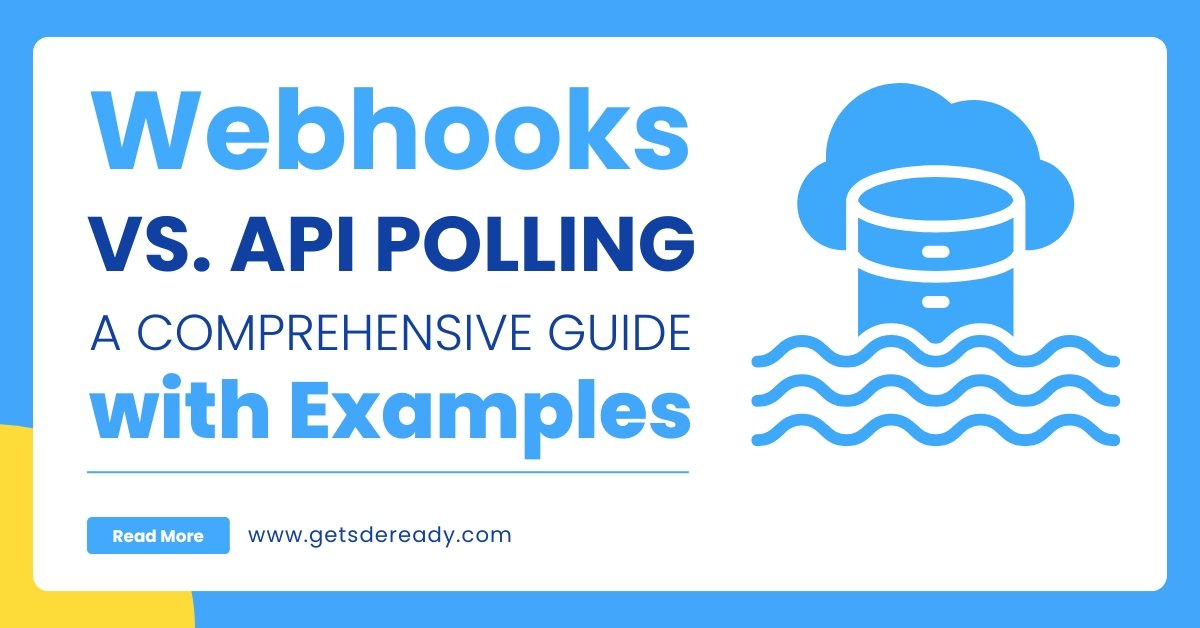
Top 15 DSA Questions Using Arrays and Strings for Coding Interviews
Data Structures and Algorithms (DSA) are essential for coding interviews in software development, as they form the backbone of problem-solving skills. Arrays and strings are two of the most frequently used data structures in coding challenges. In this article, we will explore the Top 15 DSA Questions Using Arrays and Strings for Coding Interviews to help you prepare for your next technical interview.
1. Two Sum
Problem Overview:
The Two Sum problem asks you to find two numbers in an array that add up to a specific target sum. It’s a classic problem frequently asked in coding interviews.
Solution Strategy:
This problem can be efficiently solved using a hash map to store the differences between the target sum and the array elements.
Approach:
- Loop through the array while checking if the target sum minus the current number exists in the hash map.
- If it does, you’ve found the pair; otherwise, store the number in the hash map.
Â
Complexity:
- Time complexity: O(n)
- Space complexity: O(n)
Â
Example: Given the array [2, 7, 11, 15] and a target of 9, the solution would return indices 0 and 1.
Also Read: Top 15 System Design Frameworks in 2024
2. Reverse a String

Problem Overview:
Reversing a string is a simple yet common problem in coding interviews. It’s often used to test your knowledge of basic string manipulation and algorithmic thinking.
Solution Strategy:
To reverse a string, you can use two pointers—one at the start of the string and the other at the end—swapping characters as you move towards the center.
Approach:
- Initialize two pointers, one at the beginning and one at the end of the string.
- Swap the characters at these pointers, and move the pointers towards each other until they meet.
Complexity:
- Time complexity: O(n)
- Space complexity: O(1)
Example: For the input “hello”, the output would be “olleh”.
