Data Structures and Algorithms
- Introduction to Data Structures and Algorithms
- Time and Space Complexity Analysis
- Big-O, Big-Theta, and Big-Omega Notations
- Recursion and Backtracking
- Divide and Conquer Algorithm
- Dynamic Programming: Memoization vs. Tabulation
- Greedy Algorithms and Their Use Cases
- Understanding Arrays: Types and Operations
- Linear Search vs. Binary Search
- Sorting Algorithms: Bubble, Insertion, Selection, and Merge Sort
- QuickSort: Explanation and Implementation
- Heap Sort and Its Applications
- Counting Sort, Radix Sort, and Bucket Sort
- Hashing Techniques: Hash Tables and Collisions
- Open Addressing vs. Separate Chaining in Hashing
- DSA Questions for Beginners
- Advanced DSA Questions for Competitive Programming
- Top 10 DSA Questions to Crack Your Next Coding Test
- Top 50 DSA Questions Every Programmer Should Practice
- Top Atlassian DSA Interview Questions
- Top Amazon DSA Interview Questions
- Top Microsoft DSA Interview Questions
- Top Meta (Facebook) DSA Interview Questions
- Netflix DSA Interview Questions and Preparation Guide
- Top 20 DSA Interview Questions You Need to Know
- Top Uber DSA Interview Questions and Solutions
- Google DSA Interview Questions and How to Prepare
- Airbnb DSA Interview Questions and How to Solve Them
- Mobile App DSA Interview Questions and Solutions
DSA Interview Questions
- DSA Questions for Beginners
- Advanced DSA Questions for Competitive Programming
- Top 10 DSA Questions to Crack Your Next Coding Test
- Top 50 DSA Questions Every Programmer Should Practice
- Top Atlassian DSA Interview Questions
- Top Amazon DSA Interview Questions
- Top Microsoft DSA Interview Questions
- Top Meta (Facebook) DSA Interview Questions
- Netflix DSA Interview Questions and Preparation Guide
- Top 20 DSA Interview Questions You Need to Know
- Top Uber DSA Interview Questions and Solutions
- Google DSA Interview Questions and How to Prepare
- Airbnb DSA Interview Questions and How to Solve Them
- Mobile App DSA Interview Questions and Solutions
Introduction to High-Level System Design
System Design Fundamentals
- Functional vs. Non-Functional Requirements
- Scalability, Availability, and Reliability
- Latency and Throughput Considerations
- Load Balancing Strategies
Architectural Patterns
- Monolithic vs. Microservices Architecture
- Layered Architecture
- Event-Driven Architecture
- Serverless Architecture
- Model-View-Controller (MVC) Pattern
- CQRS (Command Query Responsibility Segregation)
Scaling Strategies
- Vertical Scaling vs. Horizontal Scaling
- Sharding and Partitioning
- Data Replication and Consistency Models
- Load Balancing Strategies
- CDN and Edge Computing
Database Design in HLD
- SQL vs. NoSQL Databases
- CAP Theorem and its Impact on System Design
- Database Indexing and Query Optimization
- Database Sharding and Partitioning
- Replication Strategies
API Design and Communication
Caching Strategies
- Types of Caching
- Cache Invalidation Strategies
- Redis vs. Memcached
- Cache-Aside, Write-Through, and Write-Behind Strategies
Message Queues and Event-Driven Systems
- Kafka vs. RabbitMQ vs. SQS
- Pub-Sub vs. Point-to-Point Messaging
- Handling Asynchronous Workloads
- Eventual Consistency in Distributed Systems
Security in System Design
Observability and Monitoring
- Logging Strategies (ELK Stack, Prometheus, Grafana)
- API Security Best Practices
- Secure Data Storage and Access Control
- DDoS Protection and Rate Limiting
Real-World System Design Case Studies
- Distributed locking (Locking and its Types)
- Memory leaks and Out of memory issues
- HLD of YouTube
- HLD of WhatsApp
System Design Interview Questions
- Adobe System Design Interview Questions
- Top Atlassian System Design Interview Questions
- Top Amazon System Design Interview Questions
- Top Microsoft System Design Interview Questions
- Top Meta (Facebook) System Design Interview Questions
- Top Netflix System Design Interview Questions
- Top Uber System Design Interview Questions
- Top Google System Design Interview Questions
- Top Apple System Design Interview Questions
- Top Airbnb System Design Interview Questions
- Top 10 System Design Interview Questions
- Mobile App System Design Interview Questions
- Top 20 Stripe System Design Interview Questions
- Top Shopify System Design Interview Questions
- Top 20 System Design Interview Questions
- Top Advanced System Design Questions
- Most-Frequented System Design Questions in Big Tech Interviews
- What Interviewers Look for in System Design Questions
- Critical System Design Questions to Crack Any Tech Interview
- Top 20 API Design Questions for System Design Interviews
- Top 10 Steps to Create a System Design Portfolio for Developers
Recursion and Backtracking: Basics and Applications
Want to master recursion and backtracking? These concepts are the backbone of solving complex programming challenges, from algorithm design to coding interviews. Whether you’re preparing for a tech role or sharpening your problem-solving skills, understanding these techniques is essential. Stay ahead of the curve by signing up for free course updates and exclusive resources tailored to help you excel.
