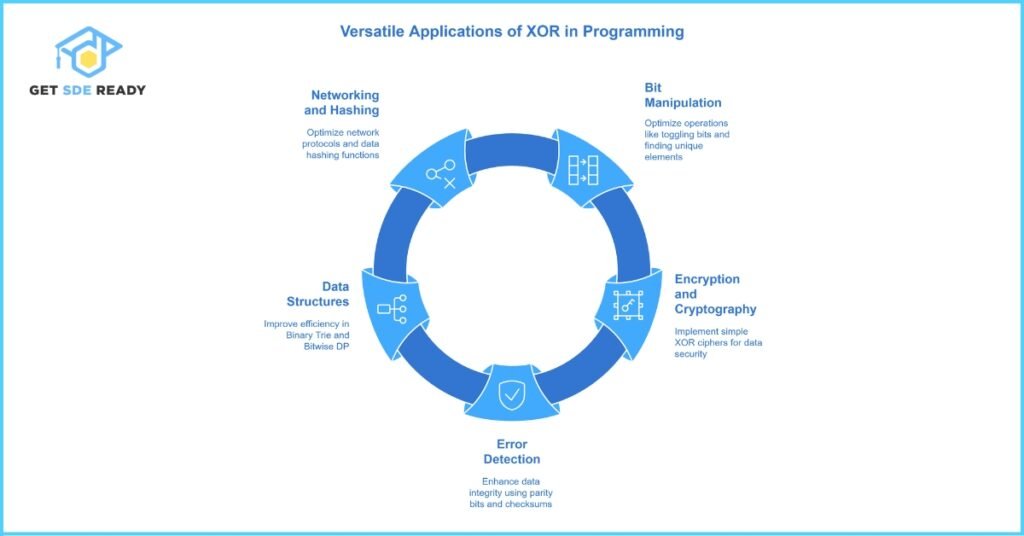
- Bit Manipulation: Optimize low-level operations such as toggling bits and finding unique elements.
- Encryption and Cryptography: Implement simple yet effective XOR ciphers.
- Error Detection: Enhance data integrity using parity bits and checksum algorithms.
- Data Structures: Improve efficiency in structures like Binary Trie and Bitwise Dynamic Programming.
- Networking and Hashing: Optimize network protocols and data hashing functions.
Conclusion
Mastering XOR operations provides a significant edge in programming, algorithm optimization, and cryptography. By integrating these techniques into your projects, you can solve complex problems more efficiently and elegantly. Explore these XOR tricks and applications to elevate your coding challenges and improve your software performance.
What Should I Focus on for Airbnb DSA Interviews?
When preparing for Airbnb DSA interviews, focus on mastering the fundamentals of data structures and algorithms. This includes arrays, linked lists, trees, graphs, dynamic programming, and sorting techniques. A well-structured study plan that includes hands-on practice with real-world problems will boost your confidence and performance. For a detailed course on DSA fundamentals, check out this course.
How Important Is Code Optimization in DSA Interviews?
Code optimization is crucial during DSA interviews because it reflects your ability to write efficient and scalable solutions. Interviewers are not only interested in getting the correct answer but also in understanding your thought process and how you handle large inputs. Ensuring your solution runs within optimal time and space limits is essential for success. To learn more about optimizing your coding techniques, explore this web development course.
Can Practicing on Paper Improve My Interview Performance?
Absolutely, practicing on paper or a whiteboard can significantly improve your interview performance. It helps simulate the real interview environment where you need to articulate your thought process without the aid of an IDE. Regular practice in this format enables you to organize your ideas better and communicate more effectively under pressure. For more insights on preparing for technical interviews, consider checking out this combined DSA and design course.