Also Read: Common React Interview Questions
3. Merge Sorted Arrays
Problem Overview:
Given two sorted arrays, the task is to merge them into a single sorted array. This problem is a great test of your understanding of merge operations and arrays.
Solution Strategy:
- Initialize two pointers for the two arrays.
- Compare elements from both arrays, and append the smaller one to the result array. Continue until all elements are processed.
Approach:
- Use a loop to iterate through both arrays, and compare their current elements.
- Append the smaller element to the result array, and increment the pointer.
Complexity:
- Time complexity: O(n + m), where n and m are the lengths of the two arrays.
- Space complexity: O(n + m)
Example: Given arrays [1, 3, 5] and [2, 4, 6], the merged result would be [1, 2, 3, 4, 5, 6].
Also Read: Top 10 Google Software Engineering Questions
4. Longest Substring Without Repeating Characters
Problem Overview:
This problem asks you to find the longest substring in a string that doesn’t contain any repeating characters. It’s a common problem to test sliding window techniques.
Solution Strategy:
- Use a sliding window technique with two pointers to keep track of the substring and its unique characters.
Approach:
- Initialize two pointers and a hash map to store the last index of each character.
- Move the second pointer to expand the window and update the hash map.
- When a duplicate character is found, move the first pointer to the right of the duplicate.
Complexity:
- Time complexity: O(n)
- Space complexity: O(min(n, m)), where n is the length of the string and m is the character set size.
Example: For the string “abcabcbb”, the longest substring without repeating characters is “abc”.
Also Read: 10 Steps for a System Design Portfolio
5. Find the Missing Number in an Array
Problem Overview:
In an array of n numbers where the numbers are from 1 to n+1, find the missing number. This problem can be solved efficiently without sorting.
Solution Strategy:
- Use the mathematical property of sum of first n numbers to calculate the missing number.
Approach:
- Compute the expected sum of numbers from 1 to n+1.
- Subtract the sum of the elements in the array from this expected sum.
Complexity:
- Time complexity: O(n)
- Space complexity: O(1)
Example: Given the array [1, 2, 4, 5, 6], the missing number is 3.
Also Read: Top 20 API Design Interview Questions
6. Rotate an Array
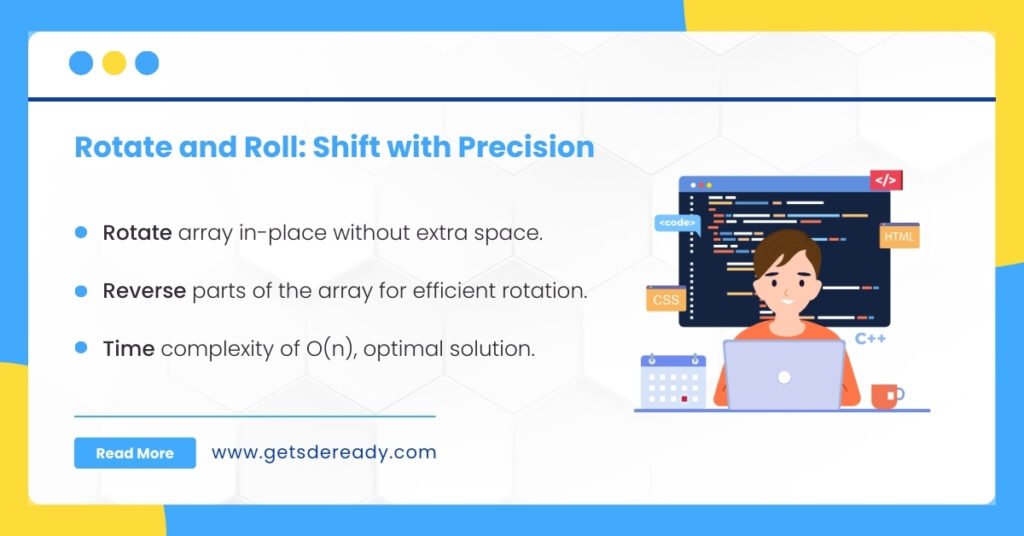
Problem Overview:
The task is to rotate an array to the right by k steps. This problem is commonly asked in interviews to assess array manipulation skills.
Solution Strategy:
- Reverse parts of the array to rotate it without using extra space.