Basics of Recursion
Recursion is a programming technique where a function calls itself to solve smaller instances of the same problem. Think of it like a Russian doll: each doll opens to reveal a smaller version until you reach the tiniest one.
What Makes Recursion Work?
- Base Case: The stopping condition that prevents infinite loops.
- Recursive Case: The part where the function calls itself with modified inputs.
For example, calculating a factorial:
def factorial(n):
if n == 0: # Base case
return 1
else: # Recursive case
return n * factorial(n-1)
Key Elements of Recursion
- Divide and Conquer: Break problems into smaller subproblems.
- Stack Utilization: Recursion uses the call stack to manage function calls.
Recursion vs. Iteration |
– Recursion simplifies code but risks stack overflow. |
– Iteration uses loops and is memory-efficient. |
How Recursion Works
When a recursive function runs, each call gets added to the call stack. Once the base case is met, the stack “unwinds,” returning results backward.
Visualizing the Call Stack
Imagine solving a maze: you mark each path you take, backtracking if you hit a dead end. Similarly, the call stack tracks every recursive step.
Common Pitfalls
- Stack Overflow: Occurs with deep recursion (e.g., factorial(10000)).
- Redundant Calculations: Repeatedly solving the same subproblem (e.g., Fibonacci recursion).
For a deeper dive into managing recursion efficiently, explore advanced DSA strategies.

Basics of Backtracking
Backtracking is a refined form of recursion used to explore all possible solutions by building candidates incrementally and abandoning paths that don’t work (“pruning”).
Why Backtracking Matters
- Solves constraint satisfaction problems (e.g., Sudoku, N-Queens).
- Tests partial solutions and backtracks upon failure.
Characteristics of Backtracking
- Incremental Construction: Build solutions step-by-step.
- Pruning: Discard invalid paths early.
Recursion vs. Backtracking |
– Recursion solves subproblems independently. |
– Backtracking explores paths and reverses decisions. |
How Backtracking Works
Backtracking follows a systematic approach:
- Choose: Pick a potential candidate.
- Explore: Recursively check if it leads to a solution.
- Unchoose: Remove the candidate if it fails.
Example: Permutations

def backtrack(path, choices):
if solution_found:
return path
for choice in choices:
if valid(choice):
path.append(choice)
result = backtrack(path, remaining_choices)
if result: return result
path.pop()
return None
Key Differences Between Recursion and Backtracking
Aspect | Recursion | Backtracking |
Purpose | Solve subproblems | Explore all possible solutions |
Efficiency | Can be inefficient | Optimized via pruning |
Use Cases | Tree traversals, Divide & Conquer | Puzzles, Optimization problems |
Applications of Recursion and Backtracking
Real-World Uses
- Recursion: File system traversal, Merge Sort, Tree/Graph algorithms.
- Backtracking: Sudoku solvers, Robot path planning, Combinatorial problems.
Industry Applications |
– AI: Pathfinding in robotics. |
– Bioinformatics: DNA sequence alignment. |
For hands-on practice, check out our comprehensive web development course that integrates DSA with real-world projects.
