Introduction to High-Level System Design
System Design Fundamentals
- Functional vs. Non-Functional Requirements
- Scalability, Availability, and Reliability
- Latency and Throughput Considerations
- Load Balancing Strategies
Architectural Patterns
- Monolithic vs. Microservices Architecture
- Layered Architecture
- Event-Driven Architecture
- Serverless Architecture
- Model-View-Controller (MVC) Pattern
- CQRS (Command Query Responsibility Segregation)
Scaling Strategies
- Vertical Scaling vs. Horizontal Scaling
- Sharding and Partitioning
- Data Replication and Consistency Models
- Load Balancing Strategies
- CDN and Edge Computing
Database Design in HLD
- SQL vs. NoSQL Databases
- CAP Theorem and its Impact on System Design
- Database Indexing and Query Optimization
- Database Sharding and Partitioning
- Replication Strategies
API Design and Communication
Caching Strategies
- Types of Caching
- Cache Invalidation Strategies
- Redis vs. Memcached
- Cache-Aside, Write-Through, and Write-Behind Strategies
Message Queues and Event-Driven Systems
- Kafka vs. RabbitMQ vs. SQS
- Pub-Sub vs. Point-to-Point Messaging
- Handling Asynchronous Workloads
- Eventual Consistency in Distributed Systems
Security in System Design
Observability and Monitoring
- Logging Strategies (ELK Stack, Prometheus, Grafana)
- API Security Best Practices
- Secure Data Storage and Access Control
- DDoS Protection and Rate Limiting
Real-World System Design Case Studies
- Distributed locking (Locking and its Types)
- Memory leaks and Out of memory issues
- HLD of YouTube
- HLD of WhatsApp
System Design Interview Questions
- Adobe System Design Interview Questions
- Top Atlassian System Design Interview Questions
- Top Amazon System Design Interview Questions
- Top Microsoft System Design Interview Questions
- Top Meta (Facebook) System Design Interview Questions
- Top Netflix System Design Interview Questions
- Top Uber System Design Interview Questions
- Top Google System Design Interview Questions
- Top Apple System Design Interview Questions
- Top Airbnb System Design Interview Questions
- Top 10 System Design Interview Questions
- Mobile App System Design Interview Questions
- Top 20 Stripe System Design Interview Questions
- Top Shopify System Design Interview Questions
- Top 20 System Design Interview Questions
- Top Advanced System Design Questions
- Most-Frequented System Design Questions in Big Tech Interviews
- What Interviewers Look for in System Design Questions
- Critical System Design Questions to Crack Any Tech Interview
- Top 20 API Design Questions for System Design Interviews
- Top 10 Steps to Create a System Design Portfolio for Developers
Introduction to High-Level System Design
System Design Fundamentals
- Functional vs. Non-Functional Requirements
- Scalability, Availability, and Reliability
- Latency and Throughput Considerations
- Load Balancing Strategies
Architectural Patterns
- Monolithic vs. Microservices Architecture
- Layered Architecture
- Event-Driven Architecture
- Serverless Architecture
- Model-View-Controller (MVC) Pattern
- CQRS (Command Query Responsibility Segregation)
Scaling Strategies
- Vertical Scaling vs. Horizontal Scaling
- Sharding and Partitioning
- Data Replication and Consistency Models
- Load Balancing Strategies
- CDN and Edge Computing
Database Design in HLD
- SQL vs. NoSQL Databases
- CAP Theorem and its Impact on System Design
- Database Indexing and Query Optimization
- Database Sharding and Partitioning
- Replication Strategies
API Design and Communication
Caching Strategies
- Types of Caching
- Cache Invalidation Strategies
- Redis vs. Memcached
- Cache-Aside, Write-Through, and Write-Behind Strategies
Message Queues and Event-Driven Systems
- Kafka vs. RabbitMQ vs. SQS
- Pub-Sub vs. Point-to-Point Messaging
- Handling Asynchronous Workloads
- Eventual Consistency in Distributed Systems
Security in System Design
Observability and Monitoring
- Logging Strategies (ELK Stack, Prometheus, Grafana)
- API Security Best Practices
- Secure Data Storage and Access Control
- DDoS Protection and Rate Limiting
Real-World System Design Case Studies
- Distributed locking (Locking and its Types)
- Memory leaks and Out of memory issues
- HLD of YouTube
- HLD of WhatsApp
System Design Interview Questions
- Adobe System Design Interview Questions
- Top Atlassian System Design Interview Questions
