Data Structures and Algorithms
- Introduction to Data Structures and Algorithms
- Time and Space Complexity Analysis
- Big-O, Big-Theta, and Big-Omega Notations
- Recursion and Backtracking
- Divide and Conquer Algorithm
- Dynamic Programming: Memoization vs. Tabulation
- Greedy Algorithms and Their Use Cases
- Understanding Arrays: Types and Operations
- Linear Search vs. Binary Search
- Sorting Algorithms: Bubble, Insertion, Selection, and Merge Sort
- QuickSort: Explanation and Implementation
- Heap Sort and Its Applications
- Counting Sort, Radix Sort, and Bucket Sort
- Hashing Techniques: Hash Tables and Collisions
- Open Addressing vs. Separate Chaining in Hashing
- DSA Questions for Beginners
- Advanced DSA Questions for Competitive Programming
- Top 10 DSA Questions to Crack Your Next Coding Test
- Top 50 DSA Questions Every Programmer Should Practice
- Top Atlassian DSA Interview Questions
- Top Amazon DSA Interview Questions
- Top Microsoft DSA Interview Questions
- Top Meta (Facebook) DSA Interview Questions
- Netflix DSA Interview Questions and Preparation Guide
- Top 20 DSA Interview Questions You Need to Know
- Top Uber DSA Interview Questions and Solutions
- Google DSA Interview Questions and How to Prepare
- Airbnb DSA Interview Questions and How to Solve Them
- Mobile App DSA Interview Questions and Solutions
DSA Interview Questions
- DSA Questions for Beginners
- Advanced DSA Questions for Competitive Programming
- Top 10 DSA Questions to Crack Your Next Coding Test
- Top 50 DSA Questions Every Programmer Should Practice
- Top Atlassian DSA Interview Questions
- Top Amazon DSA Interview Questions
- Top Microsoft DSA Interview Questions
- Top Meta (Facebook) DSA Interview Questions
- Netflix DSA Interview Questions and Preparation Guide
- Top 20 DSA Interview Questions You Need to Know
- Top Uber DSA Interview Questions and Solutions
- Google DSA Interview Questions and How to Prepare
- Airbnb DSA Interview Questions and How to Solve Them
- Mobile App DSA Interview Questions and Solutions
Introduction to High-Level System Design
System Design Fundamentals
- Functional vs. Non-Functional Requirements
- Scalability, Availability, and Reliability
- Latency and Throughput Considerations
- Load Balancing Strategies
Architectural Patterns
- Monolithic vs. Microservices Architecture
- Layered Architecture
- Event-Driven Architecture
- Serverless Architecture
- Model-View-Controller (MVC) Pattern
- CQRS (Command Query Responsibility Segregation)
Scaling Strategies
- Vertical Scaling vs. Horizontal Scaling
- Sharding and Partitioning
- Data Replication and Consistency Models
- Load Balancing Strategies
- CDN and Edge Computing
Database Design in HLD
- SQL vs. NoSQL Databases
- CAP Theorem and its Impact on System Design
- Database Indexing and Query Optimization
- Database Sharding and Partitioning
- Replication Strategies
API Design and Communication
Caching Strategies
- Types of Caching
- Cache Invalidation Strategies
- Redis vs. Memcached
- Cache-Aside, Write-Through, and Write-Behind Strategies
Message Queues and Event-Driven Systems
- Kafka vs. RabbitMQ vs. SQS
- Pub-Sub vs. Point-to-Point Messaging
- Handling Asynchronous Workloads
- Eventual Consistency in Distributed Systems
Security in System Design
Observability and Monitoring
- Logging Strategies (ELK Stack, Prometheus, Grafana)
- API Security Best Practices
- Secure Data Storage and Access Control
- DDoS Protection and Rate Limiting
Real-World System Design Case Studies
- Distributed locking (Locking and its Types)
- Memory leaks and Out of memory issues
- HLD of YouTube
- HLD of WhatsApp
System Design Interview Questions
- Adobe System Design Interview Questions
- Top Atlassian System Design Interview Questions
- Top Amazon System Design Interview Questions
- Top Microsoft System Design Interview Questions
- Top Meta (Facebook) System Design Interview Questions
- Top Netflix System Design Interview Questions
- Top Uber System Design Interview Questions
- Top Google System Design Interview Questions
- Top Apple System Design Interview Questions
- Top Airbnb System Design Interview Questions
- Top 10 System Design Interview Questions
- Mobile App System Design Interview Questions
- Top 20 Stripe System Design Interview Questions
- Top Shopify System Design Interview Questions
- Top 20 System Design Interview Questions
- Top Advanced System Design Questions
- Most-Frequented System Design Questions in Big Tech Interviews
- What Interviewers Look for in System Design Questions
- Critical System Design Questions to Crack Any Tech Interview
- Top 20 API Design Questions for System Design Interviews
- Top 10 Steps to Create a System Design Portfolio for Developers
Top Amazon DSA Interview Questions and Preparation Guide
Preparing for Amazon’s DSA (Data Structures and Algorithms) interview can feel overwhelming, but with the right strategy, you can ace it! Whether you’re a beginner or polishing your skills, this guide covers everything you need to know. Ready to dive in? Sign up for free course updates to stay ahead: Amazon Interview Prep Course.