Common Problems and Solutions
Recursion Challenges
- Stack Overflow: Solve using iteration or memoization.
- Redundant Calls: Use dynamic programming to cache results.
Backtracking Challenges
- Inefficient Pruning: Optimize constraint checks.
- Combinatorial Explosion: Limit depth-first search.
Problem | Solution |
Exponential time complexity | Memoization or iterative DP |
Memory overuse | Tail recursion optimization |

Best Practices and Optimization Techniques
- Memoization: Cache results to avoid recomputation.
- Tail Recursion: Convert recursive calls to loops.
- Pruning Heuristics: Eliminate invalid paths early.
Tip: Always define clear base cases and constraints. Struggling with system design? Our DSA and Web Development combo course covers these optimizations in depth.
How do I decide between recursion and backtracking?
Use recursion for problems with overlapping subproblems (e.g., Fibonacci). Backtracking suits scenarios requiring exhaustive search (e.g., permutations). For structured learning, enroll in our Design and DSA Combined Course.
What’s the biggest mistake beginners make?
Neglecting the base case, leading to infinite loops. Always test edge cases first.
Are these concepts used in coding interviews?
Absolutely! Companies like Google and Meta frequently test recursion and backtracking. Prepare with our Data Science and Algorithm Guide.
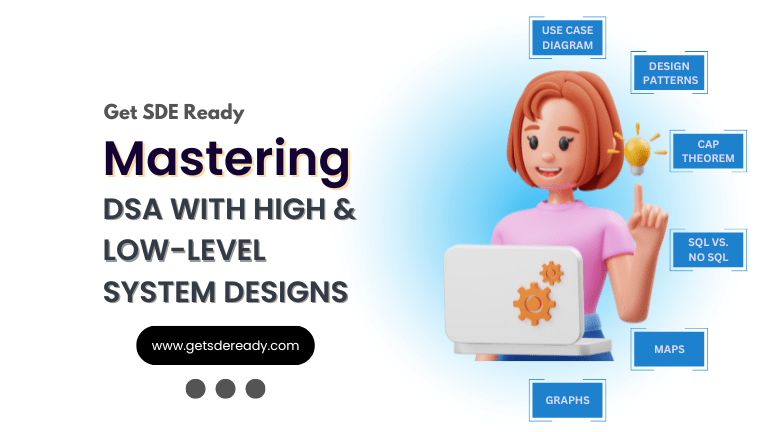
DSA, High & Low Level System Designs
- 85+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 400+ DSA Practice Questions
- Comprehensive Notes
- HackerRank Tests & Quizzes
- Topic-wise Quizzes
- Case Studies
- Access to Global Peer Community
Buy for 60% OFF
₹25,000.00 ₹9,999.00
Accelerate your Path to a Product based Career
Boost your career or get hired at top product-based companies by joining our expertly crafted courses. Gain practical skills and real-world knowledge to help you succeed.

Low & High Level System Design
- 20+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 400+ DSA Practice Questions
- Comprehensive Notes
- HackerRank Tests
- Topic-wise Quizzes
- Access to Global Peer Community
- Interview Prep Material
Buy for 65% OFF
₹20,000.00 ₹6,999.00
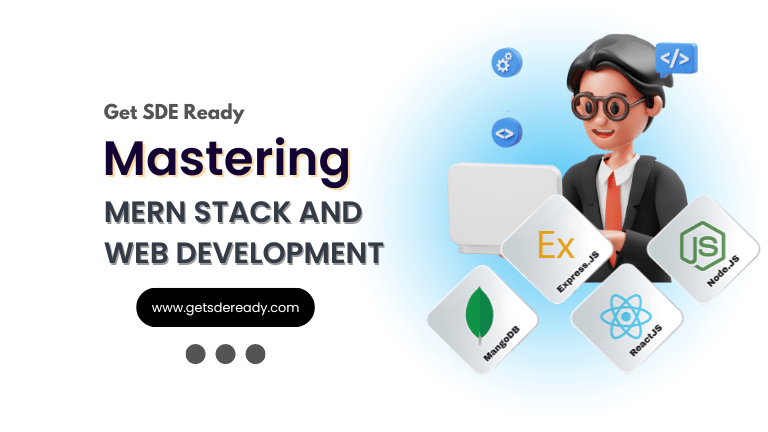
