
Top 10 Essential SQL Queries for Web Development Interviews
SQL (Structured Query Language) is a cornerstone of web development, enabling developers to interact with relational databases efficiently. Mastering SQL queries is essential for cracking web development interviews, as it demonstrates your ability to retrieve, manipulate, and analyze data effectively. This comprehensive guide covers the top 10 SQL queries every aspiring web developer should know, with detailed explanations and practical examples.
1. Selecting Data from a Table
Basic SELECT Statement
The SELECT statement retrieves data from one or more tables. It is the foundation of SQL queries and forms the basis for more complex operations.
SELECT * FROM employees;
- Retrieves all columns and rows from the employees table.
- Use specific column names to improve performance and clarity.
Advantages of SELECT Queries
- Efficiently fetch data based on specific conditions.
- Supports aggregation and grouping operations.
Recommended Topic: Top 15 Frontend Interview Questions
Example Table: Employees
EmployeeID | Name | Department | Salary |
1 | Alice | IT | 50000 |
2 | Bob | HR | 45000 |
3 | Charlie | Marketing | 55000 |
2. Filtering Data with WHERE
Adding Conditions
The WHERE clause filters records based on specified conditions.
SELECT * FROM employees WHERE Department = ‘IT’;
- Retrieves only employees from the IT department.
- Supports operators like =, <, >, LIKE, and BETWEEN.
Benefits of Filtering Data
- Reduces the amount of data retrieved.
- Helps in analyzing specific subsets of information.
Comparison Operators
Operator | Description |
= | Equals |
<> | Not equals |
> | Greater than |
< | Less than |
3. Sorting Data with ORDER BY
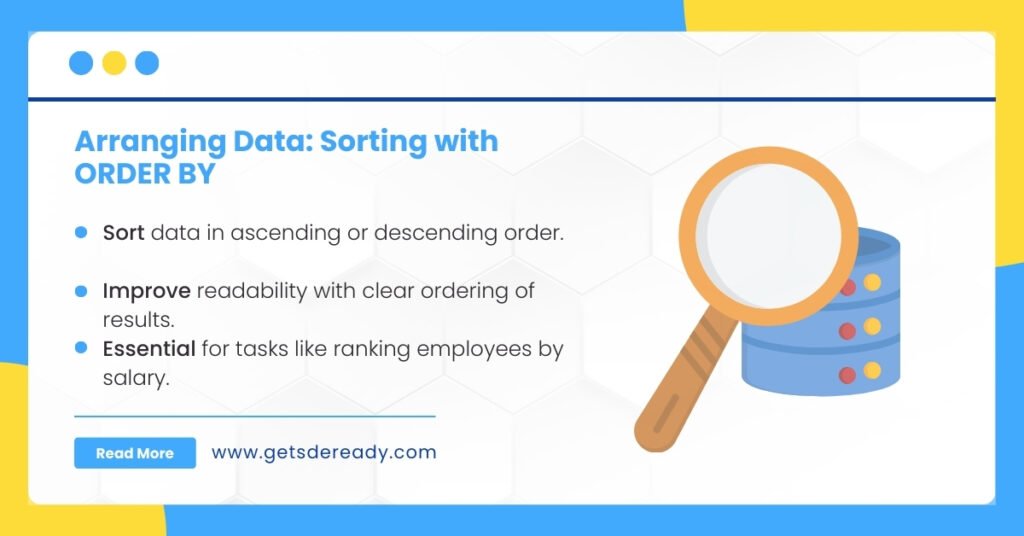
Sorting Results
ORDER BY arranges the results in ascending (default) or descending order.
SELECT Name, Salary FROM employees ORDER BY Salary DESC;
- Displays employee names and salaries in descending order of salary.
- Use ASC for ascending order.
Why Sorting Matters
- Facilitates better readability and analysis.
- Useful for ranked results like top-paid employees.
Recommended Topic: Top 10 Full-Stack Interview Questions
4. Aggregating Data with GROUP BY
Grouping and Summarizing
GROUP BY organizes data into groups and applies aggregate functions like SUM, AVG, COUNT, MIN, and MAX.
SELECT Department, AVG(Salary) AS AvgSalary FROM employees GROUP BY Department;
- Calculates the average salary for each department.
- Aggregate functions must be used with GROUP BY.
