Data Structures and Algorithms
- Introduction to Data Structures and Algorithms
- Time and Space Complexity Analysis
- Big-O, Big-Theta, and Big-Omega Notations
- Recursion and Backtracking
- Divide and Conquer Algorithm
- Dynamic Programming: Memoization vs. Tabulation
- Greedy Algorithms and Their Use Cases
- Understanding Arrays: Types and Operations
- Linear Search vs. Binary Search
- Sorting Algorithms: Bubble, Insertion, Selection, and Merge Sort
- QuickSort: Explanation and Implementation
- Heap Sort and Its Applications
- Counting Sort, Radix Sort, and Bucket Sort
- Hashing Techniques: Hash Tables and Collisions
- Open Addressing vs. Separate Chaining in Hashing
- DSA Questions for Beginners
- Advanced DSA Questions for Competitive Programming
- Top 10 DSA Questions to Crack Your Next Coding Test
- Top 50 DSA Questions Every Programmer Should Practice
- Top Atlassian DSA Interview Questions
- Top Amazon DSA Interview Questions
- Top Microsoft DSA Interview Questions
- Top Meta (Facebook) DSA Interview Questions
- Netflix DSA Interview Questions and Preparation Guide
- Top 20 DSA Interview Questions You Need to Know
- Top Uber DSA Interview Questions and Solutions
- Google DSA Interview Questions and How to Prepare
- Airbnb DSA Interview Questions and How to Solve Them
- Mobile App DSA Interview Questions and Solutions
DSA Interview Questions
- DSA Questions for Beginners
- Advanced DSA Questions for Competitive Programming
- Top 10 DSA Questions to Crack Your Next Coding Test
- Top 50 DSA Questions Every Programmer Should Practice
- Top Atlassian DSA Interview Questions
- Top Amazon DSA Interview Questions
- Top Microsoft DSA Interview Questions
- Top Meta (Facebook) DSA Interview Questions
- Netflix DSA Interview Questions and Preparation Guide
- Top 20 DSA Interview Questions You Need to Know
- Top Uber DSA Interview Questions and Solutions
- Google DSA Interview Questions and How to Prepare
- Airbnb DSA Interview Questions and How to Solve Them
- Mobile App DSA Interview Questions and Solutions
Introduction to High-Level System Design
System Design Fundamentals
- Functional vs. Non-Functional Requirements
- Scalability, Availability, and Reliability
- Latency and Throughput Considerations
- Load Balancing Strategies
Architectural Patterns
- Monolithic vs. Microservices Architecture
- Layered Architecture
- Event-Driven Architecture
- Serverless Architecture
- Model-View-Controller (MVC) Pattern
- CQRS (Command Query Responsibility Segregation)
Scaling Strategies
- Vertical Scaling vs. Horizontal Scaling
- Sharding and Partitioning
- Data Replication and Consistency Models
- Load Balancing Strategies
- CDN and Edge Computing
Database Design in HLD
- SQL vs. NoSQL Databases
- CAP Theorem and its Impact on System Design
- Database Indexing and Query Optimization
- Database Sharding and Partitioning
- Replication Strategies
API Design and Communication
Caching Strategies
- Types of Caching
- Cache Invalidation Strategies
- Redis vs. Memcached
- Cache-Aside, Write-Through, and Write-Behind Strategies
Message Queues and Event-Driven Systems
- Kafka vs. RabbitMQ vs. SQS
- Pub-Sub vs. Point-to-Point Messaging
- Handling Asynchronous Workloads
- Eventual Consistency in Distributed Systems
Security in System Design
Observability and Monitoring
- Logging Strategies (ELK Stack, Prometheus, Grafana)
- API Security Best Practices
- Secure Data Storage and Access Control
- DDoS Protection and Rate Limiting
Real-World System Design Case Studies
- Distributed locking (Locking and its Types)
- Memory leaks and Out of memory issues
- HLD of YouTube
- HLD of WhatsApp
System Design Interview Questions
- Adobe System Design Interview Questions
- Top Atlassian System Design Interview Questions
- Top Amazon System Design Interview Questions
- Top Microsoft System Design Interview Questions
- Top Meta (Facebook) System Design Interview Questions
- Top Netflix System Design Interview Questions
- Top Uber System Design Interview Questions
- Top Google System Design Interview Questions
- Top Apple System Design Interview Questions
- Top Airbnb System Design Interview Questions
- Top 10 System Design Interview Questions
- Mobile App System Design Interview Questions
- Top 20 Stripe System Design Interview Questions
- Top Shopify System Design Interview Questions
- Top 20 System Design Interview Questions
