Top 10 Libraries and Frameworks for Python Developers
System Design Interview Questions
- Adobe System Design Interview Questions
- Top Atlassian System Design Interview Questions
- Top Amazon System Design Interview Questions
- Top Microsoft System Design Interview Questions
- Top Meta (Facebook) System Design Interview Questions
- Top Netflix System Design Interview Questions
- Top Uber System Design Interview Questions
- Top Google System Design Interview Questions
- Top Apple System Design Interview Questions
- Top Airbnb System Design Interview Questions
- Top 10 System Design Interview Questions
- Mobile App System Design Interview Questions
- Top 20 Stripe System Design Interview Questions
- Top Shopify System Design Interview Questions
- Top 20 System Design Interview Questions
- Top Advanced System Design Questions
- Most-Frequented System Design Questions in Big Tech Interviews
- What Interviewers Look for in System Design Questions
- Critical System Design Questions to Crack Any Tech Interview
- Top 20 API Design Questions for System Design Interviews
- Top 10 Steps to Create a System Design Portfolio for Developers
Introduction to High-Level System Design
System Design Fundamentals
- Functional vs. Non-Functional Requirements
- Scalability, Availability, and Reliability
- Latency and Throughput Considerations
- Load Balancing Strategies
Architectural Patterns
- Monolithic vs. Microservices Architecture
- Layered Architecture
- Event-Driven Architecture
- Serverless Architecture
- Model-View-Controller (MVC) Pattern
- CQRS (Command Query Responsibility Segregation)
Scaling Strategies
- Vertical Scaling vs. Horizontal Scaling
- Sharding and Partitioning
- Data Replication and Consistency Models
- Load Balancing Strategies
- CDN and Edge Computing
Database Design in HLD
- SQL vs. NoSQL Databases
- CAP Theorem and its Impact on System Design
- Database Indexing and Query Optimization
- Database Sharding and Partitioning
- Replication Strategies
API Design and Communication
Caching Strategies
- Types of Caching
- Cache Invalidation Strategies
- Redis vs. Memcached
- Cache-Aside, Write-Through, and Write-Behind Strategies
Message Queues and Event-Driven Systems
- Kafka vs. RabbitMQ vs. SQS
- Pub-Sub vs. Point-to-Point Messaging
- Handling Asynchronous Workloads
- Eventual Consistency in Distributed Systems
Security in System Design
Observability and Monitoring
- Logging Strategies (ELK Stack, Prometheus, Grafana)
- API Security Best Practices
- Secure Data Storage and Access Control
- DDoS Protection and Rate Limiting
Real-World System Design Case Studies
- Distributed locking (Locking and its Types)
- Memory leaks and Out of memory issues
- HLD of YouTube
- HLD of WhatsApp
Python is one of the most versatile programming languages, and its popularity continues to grow. Whether you’re a beginner or an experienced developer, having the right tools can make all the difference. To help you stay ahead in your coding journey, we’re offering a free course on mastering Python libraries and frameworks! Simply sign up here to get access to the latest course updates. In this article, we’ll explore the top 10 libraries and frameworks that every Python developer should know.
1. NumPy
What is NumPy?
NumPy, short for Numerical Python, is a fundamental library for scientific computing in Python. It provides support for arrays, matrices, and many mathematical functions.
Key Features
- Efficient Array Operations: Fast operations on large datasets using the ndarray object.
- Broadcasting: Enables arithmetic operations on arrays of different shapes.
- Integration with Other Libraries: Works seamlessly with libraries like SciPy and Pandas.
Use Cases
- Data analysis
- Machine learning
- Scientific research
Example
import numpy as np Â
array = np.array([1, 2, 3])Â Â
print(array * 2)Â
If you’re preparing for interviews, you might find our guide on Top 10 Frontend Design Questions helpful for understanding client-side design principles.
2. Pandas
What is Pandas?
Pandas is a powerful library for data manipulation and analysis. It provides data structures like DataFrames and Series, making it easier to handle structured data.
Key Features
- Data Cleaning: Handles missing data and duplicates efficiently.
- Data Aggregation: Perform complex operations like grouping and pivoting.
- Time Series Analysis: Built-in support for time-based data.
