Data Structures and Algorithms
- Introduction to Data Structures and Algorithms
- Time and Space Complexity Analysis
- Big-O, Big-Theta, and Big-Omega Notations
- Recursion and Backtracking
- Divide and Conquer Algorithm
- Dynamic Programming: Memoization vs. Tabulation
- Greedy Algorithms and Their Use Cases
- Understanding Arrays: Types and Operations
- Linear Search vs. Binary Search
- Sorting Algorithms: Bubble, Insertion, Selection, and Merge Sort
- QuickSort: Explanation and Implementation
- Heap Sort and Its Applications
- Counting Sort, Radix Sort, and Bucket Sort
- Hashing Techniques: Hash Tables and Collisions
- Open Addressing vs. Separate Chaining in Hashing
- DSA Questions for Beginners
- Advanced DSA Questions for Competitive Programming
- Top 10 DSA Questions to Crack Your Next Coding Test
- Top 50 DSA Questions Every Programmer Should Practice
- Top Atlassian DSA Interview Questions
- Top Amazon DSA Interview Questions
- Top Microsoft DSA Interview Questions
- Top Meta (Facebook) DSA Interview Questions
- Netflix DSA Interview Questions and Preparation Guide
- Top 20 DSA Interview Questions You Need to Know
- Top Uber DSA Interview Questions and Solutions
- Google DSA Interview Questions and How to Prepare
- Airbnb DSA Interview Questions and How to Solve Them
- Mobile App DSA Interview Questions and Solutions
DSA Interview Questions
- DSA Questions for Beginners
- Advanced DSA Questions for Competitive Programming
- Top 10 DSA Questions to Crack Your Next Coding Test
- Top 50 DSA Questions Every Programmer Should Practice
- Top Atlassian DSA Interview Questions
- Top Amazon DSA Interview Questions
- Top Microsoft DSA Interview Questions
- Top Meta (Facebook) DSA Interview Questions
- Netflix DSA Interview Questions and Preparation Guide
- Top 20 DSA Interview Questions You Need to Know
- Top Uber DSA Interview Questions and Solutions
- Google DSA Interview Questions and How to Prepare
- Airbnb DSA Interview Questions and How to Solve Them
- Mobile App DSA Interview Questions and Solutions
Introduction to High-Level System Design
System Design Fundamentals
- Functional vs. Non-Functional Requirements
- Scalability, Availability, and Reliability
- Latency and Throughput Considerations
- Load Balancing Strategies
Architectural Patterns
- Monolithic vs. Microservices Architecture
- Layered Architecture
- Event-Driven Architecture
- Serverless Architecture
- Model-View-Controller (MVC) Pattern
- CQRS (Command Query Responsibility Segregation)
Scaling Strategies
- Vertical Scaling vs. Horizontal Scaling
- Sharding and Partitioning
- Data Replication and Consistency Models
- Load Balancing Strategies
- CDN and Edge Computing
Database Design in HLD
- SQL vs. NoSQL Databases
- CAP Theorem and its Impact on System Design
- Database Indexing and Query Optimization
- Database Sharding and Partitioning
- Replication Strategies
API Design and Communication
Caching Strategies
- Types of Caching
- Cache Invalidation Strategies
- Redis vs. Memcached
- Cache-Aside, Write-Through, and Write-Behind Strategies
Message Queues and Event-Driven Systems
- Kafka vs. RabbitMQ vs. SQS
- Pub-Sub vs. Point-to-Point Messaging
- Handling Asynchronous Workloads
- Eventual Consistency in Distributed Systems
Security in System Design
Observability and Monitoring
- Logging Strategies (ELK Stack, Prometheus, Grafana)
- API Security Best Practices
- Secure Data Storage and Access Control
- DDoS Protection and Rate Limiting
Real-World System Design Case Studies
- Distributed locking (Locking and its Types)
- Memory leaks and Out of memory issues
- HLD of YouTube
- HLD of WhatsApp
System Design Interview Questions
- Adobe System Design Interview Questions
- Top Atlassian System Design Interview Questions
- Top Amazon System Design Interview Questions
- Top Microsoft System Design Interview Questions
- Top Meta (Facebook) System Design Interview Questions
- Top Netflix System Design Interview Questions
- Top Uber System Design Interview Questions
- Top Google System Design Interview Questions
- Top Apple System Design Interview Questions
- Top Airbnb System Design Interview Questions
- Top 10 System Design Interview Questions