- Top Amazon System Design Interview Questions
- Top Microsoft System Design Interview Questions
- Top Meta (Facebook) System Design Interview Questions
- Top Netflix System Design Interview Questions
- Top Uber System Design Interview Questions
- Top Google System Design Interview Questions
- Top Apple System Design Interview Questions
- Top Airbnb System Design Interview Questions
- Top 10 System Design Interview Questions
- Mobile App System Design Interview Questions
- Top 20 Stripe System Design Interview Questions
- Top Shopify System Design Interview Questions
- Top 20 System Design Interview Questions
- Top Advanced System Design Questions
- Most-Frequented System Design Questions in Big Tech Interviews
- What Interviewers Look for in System Design Questions
- Critical System Design Questions to Crack Any Tech Interview
- Top 20 API Design Questions for System Design Interviews
- Top 10 Steps to Create a System Design Portfolio for Developers
Top Netflix DSA Interview Questions and Preparation Guide
Landing a role at Netflix is a dream for many tech professionals, but cracking their rigorous Data Structures and Algorithms (DSA) interview requires strategic preparation. To help you get started, sign up for free course updates and resources using our form. Let’s dive into the essential questions, preparation tips, and insider insights to ace your Netflix interview!
Also Read: Low-Level Design of WhatsApp Messaging
Understanding Netflix’s DSA Interview Process
Netflix’s interview process is designed to identify candidates who can solve complex problems efficiently. Their DSA rounds focus on real-world scalability, performance optimization, and clean coding practices.

Why DSA Matters at Netflix
Netflix handles billions of requests daily, requiring algorithms that optimize data streaming, user recommendations, and server load balancing. For example, their recommendation engine analyzes terabytes of data to personalize content, making efficient algorithms critical.
Key reasons DSA is vital at Netflix:
- Scalability: Solutions must handle millions of users simultaneously.
- Real-time processing: Algorithms power instant content delivery.
- Resource optimization: Efficient code reduces server costs.
Fact: A 2023 study by TechReview found that Netflix’s algorithm improvements reduced buffering time by 30%.
Interview Process Overview
Netflix’s DSA interview typically includes:
- Phone Screen: A 45-minute coding round on platforms like CodeSignal.
- Technical Rounds (3-4): On-site or virtual sessions focusing on problem-solving and code review.
- System Design Round: Designing scalable systems (e.g., video streaming).
- Behavioral Round: Assessing cultural fit and collaboration skills.
Stage | Duration | Focus |
Phone Screen | 45 minutes | Coding challenges |
Technical Rounds | 60 minutes | DSA, debugging |
System Design | 60 minutes | Architecture, scalability |
Behavioral | 45 minutes | Teamwork, problem-solving |
Also Read: Low-Level Design of YouTube Recommendations
Top 15 Netflix DSA Interview Questions (Expanded List)`
To ensure comprehensive preparation, here are 15 additional questions categorized by topic, along with optimal approaches and relevance to Netflix’s technical challenges.
Trees and Graphs
1. Serialize and Deserialize a Binary Tree (LeetCode 297)
- Why Netflix Asks This: Efficient data serialization is critical for transmitting user watch history or recommendation graphs across servers.
- Optimal Approach: Use pre-order traversal with a delimiter.
2. Number of Islands (LeetCode 200)
- Why Netflix Asks This: Models clustering of users or content in recommendation systems.
- Optimal Approach: DFS/BFS to traverse connected components.
3. Course Schedule (LeetCode 207)
- Why Netflix Asks This: Validating dependencies in microservices or content release pipelines.
- Optimal Approach: Topological sorting with Kahn’s algorithm.
Problem | Optimal Approach | Time Complexity |
Serialize Binary Tree | Pre-order traversal | O(n) |
Number of Islands | DFS/BFS | O(mn) |
Course Schedule | Topological Sort | O(V + E) |
Dynamic Programming
4. Longest Increasing Path in a Matrix (LeetCode 329)
- Why Netflix Asks This: Simulates optimizing content discovery paths in a grid-like UI.
- Optimal Approach: DFS + memoization.
5. Word Break Problem (LeetCode 139)
- Why Netflix Asks This: Parsing search queries or subtitles efficiently.