Use Cases
- Data preprocessing
- Financial analysis
- Business intelligence
Example
import pandas as pd Â
data = {‘Name’: [‘Alice’, ‘Bob’], ‘Age’: [25, 30]}Â Â
df = pd.DataFrame(data)Â Â
print(df)Â Â
If you’re exploring scalability further, check out our Top 10 Distributed System Design Challenges for a detailed breakdown of common issues and solutions.
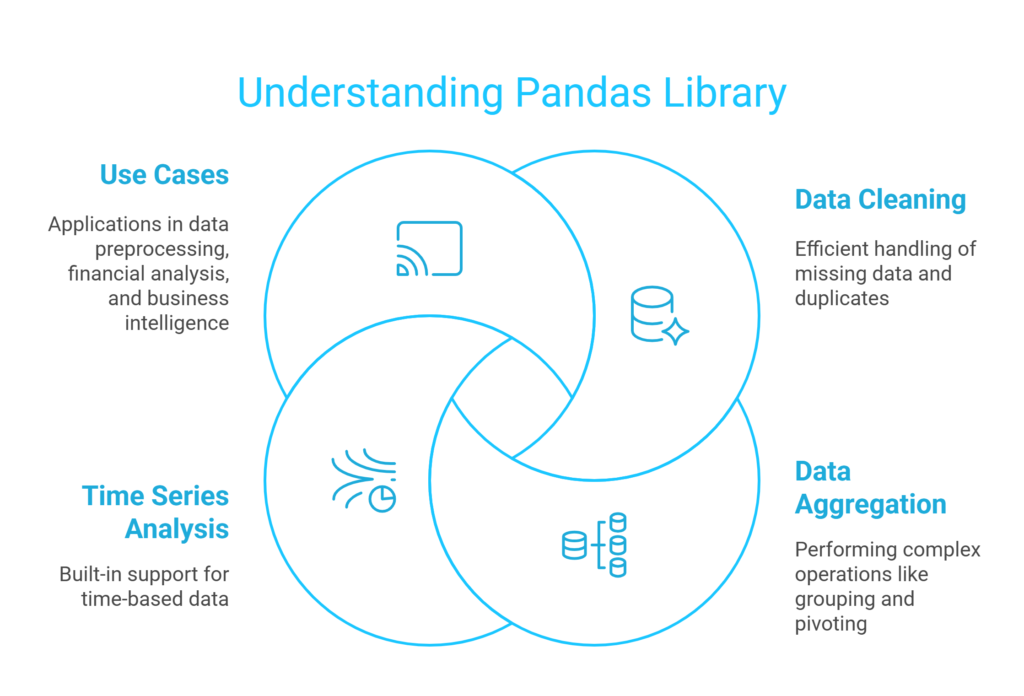
3. TensorFlow
What is TensorFlow?
TensorFlow is an open-source library developed by Google for machine learning and deep learning applications.
Key Features
- Scalability: Runs on CPUs, GPUs, and TPUs.
- Flexibility: Supports both high-level and low-level APIs.
- Community Support: Extensive documentation and tutorials.
Use Cases
- Image recognition
- Natural language processing
- Predictive analytics
Example
import tensorflow as tf Â
model = tf.keras.Sequential([tf.keras.layers.Dense(units=1, input_shape=[1])])Â Â
model.compile(optimizer=’sgd’, loss=’mean_squared_error’)Â Â
For a broader understanding of performance optimization, our Top 10 Cloud App Design Questions covers strategies for reducing latency in distributed systems.
4. Flask
What is Flask?
Flask is a lightweight web framework for Python, ideal for building small to medium-sized web applications.
Key Features
- Simplicity: Easy to set up and use.
- Extensibility: Supports a wide range of extensions.
- Flexibility: Allows developers to choose their tools and libraries.
Use Cases
- RESTful APIs
- Microservices
- Prototyping
Example
from flask import Flask Â
app = Flask(__name__)Â Â
@app.route(‘/’)Â Â
def home():Â Â
    return “Hello, World!” Â
If you’re preparing for technical interviews, our Top 10 SQL Queries for Web Dev Interviews can help you master database-related questions.
5. Django
What is Django?
Django is a high-level web framework that encourages rapid development and clean, pragmatic design.
Key Features
- Batteries Included: Comes with built-in features like authentication and ORM.
- Scalability: Suitable for large-scale applications.
- Security: Protects against common vulnerabilities like SQL injection.
