Data Structures and Algorithms
- Introduction to Data Structures and Algorithms
- Time and Space Complexity Analysis
- Big-O, Big-Theta, and Big-Omega Notations
- Recursion and Backtracking
- Divide and Conquer Algorithm
- Dynamic Programming: Memoization vs. Tabulation
- Greedy Algorithms and Their Use Cases
- Understanding Arrays: Types and Operations
- Linear Search vs. Binary Search
- Sorting Algorithms: Bubble, Insertion, Selection, and Merge Sort
- QuickSort: Explanation and Implementation
- Heap Sort and Its Applications
- Counting Sort, Radix Sort, and Bucket Sort
- Hashing Techniques: Hash Tables and Collisions
- Open Addressing vs. Separate Chaining in Hashing
- DSA Questions for Beginners
- Advanced DSA Questions for Competitive Programming
- Top 10 DSA Questions to Crack Your Next Coding Test
- Top 50 DSA Questions Every Programmer Should Practice
- Top Atlassian DSA Interview Questions
- Top Amazon DSA Interview Questions
- Top Microsoft DSA Interview Questions
- Top Meta (Facebook) DSA Interview Questions
- Netflix DSA Interview Questions and Preparation Guide
- Top 20 DSA Interview Questions You Need to Know
- Top Uber DSA Interview Questions and Solutions
- Google DSA Interview Questions and How to Prepare
- Airbnb DSA Interview Questions and How to Solve Them
- Mobile App DSA Interview Questions and Solutions
DSA Interview Questions
- DSA Questions for Beginners
- Advanced DSA Questions for Competitive Programming
- Top 10 DSA Questions to Crack Your Next Coding Test
- Top 50 DSA Questions Every Programmer Should Practice
- Top Atlassian DSA Interview Questions
- Top Amazon DSA Interview Questions
- Top Microsoft DSA Interview Questions
- Top Meta (Facebook) DSA Interview Questions
- Netflix DSA Interview Questions and Preparation Guide
- Top 20 DSA Interview Questions You Need to Know
- Top Uber DSA Interview Questions and Solutions
- Google DSA Interview Questions and How to Prepare
- Airbnb DSA Interview Questions and How to Solve Them
- Mobile App DSA Interview Questions and Solutions
Data Structures and Algorithms
- Introduction to Data Structures and Algorithms
- Time and Space Complexity Analysis
- Big-O, Big-Theta, and Big-Omega Notations
- Recursion and Backtracking
- Divide and Conquer Algorithm
- Dynamic Programming: Memoization vs. Tabulation
- Greedy Algorithms and Their Use Cases
- Understanding Arrays: Types and Operations
- Linear Search vs. Binary Search
- Sorting Algorithms: Bubble, Insertion, Selection, and Merge Sort
- QuickSort: Explanation and Implementation
- Heap Sort and Its Applications
- Counting Sort, Radix Sort, and Bucket Sort
- Hashing Techniques: Hash Tables and Collisions
- Open Addressing vs. Separate Chaining in Hashing
- DSA Questions for Beginners
- Advanced DSA Questions for Competitive Programming
- Top 10 DSA Questions to Crack Your Next Coding Test
- Top 50 DSA Questions Every Programmer Should Practice
- Top Atlassian DSA Interview Questions
- Top Amazon DSA Interview Questions
- Top Microsoft DSA Interview Questions
- Top Meta (Facebook) DSA Interview Questions
- Netflix DSA Interview Questions and Preparation Guide
- Top 20 DSA Interview Questions You Need to Know
- Top Uber DSA Interview Questions and Solutions
- Google DSA Interview Questions and How to Prepare
- Airbnb DSA Interview Questions and How to Solve Them
- Mobile App DSA Interview Questions and Solutions
DSA Interview Questions
- DSA Questions for Beginners
- Advanced DSA Questions for Competitive Programming
- Top 10 DSA Questions to Crack Your Next Coding Test
- Top 50 DSA Questions Every Programmer Should Practice
- Top Atlassian DSA Interview Questions
- Top Amazon DSA Interview Questions
- Top Microsoft DSA Interview Questions
- Top Meta (Facebook) DSA Interview Questions
- Netflix DSA Interview Questions and Preparation Guide
- Top 20 DSA Interview Questions You Need to Know
- Top Uber DSA Interview Questions and Solutions
- Google DSA Interview Questions and How to Prepare
- Airbnb DSA Interview Questions and How to Solve Them
- Mobile App DSA Interview Questions and Solutions
Priority Queues in JavaScript
Priority queues are a powerful data structure in JavaScript that allow you to manage elements based on their priority rather than their order of arrival. Unlike a standard queue, where elements follow the First-In-First-Out (FIFO) principle, a priority queue ensures that elements with higher priority are processed first. This guide will walk you through the essentials of priority queues, how to implement them in JavaScript, and their practical applications. Whether you’re preparing for coding interviews or optimizing your projects, mastering priority queues is a must-have skill. Want to level up your programming expertise? Sign up for exclusive tutorials and resources from our community!