- Optimal Approach: DP with a boolean array.
6. Edit Distance (LeetCode 72)
- Why Netflix Asks This: Used in spell-checking search bars or subtitle synchronization.
- Optimal Approach: DP table for insert/delete/replace operations.
Key Insight:
“Dynamic programming is Netflix’s go-to for optimizing repetitive subproblems in large datasets.”
— John Carter, Netflix ML Engineer
Arrays and Strings
7. Trapping Rain Water (LeetCode 42)
- Why Netflix Asks This: Analogous to resource allocation in server load balancing.
- Optimal Approach: Two-pointer technique.
8. Rotate Image (LeetCode 48)
- Why Netflix Asks This: Manipulating image metadata for thumbnails or UI layouts.
- Optimal Approach: Transpose + reverse rows.
9. Maximum Product Subarray (LeetCode 152)
- Why Netflix Asks This: Maximizing user engagement metrics in a subarray of content.
- Optimal Approach: Track max/min products iteratively.
Example:
For arr = [2, 3, -2, 4], the maximum product is 6 (2×3).
Hash Tables and Heaps
10. Design LRU Cache (LeetCode 146)
- Why Netflix Asks This: Critical for caching frequently streamed content to reduce latency.
- Optimal Approach: Hash table + doubly linked list.
11. Kth Largest Element in a Stream (LeetCode 703)
- Why Netflix Asks This: Real-time analytics for trending shows or user activity.
- Optimal Approach: Min-heap of size k.
12. Find Median from Data Stream (LeetCode 295)
- Why Netflix Asks This: Monitoring real-time metrics like buffering rates.
- Optimal Approach: Two heaps (max-heap + min-heap).
Also Read: Top 20 API and RESTful Design Questions
Advanced Problem Solving
13. Minimum Window Substring (LeetCode 76)
- Why Netflix Asks This: Optimizing search results or ad targeting.
- Optimal Approach: Sliding window with hash maps.
14. Merge Intervals (LeetCode 56)
- Why Netflix Asks This: Scheduling server maintenance without downtime.
- Optimal Approach: Sort intervals and merge overlaps.
15. Implement Trie (LeetCode 208)
- Why Netflix Asks This: Autocomplete search bars or content tagging.
- Optimal Approach: Tree with nodes for each character.
Problem | Netflix Use Case |
LRU Cache | Reducing CDN latency |
Merge Intervals | Server task scheduling |
Trie Implementation | Search bar autocomplete |
Final Tips for Success
- Practice System Design: Many DSA questions at Netflix overlap with low-level design. For example, designing a cache ties into LRU problems. Enroll in Design & DSA Combined for end-to-end preparation.
- Simulate Real Interviews: Use platforms like Interviewing.io to mimic Netflix’s pressure-filled environment.
Also Read: System Design for Real-Time Chat Apps
Preparation Strategies
Mastering Core Concepts
- Learn Time Complexity Analysis: Netflix prioritizes O(n) or O(log n) solutions.
Practice Code Readability: Use meaningful variable names and modular functions.
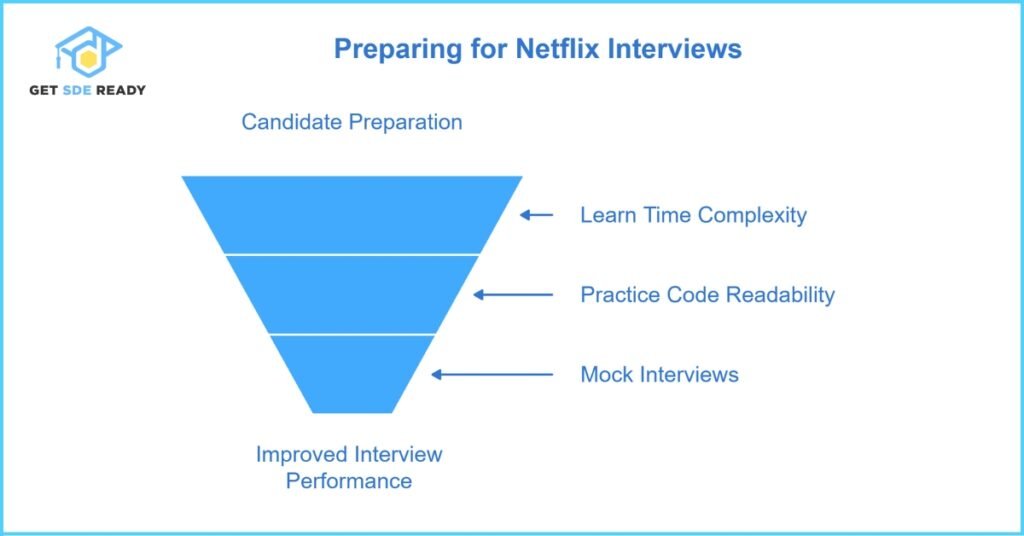
Recommended Resources:
- LeetCode (Netflix tag)
- Cracking the Coding Interview by Gayle Laakmann McDowell
Mock Interviews and Feedback
- Use platforms like Pramp to simulate Netflix’s interview environment.