Mastering Mern Stack (WEB DEVELOPMENT)
- 65+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 12+ Hands-on Live Projects & Deployments
- Comprehensive Notes & Quizzes
- Real-world Tools & Technologies
- Access to Global Peer Community
- Interview Prep Material
- Placement Assistance
Buy for 60% OFF
₹15,000.00 ₹5,999.00
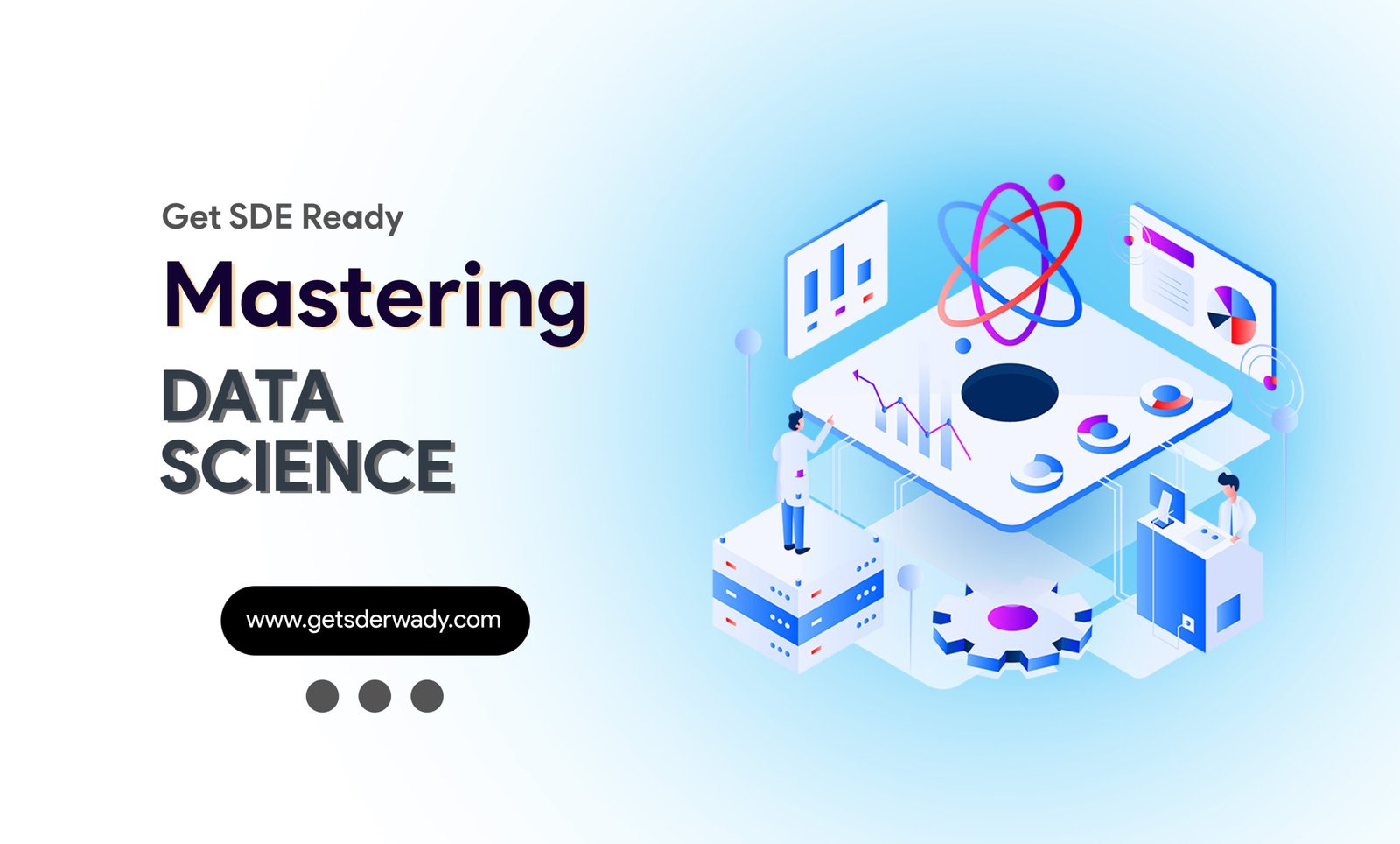
Essentials of Machine Learning and Artificial Intelligence
- 65+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 22+ Hands-on Live Projects & Deployments
- Comprehensive Notes
- Topic-wise Quizzes
- Case Studies
- Access to Global Peer Community
- Interview Prep Material
Buy for 65% OFF
₹20,000.00 ₹6,999.00
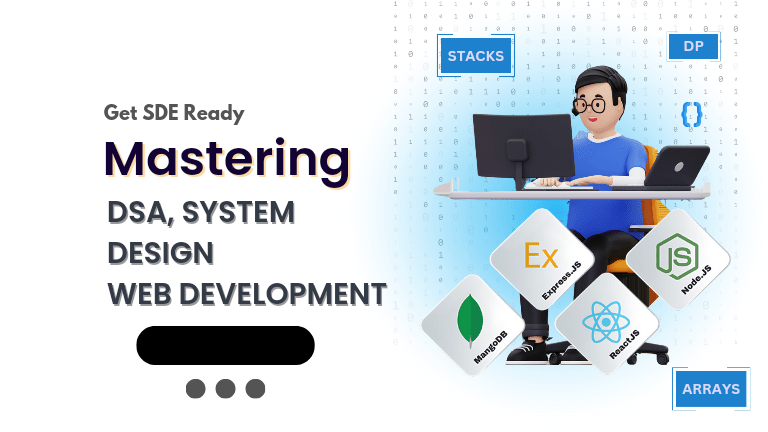
Fast-Track to Full Spectrum Software Engineering
- 120+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 400+ DSA Practice Questions
- Comprehensive Notes
- HackerRank Tests & Quizzes
- 12+ live Projects & Deployments
- Case Studies
- Access to Global Peer Community
Buy for 57% OFF
₹35,000.00 ₹14,999.00
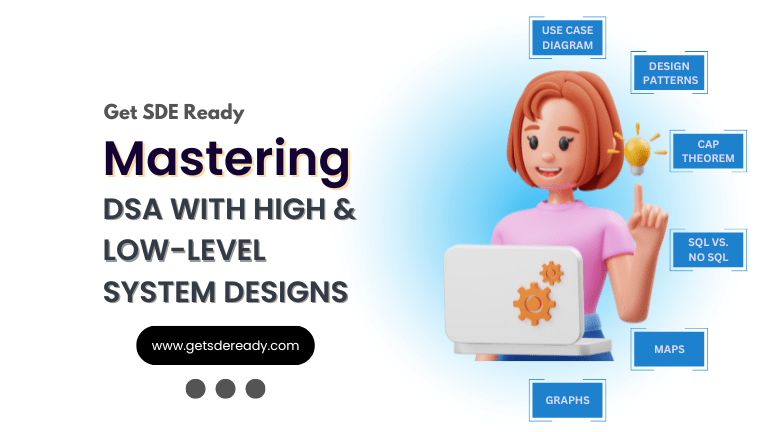
DSA, High & Low Level System Designs