What is a Priority Queue?
A priority queue is an abstract data type where each element is associated with a priority. Elements with higher priority are dequeued before those with lower priority, regardless of when they were added. This makes priority queues ideal for scenarios where certain tasks need precedence over others.
Key Characteristics
- Each element has a priority value.
- Elements are processed based on priority, not insertion order.
- Can be configured as a min-priority queue (lowest value = highest priority) or a max-priority queue (highest value = highest priority).
Priority queues shine in applications like task scheduling, bandwidth allocation, and graph algorithms such as Dijkstra’s shortest path. For a deeper dive into data structures, explore our Data Structures and Algorithms course.
Implementing a Priority Queue in JavaScript
There are multiple ways to implement a priority queue in JavaScript. Below, we’ll cover two common approaches: an array-based method and a binary heap-based method.
1. Array-Based Priority Queue
In this approach, elements are stored in an array along with their priorities. When adding an element, it’s inserted in the correct position based on priority.
Code Example
Priority queues are a powerful data structure in JavaScript that allow you to manage elements based on their priority rather than their order of arrival. Unlike a standard queue, where elements follow the First-In-First-Out (FIFO) principle, a priority queue ensures that elements with higher priority are processed first. This guide will walk you through the essentials of priority queues, how to implement them in JavaScript, and their practical applications. Whether you're preparing for coding interviews or optimizing your projects, mastering priority queues is a must-have skill. Want to level up your programming expertise? Sign up for exclusive tutorials and resources from our community!
What is a Priority Queue?
A priority queue is an abstract data type where each element is associated with a priority. Elements with higher priority are dequeued before those with lower priority, regardless of when they were added. This makes priority queues ideal for scenarios where certain tasks need precedence over others.
Key Characteristics
Each element has a priority value.
Elements are processed based on priority, not insertion order.
Can be configured as a min-priority queue (lowest value = highest priority) or a max-priority queue (highest value = highest priority).
Priority queues shine in applications like task scheduling, bandwidth allocation, and graph algorithms such as Dijkstra’s shortest path. For a deeper dive into data structures, explore our Data Structures and Algorithms course.
Implementing a Priority Queue in JavaScript
There are multiple ways to implement a priority queue in JavaScript. Below, we’ll cover two common approaches: an array-based method and a binary heap-based method.
1. Array-Based Priority Queue
In this approach, elements are stored in an array along with their priorities. When adding an element, it’s inserted in the correct position based on priority.
Code Example
Pros and Cons
- Pros: Easy to implement and understand.
- Cons: Inefficient for large datasets due to O(n) insertion time when finding the correct position.
This method works well for small datasets or when simplicity is key.
2. Binary Heap-Based Priority Queue (Min-Heap)
A binary heap offers a more efficient solution by maintaining elements in a tree-like structure where the highest-priority element is always at the root. Here, we’ll implement a min-heap where the smallest priority value has the highest priority.
Code Example
class MinHeapPriorityQueue {
constructor() {
this.heap = [];
}
add(element, priority) {
this.heap.push({ element, priority });
this.heapifyUp();
}
remove() {
if (this.isEmpty()) return null;
const item = this.heap[0];
this.heap[0] = this.heap.pop();
this.heapifyDown();
return item.element;
}
peek() {
if (this.isEmpty()) return null;
return this.heap[0].element;
}
isEmpty() {
return this.heap.length === 0;
}
heapifyUp() {
let index = this.heap.length - 1;
while (index > 0) {
const parentIndex = Math.floor((index - 1) / 2);
if (this.heap[parentIndex].priority <= this.heap[index].priority) break;
[this.heap[index], this.heap[parentIndex]] = [this.heap[parentIndex], this.heap[index]];
index = parentIndex;
}
}
heapifyDown() {
let index = 0;
while (true) {
const leftChild = 2 * index + 1;
const rightChild = 2 * index + 2;
let smallest = index;
if (leftChild < this.heap.length && this.heap[leftChild].priority < this.heap[smallest].priority) {
smallest = leftChild;
}
if (rightChild < this.heap.length && this.heap[rightChild].priority < this.heap[smallest].priority) {
smallest = rightChild;
}
if (smallest === index) break;
[this.heap[index], this.heap[smallest]] = [this.heap[smallest], this.heap[index]];
index = smallest;
}
}
}
// Usage
const heapPq = new MinHeapPriorityQueue();
heapPq.add("Task A", 3);
heapPq.add("Task B", 1);
heapPq.add("Task C", 2);
console.log(heapPq.peek()); // "Task B"
console.log(heapPq.remove()); // "Task B"
console.log(heapPq.peek()); // "Task C"
Pros and Cons
- Pros: Efficient O(log n) time for adding and removing elements.