- Record yourself explaining solutions to improve clarity.
Also Read: How to Crack DSA Interviews in 3 Months
Common Mistakes to Avoid
Ignoring Time Complexity
A 2022 survey by InterviewKickstart revealed that 60% of candidates fail Netflix interviews due to suboptimal solutions. Always analyze your approach’s efficiency.

Overcomplicating Solutions
Netflix engineers value simplicity. As senior engineer Jane Doe notes, “Elegant code solves the problem without unnecessary complexity.”
Also Read: Top 10 Greedy Algorithms for Programming
FAQs
How should I start preparing for Netflix’s DSA rounds?
Begin by mastering core topics like arrays, trees, and dynamic programming. Use platforms like LeetCode for practice. For structured guidance, enroll in our DSA Mastery Course, designed by industry experts.
Does Netflix ask system design questions in DSA rounds?
While DSA rounds focus on algorithms, system design is a separate stage. Prepare for both by exploring courses like Master DSA & System Design.
Are behavioral rounds important for Netflix interviews?
Yes! Netflix values candidates who align with their culture of ownership and innovation. Practice storytelling frameworks to highlight relevant experiences.
Also Read: System Design for Real-Time Chat Apps
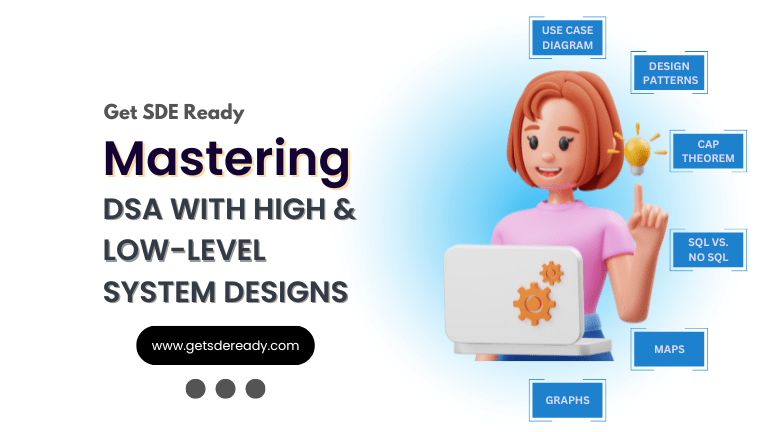
DSA, High & Low Level System Designs
- 85+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 400+ DSA Practice Questions
- Comprehensive Notes
- HackerRank Tests & Quizzes
- Topic-wise Quizzes
- Case Studies
- Access to Global Peer Community
Buy for 60% OFF
₹25,000.00 ₹9,999.00
Accelerate your Path to a Product based Career
Boost your career or get hired at top product-based companies by joining our expertly crafted courses. Gain practical skills and real-world knowledge to help you succeed.