- 85+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 400+ DSA Practice Questions
- Comprehensive Notes
- HackerRank Tests & Quizzes
- Topic-wise Quizzes
- Case Studies
- Access to Global Peer Community
Buy for 60% OFF
₹25,000.00 ₹9,999.00

Low & High Level System Design
- 20+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 400+ DSA Practice Questions
- Comprehensive Notes
- HackerRank Tests
- Topic-wise Quizzes
- Access to Global Peer Community
- Interview Prep Material
Buy for 65% OFF
₹20,000.00 ₹6,999.00
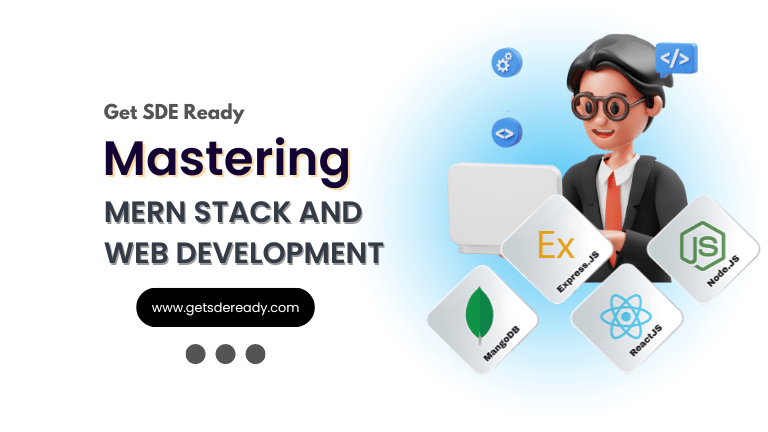
Mastering Mern Stack (WEB DEVELOPMENT)
- 65+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 12+ Hands-on Live Projects & Deployments
- Comprehensive Notes & Quizzes
- Real-world Tools & Technologies
- Access to Global Peer Community
- Interview Prep Material
- Placement Assistance
Buy for 60% OFF
₹15,000.00 ₹5,999.00
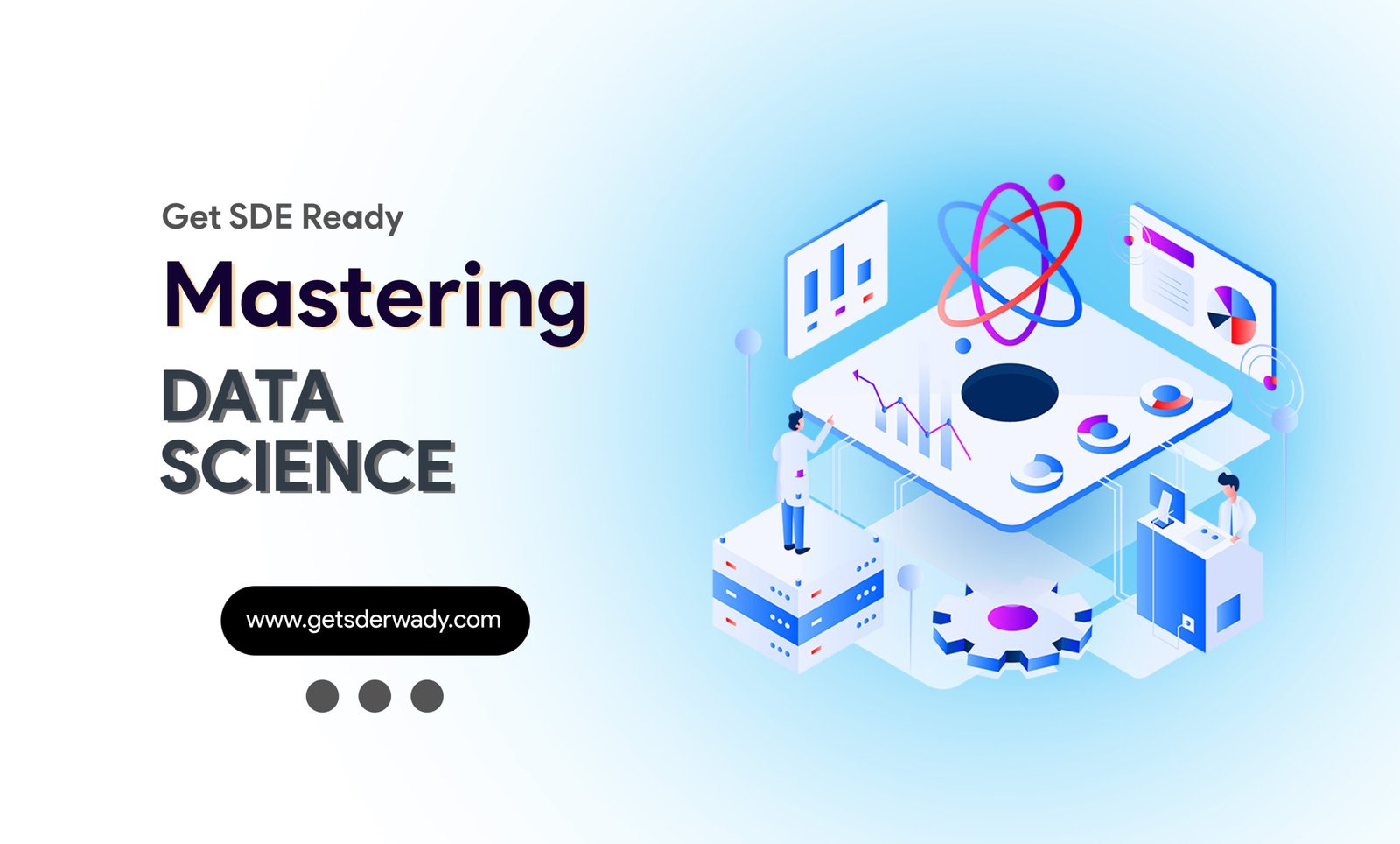
Essentials of Machine Learning and Artificial Intelligence
- 65+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 22+ Hands-on Live Projects & Deployments