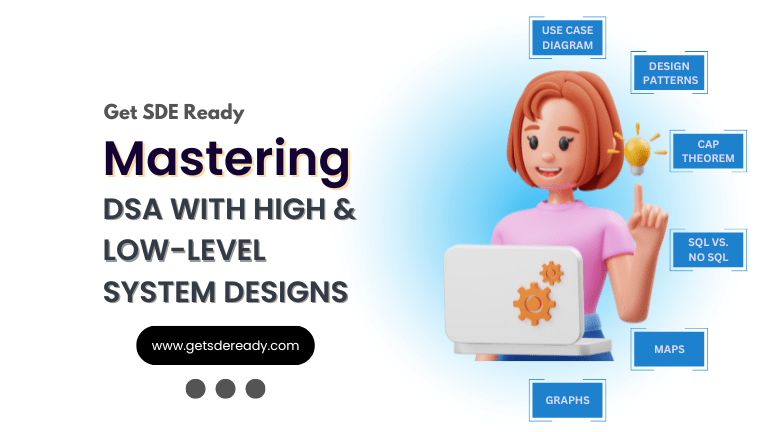
DSA, High & Low Level System Designs
- 85+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 400+ DSA Practice Questions
- Comprehensive Notes
- HackerRank Tests & Quizzes
- Topic-wise Quizzes
- Case Studies
- Access to Global Peer Community
Buy for 60% OFF
₹25,000.00 ₹9,999.00
Accelerate your Path to a Product based Career
Boost your career or get hired at top product-based companies by joining our expertly crafted courses. Gain practical skills and real-world knowledge to help you succeed.
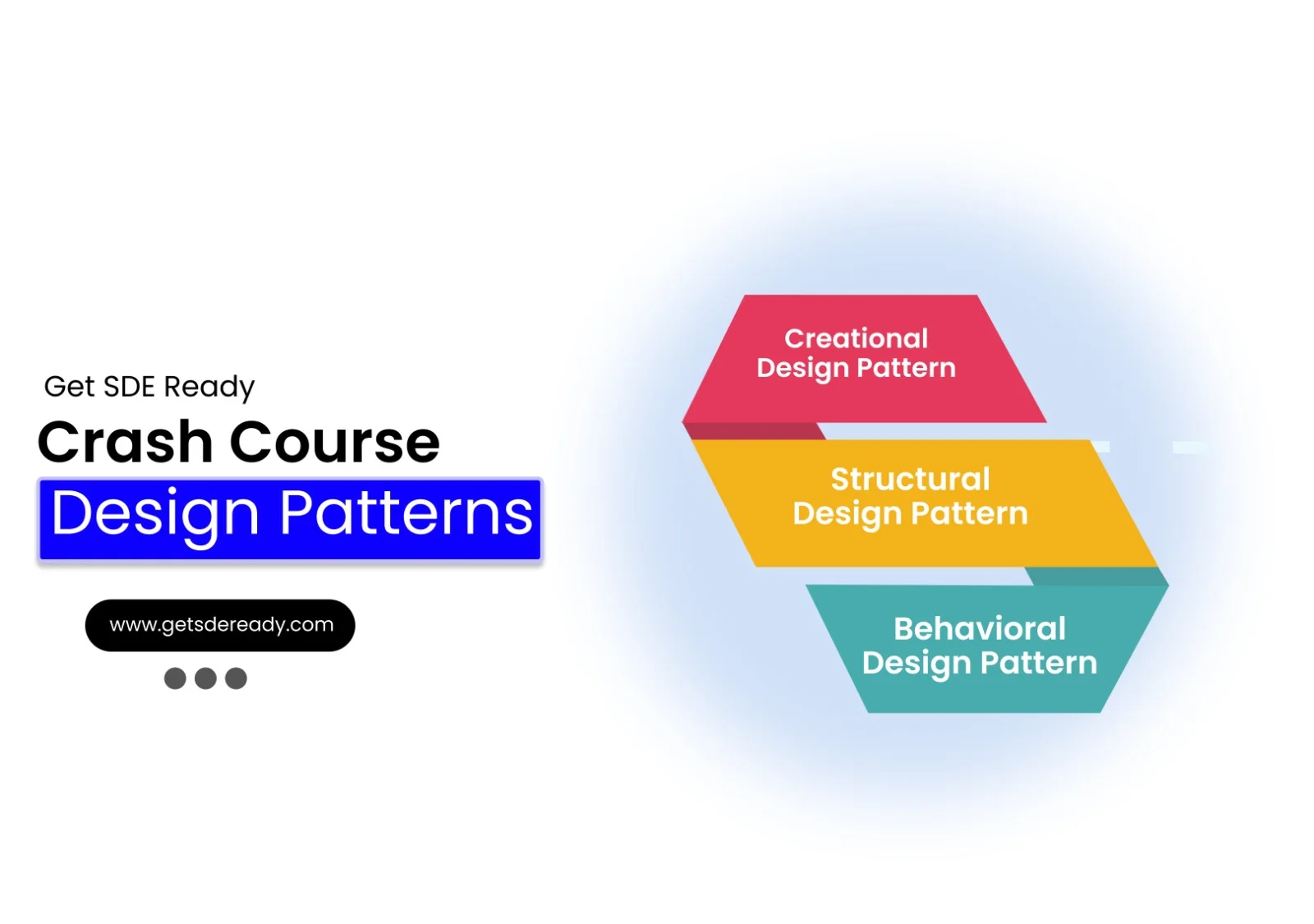
Design Patterns Bootcamp
- Live Classes & Recordings
- 24/7 Live Doubt Support
