Data Structures and Algorithms
- Introduction to Data Structures and Algorithms
- Time and Space Complexity Analysis
- Big-O, Big-Theta, and Big-Omega Notations
- Recursion and Backtracking
- Divide and Conquer Algorithm
- Dynamic Programming: Memoization vs. Tabulation
- Greedy Algorithms and Their Use Cases
- Understanding Arrays: Types and Operations
- Linear Search vs. Binary Search
- Sorting Algorithms: Bubble, Insertion, Selection, and Merge Sort
- QuickSort: Explanation and Implementation
- Heap Sort and Its Applications
- Counting Sort, Radix Sort, and Bucket Sort
- Hashing Techniques: Hash Tables and Collisions
- Open Addressing vs. Separate Chaining in Hashing
- DSA Questions for Beginners
- Advanced DSA Questions for Competitive Programming
- Top 10 DSA Questions to Crack Your Next Coding Test
- Top 50 DSA Questions Every Programmer Should Practice
- Top Atlassian DSA Interview Questions
- Top Amazon DSA Interview Questions
- Top Microsoft DSA Interview Questions
- Top Meta (Facebook) DSA Interview Questions
- Netflix DSA Interview Questions and Preparation Guide
- Top 20 DSA Interview Questions You Need to Know
- Top Uber DSA Interview Questions and Solutions
- Google DSA Interview Questions and How to Prepare
- Airbnb DSA Interview Questions and How to Solve Them
- Mobile App DSA Interview Questions and Solutions
DSA Interview Questions
- DSA Questions for Beginners
- Advanced DSA Questions for Competitive Programming
- Top 10 DSA Questions to Crack Your Next Coding Test
- Top 50 DSA Questions Every Programmer Should Practice
- Top Atlassian DSA Interview Questions
- Top Amazon DSA Interview Questions
- Top Microsoft DSA Interview Questions
- Top Meta (Facebook) DSA Interview Questions
- Netflix DSA Interview Questions and Preparation Guide
- Top 20 DSA Interview Questions You Need to Know
- Top Uber DSA Interview Questions and Solutions
- Google DSA Interview Questions and How to Prepare
- Airbnb DSA Interview Questions and How to Solve Them
- Mobile App DSA Interview Questions and Solutions
Data Structures and Algorithms
- Introduction to Data Structures and Algorithms
- Time and Space Complexity Analysis
- Big-O, Big-Theta, and Big-Omega Notations
- Recursion and Backtracking
- Divide and Conquer Algorithm
- Dynamic Programming: Memoization vs. Tabulation
- Greedy Algorithms and Their Use Cases
- Understanding Arrays: Types and Operations
- Linear Search vs. Binary Search
- Sorting Algorithms: Bubble, Insertion, Selection, and Merge Sort
- QuickSort: Explanation and Implementation
- Heap Sort and Its Applications
- Counting Sort, Radix Sort, and Bucket Sort
- Hashing Techniques: Hash Tables and Collisions
- Open Addressing vs. Separate Chaining in Hashing
- DSA Questions for Beginners
- Advanced DSA Questions for Competitive Programming
- Top 10 DSA Questions to Crack Your Next Coding Test
- Top 50 DSA Questions Every Programmer Should Practice
- Top Atlassian DSA Interview Questions
- Top Amazon DSA Interview Questions
- Top Microsoft DSA Interview Questions
- Top Meta (Facebook) DSA Interview Questions
- Netflix DSA Interview Questions and Preparation Guide
- Top 20 DSA Interview Questions You Need to Know
- Top Uber DSA Interview Questions and Solutions
- Google DSA Interview Questions and How to Prepare
- Airbnb DSA Interview Questions and How to Solve Them
- Mobile App DSA Interview Questions and Solutions
DSA Interview Questions
- DSA Questions for Beginners
- Advanced DSA Questions for Competitive Programming
- Top 10 DSA Questions to Crack Your Next Coding Test
- Top 50 DSA Questions Every Programmer Should Practice
- Top Atlassian DSA Interview Questions
- Top Amazon DSA Interview Questions
- Top Microsoft DSA Interview Questions
- Top Meta (Facebook) DSA Interview Questions
- Netflix DSA Interview Questions and Preparation Guide
- Top 20 DSA Interview Questions You Need to Know
- Top Uber DSA Interview Questions and Solutions
- Google DSA Interview Questions and How to Prepare
- Airbnb DSA Interview Questions and How to Solve Them
- Mobile App DSA Interview Questions and Solutions
Priority Queues: A Comprehensive Guide
Introduction to Priority Queues
A priority queue is a specialized data structure in computer science that extends the concept of a regular queue by assigning a priority to each element. Unlike traditional queues that adhere to the First-In-First-Out (FIFO) principle, priority queues process elements based on their priority levels, ensuring that higher-priority items are handled first. This makes them invaluable in applications like task scheduling in operating systems or pathfinding in algorithms such as Dijkstra’s shortest path. Typically implemented using binary heaps, priority queues offer efficient access to the highest or lowest priority elements.