- Comprehensive Notes
- Topic-wise Quizzes
- Case Studies
- Access to Global Peer Community
- Interview Prep Material
Buy for 65% OFF
₹20,000.00 ₹6,999.00
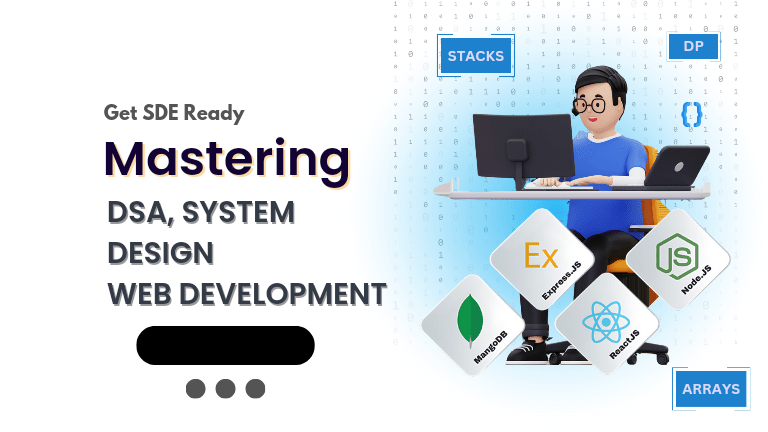
Fast-Track to Full Spectrum Software Engineering
- 120+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 400+ DSA Practice Questions
- Comprehensive Notes
- HackerRank Tests & Quizzes
- 12+ live Projects & Deployments
- Case Studies
- Access to Global Peer Community
Buy for 57% OFF
₹35,000.00 ₹14,999.00
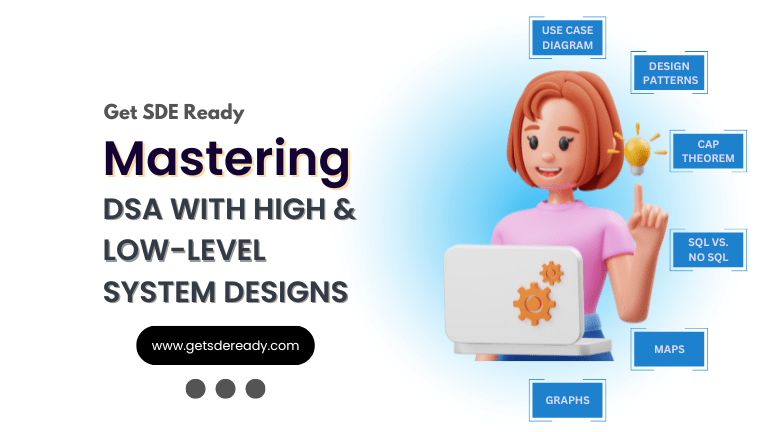
DSA, High & Low Level System Designs
- 85+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 400+ DSA Practice Questions
- Comprehensive Notes
- HackerRank Tests & Quizzes
- Topic-wise Quizzes
- Case Studies
- Access to Global Peer Community
Buy for 60% OFF
₹25,000.00 ₹9,999.00
Reach Out Now
If you have any queries, please fill out this form. We will surely reach out to you.
Contact Email
Reach us at the following email address.
arun@getsdeready.com
Phone Number
You can reach us by phone as well.
+91-97737 28034
Our Location
Rohini, Sector-3, Delhi-110085