Applications of GROUP BY
- Summarizes data for reporting.
- Enables detailed insights into grouped metrics.
Department | AvgSalary |
IT | 50000 |
HR | 45000 |
Marketing | 55000 |
Recommended Topic: Top 20 API Design Interview Questions
5. Using Joins to Combine Data
Types of Joins
Joins combine rows from two or more tables based on related columns.
SELECT employees.Name, departments.DepartmentName FROM employees
JOIN departments ON employees.DepartmentID = departments.DepartmentID;
- INNER JOIN fetches matching rows from both tables.
- Other types include LEFT JOIN, RIGHT JOIN, and FULL OUTER JOIN.
Practical Use Cases
- Combines detailed information from multiple tables.
- Enhances relational database management.
Recommended Topic: Top 15 System Design Frameworks in 2024
6. Limiting Data with TOP or LIMIT
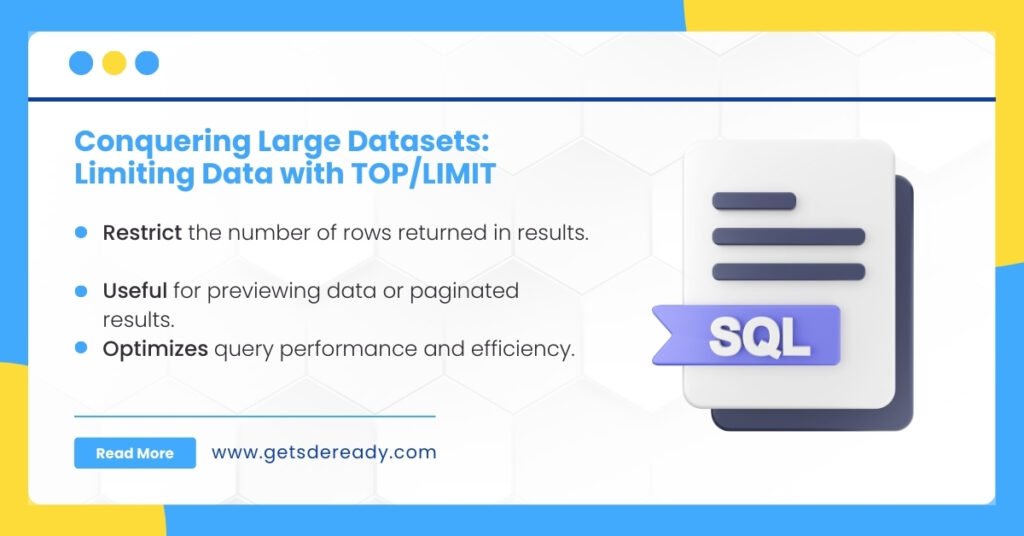
Limiting Results
TOP (SQL Server) or LIMIT (MySQL) restricts the number of rows returned.
SELECT * FROM employees LIMIT 5;
- Fetches only the top 5 rows from the table.
- Useful for paginated results or previews.
Benefits of Using Limits
- Optimizes query performance.
- Avoids overwhelming the user with excessive data.
EmployeeID | Name |
1 | Alice |
2 | Bob |
3 | Charlie |
Recommended Topic: Top 10 Agile Developer Questions
7. Inserting Data into Tables
Adding Records
The INSERT statement adds new rows to a table.
INSERT INTO employees (Name, Department, Salary)
VALUES (‘David’, ‘Finance’, 60000);
- Ensure proper data types and constraints.
- Bulk inserts improve efficiency.
Common Mistakes
- Mismatched column order.
- Violating primary key constraints.
8. Updating Data in a Table
Modifying Existing Records
The UPDATE statement changes data in a table.
UPDATE employees SET Salary = 52000 WHERE Name = ‘Alice’;
- Updates salary for Alice to 52000.
- Use WHERE carefully to avoid updating all rows.
Best Practices
- Test updates in a safe environment.
- Back up data before executing bulk updates.