Looking to sharpen your skills in data structures like priority queues? Sign up for our free courses or stay updated with the latest offerings through our lead capture form.
Types of Priority Queues
Priority queues come in two primary flavors, depending on how they order elements:
- Ascending Order Priority Queue (Min-Heap): Here, elements with lower values are assigned higher priority. For instance, in a queue containing 3, 7, 9, and 12, the number 3 would be dequeued first as it’s the smallest.
- Descending Order Priority Queue (Max-Heap): In this type, higher values equate to higher priority. Using the same example (3, 7, 9, 12), 12 would be dequeued first as the largest element.

Example of Descending order Priority Queue

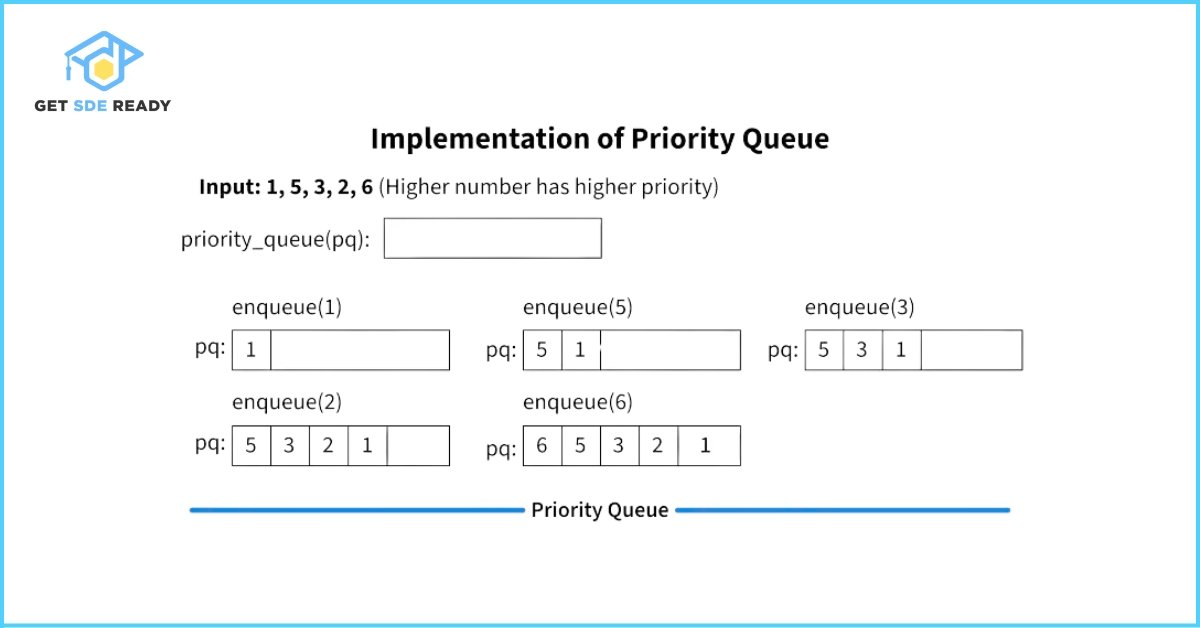
Determining Priority in a Priority Queue
Priority in a queue is generally tied to an element’s value—higher values might indicate higher priority, or vice versa, depending on the design. For example, in a task scheduler, a task with an urgent deadline could be given top priority. Flexibility is key, as custom priority rules can be defined to suit unique requirements, enhancing the adaptability of this data structure.
