DSA Interview Questions
- DSA Questions for Beginners
- Advanced DSA Questions for Competitive Programming
- Top 10 DSA Questions to Crack Your Next Coding Test
- Top 50 DSA Questions Every Programmer Should Practice
- Top Atlassian DSA Interview Questions
- Top Amazon DSA Interview Questions
- Top Microsoft DSA Interview Questions
- Top Meta (Facebook) DSA Interview Questions
- Netflix DSA Interview Questions and Preparation Guide
- Top 20 DSA Interview Questions You Need to Know
- Top Uber DSA Interview Questions and Solutions
- Google DSA Interview Questions and How to Prepare
- Airbnb DSA Interview Questions and How to Solve Them
- Mobile App DSA Interview Questions and Solutions
Introduction to High-Level System Design
System Design Fundamentals
- Functional vs. Non-Functional Requirements
- Scalability, Availability, and Reliability
- Latency and Throughput Considerations
- Load Balancing Strategies
Architectural Patterns
- Monolithic vs. Microservices Architecture
- Layered Architecture
- Event-Driven Architecture
- Serverless Architecture
- Model-View-Controller (MVC) Pattern
- CQRS (Command Query Responsibility Segregation)
Scaling Strategies
- Vertical Scaling vs. Horizontal Scaling
- Sharding and Partitioning
- Data Replication and Consistency Models
- Load Balancing Strategies
- CDN and Edge Computing
Database Design in HLD
- SQL vs. NoSQL Databases
- CAP Theorem and its Impact on System Design
- Database Indexing and Query Optimization
- Database Sharding and Partitioning
- Replication Strategies
API Design and Communication
Caching Strategies
- Types of Caching
- Cache Invalidation Strategies
- Redis vs. Memcached
- Cache-Aside, Write-Through, and Write-Behind Strategies
Message Queues and Event-Driven Systems
- Kafka vs. RabbitMQ vs. SQS
- Pub-Sub vs. Point-to-Point Messaging
- Handling Asynchronous Workloads
- Eventual Consistency in Distributed Systems
Security in System Design
Observability and Monitoring
- Logging Strategies (ELK Stack, Prometheus, Grafana)
- API Security Best Practices
- Secure Data Storage and Access Control
- DDoS Protection and Rate Limiting
Real-World System Design Case Studies
- Distributed locking (Locking and its Types)
- Memory leaks and Out of memory issues
- HLD of YouTube
- HLD of WhatsApp
System Design Interview Questions
- Adobe System Design Interview Questions
- Top Atlassian System Design Interview Questions
- Top Amazon System Design Interview Questions
- Top Microsoft System Design Interview Questions
- Top Meta (Facebook) System Design Interview Questions
- Top Netflix System Design Interview Questions
- Top Uber System Design Interview Questions
- Top Google System Design Interview Questions
- Top Apple System Design Interview Questions
- Top Airbnb System Design Interview Questions
- Top 10 System Design Interview Questions
- Mobile App System Design Interview Questions
- Top 20 Stripe System Design Interview Questions
- Top Shopify System Design Interview Questions
- Top 20 System Design Interview Questions
- Top Advanced System Design Questions
- Most-Frequented System Design Questions in Big Tech Interviews
- What Interviewers Look for in System Design Questions
- Critical System Design Questions to Crack Any Tech Interview
- Top 20 API Design Questions for System Design Interviews
- Top 10 Steps to Create a System Design Portfolio for Developers
Searching Algorithms: Linear Search vs. Binary Search
In today’s data-driven world, knowing how to efficiently locate information in a list or database can make a real difference in application performance. If you’d like to stay ahead of the curve and be the first to receive free resources and course updates in algorithms, you can sign up through our free crash course portal, which will deliver the latest tips and tricks straight to your inbox.
Linear Search
How Linear Search Works
Linear search is the simplest way to find a target value in a list. It begins at the first element and checks each item one by one until it either finds the target or reaches the end of the list. Imagine scanning a row of numbered lockers sequentially until you hit locker number 42—you check 1, 2, 3, and so on until you find your match.
Because of its straightforward nature, linear search does not require the list to be sorted. You can apply it to any collection—arrays, linked lists, or daily log entries—without any preprocessing. This makes it a great first step before diving deeper into more advanced algorithmic strategies, especially if you’re just getting started with our comprehensive DSA curriculum.