Understanding Amazon’s DSA Interview Process
What is DSA?
Data Structures and Algorithms (DSA) form the backbone of technical interviews at Amazon. DSA tests your problem-solving skills, coding efficiency, and ability to optimize solutions. According to HackerRank’s 2023 Developer Survey, 78% of tech companies prioritize DSA over other skills.
Amazon’s Focus on DSA
Amazon’s interview process emphasizes problem-solving under constraints, mirroring real-world scenarios. Candidates often face questions on arrays, trees, graphs, and dynamic programming.
Key Stats
- 60% of Amazon interview rounds focus on DSA.
- 40% of rejected candidates fail due to poor DSA performance (Amazon Hiring Report, 2022).
Top 10 Amazon DSA Interview Questions
1. Find the Missing Number in an Array
Explanation
Given an array of integers from 1 to N with one missing number, find the missing value.
Example
Input: [1, 2, 4, 6, 3, 7, 8]
Output: 5
Approach
Use the formula for the sum of the first N natural numbers.
Tips
- Optimize for O(n) time and O(1) space.
- Avoid nested loops.
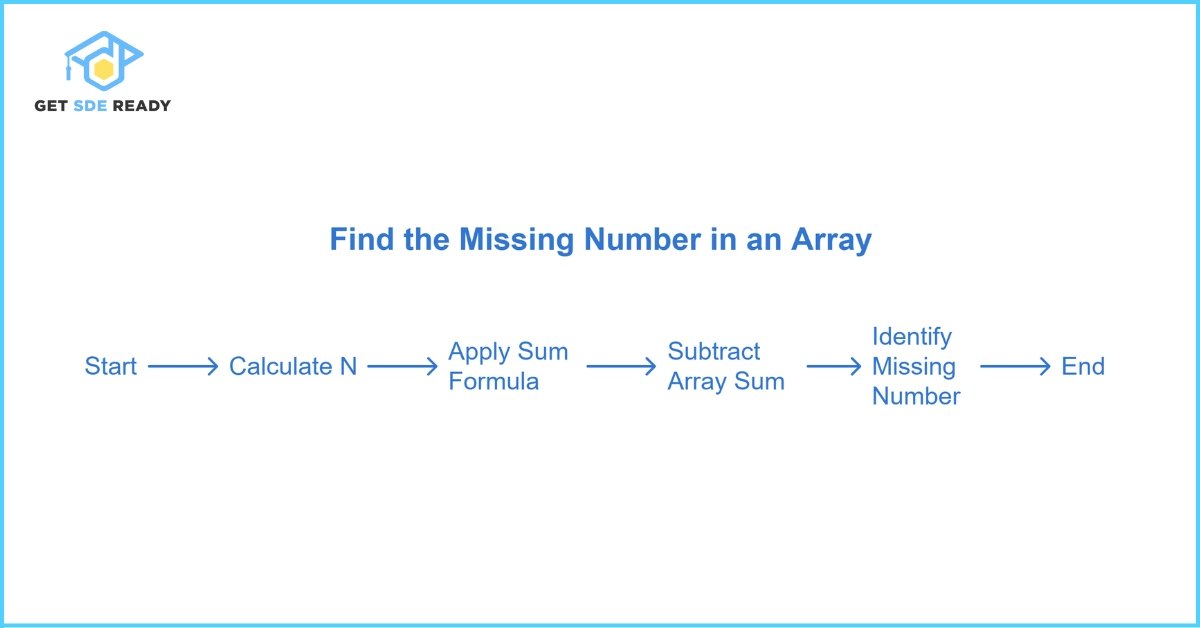
2. Detect a Cycle in a Linked List
Explanation
Determine if a linked list has a cycle using Floyd’s Tortoise and Hare algorithm.