- Practice Questions
- Case Studies
- Access to Global Peer Community
- Topic wise Quizzes
- Referrals
- Certificate of Completion
Buy for 50% OFF
₹2,000.00 ₹999.00

ML & AI Kickstart
- Live Classes & Recordings
- 24/7 Live Doubt Support
- 2 Live Projects
- Case Studies
- Topic wise Quizzes
- Access to Global Peer Community
- Certificate of Completion
- Referrals
Buy for 50% OFF
₹2,000.00 ₹999.00
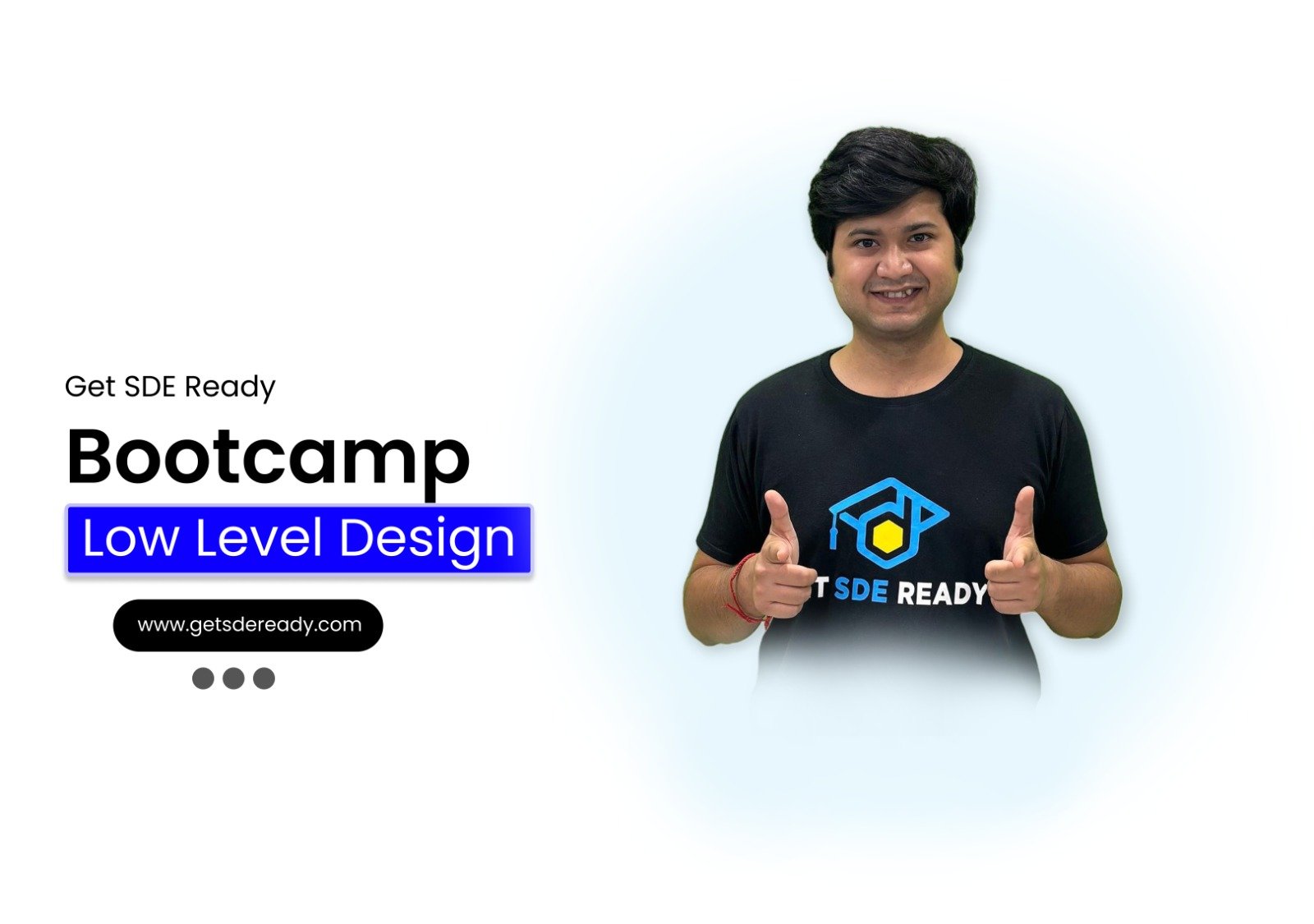
LLD Bootcamp
- 7+ Live Classes & Recordings
- Practice Questions
- 24/7 Live Doubt Support
- Case Studies
- Topic wise Quizzes
- Access to Global Peer Community
- Certificate of Completion
- Referrals
Buy for 50% OFF