- Mobile App System Design Interview Questions
- Top 20 Stripe System Design Interview Questions
- Top Shopify System Design Interview Questions
- Top 20 System Design Interview Questions
- Top Advanced System Design Questions
- Most-Frequented System Design Questions in Big Tech Interviews
- What Interviewers Look for in System Design Questions
- Critical System Design Questions to Crack Any Tech Interview
- Top 20 API Design Questions for System Design Interviews
- Top 10 Steps to Create a System Design Portfolio for Developers
Doubly Linked List: Operations and Use Cases
Ready to explore a key data structure in programming? Doubly linked lists are a step up from singly linked lists, offering unique advantages that make them essential for many applications. Before we dive in, sign up for our exclusive updates and free resources to boost your skills: Sign Up Now. Let’s unravel the power of doubly linked lists together!
Introduction to Linked Lists
Linked lists are a dynamic way to store data, unlike arrays that use fixed, contiguous memory. Each element, or node, in a linked list holds data and a pointer to the next node, allowing flexible additions and removals without shifting elements.
There are two primary types:
- Singly Linked Lists: Nodes point only to the next node, like a one-way path.
- Doubly Linked Lists: Nodes link to both the next and previous nodes, enabling movement in either direction, much like a two-way street.
Doubly linked lists excel when you need to traverse both ways or frequently modify the list. They’re more intricate but highly practical. Learn more in our Data Structures and Algorithms course.
Structure of a Doubly Linked List
A doubly linked list consists of nodes, each with three components:
- Data: The value stored, such as a number or string.
- Next Pointer: Links to the following node.
- Previous Pointer: Links to the preceding node.
In languages like C++ or Java, nodes are often defined using a structure or class containing these fields. This setup allows traversal from the head (first node) to the tail (last node) or vice versa, resembling a chain where each link knows its neighbors. The head’s previous pointer and the tail’s next pointer are null, defining the list’s ends. This dual-pointer design uses more memory but simplifies operations like reversing or deleting nodes.
Imagine a list like this:
- Node 1: Data = 5, Next = Node 2, Previous = Null (head).
- Node 2: Data = 10, Next = Node 3, Previous = Node 1.
- Node 3: Data = 15, Next = Null (tail), Previous = Node 2.

Basic Operations on Doubly Linked Lists
Doubly linked lists support essential operations, made efficient by their bidirectional links. Let’s explore them with simple analogies.
Traversal
Traversal involves visiting each node to process its data. With doubly linked lists, you can:
- Move Forward: Start at the head, process the data, and follow the next pointer until reaching the tail (where next is null). It’s like walking a trail from start to finish.
- Move Backward: Begin at the tail, process the data, and follow the previous pointer until hitting the head (where previous is null). This is like retracing your steps.
This flexibility is perfect for tasks requiring data access in both directions.
Insertion
Adding a new node is like linking a new piece into a chain. There are three main methods:
At the Beginning
- Create a new node with the data.
- Point its next pointer to the current head.
- If the list isn’t empty, set the current head’s previous pointer to the new node.
- Make the new node the head.
This is quick and doesn’t require traversing the list.
At the End
- Create a new node.
- If the list is empty, it becomes the head.
- Otherwise, find the last node (where next is null), link its next pointer to the new node, and set the new node’s previous pointer to the last node.
- Update the tail to the new node.
With a tail pointer, this is fast; otherwise, traversal is needed.
At a Specific Position
- Create a new node.
- Traverse to the target position.
- Link the new node’s next pointer to the following node and its previous pointer to the current node.
- Adjust the surrounding nodes’ pointers to include the new node.
