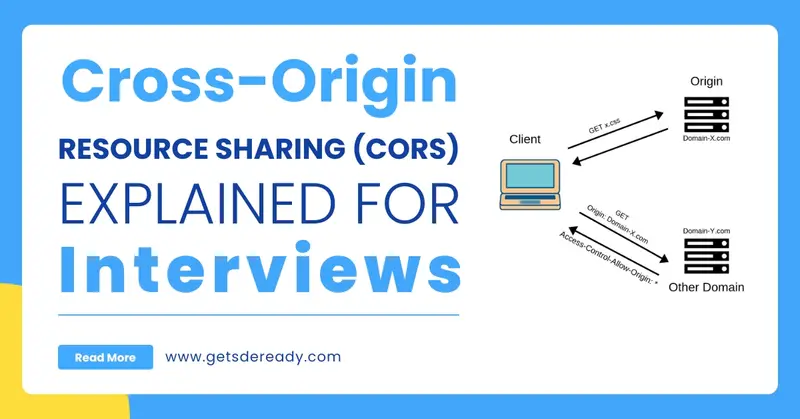
Top 15 Low-Level Design Questions Asked in Facebook Interviews
In tech interviews, low-level design (LLD) questions are essential for evaluating a candidate’s problem-solving and analytical abilities. Facebook, one of the biggest tech giants, is known for its challenging interview process. A candidate must be well-prepared to tackle LLD questions as these focus on how well you can design components, data structures, and algorithms at a granular level.
In this blog post, we’ll explore the top 15 low-level design questions commonly asked in Facebook interviews. Understanding these questions and how to approach them will help you excel in your interview preparation. Let’s dive in!
1. Design a URL Shortener (e.g., Bitly)
Key Considerations: A URL shortener is an application that takes a long URL and converts it into a shorter, unique identifier. The main challenge here is to ensure that the generated short URLs are unique, irreversible, and easy to access.
How to approach:
- Hashing: A strong hash function can generate unique short URLs. Use base62 encoding for a compact representation of the hashed value.
- Collision Handling: Implement mechanisms like linear probing or separate chaining to handle collisions efficiently.
- Database Storage: Store mapping of long URLs to short URLs, and ensure scalability for millions of records.
Considerations:
- Redirection performance
- Ensuring minimal database lookup time
- Security (avoiding malicious redirects)
Example structure:
- API: /shorten for URL shortening
- Database: A key-value store (e.g., Redis or MongoDB) for storing long URL to short URL mappings.
Also Read: Top 20 API Design Questions for System Design Interviews
2. Design a File Storage System
Key Considerations: This question tests your ability to build scalable and efficient file storage systems. Think of platforms like Dropbox, Google Drive, or iCloud.
How to approach:
- Scalability: Use distributed storage systems (e.g., Hadoop, Amazon S3).
- Replication: Implement replication across multiple servers to ensure data redundancy and availability.
- Metadata Management: Store metadata like file names, timestamps, and user information in a database.
Considerations:
- File retrieval speed
- Managing large-scale data efficiently
- User access control and security
Recommended Topic: Critical System Design Questions to Crack Any Tech Interview
Also Read: Airbnb System Design Questions
3. Design an In-Memory Cache

Key Considerations: An in-memory cache improves application performance by storing frequently accessed data in memory, reducing latency.
How to approach:
- Eviction Policies: Use algorithms like LRU (Least Recently Used) or LFU (Least Frequently Used) for cache eviction.
- Data Expiry: Implement time-based expiry for cache entries to keep the data fresh.
- Concurrency: Ensure thread-safety when multiple clients access the cache simultaneously.
Considerations:
- Data consistency
- Cache size management
- Handling cache misses and fallback
4. Design a Rate Limiter
Key Considerations: A rate limiter restricts the number of requests a client can make to a server in a given period. It’s essential for preventing abuse and ensuring fair use.
How to approach:
- Token Bucket Algorithm: Allows bursts of traffic but enforces long-term limits.
- Leaky Bucket Algorithm: Ensures that the requests are processed at a constant rate.
- Distributed Rate Limiting: For large-scale systems, implement distributed rate limiting using tools like Redis.
Considerations:
- Handling high-frequency requests
- Distributed and global rate limits
- Error handling for rate-limited users
Also Read: Top 15 Web Development Interview Questions
5. Design a Chat Application
Key Considerations: A chat application needs to support real-time messaging and user notifications.
How to approach:
- WebSocket Protocol: Use WebSocket for bidirectional communication to handle real-time updates.
- Database: Store message history, user data, and chat metadata in a relational or NoSQL database.
- Scalability: Design with horizontal scaling in mind, using microservices and load balancing.
Considerations:
- Handling message delivery failures
- User presence and notification systems
- Data privacy and encryption
Recommended Topic: Top 10 Web Development Interview QuestionsÂ
6. Design a Search Autocomplete System
Key Considerations: Autocomplete systems offer suggestions as users type search queries, improving user experience and search efficiency.
How to approach:
- Prefix Trees (Tries): Use tries for fast retrieval of autocomplete suggestions.
- Fuzzy Matching: Implement fuzzy search to handle typos and variations.
- Ranking: Rank the suggestions based on user behavior, search history, and relevance.
Considerations:
- Handling large sets of data efficiently
- Real-time data updates
- Personalization for users
Also Read: Top 15 Python Machine Learning Interview Questions
7. Design a Notification System