₹2,000.00 ₹999.00
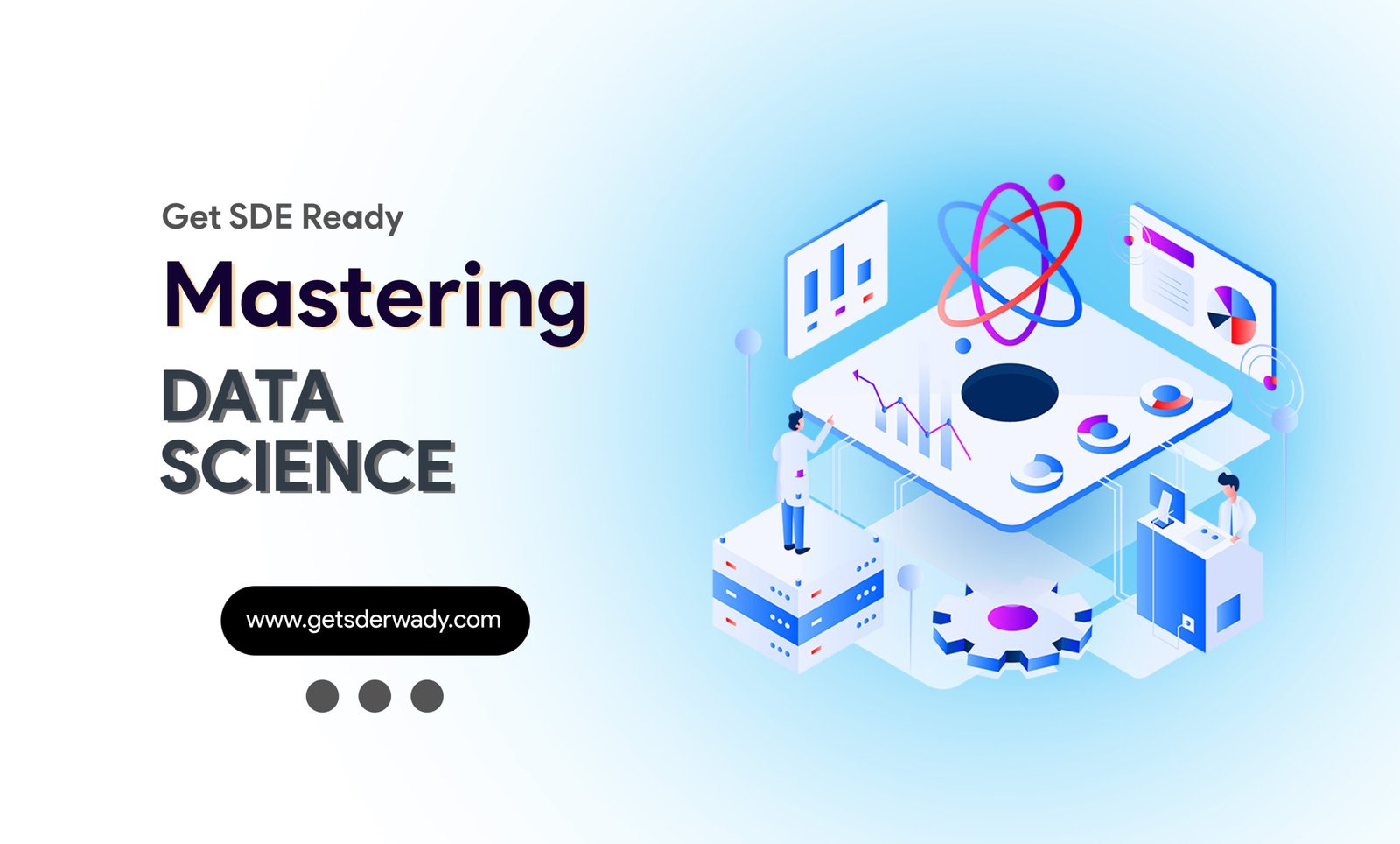
Essentials of Machine Learning and Artificial Intelligence
- 65+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 22+ Hands-on Live Projects & Deployments
- Comprehensive Notes
- Topic-wise Quizzes
- Case Studies
- Access to Global Peer Community
- Interview Prep Material
Buy for 65% OFF
₹20,000.00 ₹6,999.00
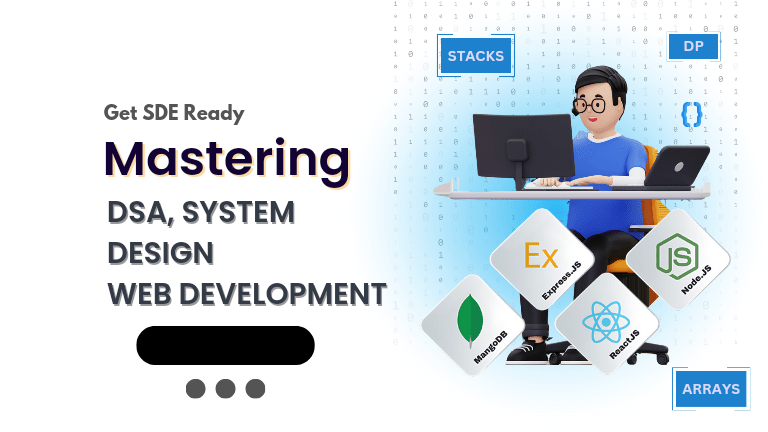
Fast-Track to Full Spectrum Software Engineering
- 120+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 400+ DSA Practice Questions
- Comprehensive Notes
- HackerRank Tests & Quizzes
- 12+ live Projects & Deployments
- Case Studies