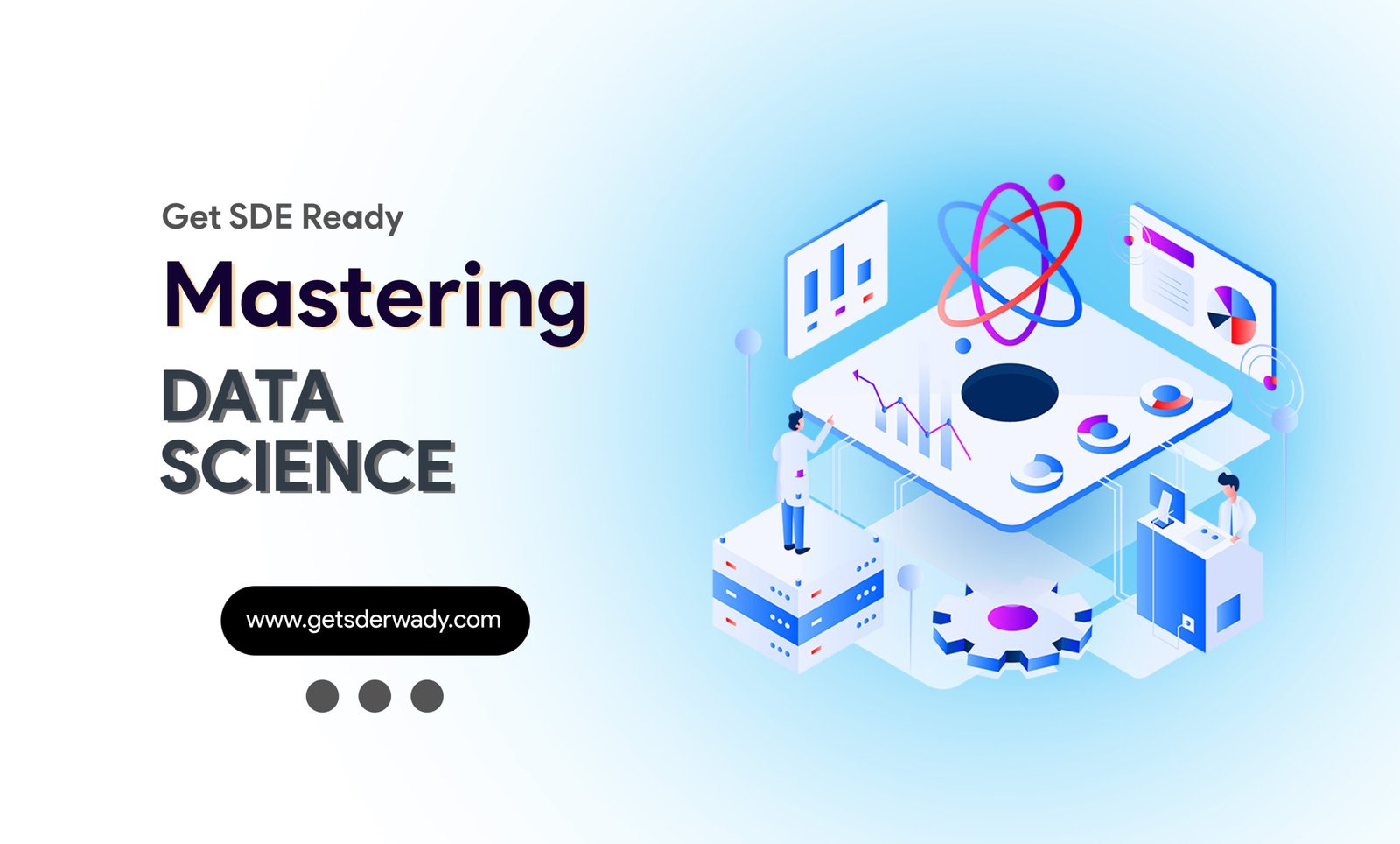
Essentials of Machine Learning and Artificial Intelligence
- 65+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 22+ Hands-on Live Projects & Deployments
- Comprehensive Notes
- Topic-wise Quizzes
- Case Studies
- Access to Global Peer Community
- Interview Prep Material
Buy for 65% OFF
₹20,000.00 ₹6,999.00
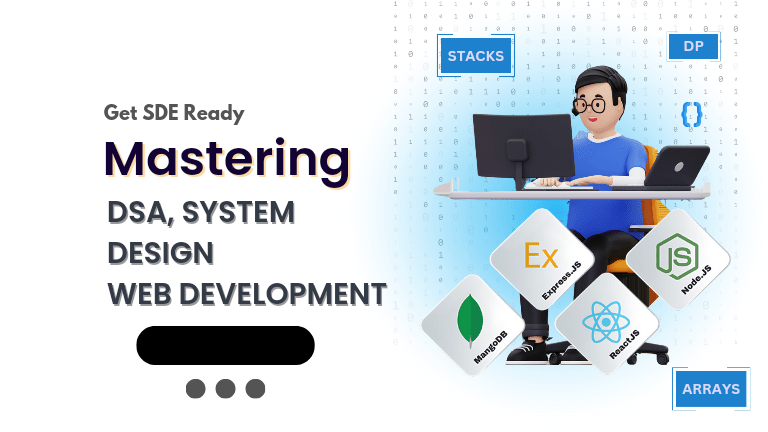
Fast-Track to Full Spectrum Software Engineering
- 120+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 400+ DSA Practice Questions
- Comprehensive Notes
- HackerRank Tests & Quizzes
- 12+ live Projects & Deployments
- Case Studies
- Access to Global Peer Community
Buy for 57% OFF
₹35,000.00 ₹14,999.00
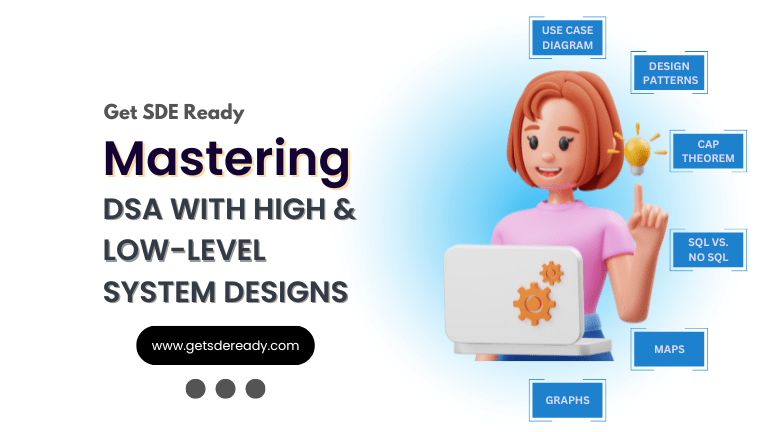
DSA, High & Low Level System Designs
- 85+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 400+ DSA Practice Questions
- Comprehensive Notes
- HackerRank Tests & Quizzes
- Topic-wise Quizzes
- Case Studies
- Access to Global Peer Community