This keeps the chain intact by updating both directions.
Deletion
Removing a node is like unlinking a chain segment. Here’s how:
From the Beginning
- If the list isn’t empty, move the head to the next node.
- Set the new head’s previous pointer to null.
- Free the old head’s memory.
This is straightforward and fast.
From the End
- If the list isn’t empty, locate the last node.
- Set the second-last node’s next pointer to null, making it the new tail.
- Free the old tail’s memory.
A tail pointer speeds this up.
From a Specific Position
- Traverse to the node to remove.
- Link the previous node’s next pointer to the following node.
- Link the following node’s previous pointer to the previous node.
- Free the removed node’s memory.
This ensures the list remains connected.
Finding the Length
To count nodes, start at the head, increment a counter for each node, and follow the next pointers until reaching the tail. This takes O(n) time, as every node must be visited, similar to counting links in a chain.
Time Complexities:
Operation | Time Complexity |
Traversal (Forward/Backward) | O(n) |
Insertion (Beginning/End) | O(1) |
Insertion (Specific Position) | O(n) |
Deletion (Beginning/End) | O(1) |
Deletion (Specific Position) | O(n) |
Finding Length | O(n) |
Note: O(1) applies when positions are known (e.g., head or tail); traversal raises it to O(n).
Advanced Operations
Doubly linked lists also handle more advanced tasks:
- Searching: Traverse forward or backward to find a value, taking O(n) time.
- Reversing: Swap each node’s next and previous pointers, then swap head and tail, in O(n) time.
- Sorting: Rearrange nodes (e.g., via merge sort) in O(n log n) time, though less common.
These are popular in interviews. Check our Top DSA Interview Questions.
Use Cases for Doubly Linked Lists
Doubly linked lists shine in real-world scenarios:
- Text Editors: Store actions for undo (previous) and redo (next).
- Caches: Enable fast addition/removal in systems like LRU caches.
- Browser History: Navigate back and forth between pages.
- Music Playlists: Skip forward or backward in apps like Spotify.
- Deques: Support efficient operations at both ends.
Explore these in our Web Development course.

Advantages and Disadvantages
Advantages
- Dual Traversal: Move in both directions easily.