Example
Input: 1 → 2 → 3 → 4 → 5 → 2 (cycle)
Output: True
Approach
Use two pointers moving at different speeds.
Tips
- Practice edge cases (e.g., empty lists).
- Understand the mathematical proof behind the algorithm.
3. Merge Two Sorted Arrays
Explanation
Combine two sorted arrays into one sorted array.
Example
Input: [1, 3, 5], [2, 4, 6]
Output: [1, 2, 3, 4, 5, 6]
Approach
Use a two-pointer technique for O(n) time.
4. Implement a Binary Search Tree Iterator
Explanation
Design an iterator for in-order traversal of a BST.
Approach
Use a stack to simulate recursion.
5. Longest Palindromic Substring
Explanation
Find the longest substring that reads the same backward and forward.
Approach
Expand around potential centers (O(n²) time).
6. Serialize and Deserialize a Binary Tree
Explanation
Convert a binary tree into a string and reconstruct it.
Approach
Use pre-order traversal with markers for null nodes.
7. Top K Frequent Elements
Explanation
Return the k most frequent elements in an array.
Approach
Use a hash map + min-heap for O(n log k) time.
8. Clone a Graph
Explanation
Create a deep copy of a graph with nodes and edges.
Approach
Use BFS + a hash map to track cloned nodes.
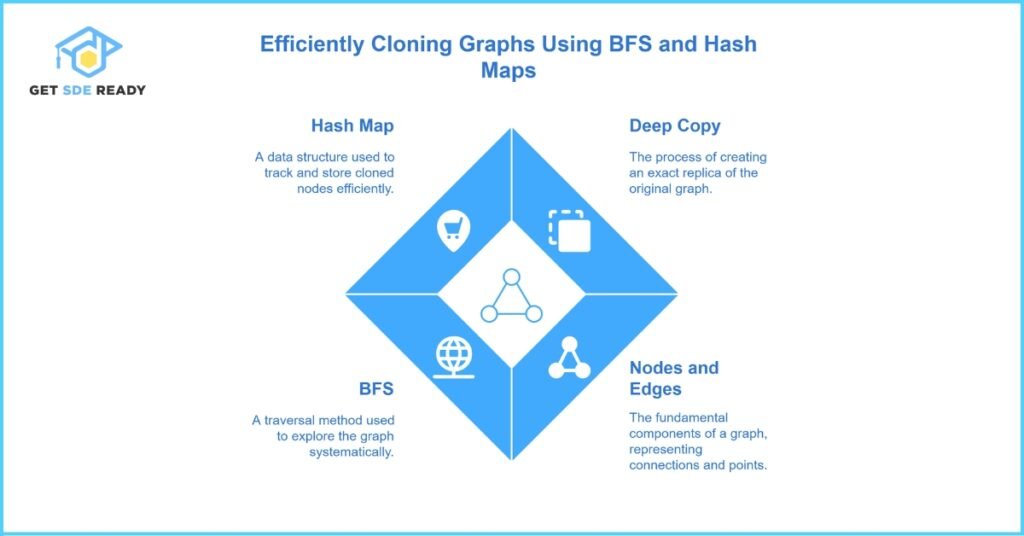
9. Minimum Spanning Tree
Explanation
Find the subset of edges connecting all nodes with minimal total weight.
Approach
Kruskal’s or Prim’s algorithm.
10. Dynamic Programming: Coin Change Problem
Explanation
Determine the fewest coins needed to make a target amount.