Operations on Priority Queues
Priority queues support a core set of operations:
- Insertion (Enqueue): Adds a new element, placing it according to its priority. A high-priority item might jump to the front, while others slot in behind.
- Deletion (Dequeue): Removes the element with the highest priority, adjusting the queue to maintain order.
- Peek: Retrieves the highest-priority element without removing it, offering a quick check of what’s next.
These operations, optimized in structures like binary heaps, ensure efficient performance. Learn more about optimizing such operations in our DSA course.
Priority Queue vs. Normal Queue
The key difference between these two lies in their processing logic:
- Normal Queue: Operates on FIFO, where the earliest arrival exits first.
- Priority Queue: Prioritizes elements by their assigned importance, ignoring insertion order.
This makes priority queues ideal for scenarios requiring selective processing over sequential order.
Library Implementations of Priority Queues
Many programming languages offer built-in priority queue support:
These tools simplify usage across diverse projects, from web development to data science.
Implementing Priority Queues
Priority queues can be built using various data structures, each with distinct performance characteristics:
Using Arrays
- Enqueue: O(1) – Add to the end.
- Dequeue: O(n) – Scan for the highest priority.
- Peek: O(n) – Locate the top element.
Using Linked Lists
- Enqueue: O(n) – Insert in priority order.
- Dequeue: O(1) – Remove the front.
- Peek: O(1) – Access the front.
Using Binary Heaps
- Enqueue: O(log n) – Insert and rebalance.
- Dequeue: O(log n) – Remove root and adjust.
- Peek: O(1) – Check the root.
Using Binary Search Trees (BSTs)
- Enqueue: O(log n) – Insert into a balanced BST.
- Dequeue: O(log n) – Remove the extreme value.
- Peek: O(1) – Access the extreme value.
Binary heaps are the go-to choice for their balance of speed and simplicity. Explore these implementations further in our Master DSA, Web Dev, and System Design course.
Problems Based on Priority Queues
Mastering priority queues is easier with practice. Try these problems:
For more, check out our Top 20 DSA Interview Questions.
Applications of Priority Queues
Priority queues shine in numerous real-world scenarios:
- CPU Scheduling: Prioritizes critical processes in operating systems.
- Graph Algorithms: Powers Dijkstra’s and Prim’s algorithms for efficient pathfinding.
- Data Compression: Drives Huffman coding for optimized encoding.
- Event Simulation: Manages time-sensitive events, like customer queues.
These use cases highlight their role in both data science and system design.
Advantages and Disadvantages of Priority Queues
Advantages
- Fast access to top-priority elements.
- Flexible reordering as priorities shift.
- Boosts efficiency in priority-driven algorithms.
Disadvantages
- More complex than basic structures like arrays.
- Higher memory usage due to priority storage.
- Not always optimal for every operation.
Weighing these factors helps in selecting the right tool for the job.
Frequently Asked Questions (FAQs)
What’s the difference between a priority queue and a heap?
A priority queue is a concept defining priority-based access, while a heap is a common implementation method. Learn more in our DSA course.
Can I use an array for a priority queue?
Yes, though it’s less efficient for large datasets due to O(n) dequeue operations. Our Web Development course covers array-based structures.
How are equal-priority elements handled?
Typically, they follow FIFO order within the same priority level. Dive deeper with our Design and DSA Combined course.
Are priority queues always heap-based?
No, alternatives like BSTs or arrays work too, depending on needs. Explore options in our Master DSA, Web Dev, and System Design course.
How can I prepare for DSA interviews using priority queues?
Practice problems and concepts with our Data Science course, tailored for interview success.