- Access to Global Peer Community
Buy for 57% OFF
₹35,000.00 ₹14,999.00
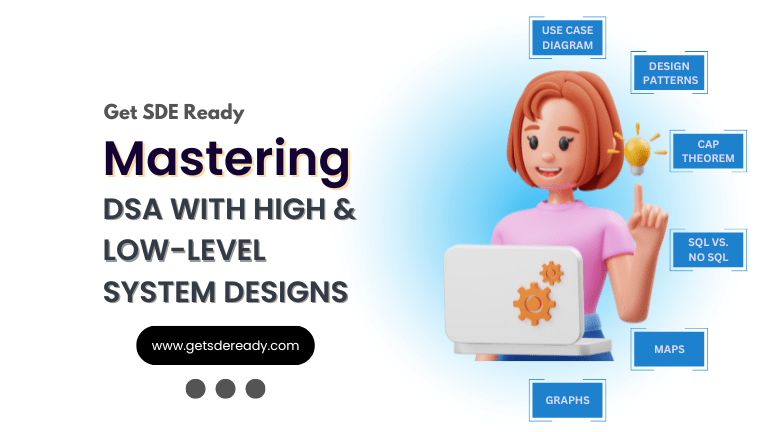
DSA, High & Low Level System Designs
- 85+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 400+ DSA Practice Questions
- Comprehensive Notes
- HackerRank Tests & Quizzes
- Topic-wise Quizzes
- Case Studies
- Access to Global Peer Community
Buy for 60% OFF
₹25,000.00 ₹9,999.00
Reach Out Now
If you have any queries, please fill out this form. We will surely reach out to you.
Contact Email
Reach us at the following email address.
Phone Number
You can reach us by phone as well.
+91-97737 28034
Our Location
Rohini, Sector-3, Delhi-110085