Time Complexity of Linear Search
Linear search’s performance depends on the list length, nn:
- Best case: The target is at the first position → O(1)O(1)
- Average case: On average, you check half the list → O(n)O(n); roughly n+12\tfrac{n+1}{2} comparisons
- Worst case: The target is at the last position or absent → O(n)O(n)
Space complexity: Iterative implementations use O(1)O(1) extra space, since only an index is needed.
Advantages of Linear Search
- Simplicity: Easy to understand and implement in just a few lines of code.
- Universal: Works on sorted or unsorted data without any setup.
- No extra memory: Uses constant auxiliary space.
Disadvantages of Linear Search
- Inefficiency for large lists: O(n)O(n) time becomes costly as nn grows.
- High average comparisons: Roughly half the elements must be checked on average.
Scalability issues: Becomes impractical for very large datasets or performance-critical applications.

Binary Search
How Binary Search Works
Binary search dramatically reduces the number of comparisons by halving the search space each step. It requires a sorted array:
- Compare the target with the middle element.
- If equal, you’ve found the target.
- If the target is less, repeat on the left half.
- If the target is greater, repeat on the right half.
This divide-and-conquer approach continues until the target is found or the subarray becomes empty—perfect for systems where speed is key, like those you’ll build in our Master DSA, Web Dev & System Design program.
Time Complexity of Binary Search
Binary search’s efficiency shines in its comparison count:
- Best case: The middle element is the target → O(1)O(1)
- Average/Worst case: Each comparison halves the list → O(logn)O(\log n)
Space complexity: Iterative version uses O(1)O(1), while recursive can incur O(logn)O(\log n) call-stack usage.
Advantages of Binary Search
- Speed: Significantly fewer comparisons for large nn.
- Predictability: Guaranteed logarithmic performance.
- Approximate matching: Can find closest elements or rank queries in O(logn)O(\log n).
Disadvantages of Binary Search
- Sorting requirement: Data must be sorted first, which can cost O(nlogn)O(n \log n).
- Random access needed: Best on arrays or structures supporting direct indexing.
Implementation nuance: Recursive variants risk deep-call issues without tail-recursion optimizations.

Comparison of Linear Search and Binary Search
Performance Comparison
Feature | Linear Search | Binary Search |
Best-case time | O(1)O(1) | O(1)O(1) |
Average-case time | O(n)O(n) | O(logn)O(\log n) |
Worst-case time | O(n)O(n) | O(logn)O(\log n) |
Space complexity | O(1)O(1) | O(1)O(1) / O(logn)O(\log n) |
Data requirement | Unsorted | Sorted |
Implementation | Very simple | Moderate |
Use Cases and Applicability
- Linear Search is ideal for small lists (e.g., under 20 items) or datasets that change often, where continuous sorting would add overhead.
- Binary Search excels in large, static datasets—think millions of records in a financial app—where lightning-fast lookups are non-negotiable.
If you’re building any kind of search feature, our full-stack web development pathway walks you through crafting blazing-fast interfaces and back-ends that leverage these algorithms.
Memory Usage
Linear search benefits from sequential memory access, which plays nicely with caches. Binary search’s jumps can cause more cache misses on huge arrays, a detail you’ll learn to manage in our data-science tutorials on memory profiling.
When to Use Linear Search
- Data is small or unsorted.
- No desire to preprocess.
- Simplicity outweighs performance.
When to Use Binary Search
- Data is sorted or can be kept sorted efficiently.
- Fast query responses are critical.
- You need order-statistics or approximate matches.
Implementing Searching Algorithms in Practice
Pseudocode Examples
Linear Search
function linearSearch(array, target):
for index from 0 to array.length - 1:
if array[index] == target:
return index
return -1
Binary Search
function binarySearch(array, target):
left = 0
right = array.length - 1
while left <= right:
mid = floor((left + right) / 2)
if array[mid] == target:
return mid
else if array[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1
For hands-on coding exercises and deeper examples, our Crash Course in Data Structures & Algorithms is an excellent quick-start resource.
Optimizing Search Performance
Algorithmic Improvements
- Sentinel search: A tweak on linear search that adds a sentinel to avoid boundary checks, shaving off comparisons.