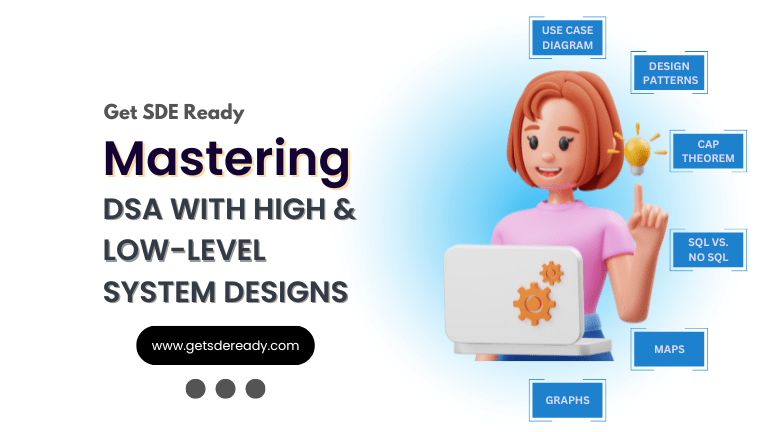
DSA, High & Low Level System Designs
- 85+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 400+ DSA Practice Questions
- Comprehensive Notes
- HackerRank Tests & Quizzes
- Topic-wise Quizzes
- Case Studies
- Access to Global Peer Community
Buy for 60% OFF
₹25,000.00 ₹9,999.00
Accelerate your Path to a Product based Career
Boost your career or get hired at top product-based companies by joining our expertly crafted courses. Gain practical skills and real-world knowledge to help you succeed.
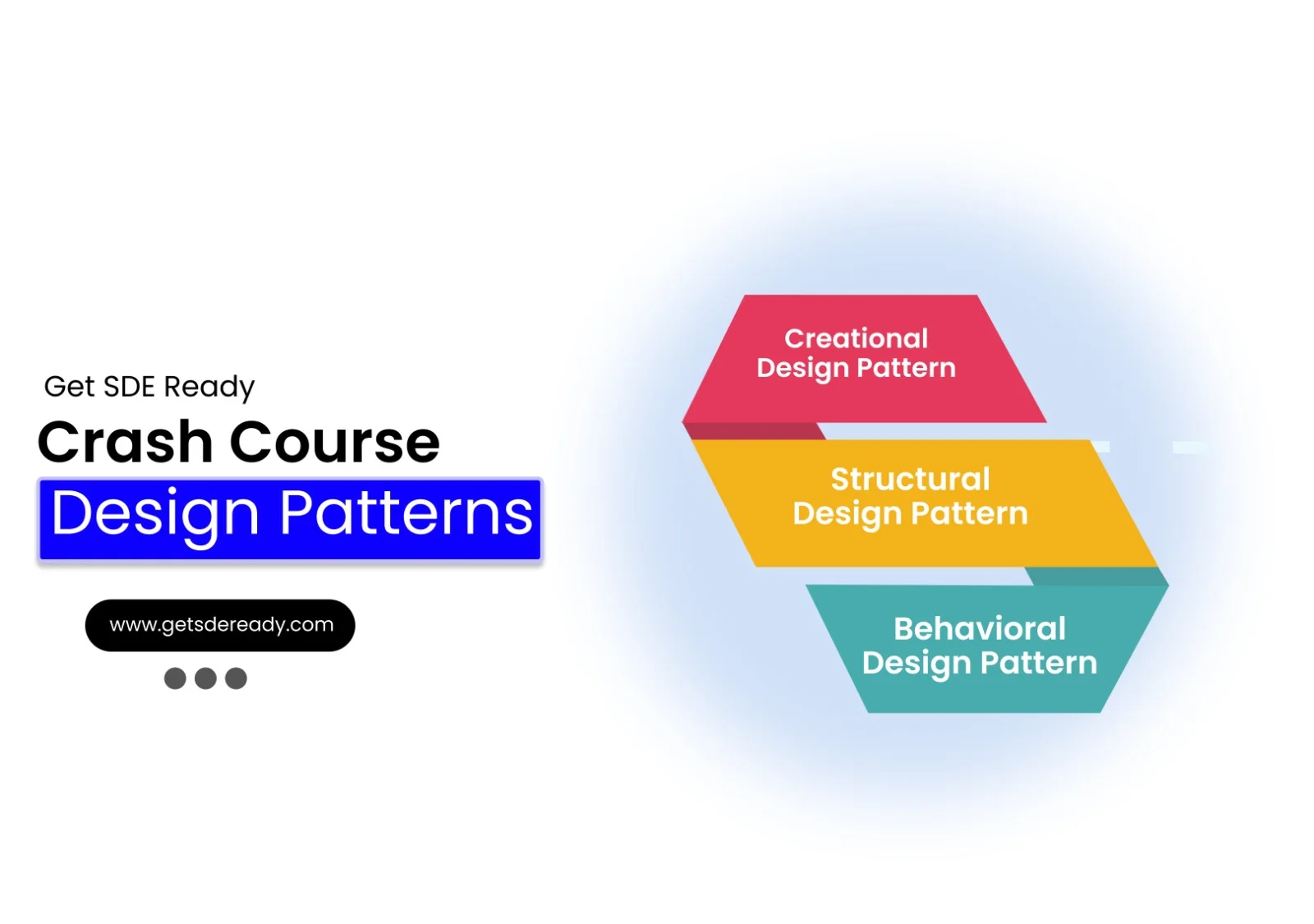
Design Patterns Bootcamp
- Live Classes & Recordings
- 24/7 Live Doubt Support