Approach
Use DP with a 1D array to track minimum coins.
Preparation Strategies for Amazon DSA Interviews
Master Core Concepts
Focus on arrays, linked lists, trees, graphs, and dynamic programming. Use platforms like LeetCode or HackerRank for practice.
Practice Problem-Solving
Solve at least 2-3 problems daily. Track your progress with a spreadsheet.
Table: Study Plan Example
Week | Focus Area | Target Problems |
1 | Arrays/Strings | 15 |
2 | Trees | 10 |
Mock Interviews
Simulate real interviews with peers or platforms like Pramp.

Common Mistakes to Avoid
Ignoring Time Complexity
Amazon expects solutions with optimal time/space complexity.
Poor Code Readability
Write clean code with proper indentation and variable names.
Overcomplicating Solutions
Start with brute-force approaches, then optimize.
Post-Interview Follow-Up
Reflect on Performance
Identify weak areas and revisit concepts.
Ask for Feedback
If rejected, request feedback to improve.
Recommended Resources
- Books: Cracking the Coding Interview by Gayle Laakmann McDowell.
- Courses: Master DSA, Web Dev & System Design.
FAQ
How do I start preparing for Amazon’s DSA interview?
Begin by mastering core data structures like arrays and linked lists. Practice daily on platforms like LeetCode and review Amazon-specific questions. For structured learning, check out DSA Courses.
What are the most common DSA topics at Amazon?
Amazon frequently tests arrays, trees, dynamic programming, and graph algorithms. Focus on solving problems in these areas with optimal time complexity.
How important is system design for Amazon interviews?
While DSA is critical, system design is also key for senior roles. Learn to design scalable systems using resources like System Design for Real-Time Chat Apps.
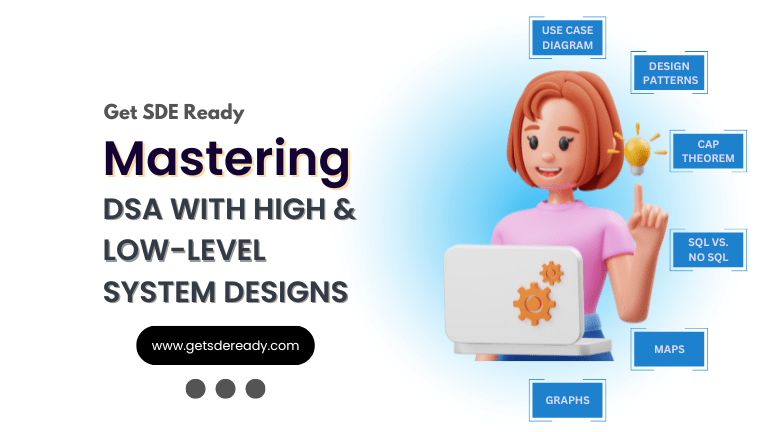
DSA, High & Low Level System Designs
- 85+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 400+ DSA Practice Questions
- Comprehensive Notes
- HackerRank Tests & Quizzes
- Topic-wise Quizzes
- Case Studies
- Access to Global Peer Community
Buy for 60% OFF
₹25,000.00 ₹9,999.00
Accelerate your Path to a Product based Career
Boost your career or get hired at top product-based companies by joining our expertly crafted courses. Gain practical skills and real-world knowledge to help you succeed.