- Cons: More complex to implement than the array-based method.
This approach is ideal for performance-critical applications or larger datasets. Learn more optimization techniques in our Crash Course.
Key Operations
Priority queues support the following operations:
- Enqueue (add): Adds an element with a priority.
- Dequeue (remove): Removes and returns the highest-priority element.
- Peek (front): Returns the highest-priority element without removing it.
- Is Empty: Checks if the queue is empty.
Time Complexity Comparison
Operation | Array-Based | Binary Heap |
Enqueue | O(n) | O(log n) |
Dequeue | O(1) | O(log n) |
Peek | O(1) | O(1) |
Is Empty | O(1) | O(1) |
The binary heap is more efficient for frequent insertions, making it a better choice for dynamic applications.
Practical Applications
Priority queues are used in:
- Task Scheduling: Prioritize urgent tasks.
- Graph Algorithms: Optimize paths in Dijkstra’s algorithm.
- Bandwidth Management: Allocate resources based on priority.
- Event Simulations: Process events in order of importance.
For real-world examples, check out our Data Science course.
Best Practices
- Choose Wisely: Use arrays for simplicity, heaps for performance.
- Clear Priorities: Define consistent priority rules.
- Handle Ties: Decide how to manage equal priorities (e.g., FIFO).
- Avoid Direct Changes: Re-insert elements instead of modifying priorities in place.
Conclusion
Priority queues in JavaScript offer a flexible way to handle prioritized data, with implementations ranging from simple arrays to efficient binary heaps. By understanding their mechanics and applications, you can tackle a wide range of programming challenges. Start experimenting with priority queues in your projects today!
FAQs
What’s the difference between a priority queue and a regular queue?
A priority queue processes elements by priority, while a regular queue uses FIFO. Learn more in our Data Structures and Algorithms course.
How do I create a max-priority queue?
Reverse the comparison logic in the binary heap to prioritize larger values. See examples in our Web Development course.
What are priority queues used for?
They’re essential for task scheduling, graph algorithms, and resource allocation. Explore use cases in our Design and DSA Combined course.
How can I ace data structure interviews?
Practice core concepts and problems like those in our Master DSA, Web Dev, and System Design course. Check top questions from Netflix or Amazon.
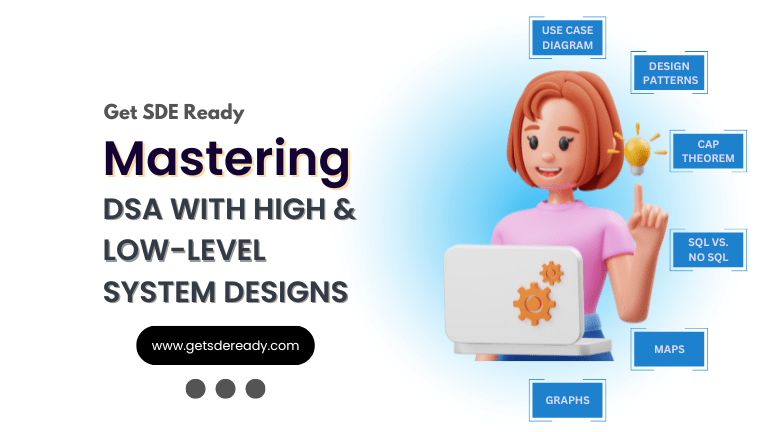
DSA, High & Low Level System Designs
- 85+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 400+ DSA Practice Questions
- Comprehensive Notes
- HackerRank Tests & Quizzes
- Topic-wise Quizzes
- Case Studies
- Access to Global Peer Community
Buy for 60% OFF
₹25,000.00 ₹9,999.00
Accelerate your Path to a Product based Career
Boost your career or get hired at top product-based companies by joining our expertly crafted courses. Gain practical skills and real-world knowledge to help you succeed.