Key Considerations: A notification system sends alerts and messages to users about various events, such as messages, system alerts, or reminders.
How to approach:
- Event-Driven Architecture: Design the system to listen for events and trigger notifications based on the event type.
- Queue System: Use a message queue like RabbitMQ to handle notification delivery.
- Multiple Channels: Support multiple notification channels, such as email, SMS, and push notifications.
Considerations:
- Managing message priority
- Handling failure scenarios (e.g., when the notification fails to send)
- Scalability to handle millions of users
8. Design a Distributed Logging System
Key Considerations: A distributed logging system is essential for tracking events in distributed systems. It collects logs from multiple services and centralizes them for analysis.
How to approach:
- Log Aggregation: Use tools like ELK (Elasticsearch, Logstash, and Kibana) or Splunk for aggregating logs from different sources.
- Log Storage: Store logs in a distributed database for easy retrieval and analysis.
- Real-Time Processing: Implement real-time log streaming for monitoring purposes.
Considerations:
- High write throughput for log data
- Efficient log search and filtering
- Retention policies for log data
Recommended Topic: Top 15 Most Popular System Design Frameworks
9. Design a Session Management System
Key Considerations: Session management is vital for user authentication and maintaining state across multiple requests.
How to approach:
- Session Tokens: Use secure, expiring session tokens to authenticate users.
- Stateful vs Stateless: Decide whether to use stateful sessions (stored on the server) or stateless sessions (e.g., JWT tokens).
- Database: Use a fast in-memory store like Redis for session storage.
Considerations:
- Handling session expiration
- Securing session data
- Scaling session management across distributed systems
10. Design an E-commerce System
Key Considerations: E-commerce systems manage product catalogs, inventory, orders, and customer data.
How to approach:
- Product Database: Store product details, prices, and availability in a relational database.
- Shopping Cart: Design a cart system to handle product additions, removals, and quantity updates.
- Order Management: Implement an order management system to track user purchases, payments, and shipments.
Considerations:
- Transactional consistency (ACID properties)
- Inventory management
- Scalability to handle high traffic
Also Read: Top 10 Software Engineering Interview QuestionsÂ
11. Design a Social Media Feed
Key Considerations: Designing a social media feed involves displaying user-generated content in a timely and relevant manner.
How to approach:
- Feed Ranking Algorithm: Use ranking algorithms based on user engagement, relevance, and recency.
- Data Caching: Cache popular posts to reduce database load and increase response time.
- User Customization: Allow users to filter and customize their feed based on interests.
Considerations:
- Handling large-scale data
- Real-time feed updates
- Personalization
12. Design a Library Management System
Key Considerations: A library management system handles book checkouts, returns, and inventory management.
How to approach:
- Book Database: Store book details like title, author, and ISBN in a relational database.
- Transaction History: Keep track of borrowed and returned books.
- User Authentication: Ensure secure access for users to borrow and return books.
Considerations:
- Tracking overdue books
- Handling book availability
- User access control
13. Design an Event Scheduling System
Key Considerations: An event scheduling system enables users to schedule and manage appointments and events.
How to approach:
- Calendar Integration: Integrate with calendar APIs to manage event timings and conflicts.
- Event Reminders: Send notifications or alerts to users before an event.
- Availability: Allow users to view available time slots for scheduling.
Considerations:
- Handling time zone differences
- Conflict resolution for overlapping events
- User customization for event preferences
14. Design a Voting System

Key Considerations: A voting system allows users to cast votes and display results in real-time.
How to approach:
- Vote Integrity: Ensure that votes are counted accurately and cannot be tampered with.
- Scalability: Design the system to handle large volumes of votes without delay.
- Result Calculation: Use efficient algorithms to calculate results and display them quickly.
Considerations:
- Preventing vote manipulation
- User anonymity
- High availability and reliability
15. Design a Recommendation System
Key Considerations: A recommendation system suggests products, services, or content based on user preferences.
How to approach:
- Collaborative Filtering: Recommend items based on user behavior and preferences.
- Content-Based Filtering: Recommend items based on the content the user has interacted with.
- Hybrid Systems: Combine multiple techniques for better recommendations.
Considerations:
- Personalization for each user
- Handling large datasets
- Algorithm optimization for real-time recommendations
Â
FAQs
What is the difference between low-level and high-level design?
Low-level design involves detailed system components, algorithms, data structures, and architecture, while high-level design focuses on the overall system structure and its interaction between components. Low-level design includes specific implementation details, whereas high-level design covers broad architectural decisions.
How can I prepare for low-level design interviews?
To prepare for low-level design interviews, practice solving system design problems and focus on understanding core concepts such as algorithms, data structures, scalability, and fault tolerance. Reviewing real-world applications like chat applications or file storage systems will also help you build a solid foundation for answering these questions.
What tools can help in low-level design?
There are several tools that can assist with low-level design, such as flowchart software, diagramming tools like Lucidchart, and UML tools. These tools can help you visually represent your design and ensure clarity in your solutions during the interview process.
Accelerate your Path to a Product based Career
Boost your career or get hired at top product-based companies by joining our expertly crafted courses. Gain practical skills and real-world knowledge to help you succeed.
Reach Out Now
If you have any queries, please fill out this form. We will surely reach out to you.
Contact Email
Reach us at the following email address.
Phone Number
You can reach us by phone as well.
+91-97737 28034
Our Location
Rohini, Sector-3, Delhi-110085