Use Cases
- E-commerce platforms
- Content management systems
- Social networks
Example
from django.http import HttpResponse Â
def home(request):Â Â
return HttpResponse(“Welcome to Django!”)Â Â
For a deeper dive into data structures, our Top 15 DSA Questions on Arrays & Strings is a great resource to strengthen your fundamentals.

6. Scikit-learn
What is Scikit-learn?
Scikit-learn is a library for machine learning in Python, built on NumPy, SciPy, and Matplotlib.
Key Features
- Algorithms: Offers a wide range of supervised and unsupervised learning algorithms.
- Model Evaluation: Tools for cross-validation and metrics.
- Preprocessing: Functions for scaling and encoding data.
Use Cases
- Predictive modeling
- Clustering
- Dimensionality reduction
Example
from sklearn.linear_model import LinearRegression Â
model = LinearRegression()Â Â
model.fit(X_train, y_train)Â Â
If you’re interested in advanced security topics, our Cracking Tech Giants’ Design Rounds guide covers encryption and other critical design aspects.
7. Matplotlib
What is Matplotlib?
Matplotlib is a plotting library for creating static, animated, and interactive visualizations in Python.
For more on authentication and authorization, our Instagram: Low-Level Design Guide provides practical insights into implementing secure systems.
Key Features
- Customization: Highly customizable plots.
- Integration: Works well with Pandas and NumPy.
- Variety: Supports line plots, bar charts, histograms, and more.
Use Cases
- Data visualization
- Scientific research
- Financial analysis
Example
import matplotlib.pyplot as plt Â
plt.plot([1, 2, 3], [4, 5, 1])Â Â
plt.show()Â Â
Comparison Table
Library/Framework | Use Case | Key Feature |
NumPy | Scientific Computing | Efficient Array Operations |
Pandas | Data Analysis | Data Cleaning |
TensorFlow | Machine Learning | Scalability |
Flask | Web Development | Simplicity |
Django | Web Development | Batteries Included |
Scikit-learn | Machine Learning | Algorithms |
Matplotlib | Data Visualization | Customization |
PyTorch | Deep Learning | Dynamic Graphs |
FastAPI | API Development | Performance |
Keras | Deep Learning | User-Friendly |

10. Repl.it
What is Repl.it?
Repl.it is an AI-powered online coding environment that supports multiple programming languages.
How Does It Help Developers?
- Online Coding: Allows you to write and run code directly in your browser.
- Collaboration: Offers features for real-time collaboration, making it easier to work on shared projects.
- Learning: Provides a platform for learning new programming languages and technologies.
Key Features
- Multi-Language Support: Supports over 50 programming languages.
- Integration: Integrates with popular version control systems like GitHub.
- Templates: Offers templates for quick project setup.
For those interested in real-time systems, our guide on System Design for Real-Time Chat Apps provides valuable insights.
What is the best Python library for data analysis?
Pandas is the go-to library for data analysis in Python. It provides powerful tools for data manipulation, cleaning, and aggregation, making it ideal for handling structured data. To further enhance your skills, explore our Data Science Course.
Which framework should I choose for web development: Flask or Django?
Flask is better for small to medium-sized projects due to its simplicity and flexibility, while Django is ideal for large-scale applications with built-in features. Learn more through our Web Development Course.
How do I choose between TensorFlow and PyTorch?
TensorFlow is great for production-level applications, while PyTorch is preferred for research and experimentation. If you’re serious about mastering deep learning, check out our Master DSA, Web Dev & System Design Course.
What are the best resources to prepare for DSA interviews?
Mastering DSA is crucial for coding interviews. We recommend our DSA Course, which covers all fundamental and advanced concepts to help you ace your interviews.
How can I become proficient in system design?
System design is essential for scaling applications. Our Design & DSA Combined Course provides in-depth insights into system architecture, best practices, and real-world applications.
