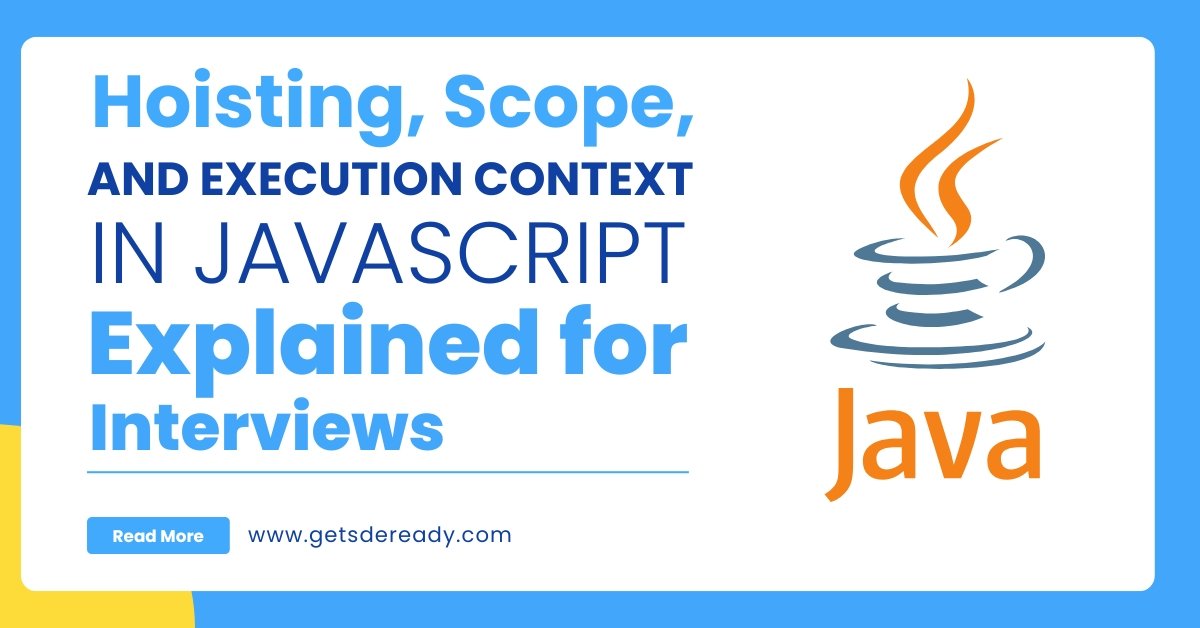
Top 20 Data Structures and Algorithms (DSA) Questions for Coding Interviews in 2025
Data Structures and Algorithms (DSA) are the backbone of technical interviews for aspiring software engineers. As 2025 approaches, the demand for DSA knowledge continues to grow, and coding interviews remain a key hurdle for candidates. In this article, we will explore the top 20 DSA questions that are commonly asked in coding interviews. These questions will help you prepare effectively for your upcoming tech interviews and improve your problem-solving skills.
1. What is the difference between an array and a linked list?
Arrays and linked lists are fundamental data structures, and understanding their differences is crucial for solving many coding problems.
Key Differences Between Arrays and Linked Lists
Arrays are contiguous blocks of memory, while linked lists consist of nodes where each node points to the next node in the list. This results in different time complexities for insertion, deletion, and access operations.
- Arrays:
- Fixed size
- Efficient random access
- Insertion and deletion require shifting elements
- Linked Lists:
- Dynamic size
- Efficient insertion and deletion
- Slow random access
Arrays are ideal when you need fast access to elements, but linked lists are more suitable for scenarios where frequent insertions or deletions occur.
Recommended Topic: Top 20 Software Frameworks for 2025
2. Explain how a stack works.
A stack is a linear data structure that follows the Last In First Out (LIFO) principle, where the last element added is the first to be removed.
Working of a Stack
Stacks are used in various applications such as expression evaluation, function calls, and backtracking algorithms. Elements can only be pushed (added) or popped (removed) from the top of the stack.
- Push Operation: Adds an element to the top
- Pop Operation: Removes the topmost element
- Peek Operation: Views the top element without removing it
Stacks are efficient when you need to track recursive operations or backtrack in algorithms.
Also Read: Top 20 API Design Interview Questions
3. What is a queue and how does it differ from a stack?

A queue is a linear data structure that follows the First In First Out (FIFO) principle, which means the first element added will be the first one to be removed.
Key Differences Between a Queue and Stack
- Queue:
- Follows FIFO
- Useful in scheduling tasks or managing requests in real-time systems
- Stack:
- Follows LIFO
- Useful for undo operations and backtracking algorithms
Recommended Topic: Top 10 Full-Stack Interview Questions
4. What is the difference between a depth-first search (DFS) and a breadth-first search (BFS)?
Both DFS and BFS are graph traversal algorithms, but they differ significantly in their approach.
Key Differences
- DFS explores as far as possible along each branch before backtracking.
- BFS explores all neighbors at the present depth level before moving on to the next level.