Buy for 60% OFF
₹25,000.00 ₹9,999.00

Low & High Level System Design
- 20+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 400+ DSA Practice Questions
- Comprehensive Notes
- HackerRank Tests
- Topic-wise Quizzes
- Access to Global Peer Community
- Interview Prep Material
Buy for 65% OFF
₹20,000.00 ₹6,999.00
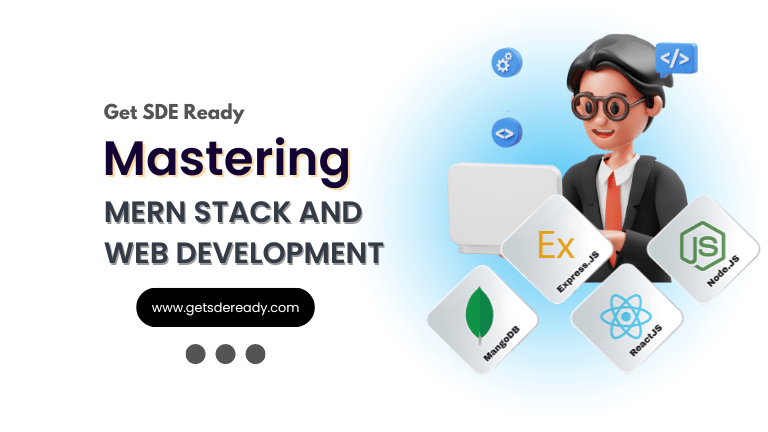
Mastering Mern Stack (WEB DEVELOPMENT)
- 65+ Live Classes & Recordings
- 24*7 Live Doubt Support
- 12+ Hands-on Live Projects & Deployments
- Comprehensive Notes & Quizzes
- Real-world Tools & Technologies
- Access to Global Peer Community
- Interview Prep Material
- Placement Assistance
Buy for 60% OFF
₹15,000.00 ₹5,999.00
Reach Out Now
If you have any queries, please fill out this form. We will surely reach out to you.
Contact Email
Reach us at the following email address.
arun@getsdeready.com
Phone Number
You can reach us by phone as well.
+91-97737 28034
Our Location
Rohini, Sector-3, Delhi-110085