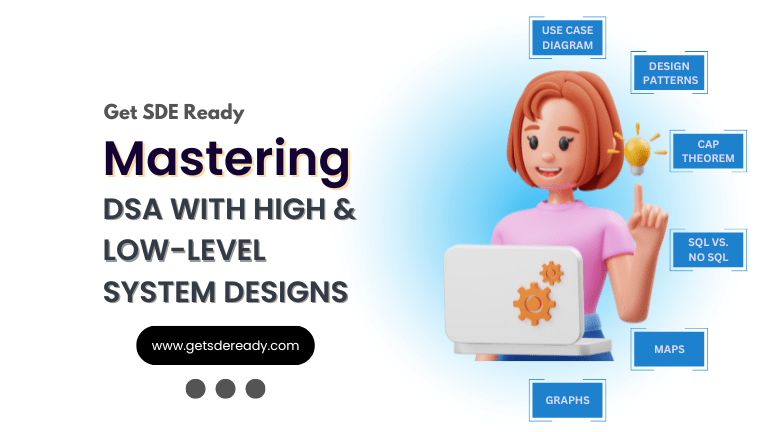
DSA, High & Low Level System Designs
- 85+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 400+ DSA Practice Questions
- Comprehensive Notes
- HackerRank Tests & Quizzes
- Topic-wise Quizzes
- Case Studies
- Access to Global Peer Community
Buy for 60% OFF
₹25,000.00 ₹9,999.00
Accelerate your Path to a Product based Career
Boost your career or get hired at top product-based companies by joining our expertly crafted courses. Gain practical skills and real-world knowledge to help you succeed.

ML & AI Kickstart
- Live Classes & Recordings
- 24/7 Live Doubt Support
- Practice Questions
- Case Studies