- Top Advanced System Design Questions
- Most-Frequented System Design Questions in Big Tech Interviews
- What Interviewers Look for in System Design Questions
- Critical System Design Questions to Crack Any Tech Interview
- Top 20 API Design Questions for System Design Interviews
- Top 10 Steps to Create a System Design Portfolio for Developers
Singly Linked List: Operations and Use Cases
In the world of computer science, data structures are like the building blocks that help us store and organize information in a way that computers can use efficiently. One of these amazing tools is the singly linked list—a simple yet powerful way to manage data. Whether you’re just starting to code or you’re already a pro, learning about singly linked lists can open doors to solving all kinds of problems. At GetSDEReady, we’re excited to help you on this journey with awesome resources like our DSA course. Want to get the latest tips and free updates to boost your skills? Sign up here and join our community of learners!
This article is your guide to understanding singly linked lists. We’ll explore how they work, the operations you can do with them, and where they pop up in real life. You’ll see why they’re so cool and useful by the end!
What is a Singly Linked List?
Imagine a treasure hunt where each clue leads you to the next one. A singly linked list is a bit like that! It’s a chain of boxes (called nodes) where each box holds some treasure (data) and a map (a pointer) to the next box. The last box doesn’t have a map—it just says “The End” (or null, in computer terms).
Each node in a singly linked list has two parts: the data (like a number or a word) and the “next” pointer that shows where to go next. Unlike a row of lockers (arrays), these nodes don’t need to sit side by side in memory. They can be scattered around, which makes adding or removing boxes super flexible.
Here’s a simple picture of what it looks like:
Head -> [5|Next] -> [10|Next] -> [15|Next] -> null
The “Head” is where we start, and “null” marks the finish line. This setup lets the list grow or shrink whenever we want, which is a big win over arrays that have a fixed size.

Operations on Singly Linked Lists
Singly linked lists are like a playground for operations—you can add, remove, explore, and even rearrange the nodes! These operations come in two flavors: basic ones that everyone learns first and advanced ones that solve trickier puzzles. Let’s dive into both.
Basic Operations
These are the everyday moves you’ll use with singly linked lists. They’re like the ABCs of coding with this data structure: insertion, deletion, traversal, and searching.
Insertion
Insertion is all about adding a new node to the list. You can stick it in different spots, and each spot has its own trick.
- At the Beginning: This is the fastest way to add a node. You make a new node, point it to the current first node (the head), and then make it the new head. It’s like putting a new toy at the front of your toy box—it takes no time at all, just O(1) in tech speak.
- At the End: Here, you add a node to the tail of the list. You have to walk through the whole chain to find the last node, then point its “next” to your new node. This takes longer—O(n) time—because you might have to visit every node.
- At a Specific Spot: Want to squeeze a node between two others? You walk to the node just before where you want it, then adjust the pointers so the new node fits in. This also takes O(n) time since you need to find the right spot.
For example, if your list is 2 -> 3 -> null and you add 1 at the start, it becomes 1 -> 2 -> 3 -> null. Easy peasy!
Insertion is great because it doesn’t need to shuffle everything around like arrays do. Here’s a quick table to sum it up:
Position | Time Complexity | Why It’s Useful |
Beginning | O(1) | Super fast for stacks |
End | O(n) | Adds to the tail |
Specific Spot | O(n) | Flexible placement |
Deletion
Deletion is about taking a node out of the list. Just like adding, you can remove from different places.
- From the Beginning: Snip off the first node by moving the head to the next one. It’s quick—O(1)—because you don’t need to search.
- From the End: To remove the last node, you walk to the second-to-last node and cut its link to the final one by setting “next” to null. This takes O(n) time since you have to travel the list.