- Practice Questions
- Case Studies
- Access to Global Peer Community
- Topic wise Quizzes
- Referrals
- Certificate of Completion
Buy for 50% OFF
₹2,000.00 ₹999.00
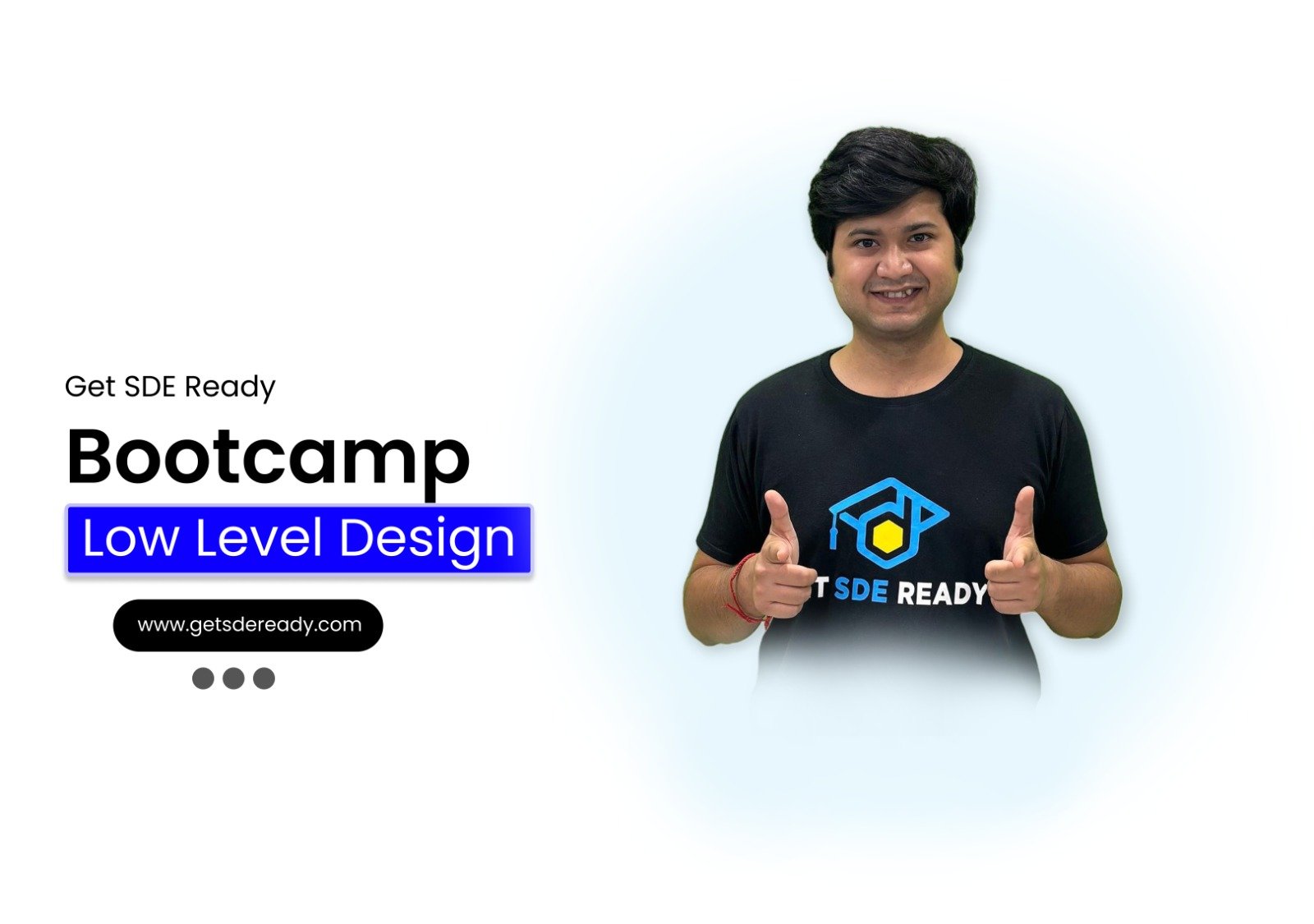
LLD Bootcamp
- 7+ Live Classes & Recordings
- Practice Questions
- 24/7 Live Doubt Support
- Case Studies
- Topic wise Quizzes
- Access to Global Peer Community
- Certificate of Completion
- Referrals
Buy for 50% OFF
₹2,000.00 ₹999.00
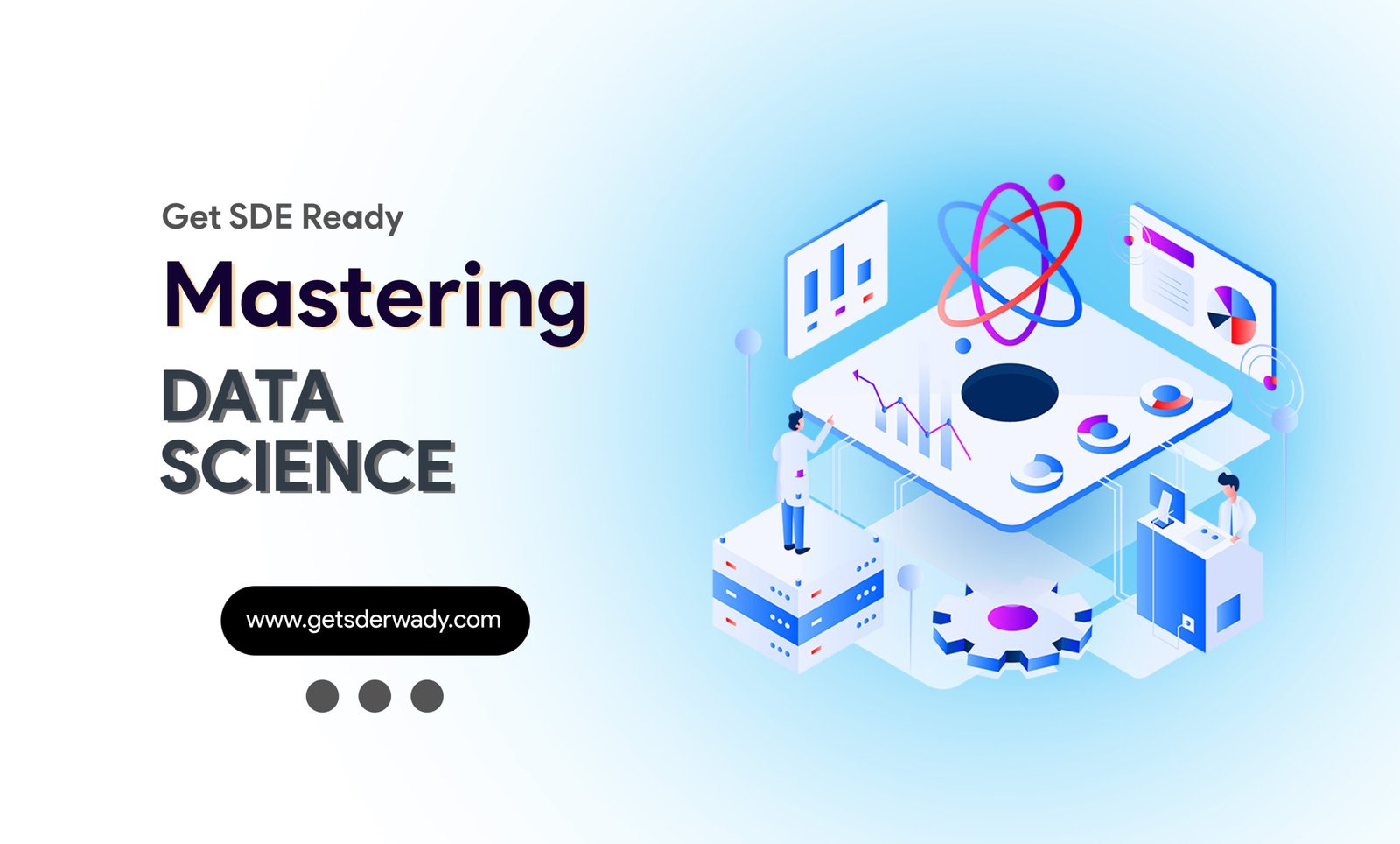
Essentials of Machine Learning and Artificial Intelligence
- 65+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 22+ Hands-on Live Projects & Deployments
- Comprehensive Notes