- Interpolation search: Predicts where the target might be based on distribution—offering O(loglogn)O(\log \log n) performance on uniformly distributed data.
Data Structures That Enhance Search
- Binary Search Trees (BSTs): Average O(logn)O(\log n) for search/insert/delete.
- Hash Tables: Near-constant lookup time for exact matches—ideal for dictionary apps or symbol tables.
- Specialized trees: Van Emde Boas trees or tries give sub-logarithmic lookups on integer keys.
To see these structures in action and optimize for real-world constraints, check out our Essential DSA & Web Dev Courses for Programmers.

Which search algorithm should I choose for unsorted data?
Linear search shines on unsorted or very small datasets because it skips any sorting step and works immediately. If you’re just exploring the fundamentals before tackling larger problems, try building a few examples with our top-20 DSA interview questions guide, which walks you through both implementations.
How much faster is binary search compared to linear search?
For a million-element array, linear search might check up to a million items in the worst case, while binary search caps out at around 20 comparisons (log2106≈20\log_2 10^6\approx20). To experiment with these numbers yourself, our Netflix-style DSA prep guide for 2025 offers hands-on drills.
Can I use binary search on linked lists?
No—binary search relies on direct indexing, which linked lists can’t provide efficiently. To convert your data or choose an alternative, our Meta & Facebook interview prep course shows you how seasoned engineers handle these trade-offs in real interviews.
What’s the best way to prepare for search algorithm questions in interviews?
Practice both algorithms until you can code them blindfolded, and then move on to variations like interpolation search. For a targeted collection of problems and solutions, you’ll find our Amazon DSA interview prep guide for 2025 and Atlassian’s 2025 solutions guide indispensable.
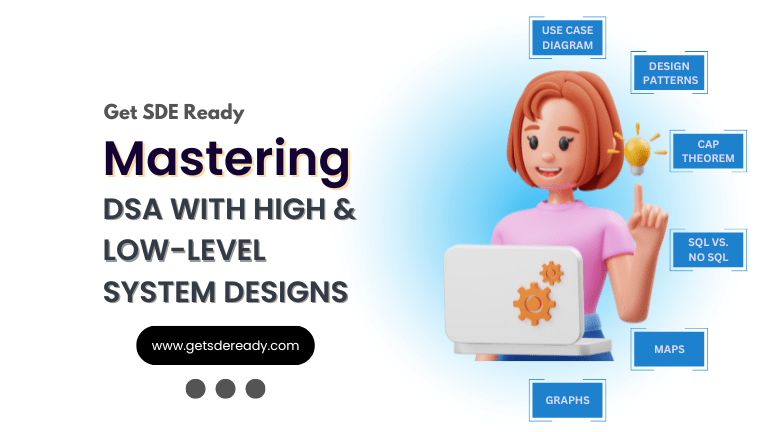
DSA, High & Low Level System Designs
- 85+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 400+ DSA Practice Questions
- Comprehensive Notes
- HackerRank Tests & Quizzes
- Topic-wise Quizzes
- Case Studies
- Access to Global Peer Community
Buy for 60% OFF
₹25,000.00 ₹9,999.00
Accelerate your Path to a Product based Career
Boost your career or get hired at top product-based companies by joining our expertly crafted courses. Gain practical skills and real-world knowledge to help you succeed.