Approach:
- Reverse the entire array.
- Reverse the first k elements.
- Reverse the remaining n-k elements.
Complexity:
- Time complexity: O(n)
- Space complexity: O(1)
Example: For the array [1, 2, 3, 4, 5] and k = 2, the rotated array will be [4, 5, 1, 2, 3].
7. Valid Anagram
Problem Overview:
Check whether two strings are anagrams of each other. This problem tests your ability to manipulate strings efficiently.
Solution Strategy:
- Sort both strings and compare them, or use a hash map to count the frequency of characters.
Approach:
- Sort both strings and check if they are equal.
- Alternatively, use a hash map to count the characters in both strings.
Complexity:
- Time complexity: O(n log n) for sorting or O(n) for using a hash map.
- Space complexity: O(n) for hash map approach.
Example: For the strings “anagram” and “nagaram”, they are anagrams.
Also Read: Top 20 Software Frameworks for 2025
8. Longest Palindromic Substring
Problem Overview:
This problem requires finding the longest palindromic substring in a given string. A palindrome reads the same forward and backward.
Solution Strategy:
- Use dynamic programming or expand around center approach to find the longest palindrome.
Approach:
- For each character in the string, expand around it as a potential center of the palindrome.
- Track the longest palindrome encountered.
Complexity:
- Time complexity: O(n^2)
- Space complexity: O(1) for the expanding center approach.
Example: For the string “babad”, the longest palindromic substring is “bab” or “aba”.
9. Rotate String
Problem Overview:
Given two strings, check if one is a rotation of the other. This problem helps in understanding string manipulations and transformations.
Solution Strategy:
- Concatenate one string with itself and check if the other string exists in it.
Approach:
- Concatenate the first string with itself.
- Check if the second string is a substring of the concatenated string.
Complexity:
- Time complexity: O(n)
- Space complexity: O(n)
Example: For strings “abcde” and “cdeab”, the second string is a rotation of the first.
10. Subarray Sum Equals K
Problem Overview:
Given an array of integers, find the number of contiguous subarrays that sum up to a given target k. This problem tests the ability to handle subarray sum queries efficiently.
Solution Strategy:
- Use a hash map to track the cumulative sum of elements and find subarrays that sum up to k.
Approach:
- Use a hash map to store the cumulative sum and check if the target sum can be formed.
Complexity:
- Time complexity: O(n)
- Space complexity: O(n)
Example: For the array [1, 2, 3] and k = 3, there is one subarray [1, 2] that sums to 3.
11. Find Duplicate in an Array
Problem Overview:
Given an array where each element appears once except for one element that appears twice, find the duplicate element.
Solution Strategy:
- Use a hash set to store elements and detect duplicates.
Approach:
- Traverse the array, and for each element, check if it already exists in the hash set.
Complexity:
- Time complexity: O(n)
- Space complexity: O(n)
Example: For the array [1, 3, 4, 2, 2], the duplicate element is 2.
Also Read: Top 15 Frontend Interview Questions
12. Move Zeroes
Problem Overview:
Move all zeroes in an array to the end without changing the relative order of non-zero elements. This problem evaluates array manipulation and in-place operations.
Solution Strategy:
- Use two pointers to rearrange the array in-place.
Approach:
- Initialize a pointer for non-zero elements and iterate through the array to move zeroes to the end.
Complexity:
- Time complexity: O(n)
- Space complexity: O(1)
Example: For the array [0, 1, 0, 3, 12], the array after moving zeroes will be [1, 3, 12, 0, 0].
13. Spiral Matrix
Problem Overview:
Given a matrix, print the elements in a spiral order. This problem is commonly used to assess knowledge of matrix traversal.
Solution Strategy:
- Use four pointers (top, bottom, left, right) to traverse the matrix in a spiral order.
Approach:
- Traverse the matrix in layers, moving from left to right, top to bottom, right to left, and bottom to top.
Complexity:
- Time complexity: O(m * n)
- Space complexity: O(1)
Example: For the matrix:
Â
1Â 2Â 3
4Â 5Â 6
7Â 8Â 9
Â
The spiral order will be [1, 2, 3, 6, 9, 8, 7, 4, 5].