- Quick Modifications: Insert or delete in O(1) at known spots.
- Flexibility: Can build stacks, queues, or deques.
Disadvantages
- Memory Use: Two pointers per node increase overhead.
- Complexity: More intricate than singly linked lists.
Comparison Table:
Feature | Doubly Linked List | Singly Linked List |
Traversal | Both Directions | Forward Only |
Memory per Node | Data + 2 Pointers | Data + 1 Pointer |
Modification Time | O(1) (known spot) | O(n) (deletion) |
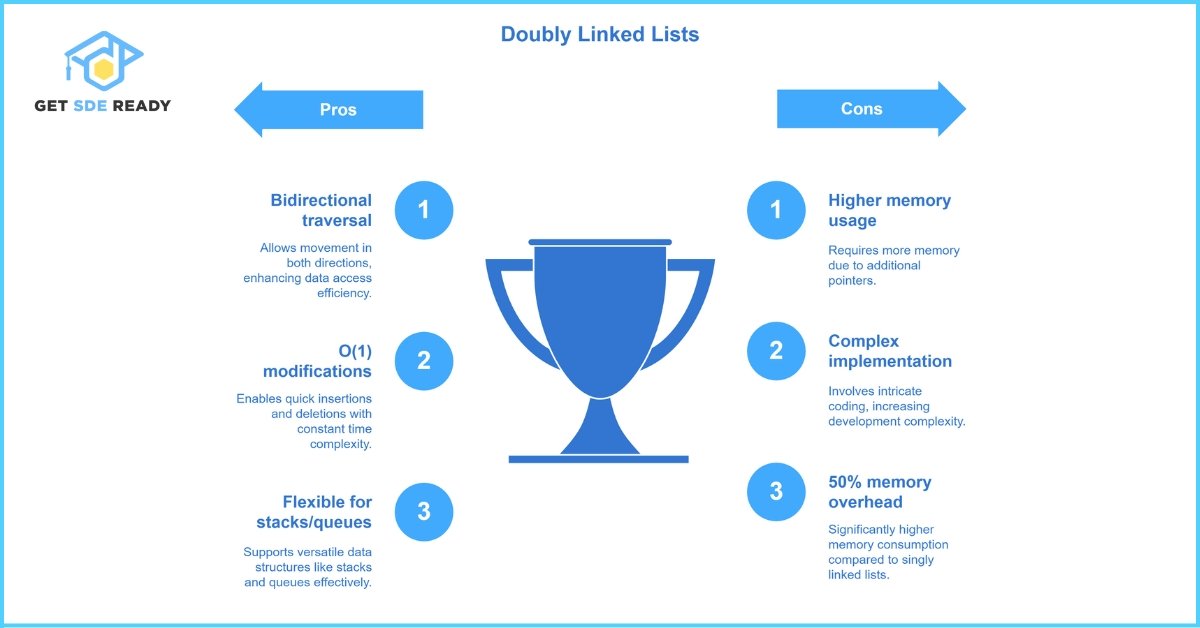
Comparison with Other Data Structures
- Arrays: Fast access (O(1)), but slow modifications (O(n)).
- Singly Linked Lists: Less memory, limited traversal.
- Circular Doubly Linked Lists: Loop head to tail for cyclic tasks.
See more in our Essential DSA Courses.
Implementing a Doubly Linked List
Implementation involves managing pointers carefully. For instance, inserting at the end requires linking the new node to the current tail and updating the tail. Languages like C++ use structures with data and dual pointers to define nodes, ensuring smooth operations.
Try it in our Master DSA, Web Dev & System Design course.
Common Interview Questions
- Reverse a Doubly Linked List: Swap pointers in O(n).
- Find the Middle: Use fast/slow pointers in O(n).
- Time Complexity: O(1) for known-position edits, O(n) for searches.
Prep with our Amazon DSA Interview Questions Guide.
What’s the time complexity of insertion/deletion?
O(1) at known positions, O(n) if traversal is needed. See our Data Structures and Algorithms course.
How much memory does it use?
More than singly linked lists due to dual pointers. Learn more in our System Design course.
Can it be circular?
Yes, linking tail to head creates a loop, useful for cyclic tasks.
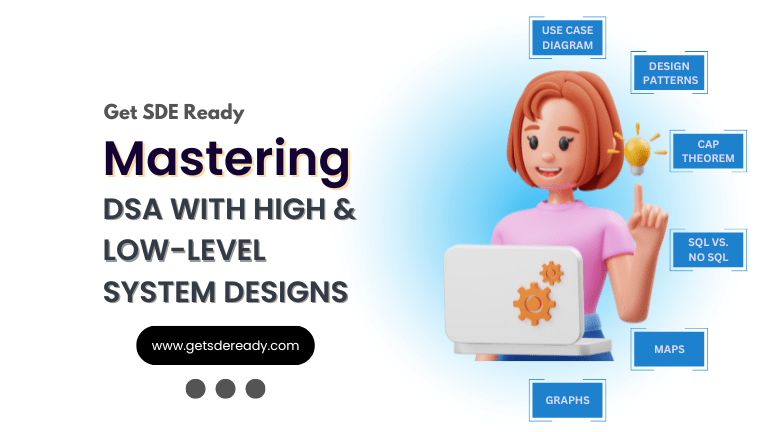
DSA, High & Low Level System Designs
- 85+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 400+ DSA Practice Questions
- Comprehensive Notes
- HackerRank Tests & Quizzes
- Topic-wise Quizzes
- Case Studies
- Access to Global Peer Community
Buy for 60% OFF
₹25,000.00 ₹9,999.00
Accelerate your Path to a Product based Career
Boost your career or get hired at top product-based companies by joining our expertly crafted courses. Gain practical skills and real-world knowledge to help you succeed.