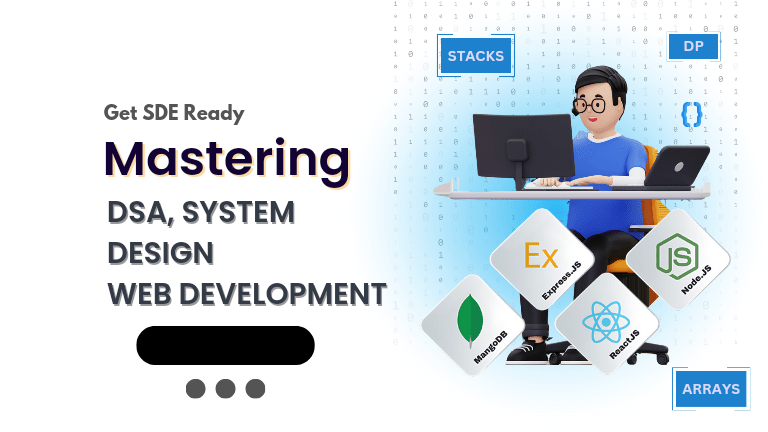
Fast-Track to Full Spectrum Software Engineering
- 120+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 400+ DSA Practice Questions
- Comprehensive Notes
- HackerRank Tests & Quizzes
- 12+ live Projects & Deployments
- Case Studies
- Access to Global Peer Community
Buy for 57% OFF
₹35,000.00 ₹14,999.00
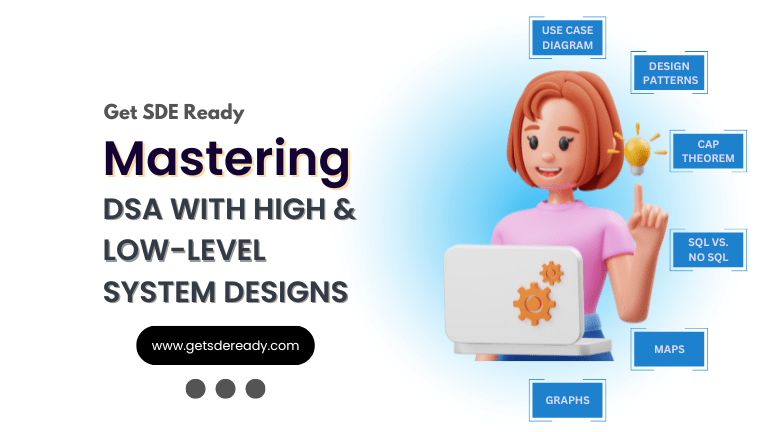
DSA, High & Low Level System Designs
- 85+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 400+ DSA Practice Questions
- Comprehensive Notes
- HackerRank Tests & Quizzes
- Topic-wise Quizzes
- Case Studies
- Access to Global Peer Community
Buy for 60% OFF
₹25,000.00 ₹9,999.00

Mastering Data Structures & Algorithms
- 65+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 400+ DSA Practice Questions
- Comprehensive Notes
- HackerRank Tests
- Access to Global Peer Community
- Topic-wise Quizzes
- Interview Prep Material
Buy for 50% OFF
₹9,999.00 ₹4,999.00
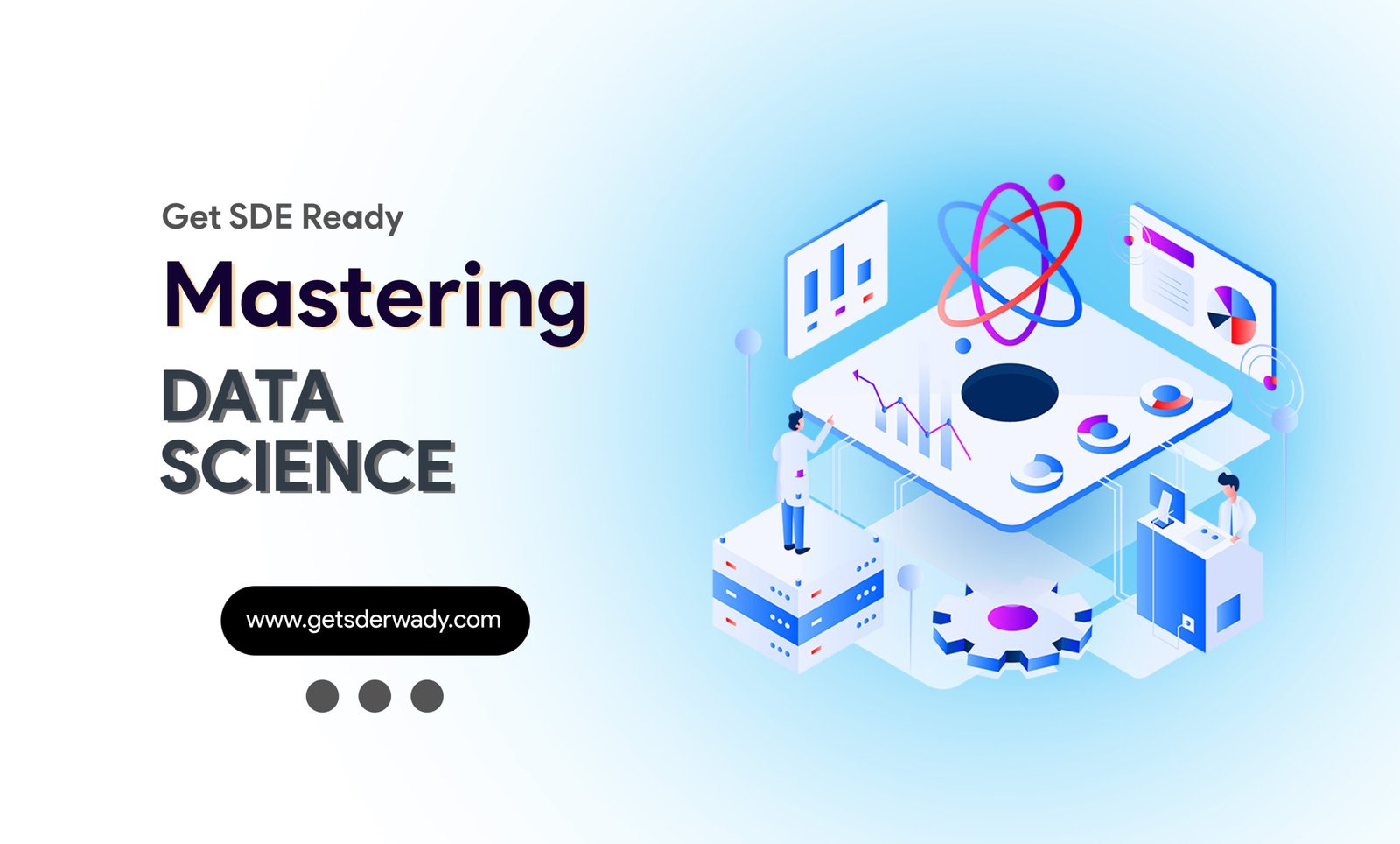
Essentials of Machine Learning and Artificial Intelligence
- 65+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 22+ Hands-on Live Projects & Deployments