Â
14. Set Matrix Zeroes
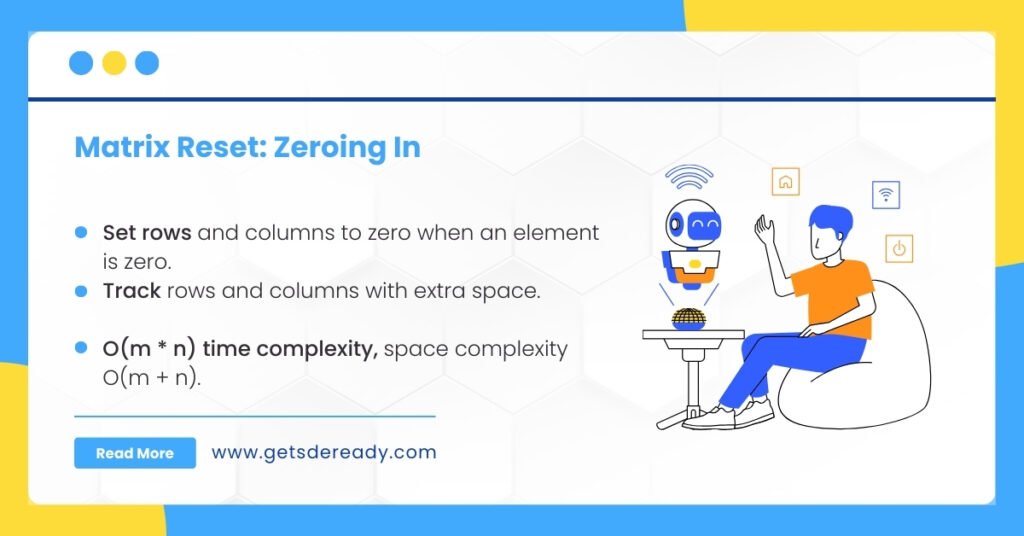
Problem Overview:
Given a matrix, set the entire row and column to zero if any element in the matrix is zero.
Solution Strategy:
- Use extra space to record the rows and columns that should be set to zero.
Approach:
- Traverse the matrix and mark the rows and columns that need to be set to zero.
- Set the identified rows and columns to zero.
Complexity:
- Time complexity: O(m * n)
- Space complexity: O(m + n)
Example: For the matrix:
Â
1Â 0Â 3
4Â 5Â 6
7Â 8Â 9
Â
After setting rows and columns to zero, the matrix will be:
Â
0Â 0Â 0
4Â 0Â 6
7Â 0Â 9
Â
15. Container With Most Water
Problem Overview:
This problem involves finding the two lines that, together with the x-axis, form a container that holds the most water. This problem is often used to test the understanding of two-pointer techniques.
Solution Strategy:
- Use two pointers at the ends of the array and move towards the center.
Approach:
- Calculate the area between the lines at the two pointers and update the maximum area found.
Complexity:
- Time complexity: O(n)
- Space complexity: O(1)
Example: For the array [1, 8, 6, 2, 5, 4, 8, 3, 7], the maximum area is 49.
FAQ
What are the best strategies to solve DSA problems?
The best strategies for solving DSA problems include understanding the problem constraints, breaking the problem into smaller subproblems, and practicing common techniques like recursion, dynamic programming, and greedy algorithms. Practice and consistency are key to mastering DSA concepts.
How can I improve my understanding of arrays and strings?
Improving your understanding of arrays and strings can be done through consistent practice and learning various techniques, such as sliding window, two-pointer approach, and sorting. Implementing algorithms that involve arrays and strings will help solidify your knowledge.
How do I prepare for DSA interviews?
To prepare for DSA interviews, focus on mastering the fundamental data structures like arrays, linked lists, trees, and graphs. Solve problems on platforms like LeetCode, HackerRank, and Codeforces, and work on time and space optimization techniques.
Accelerate your Path to a Product based Career
Boost your career or get hired at top product-based companies by joining our expertly crafted courses. Gain practical skills and real-world knowledge to help you succeed.
Reach Out Now
If you have any queries, please fill out this form. We will surely reach out to you.
Contact Email
Reach us at the following email address.
Phone Number
You can reach us by phone as well.
+91-97737 28034
Our Location
Rohini, Sector-3, Delhi-110085