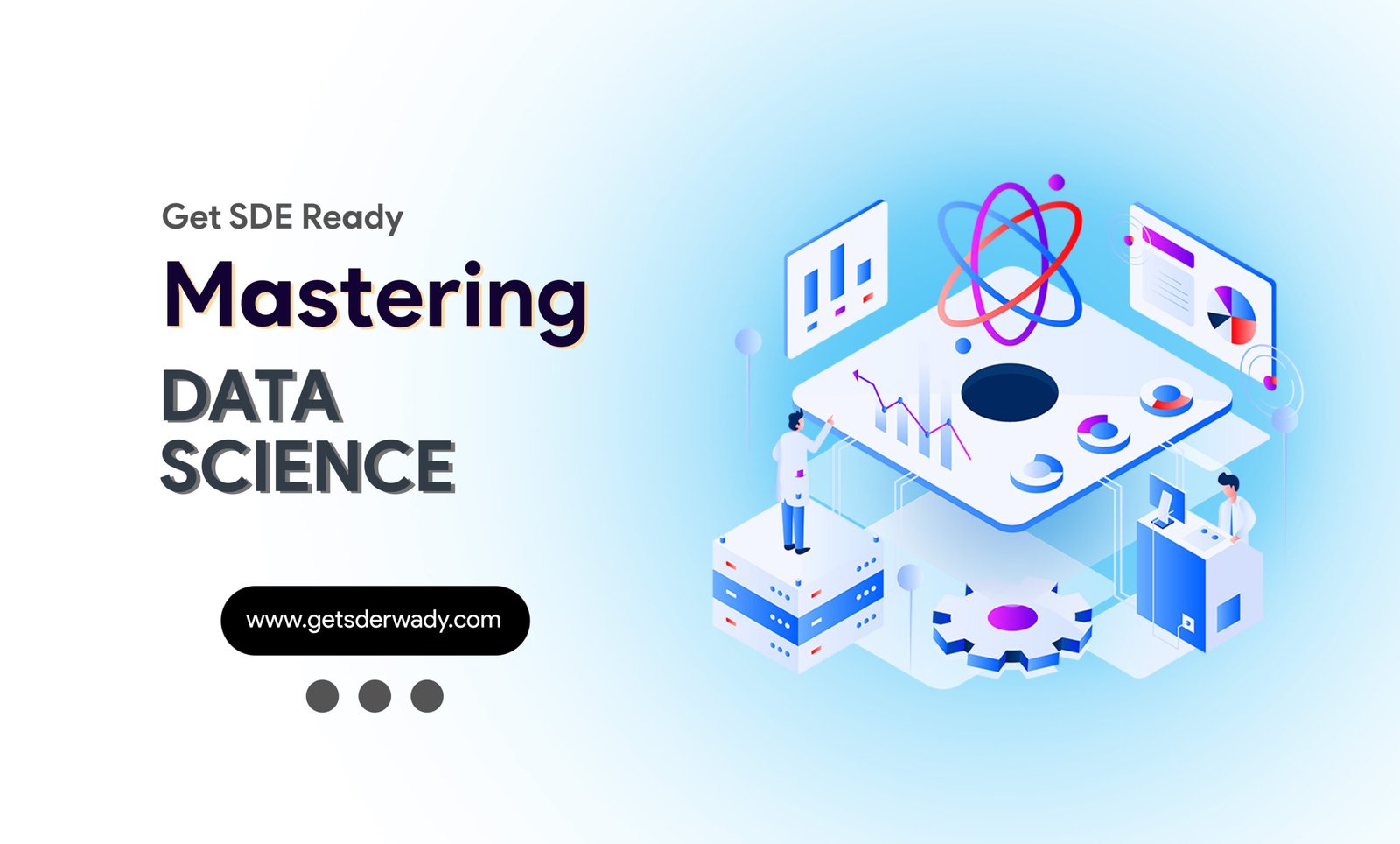
Essentials of Machine Learning and Artificial Intelligence
- 65+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 22+ Hands-on Live Projects & Deployments
- Comprehensive Notes
- Topic-wise Quizzes
- Case Studies
- Access to Global Peer Community
- Interview Prep Material
Buy for 65% OFF
₹20,000.00 ₹6,999.00
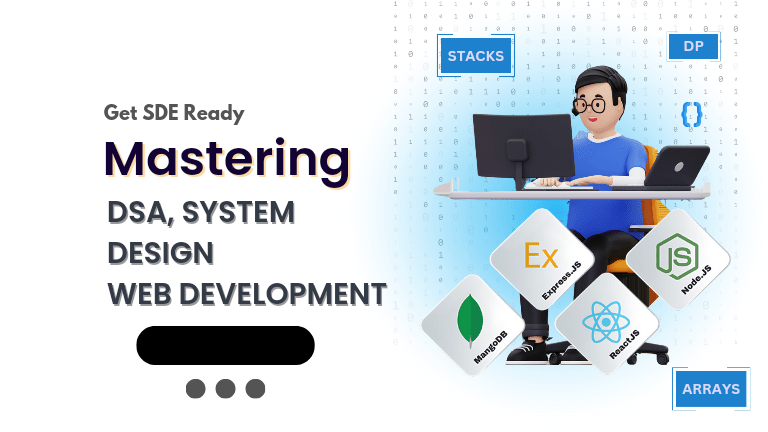
Fast-Track to Full Spectrum Software Engineering
- 120+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 400+ DSA Practice Questions
- Comprehensive Notes
- HackerRank Tests & Quizzes
- 12+ live Projects & Deployments
- Case Studies
- Access to Global Peer Community
Buy for 57% OFF
₹35,000.00 ₹14,999.00
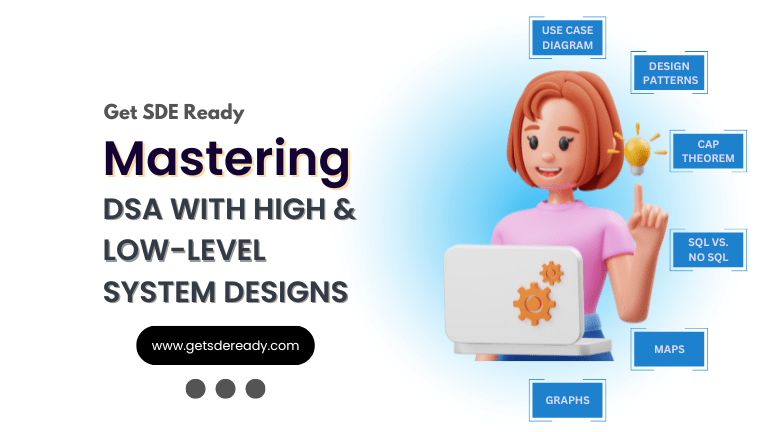
DSA, High & Low Level System Designs
- 85+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 400+ DSA Practice Questions
- Comprehensive Notes
- HackerRank Tests & Quizzes
- Topic-wise Quizzes
- Case Studies
- Access to Global Peer Community
Buy for 60% OFF
₹25,000.00 ₹9,999.00

Low & High Level System Design
- 20+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 400+ DSA Practice Questions