Recommended Topic: Top 15 Python ML Interview Questions
9. Deleting Data from Tables
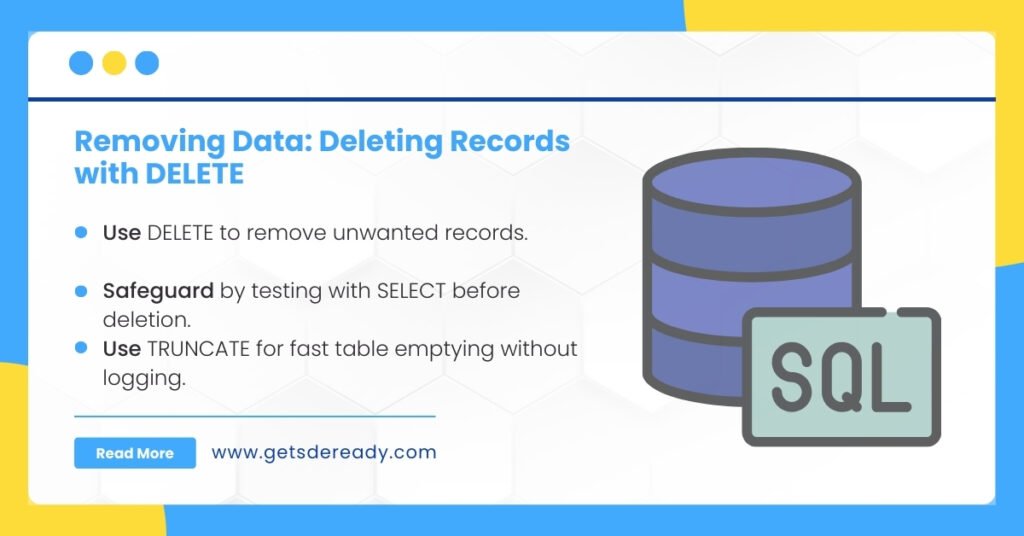
Removing Records
The DELETE statement removes rows from a table.
DELETE FROM employees WHERE Name = ‘David’;
- Deletes David’s record from the table.
- Use WHERE to specify which rows to delete.
Safeguards for DELETE
- Always test with SELECT before deletion.
- Use TRUNCATE for emptying a table without logging.
10. Using Subqueries
Querying Within Queries
Subqueries are nested queries used within a larger SQL statement.
SELECT Name FROM employees
WHERE Salary > (SELECT AVG(Salary) FROM employees);
- Finds employees earning above the average salary.
- Can be used in SELECT, WHERE, or FROM clauses.
Advantages of Subqueries
- Breaks complex problems into manageable parts.
- Enhances query readability and modularity.
Â
Employee | Salary |
Charlie | 55000 |
Frequently Asked Questions
What are the most important SQL queries to know for web development interviews?
Key SQL queries for web development interviews include SELECT, JOIN, GROUP BY, and INDEX queries. Mastering these will help you handle real-world data scenarios effectively.
Explore our Master DSA, Web Dev, and System Design course for a complete learning package.
How can I improve my SQL skills for interviews?
Practice solving real-world problems using SQL and focus on optimizing queries for performance. Developing structured solutions and understanding query execution plans can also help.
Our DSA and Web Development Combined course provides hands-on projects and structured guidance to enhance your SQL skills.
What are advanced SQL topics for interview preparation?
Advanced SQL topics for interviews include:
- Query optimization techniques.
- Window functions like ROW_NUMBER(), RANK(), and PARTITION BY.
- Complex subqueries for nested data retrieval.
Master these skills with hands-on learning through our Master SQL and System Design course.
Where can I find comprehensive SQL and web development learning resources?
You can find structured courses tailored to SQL and web development at Get SDE Ready. Our courses like Web Development or Master DSA and SQL are designed to build strong foundations and help you become interview-ready.
Is SQL necessary for data science roles?
Yes, SQL is a fundamental skill for data science as it allows you to query and manage large datasets effectively. SQL proficiency is often required to perform data analysis and preprocessing.
Check out our Data Science Course to master SQL alongside other essential skills for data science roles.
Accelerate your Path to a Product based Career
Boost your career or get hired at top product-based companies by joining our expertly crafted courses. Gain practical skills and real-world knowledge to help you succeed.
Reach Out Now
If you have any queries, please fill out this form. We will surely reach out to you.
Contact Email
Reach us at the following email address.
arun@getsdeready.com
Phone Number
You can reach us by phone as well.
+91-97737 28034
Our Location
Rohini, Sector-3, Delhi-110085