- Topic-wise Quizzes
- Case Studies
- Access to Global Peer Community
- Interview Prep Material
Buy for 65% OFF
₹20,000.00 ₹6,999.00
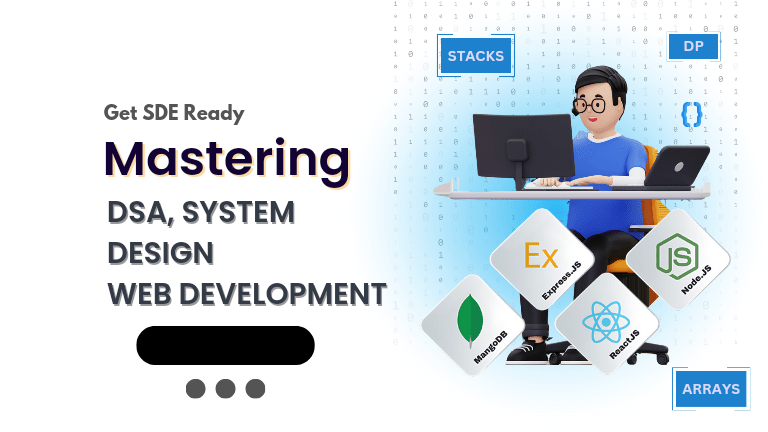
Fast-Track to Full Spectrum Software Engineering
- 120+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 400+ DSA Practice Questions
- Comprehensive Notes
- HackerRank Tests & Quizzes
- 12+ live Projects & Deployments
- Case Studies
- Access to Global Peer Community
Buy for 57% OFF
₹35,000.00 ₹14,999.00
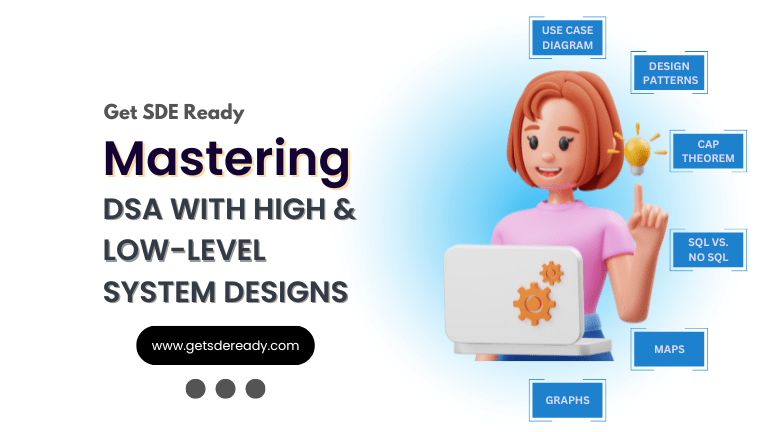
DSA, High & Low Level System Designs
- 85+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 400+ DSA Practice Questions
- Comprehensive Notes
- HackerRank Tests & Quizzes
- Topic-wise Quizzes
- Case Studies
- Access to Global Peer Community
Buy for 60% OFF
₹25,000.00 ₹9,999.00

Low & High Level System Design
- 20+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 400+ DSA Practice Questions
- Comprehensive Notes
- HackerRank Tests
- Topic-wise Quizzes
- Access to Global Peer Community
- Interview Prep Material
Buy for 65% OFF
₹20,000.00 ₹6,999.00
Reach Out Now
If you have any queries, please fill out this form. We will surely reach out to you.
Contact Email
Reach us at the following email address.
Phone Number
You can reach us by phone as well.
+91-97737 28034
Our Location
Rohini, Sector-3, Delhi-110085