- Topic wise Quizzes
- Access to Global Peer Community
- Cartificate of Completion
- Referrals
Buy for 65% OFF
₹1,700.00 ₹999.00
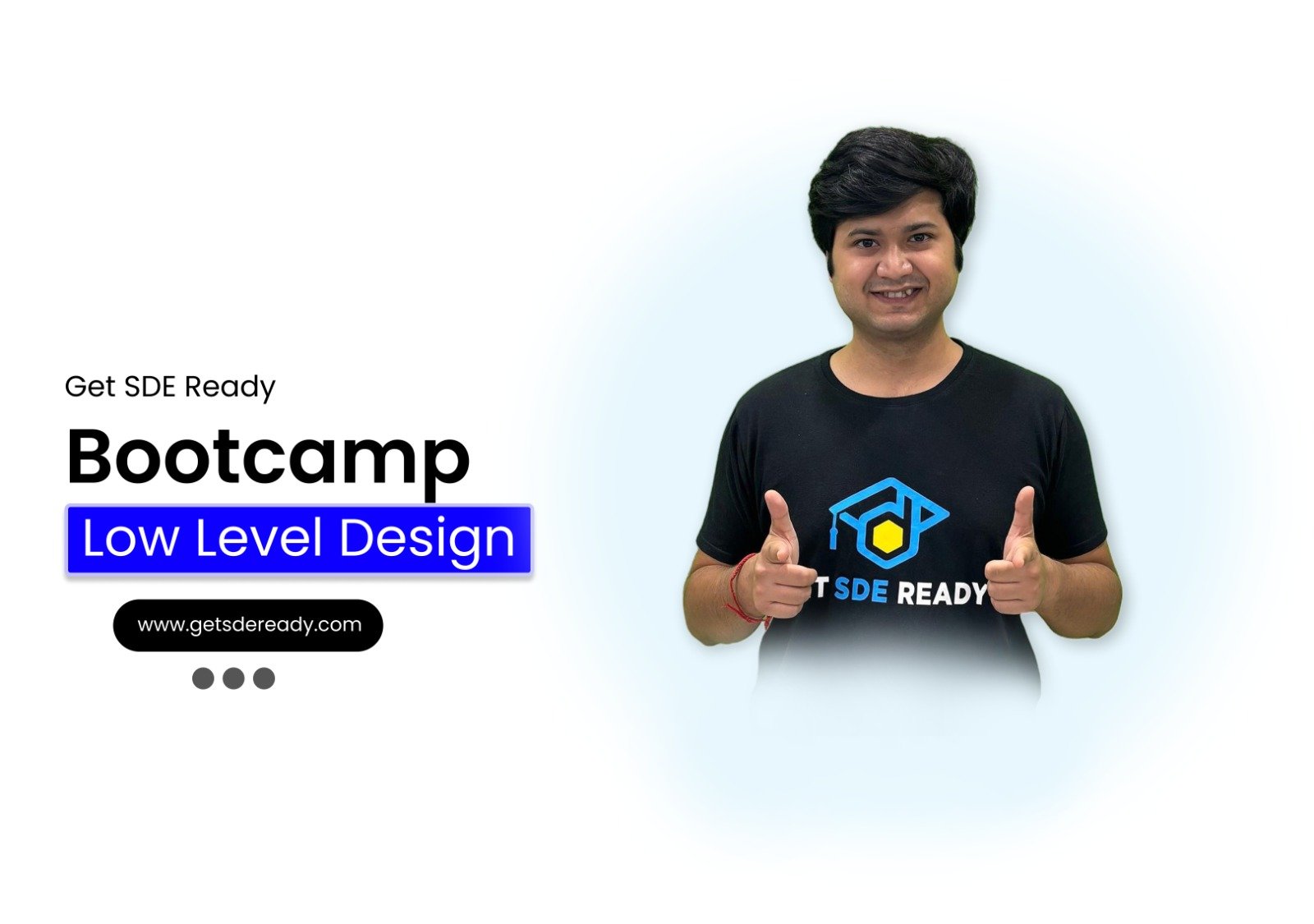
LLD Bootcamp
- 7+ Live Classes & Recordings
- Practice Questions
- 24/7 Live Doubt Support
- Case Studies
- Topic wise Quizzes
- Access to Global Peer Community
- Cartificate of Completion
- Referrals
Buy for 65% OFF
₹1,700.00 ₹999.00
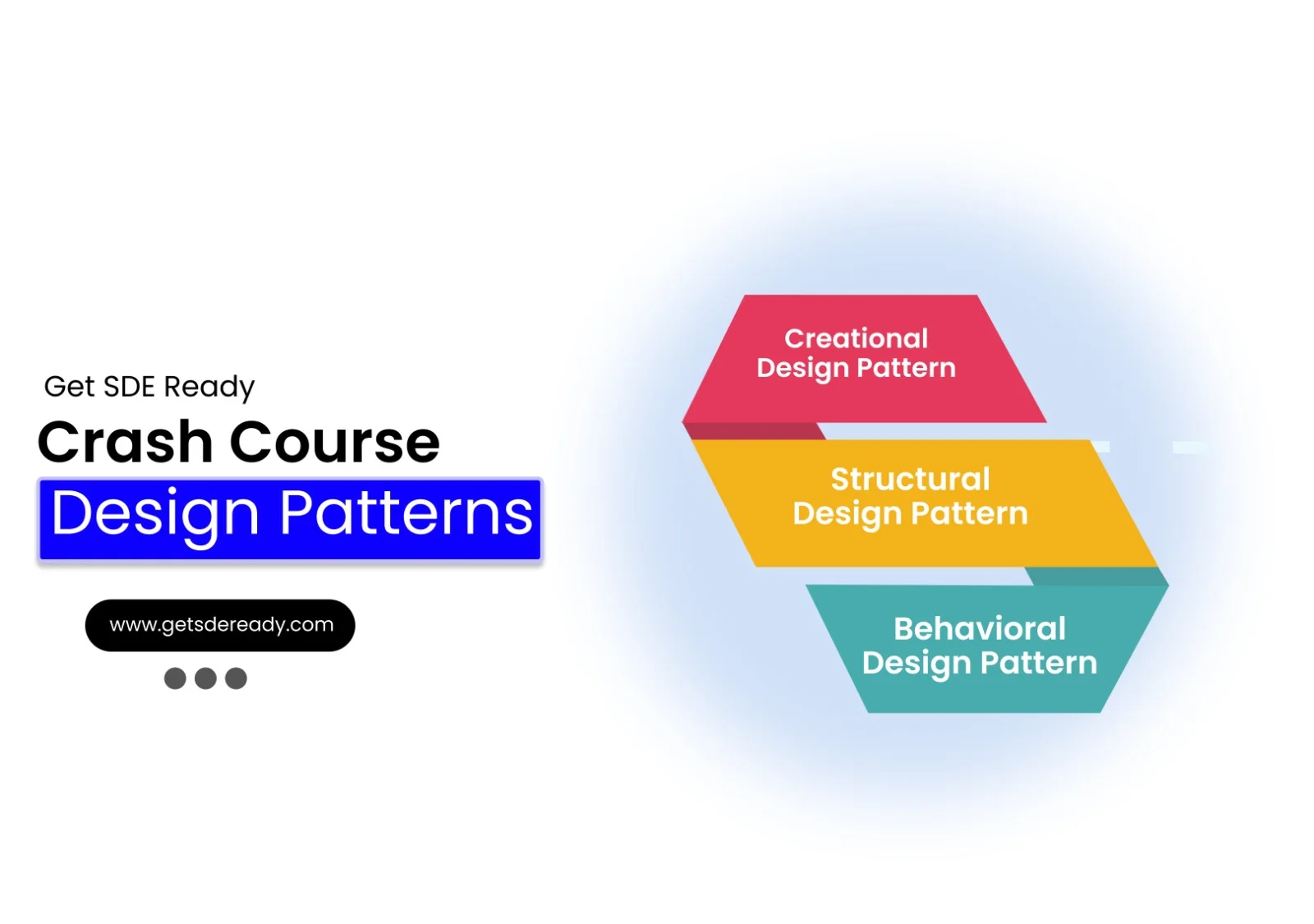
Design Patterns Bootcamp
- Live Classes & Recordings
- 24/7 Live Doubt Support
- Practice Questions
- Case Studies
- Access to Global Peer Community
- Topic wise Quizzes
- Referrals
- Cartificate of Completion
Buy for 65% OFF