DFS is implemented using recursion or a stack, while BFS uses a queue. DFS is useful in solving puzzles like mazes, while BFS is better for shortest path algorithms.
5. What are binary search trees (BST) and how are they used?
A Binary Search Tree (BST) is a tree data structure where each node has at most two children, and the left child is smaller than the parent, while the right child is larger.
Key Properties of BST
- Efficient Search: The height of a balanced BST is logarithmic, which ensures fast searches.
- Insertion and Deletion: Both operations can be done efficiently if the tree is balanced.
BSTs are widely used in searching algorithms, database indexing, and maintaining sorted data.
6. How does a heap differ from a binary tree?
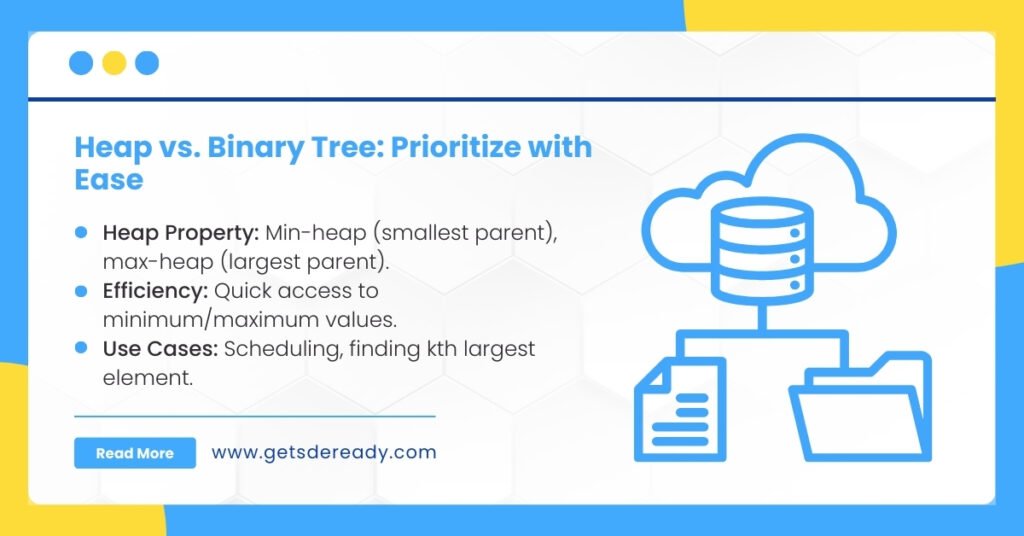
A heap is a special type of binary tree that satisfies the heap property. It is used primarily for implementing priority queues.
Key Properties of Heaps
- Min-Heap: The parent node is smaller than its children.
- Max-Heap: The parent node is larger than its children.
Heaps provide efficient solutions for problems that require quick access to the maximum or minimum element, such as scheduling tasks or finding the kth largest element.
7. What is a hash table?
A hash table is a data structure that stores key-value pairs and allows for fast retrieval of values based on the key.
Key Properties
- Hashing: The key is passed through a hash function to determine its position in the table.
- Collision Handling: Hash tables must handle cases where two keys hash to the same index.
Hash tables are essential in scenarios where you need fast lookups, such as implementing caches or counting frequencies of elements.
Also Read: Top 10 Google Software Engineering Questions
8. Explain the concept of dynamic programming

Dynamic programming is a technique used to solve problems by breaking them down into simpler subproblems and storing the results of subproblems to avoid redundant calculations.
Key Benefits of Dynamic Programming
- Optimal Substructure: Problems that can be broken down into smaller subproblems.
- Overlapping Subproblems: The same subproblems are solved multiple times in a naive recursive approach.
Dynamic programming is widely used in problems like finding the shortest path, knapsack problems, and optimization problems.
Recommended Topic: Top 15 System Design Frameworks in 2024
9. What is the time complexity of different sorting algorithms?
Sorting is an essential operation in many algorithms. Understanding the time complexity of sorting algorithms helps in choosing the right one for the task.
Key Sorting Algorithms
- Bubble Sort: O(n^2)
- Quick Sort: O(n log n) on average
- Merge Sort: O(n log n)
- Insertion Sort: O(n^2)
Quick sort and merge sort are the most efficient for large datasets, but bubble sort and insertion sort can be useful for small or nearly sorted datasets.
10. How do you reverse a linked list?
Reversing a linked list is a common interview question that tests understanding of pointers and memory manipulation.
Key Steps to Reverse a Linked List
- Initialize three pointers: previous, current, and next.
- Traverse the list and reverse the links one by one.
- Set the head of the list to the last node.
Reversing a linked list is an essential operation used in problems like checking for palindromes or reversing data flows.
11. What is the difference between a shallow copy and a deep copy?
In programming, copying objects can be done in two ways: shallow copy and deep copy.
Key Differences Between Shallow and Deep Copy
- Shallow Copy: Copies the reference to the object, not the object itself.
- Deep Copy: Copies the object and all of its nested objects.