- From a Specific Spot: Find the node before the one you want to delete, then skip over the unwanted node by linking to the one after it. This is O(n) too.
Say your list is 1 -> 2 -> 3 -> null. Delete the first node, and it’s 2 -> 3 -> null. Deletion is smooth because you only tweak pointers, not move tons of data.
Here’s a table for deletion:
Position | Time Complexity | Common Use |
Beginning | O(1) | Popping from a stack |
End | O(n) | Removing the last item |
Specific Spot | O(n) | Targeted removal |
Traversal
Traversal is like taking a walk through the list to see what’s inside. You start at the head and follow each “next” pointer until you hit null. It’s how you print the list or count the nodes.
For example, with 1 -> 2 -> 3 -> null, traversal visits 1, then 2, then 3. It takes O(n) time because you check every node. It’s simple but super important for lots of tasks.
Searching
Searching is finding a specific treasure in the list. You start at the head, peek at each node’s data, and keep going until you find it or reach the end. If you’re looking for 2 in 1 -> 2 -> 3 -> null, you’ll find it after two steps. Worst case, it’s O(n) because you might check every node.
Searching isn’t as fast as in arrays (where you can jump straight to a spot), but it’s still handy. Want to ace these basics? Our crash course has you covered!
Advanced Operations
Now, let’s level up with some fancier moves. These operations solve bigger problems and show off the list’s flexibility.
Reversing the List
Reversing flips the list so the last node becomes the first. Imagine 1 -> 2 -> 3 -> null turning into 3 -> 2 -> 1 -> null. You do this by changing each node’s “next” pointer to point backward.
Here’s the trick: use three helpers—previous, current, and next. Move through the list, flipping pointers as you go. It takes O(n) time and is a classic challenge in coding interviews. Check out our Top 20 DSA Interview Questions for practice!
Detecting Loops
Sometimes, a list might loop back on itself, like 1 -> 2 -> 3 -> 2. This can mess up your code with an endless circle! To catch it, use the “tortoise and hare” trick: one pointer moves slow (one step), and another moves fast (two steps). If they meet, there’s a loop. It’s O(n) time and super clever.
Finding the Middle Element
Need the middle node? Use two pointers: slow moves one step, fast moves two. When fast hits the end, slow is at the middle. For 1 -> 2 -> 3 -> 4 -> null, slow lands on 2 or 3 (depending on how you count). It’s O(n) and great for splitting lists.
These advanced moves show how versatile singly linked lists can be. They’re key skills for big projects and interviews!
Use Cases of Singly Linked Lists
Singly linked lists aren’t just for textbooks—they’re used all over the real world! Here are some cool ways they shine.

Implementing Stacks and Queues
Stacks and queues are like toy dispensers—stacks are “last in, first out,” and queues are “first in, first out.” With a singly linked list, you can make a stack by adding and removing from the front (both O(1)). For a queue, add at the end and remove from the front, though end-adds take O(n) unless you keep a tail pointer.
This is perfect for tasks like managing orders or undoing moves. It’s a building block for bigger systems—learn more in our System Design course.
Polynomial Representation
Math lovers, this one’s for you! Polynomials like 3x² + 2x + 1 can be stored as a linked list where each node holds a number (coefficient) and a power (exponent). It’s easy to add or multiply them by tweaking the list. Each node might look like [3,2] -> [2,1] -> [1,0] -> null.
Dynamic Memory Allocation
Computers use linked lists to keep track of free memory. Each node is a chunk of space waiting to be used. When a program needs room, the system picks a node from the list. It’s like handing out toys from a big pile—flexible and efficient.
Undo Functionality in Applications
Ever hit “undo” in a game or text editor? That’s often a linked list at work! Each action (like typing a word) becomes a node. To undo, you step back in the list. It’s a simple way to save and restore steps.
Here’s a quick list of uses:
- Stacks and queues for organizing tasks.
- Polynomials for math magic.
- Memory management in tech.
- Undo features in apps.
These examples show why linked lists are a coder’s friend!
Advantages and Disadvantages
Every tool has its ups and downs, and singly linked lists are no exception. Let’s break it down.