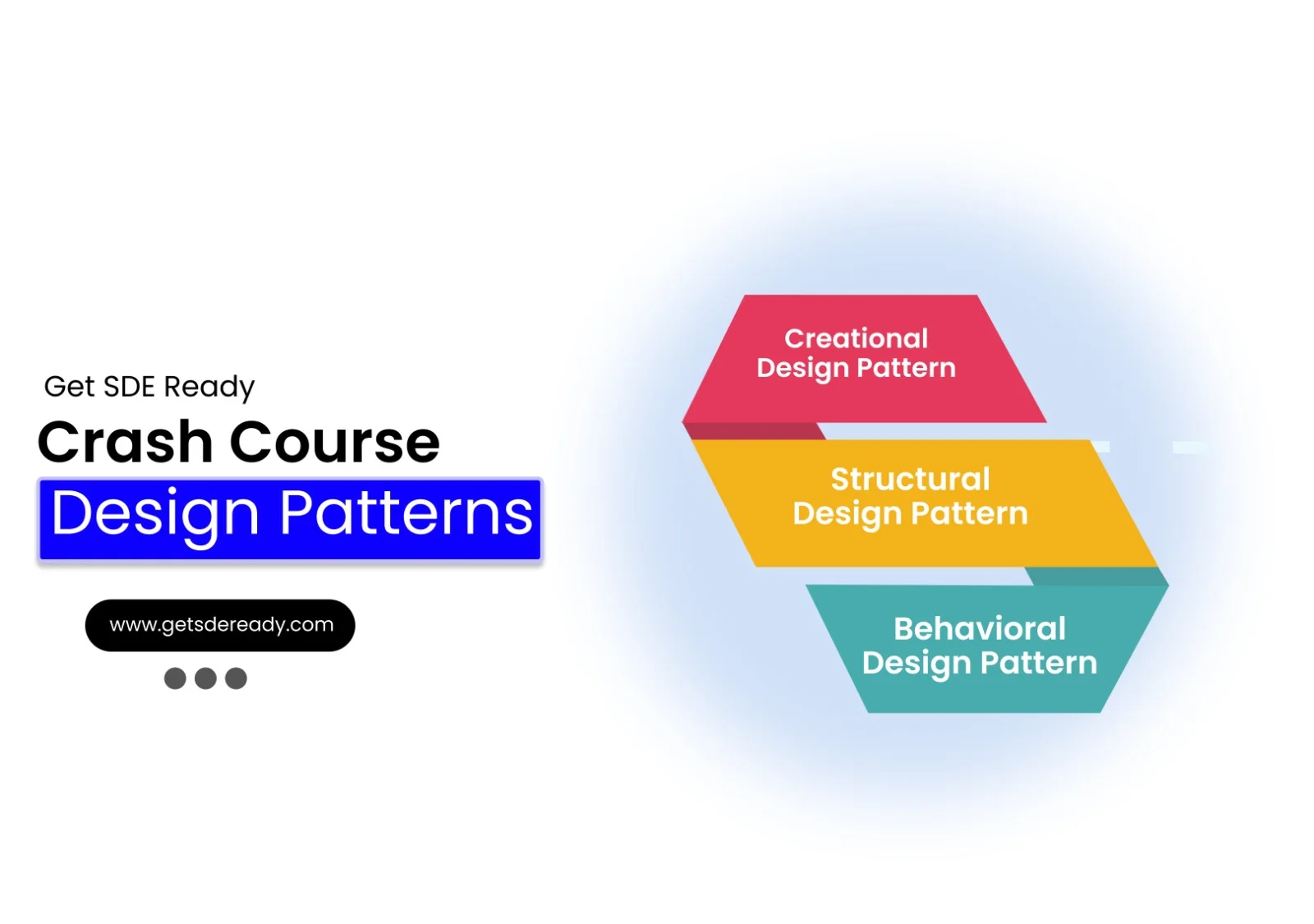
Design Patterns Bootcamp
- Live Classes & Recordings
- 24/7 Live Doubt Support
- Practice Questions
- Case Studies
- Access to Global Peer Community
- Topic wise Quizzes
- Referrals
- Certificate of Completion
Buy for 50% OFF
₹2,000.00 ₹999.00

ML & AI Kickstart
- Live Classes & Recordings
- 24/7 Live Doubt Support
- 2 Live Projects
- Case Studies
- Topic wise Quizzes
- Access to Global Peer Community
- Certificate of Completion
- Referrals
Buy for 50% OFF
₹2,000.00 ₹999.00