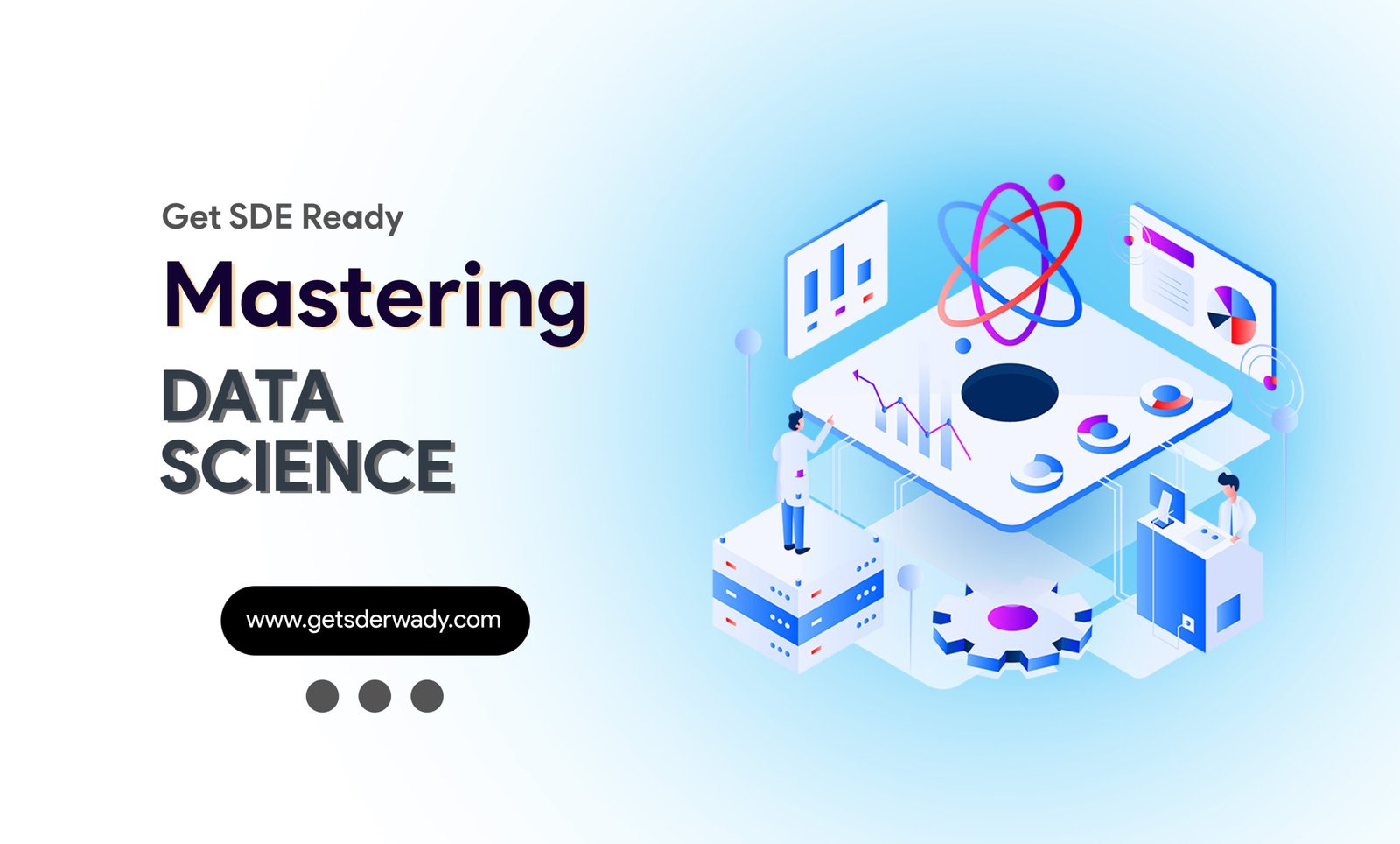
Essentials of Machine Learning and Artificial Intelligence
- 65+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 22+ Hands-on Live Projects & Deployments
- Comprehensive Notes
- Topic-wise Quizzes
- Case Studies
- Access to Global Peer Community
- Interview Prep Material
Buy for 65% OFF
₹20,000.00 ₹6,999.00
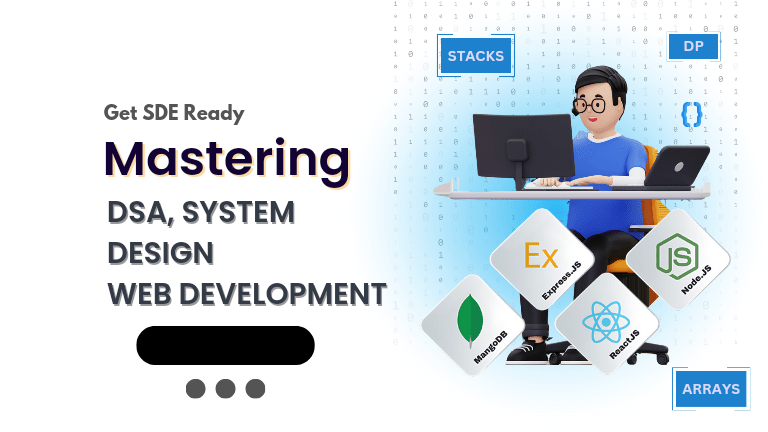
Fast-Track to Full Spectrum Software Engineering
- 120+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 400+ DSA Practice Questions
- Comprehensive Notes
- HackerRank Tests & Quizzes
- 12+ live Projects & Deployments
- Case Studies
- Access to Global Peer Community
Buy for 57% OFF
₹35,000.00 ₹14,999.00
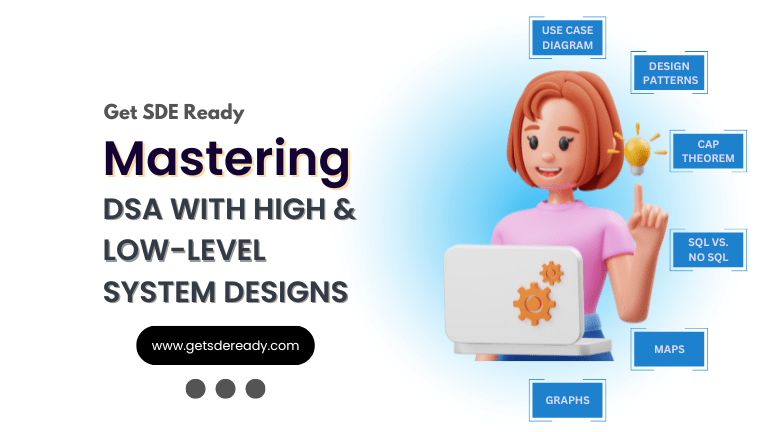
DSA, High & Low Level System Designs
- 85+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 400+ DSA Practice Questions
- Comprehensive Notes
- HackerRank Tests & Quizzes
- Topic-wise Quizzes
- Case Studies
- Access to Global Peer Community