Singly linked lists are awesome because they can grow or shrink as needed—no need to guess the size upfront like with arrays. Adding or removing from the front is lightning-fast (O(1)), and they don’t waste memory by needing one big block. “They’re like a stretchy rubber band compared to the stiff box of an array,” says a coding expert from a popular tech blog.
But they’re not perfect. You can’t jump to a spot in the middle—you have to walk from the start, which takes O(n) time. Plus, each node needs extra space for the “next” pointer, and they’re not great for super-fast memory tricks because the nodes are scattered.
Here’s a table to compare:
Feature | Advantage | Disadvantage |
Size | Grows as needed | – |
Speed (front) | O(1) add/remove | – |
Access | – | O(n) to reach a spot |
Memory | No big block needed | Extra space for pointers |
Comparison with Other Data Structures
How do singly linked lists stack up against arrays and doubly linked lists? Let’s see!
Vs. Arrays
Arrays are like a row of lockers—everything’s lined up, so grabbing item #5 is instant (O(1)). But adding or removing means shifting stuff, which is slow (O(n)). Singly linked lists take O(n) to find something but shine with quick front-end changes.
Vs. Doubly Linked Lists
Doubly linked lists are like singly linked lists with a superpower: they point backward too. This makes moving both ways easier, but they use more memory for the extra pointers. Deleting from the end is faster, though.
Here’s a comparison table:
Feature | Singly Linked List | Array | Doubly Linked List |
Access Time | O(n) | O(1) | O(n) |
Add (front) | O(1) | O(n) | O(1) |
Memory Use | One pointer | None extra | Two pointers |
Traversal | One way | Any way | Both ways |
Picking the right one depends on your task. Need fast access? Go arrays. Need flexibility? Linked lists rock! For more on this, try our essential courses.

What is the time complexity for inserting an element at the beginning of a singly linked list?
Inserting at the beginning is a snap—it’s O(1)! You just make a new node and point it to the old head, then update the head. No need to wander through the list. Want to nail this for interviews? Our DSA course breaks it down step-by-step.
How does a singly linked list differ from an array?
Think of arrays as a neat row of boxes—you can peek inside any box instantly, but adding or removing means shuffling everything. Singly linked lists are a chain where each link leads to the next, so they’re slower to search (O(n)) but quick to change at the front (O(1)). Arrays are fixed; linked lists stretch. Curious about using this in apps? Check out our Web Development course.
Can a singly linked list be used to implement a stack?
Yes, it’s a perfect fit! Add and remove from the front, and both are O(1)—ideal for a stack’s “last in, first out” style. It’s like stacking plates and taking the top one off. Learn this and more with our Master DSA Web Dev System Design course.
What are some real-world applications of singly linked lists?
They’re everywhere! Software uses them for “undo” buttons, operating systems track memory with them, math programs handle polynomials, and they power stacks and queues. They’re champs at growing and shrinking fast. Explore practical uses in our Data Science course.
How do you detect a loop in a singly linked list?
Use the “tortoise and hare” trick! One pointer moves slow, one fast—if they meet, there’s a loop. It’s O(n) and doesn’t need extra space. Prep for this question with our Crash Course—it’s a favorite in tech interviews!
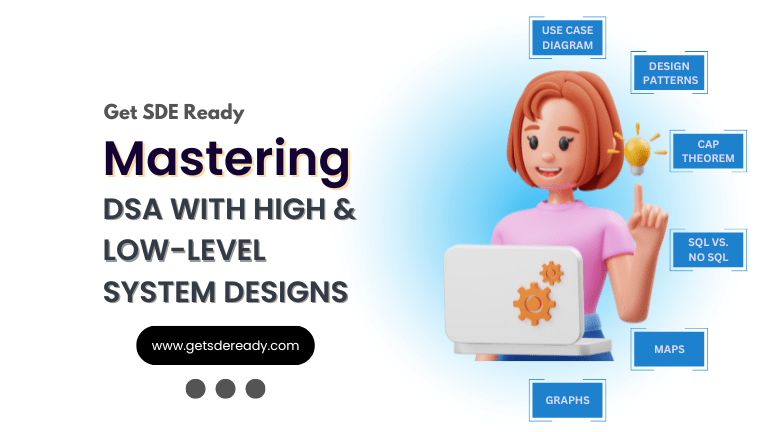
DSA, High & Low Level System Designs
- 85+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 400+ DSA Practice Questions
- Comprehensive Notes
- HackerRank Tests & Quizzes
- Topic-wise Quizzes
- Case Studies
- Access to Global Peer Community
Buy for 60% OFF
₹25,000.00 ₹9,999.00
Accelerate your Path to a Product based Career
Boost your career or get hired at top product-based companies by joining our expertly crafted courses. Gain practical skills and real-world knowledge to help you succeed.