- Comprehensive Notes
- Topic-wise Quizzes
- Case Studies
- Access to Global Peer Community
- Interview Prep Material
Buy for 65% OFF
₹20,000.00 ₹6,999.00

Low & High Level System Design
- 20+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 400+ DSA Practice Questions
- Comprehensive Notes
- HackerRank Tests
- Topic-wise Quizzes
- Access to Global Peer Community
- Interview Prep Material
Buy for 65% OFF
₹20,000.00 ₹6,999.00
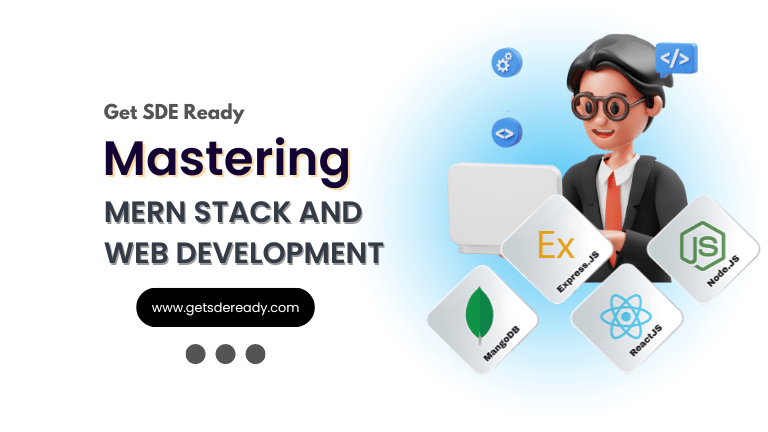
Mastering Mern Stack (WEB DEVELOPMENT)
- 65+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 12+ Hands-on Live Projects & Deployments
- Comprehensive Notes & Quizzes
- Real-world Tools & Technologies
- Access to Global Peer Community
- Interview Prep Material
- Placement Assistance
Buy for 60% OFF
₹15,000.00 ₹5,999.00
Reach Out Now
If you have any queries, please fill out this form. We will surely reach out to you.
Contact Email
Reach us at the following email address.
arun@getsdeready.com
Phone Number
You can reach us by phone as well.
+91-97737 28034
Our Location
Rohini, Sector-3, Delhi-110085