Buy for 60% OFF
₹25,000.00 ₹9,999.00

Low & High Level System Design
- 20+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 400+ DSA Practice Questions
- Comprehensive Notes
- HackerRank Tests
- Topic-wise Quizzes
- Access to Global Peer Community
- Interview Prep Material
Buy for 65% OFF
₹20,000.00 ₹6,999.00
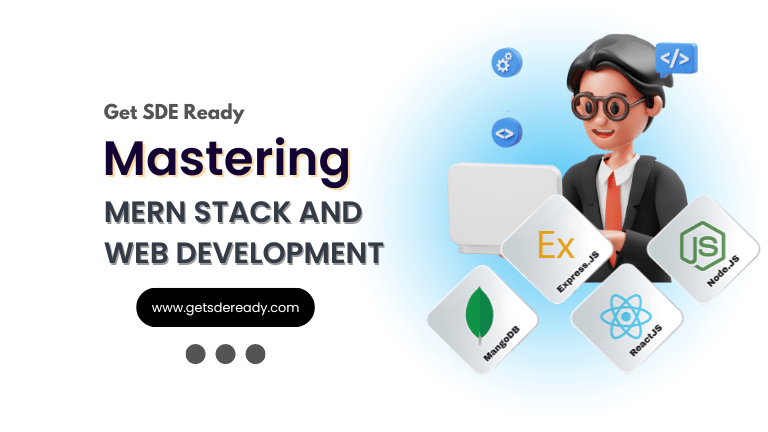
Mastering Mern Stack (WEB DEVELOPMENT)
- 65+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 12+ Hands-on Live Projects & Deployments
- Comprehensive Notes & Quizzes
- Real-world Tools & Technologies
- Access to Global Peer Community
- Interview Prep Material
- Placement Assistance
Buy for 60% OFF
₹15,000.00 ₹5,999.00
Reach Out Now
If you have any queries, please fill out this form. We will surely reach out to you.
Contact Email
Reach us at the following email address.
arun@getsdeready.com
Phone Number
You can reach us by phone as well.
+91-97737 28034
Our Location
Rohini, Sector-3, Delhi-110085