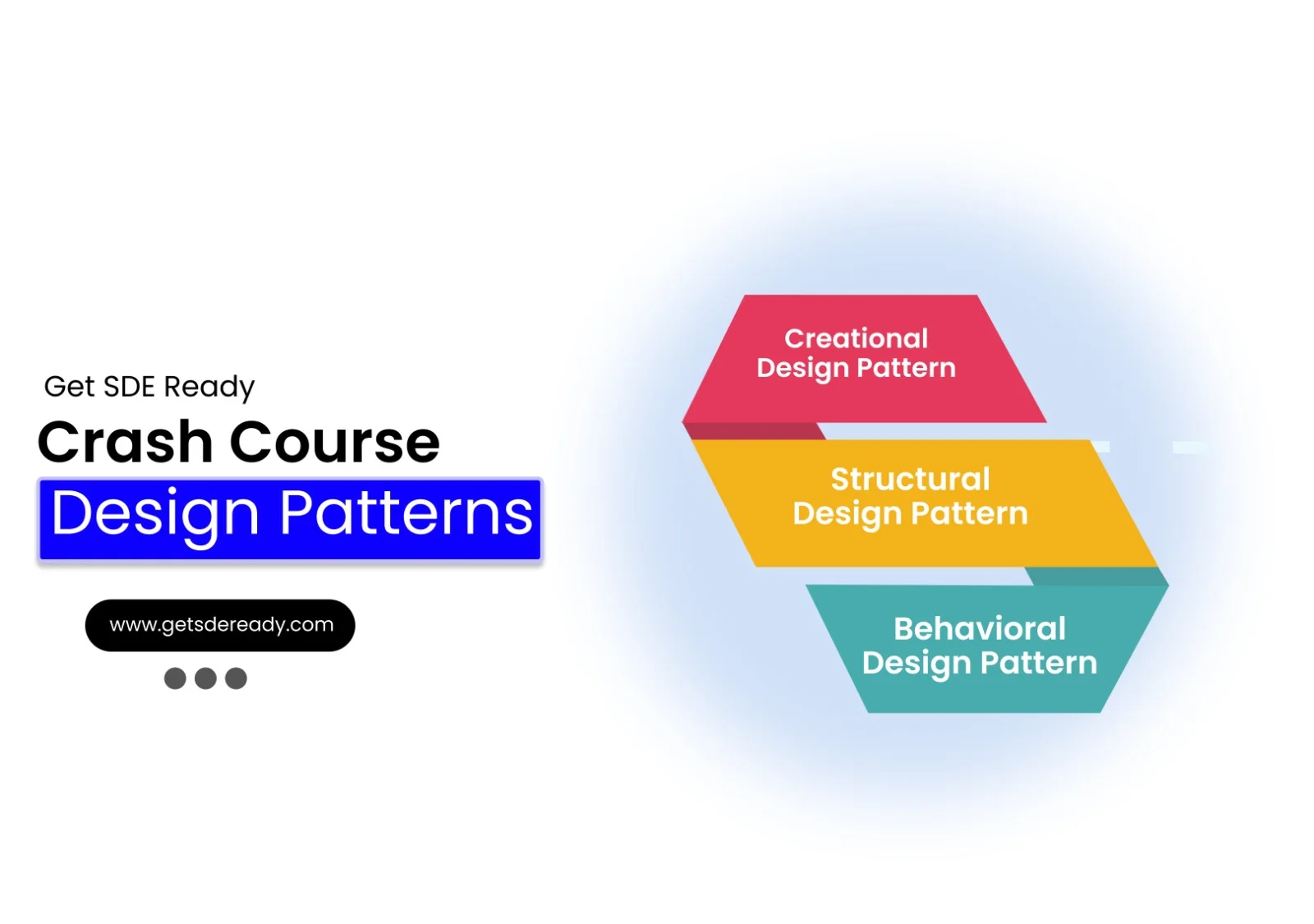
Design Patterns Bootcamp
- Live Classes & Recordings
- 24/7 Live Doubt Support
- Practice Questions
- Case Studies
- Access to Global Peer Community
- Topic wise Quizzes
- Referrals
- Certificate of Completion
Buy for 50% OFF
₹2,000.00 ₹999.00

ML & AI Kickstart
- Live Classes & Recordings
- 24/7 Live Doubt Support
- 2 Live Projects
- Case Studies
- Topic wise Quizzes
- Access to Global Peer Community
- Certificate of Completion
- Referrals
Buy for 50% OFF
₹2,000.00 ₹999.00