₹1,700.00 ₹999.00
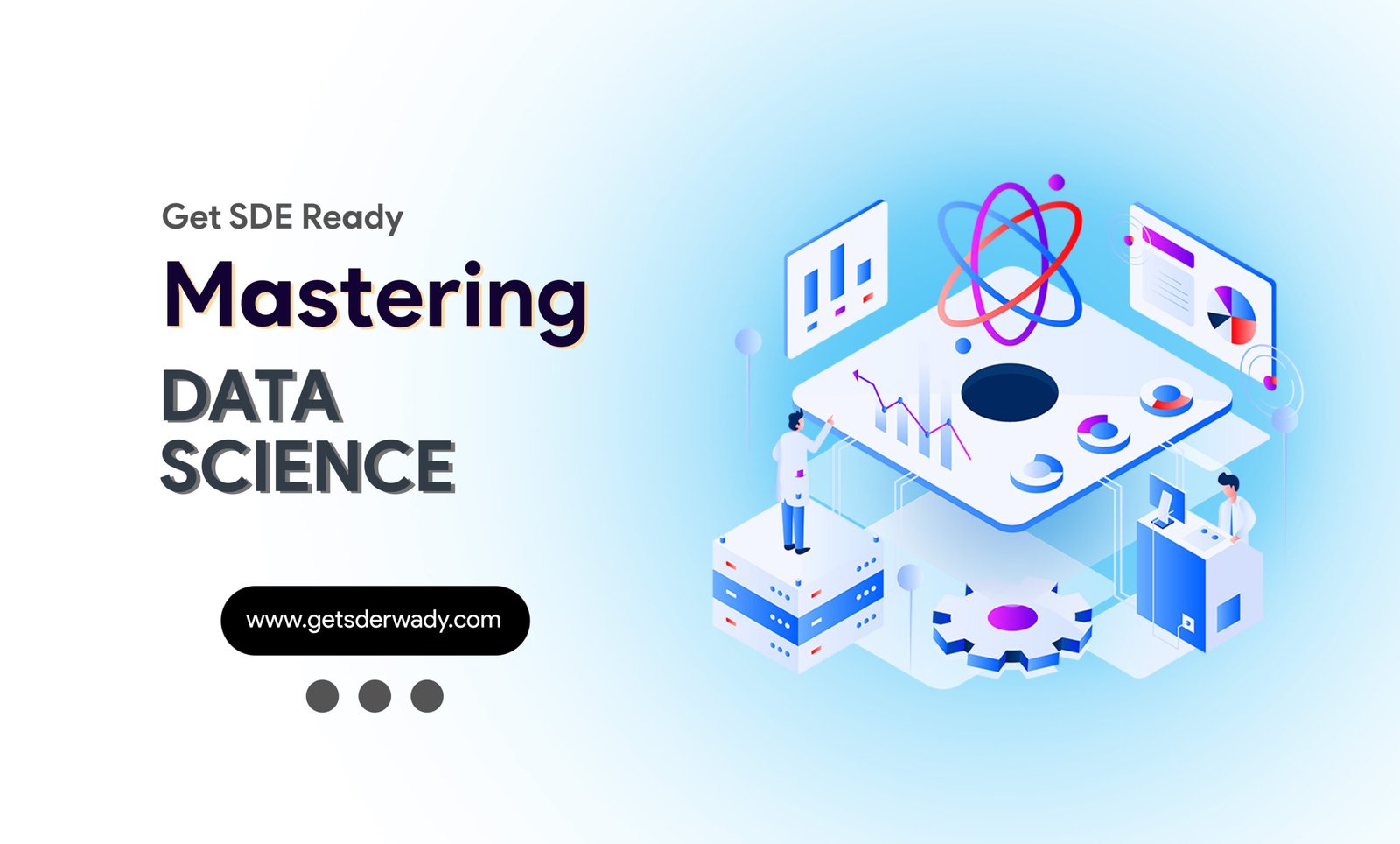
Essentials of Machine Learning and Artificial Intelligence
- 65+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 22+ Hands-on Live Projects & Deployments
- Comprehensive Notes
- Topic-wise Quizzes
- Case Studies
- Access to Global Peer Community
- Interview Prep Material
Buy for 65% OFF
₹20,000.00 ₹6,999.00
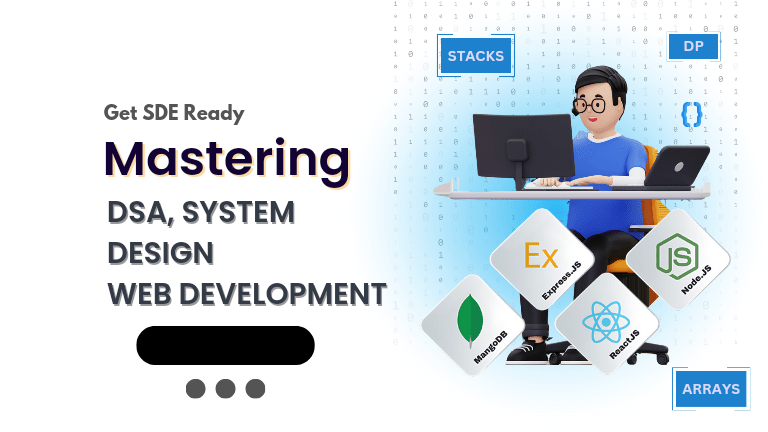
Fast-Track to Full Spectrum Software Engineering
- 120+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 400+ DSA Practice Questions
- Comprehensive Notes
- HackerRank Tests & Quizzes
- 12+ live Projects & Deployments
- Case Studies