Shallow copy is faster but may lead to unexpected results when modifying objects, while deep copy ensures that the copy is entirely independent.
12. What is a trie and when would you use it?
A trie, also known as a prefix tree, is a tree data structure used to store a dynamic set of strings, such as a dictionary or a search engine autocomplete system.
Key Features of a Trie
- Efficient Prefix Searching: Can quickly search for words with common prefixes.
- Space Complexity: While it can take up more memory, it is efficient in terms of search time.
Tries are ideal for problems related to string matching, such as autocomplete suggestions or dictionary lookups.
13. What are some common interview questions for arrays?
Arrays are fundamental data structures, and many coding interviews focus on operations involving arrays, such as searching, sorting, and manipulation.
Key Array Operations in Interviews
- Find Maximum/Minimum: Efficient methods to find the largest or smallest element.
- Find Duplicates: Identifying and removing duplicates from an array.
- Array Rotation: Rotating the elements of an array by a given number.
Arrays are useful for solving problems in data storage and manipulation.
14. What are graph algorithms and their types?
Graphs are versatile data structures, and graph algorithms help in finding paths, cycles, and shortest distances in graphs.
Key Graph Algorithms
- Dijkstra’s Algorithm: Finds the shortest path between nodes in a graph.
- Floyd-Warshall Algorithm: Calculates shortest paths between all pairs of nodes.
- Kruskal’s Algorithm: Finds the minimum spanning tree of a graph.
Graph algorithms are used in networking, transportation systems, and social network analysis.
15. What is the knapsack problem?
The knapsack problem is a famous optimization problem where the goal is to fill a knapsack with items to maximize the total value, subject to weight constraints.
Solution Approaches
- Greedy Approach: Picks items based on their value-to-weight ratio.
- Dynamic Programming: Solves using overlapping subproblems to find the optimal solution.
This problem is widely used in resource allocation and financial planning.
16. What are the different types of binary trees?
Binary trees are essential structures in computer science. They have various types depending on their properties.
Key Types of Binary Trees
- Full Binary Tree: Every node has either 0 or 2 children.
- Complete Binary Tree: Every level is completely filled, except possibly the last.
- Balanced Binary Tree: The height difference between left and right subtrees is at most 1.
17. What is a hash map and how does it work?
A hash map is a data structure that stores key-value pairs and allows for fast lookups by using a hash function.
Key Properties of a Hash Map
- Constant Time Access: Allows O(1) average time complexity for insertions and lookups.
- Collisions: Hash maps use methods like chaining or open addressing to handle collisions.
Hash maps are widely used for implementing caches, storing configuration data, and counting frequencies of items.
18. What is a sliding window technique?
The sliding window technique is used for solving problems involving subarrays or substrings in a given array.
How Sliding Window Works
- Fixed Window: The window size is constant, and we slide it across the array.
- Variable Window: The window size adjusts dynamically based on the condition of the problem.
This technique is useful for problems related to finding subarrays with specific properties, such as the maximum sum or longest substring without repeating characters.
19. What is recursion and how is it used in coding interviews?
Recursion is a technique where a function calls itself to solve a problem by breaking it into smaller subproblems.
Key Points About Recursion
- Base Case: The simplest form of the problem.
- Recursive Case: The function calls itself with a reduced problem size.
Recursion is widely used in algorithms like binary search, tree traversals, and dynamic programming.
20. How do you detect a cycle in a graph?
Detecting cycles in a graph is an important task in many algorithms, particularly when dealing with directed or undirected graphs.
Cycle Detection Algorithms
- DFS Traversal: Mark nodes during traversal to detect cycles.
- Union-Find Algorithm: Used for detecting cycles in undirected graphs.
Detecting cycles is crucial for tasks such as scheduling and network design.
FAQs
How important are Data Structures and Algorithms for coding interviews?
Data structures and algorithms are the core of most coding interviews. A strong understanding of DSA is essential for solving complex problems, optimizing solutions, and performing well in interviews for tech companies.
What are the most common types of DSA interview questions?
The most common DSA interview questions include array manipulation, string operations, linked list operations, graph algorithms, dynamic programming, and tree traversals.
How can I improve my DSA skills?
Improving DSA skills requires practice. You should solve problems from coding platforms like LeetCode, HackerRank, and Codeforces. Additionally, taking online courses and reading books on DSA can help strengthen your understanding.
What resources can help with DSA interview preparation?
Resources such as online coding platforms, textbooks, and structured courses like Master DSA & Web Development with System Design can be valuable in preparing for DSA interviews.
What are the best courses for learning DSA and Web Development?
Courses like DSA Web Development and Master DSA & Web Development are excellent options for in-depth learning.
Accelerate your Path to a Product based Career
Boost your career or get hired at top product-based companies by joining our expertly crafted courses. Gain practical skills and real-world knowledge to help you succeed.
Reach Out Now
If you have any queries, please fill out this form. We will surely reach out to you.
Contact Email
Reach us at the following email address.
Phone Number
You can reach us by phone as well.
+91-97737 28034
Our Location
Rohini, Sector-3, Delhi-110085