- Comprehensive Notes
- HackerRank Tests
- Topic-wise Quizzes
- Access to Global Peer Community
- Interview Prep Material
Buy for 65% OFF
₹20,000.00 ₹6,999.00
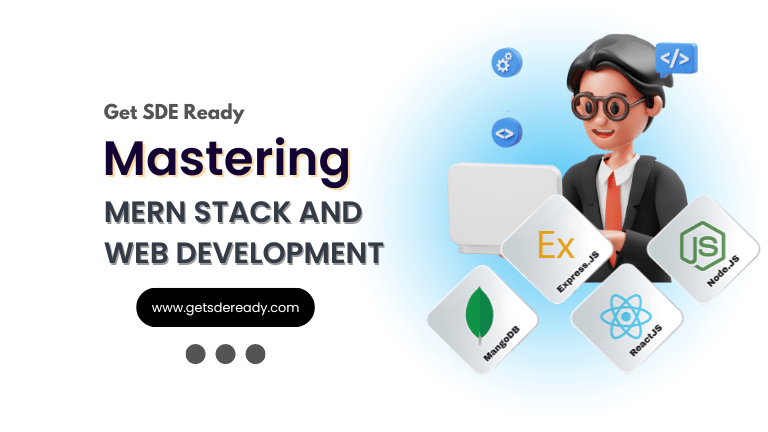
Mastering Mern Stack (WEB DEVELOPMENT)
- 65+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 12+ Hands-on Live Projects & Deployments
- Comprehensive Notes & Quizzes
- Real-world Tools & Technologies
- Access to Global Peer Community
- Interview Prep Material
- Placement Assistance
Buy for 60% OFF
₹15,000.00 ₹5,999.00

Mastering Data Structures & Algorithms
- 65+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 400+ DSA Practice Questions
- Comprehensive Notes
- HackerRank Tests
- Access to Global Peer Community
- Topic-wise Quizzes
- Interview Prep Material
Buy for 50% OFF
₹9,999.00 ₹4,999.00
Reach Out Now
If you have any queries, please fill out this form. We will surely reach out to you.
Contact Email
Reach us at the following email address.
arun@getsdeready.com
Phone Number
You can reach us by phone as well.
+91-97737 28034
Our Location
Rohini, Sector-3, Delhi-110085