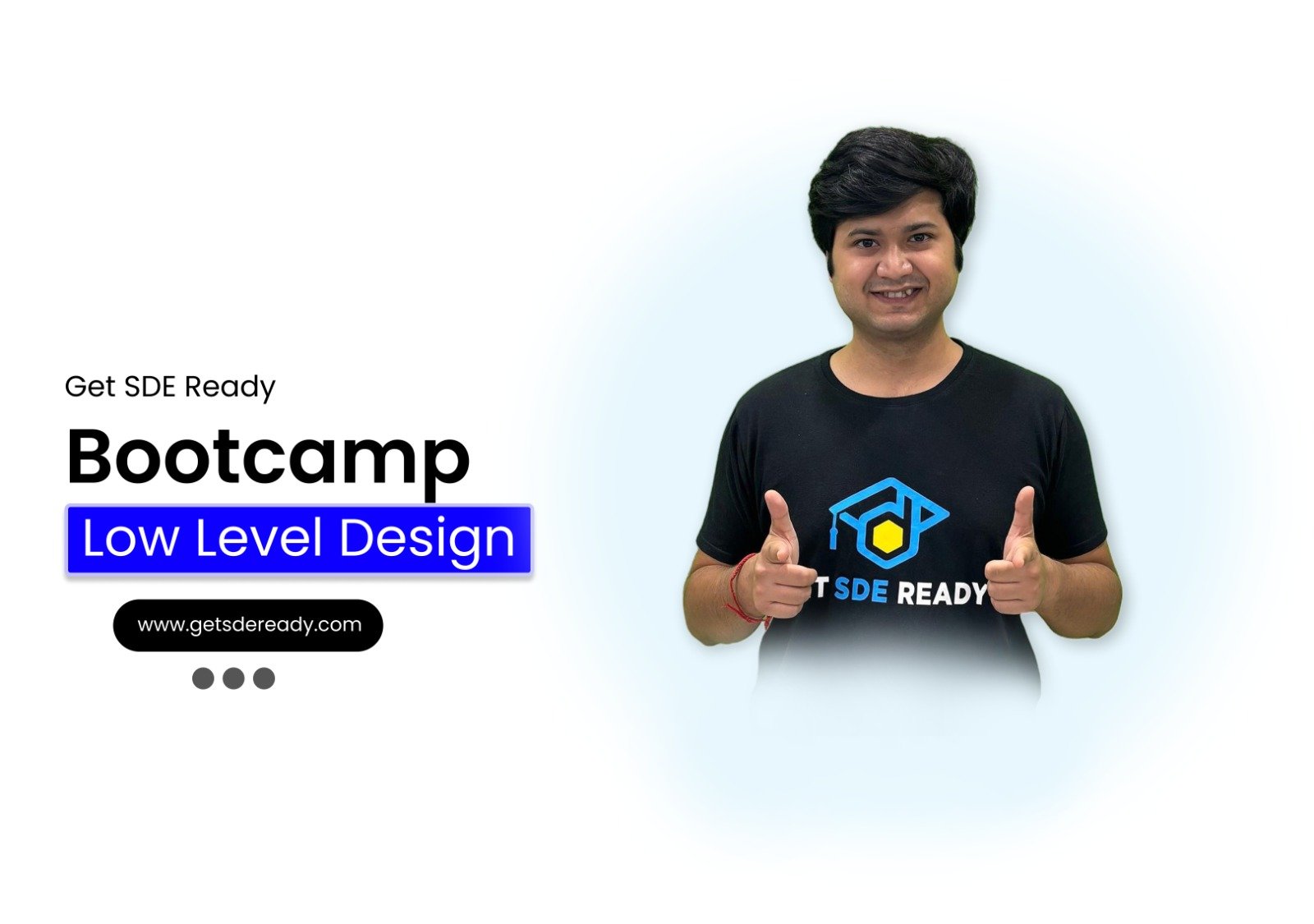
LLD Bootcamp
- 7+ Live Classes & Recordings
- Practice Questions
- 24/7 Live Doubt Support
- Case Studies
- Topic wise Quizzes
- Access to Global Peer Community
- Certificate of Completion
- Referrals
Buy for 50% OFF
₹2,000.00 ₹999.00
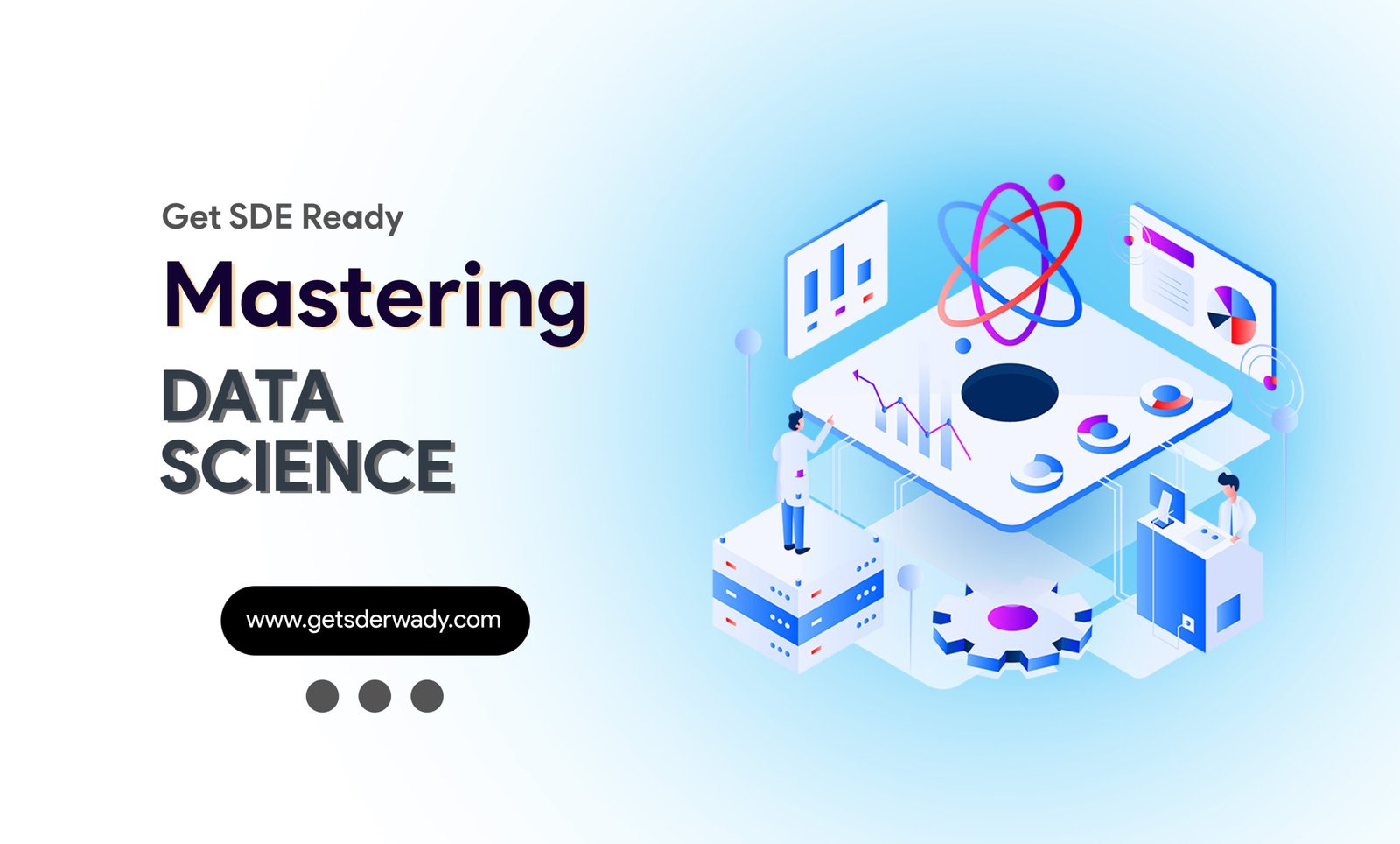
Essentials of Machine Learning and Artificial Intelligence
- 65+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 22+ Hands-on Live Projects & Deployments
- Comprehensive Notes
- Topic-wise Quizzes