- Access to Global Peer Community
Buy for 57% OFF
₹35,000.00 ₹14,999.00
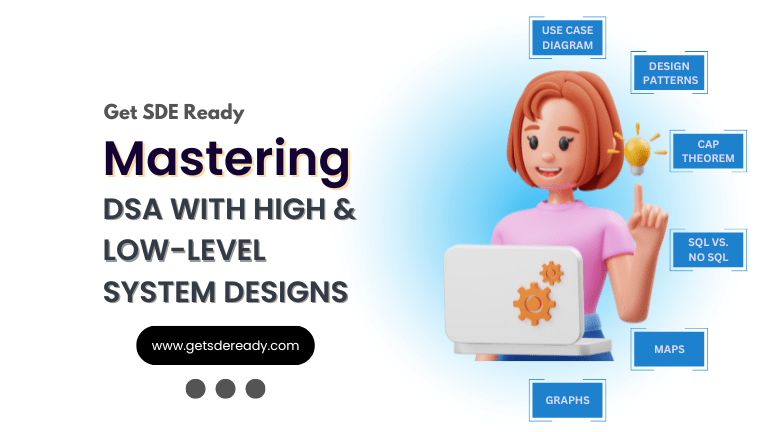
DSA, High & Low Level System Designs
- 85+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 400+ DSA Practice Questions
- Comprehensive Notes
- HackerRank Tests & Quizzes
- Topic-wise Quizzes
- Case Studies
- Access to Global Peer Community
Buy for 60% OFF
₹25,000.00 ₹9,999.00
Reach Out Now
If you have any queries, please fill out this form. We will surely reach out to you.
Contact Email
Reach us at the following email address.
Phone Number
You can reach us by phone as well.
+91-97737 28034
Our Location
Rohini, Sector-3, Delhi-110085