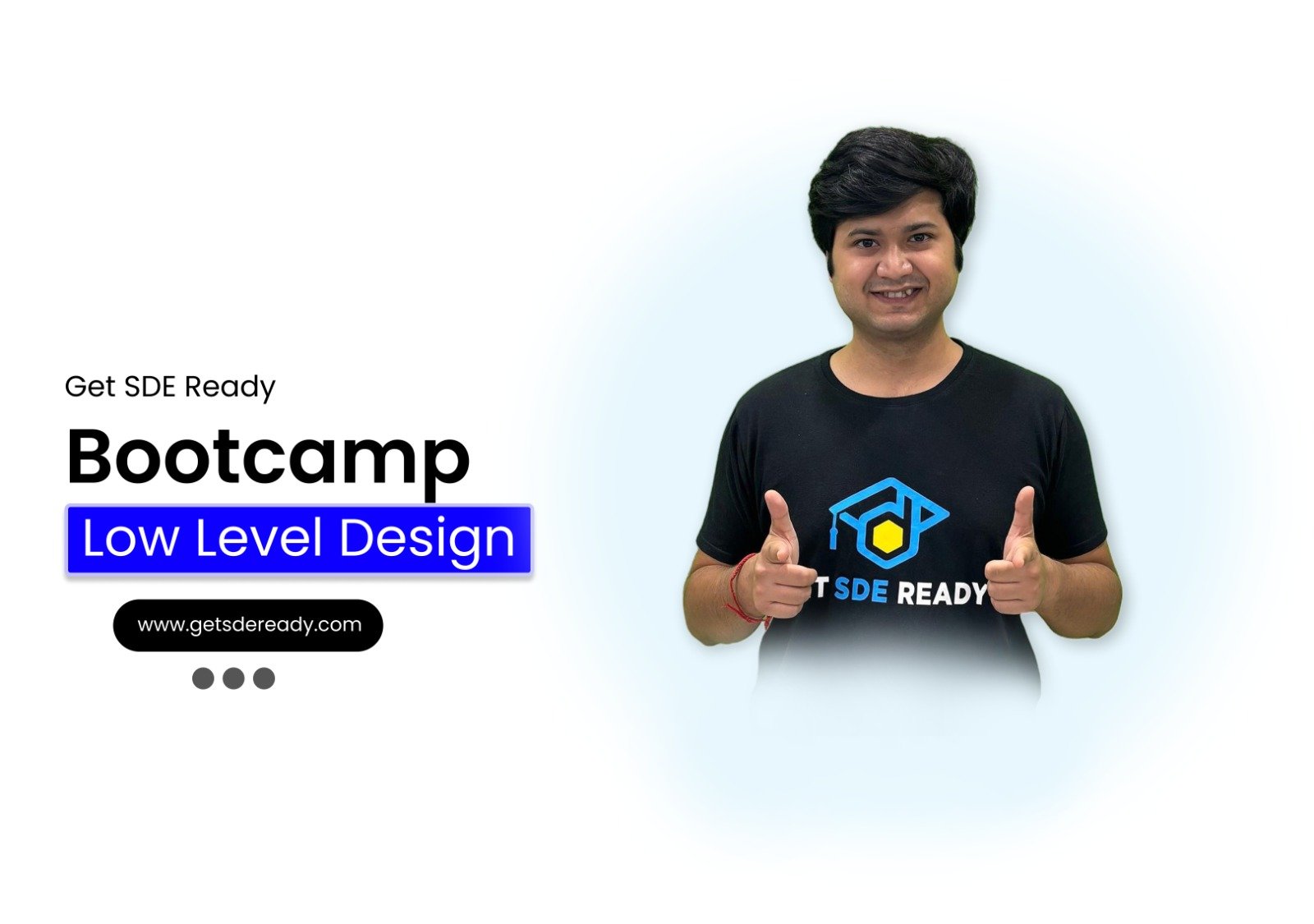
LLD Bootcamp
- 7+ Live Classes & Recordings
- Practice Questions
- 24/7 Live Doubt Support
- Case Studies
- Topic wise Quizzes
- Access to Global Peer Community
- Certificate of Completion
- Referrals
Buy for 50% OFF
₹2,000.00 ₹999.00
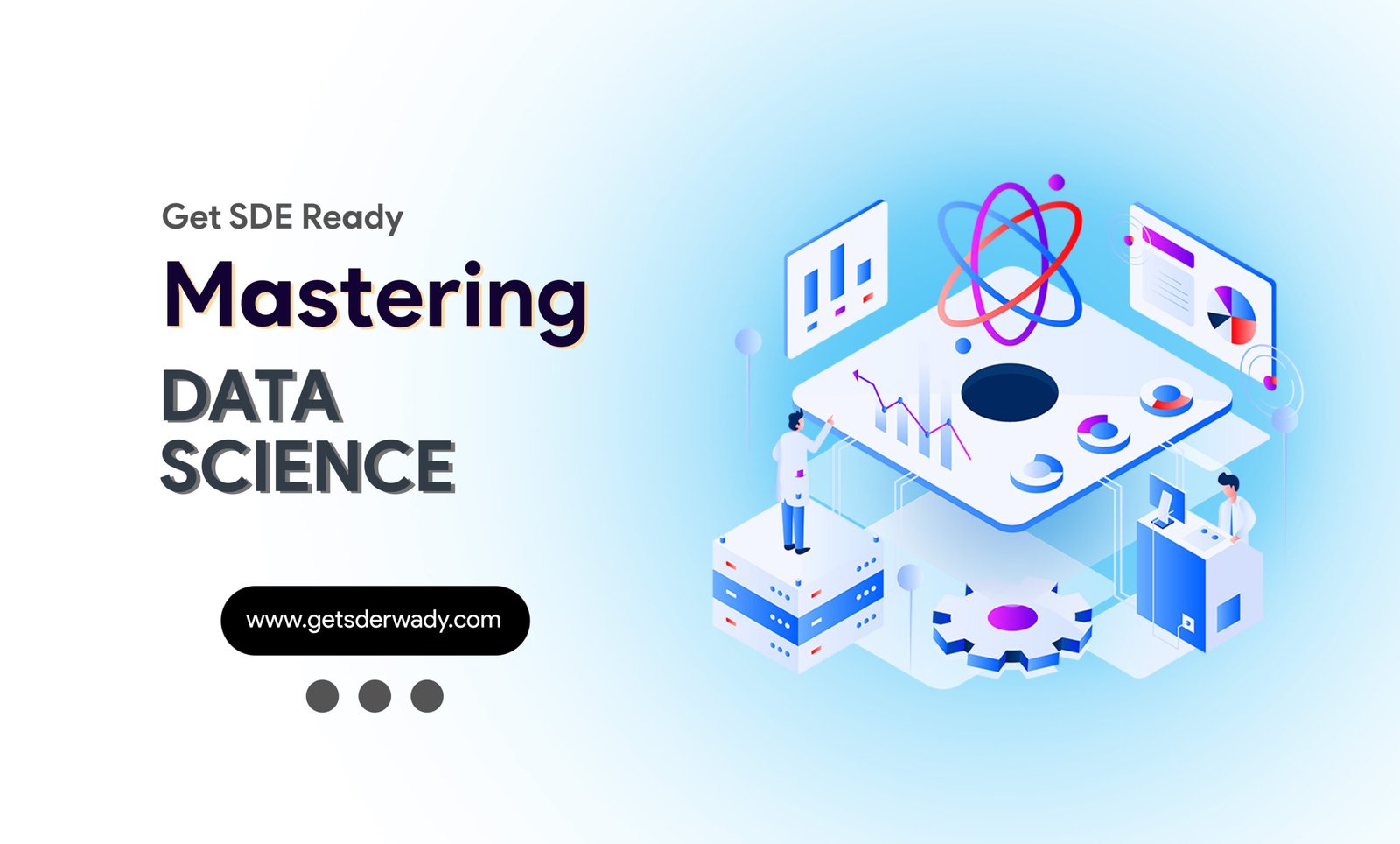
Essentials of Machine Learning and Artificial Intelligence
- 65+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 22+ Hands-on Live Projects & Deployments
- Comprehensive Notes
- Topic-wise Quizzes