- Case Studies
- Access to Global Peer Community
- Interview Prep Material
Buy for 65% OFF
₹20,000.00 ₹6,999.00
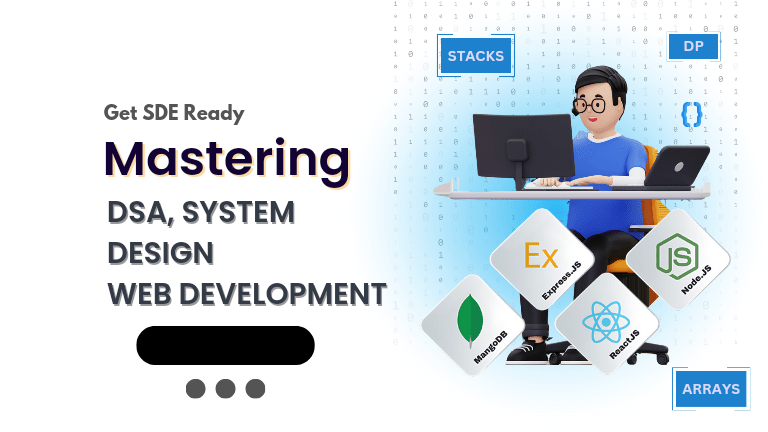
Fast-Track to Full Spectrum Software Engineering
- 120+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 400+ DSA Practice Questions
- Comprehensive Notes
- HackerRank Tests & Quizzes
- 12+ live Projects & Deployments
- Case Studies
- Access to Global Peer Community
Buy for 57% OFF
₹35,000.00 ₹14,999.00
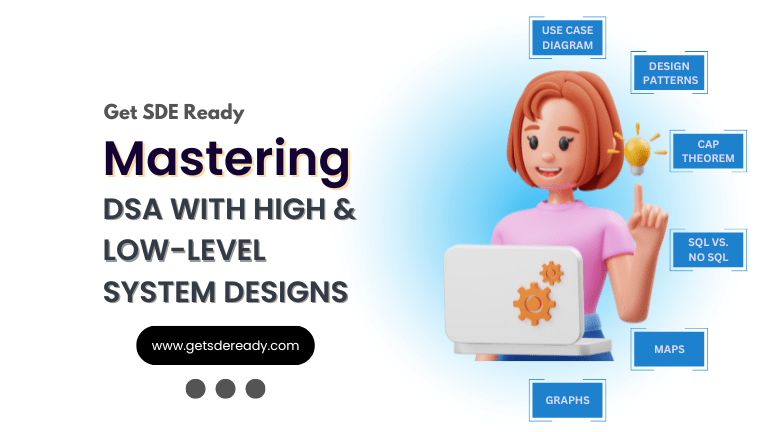
DSA, High & Low Level System Designs
- 85+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 400+ DSA Practice Questions
- Comprehensive Notes
- HackerRank Tests & Quizzes
- Topic-wise Quizzes
- Case Studies
- Access to Global Peer Community
Buy for 60% OFF
₹25,000.00 ₹9,999.00
Reach Out Now
If you have any queries, please fill out this form. We will surely reach out to you.
Contact Email
Reach us at the following email address.
Phone Number
You can reach us by phone as well.
+91-97737 28034
Our Location
Rohini, Sector-3, Delhi-110085