- Case Studies
- Access to Global Peer Community
- Interview Prep Material
Buy for 65% OFF
₹20,000.00 ₹6,999.00
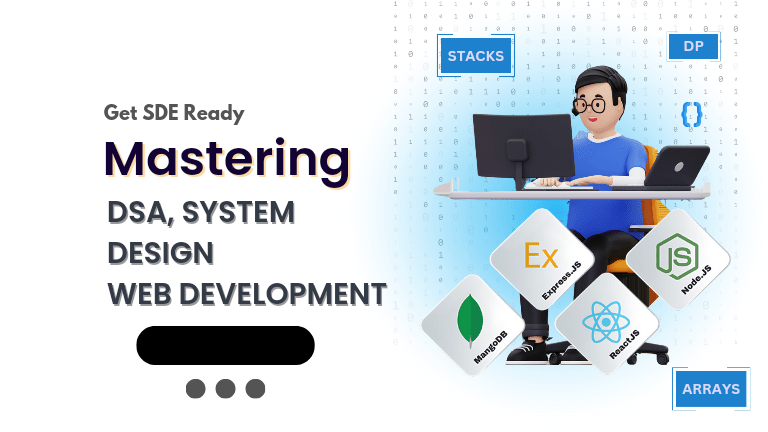
Fast-Track to Full Spectrum Software Engineering
- 120+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 400+ DSA Practice Questions
- Comprehensive Notes
- HackerRank Tests & Quizzes
- 12+ live Projects & Deployments
- Case Studies
- Access to Global Peer Community
Buy for 57% OFF
₹35,000.00 ₹14,999.00
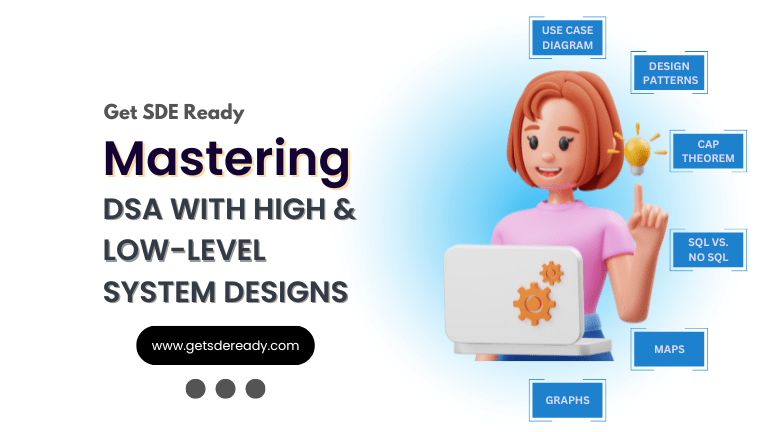
DSA, High & Low Level System Designs
- 85+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 400+ DSA Practice Questions
- Comprehensive Notes
- HackerRank Tests & Quizzes
- Topic-wise Quizzes
- Case Studies
- Access to Global Peer Community
Buy for 60% OFF
₹25,000.00 ₹9,999.00
Reach Out Now
If you have any queries, please fill out this form. We will surely reach out to you.
Contact Email
Reach us at the following email address.
Phone Number
You can reach us by phone as well.
+91-97737 28034
Our Location
Rohini, Sector-3, Delhi-110085