
How to Solve Tricky Data Structures and Algorithms Questions in Coding Interviews
Cracking the coding interview is a daunting challenge for many aspiring software developers. One of the most difficult hurdles in interviews is tackling complex Data Structures and Algorithms (DSA) questions. These questions test not only your knowledge of data structures but also your problem-solving skills. Tricky DSA problems often require creative thinking, a structured approach, and a strong understanding of key concepts. In this article, we will break down strategies to effectively approach coding interview questions related to data structures and algorithms, providing practical steps to enhance your problem-solving skills and improve your chances of success.
Understanding the Problem
Before jumping into coding, the first and most important step is understanding the problem. Many candidates lose time or fail to solve DSA questions because they don’t grasp the problem fully. To tackle tricky DSA problems, follow a systematic approach to analyze the problem at hand.
Break the Problem Down
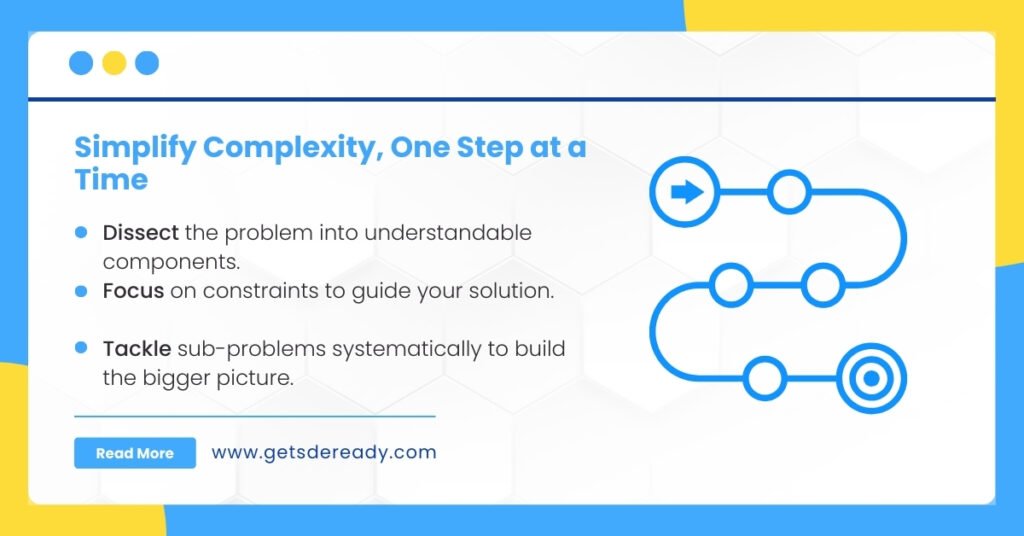
Start by reading the problem carefully and understanding the inputs and outputs. Ask yourself the following questions:
- What are the inputs?
- What are the outputs?
- Are there constraints mentioned?
- What’s the expected time complexity?
Once you have these key details, break down the problem into smaller, manageable chunks. This helps simplify the problem and makes it easier to solve step-by-step.
Key Steps:
- Read the problem multiple times if necessary.
- Identify edge cases and constraints.
- Divide the problem into smaller sub-problems for easier tackling.
Example Breakdown
For example, if you are asked to find the longest increasing subsequence, break the problem down as follows:
- Inputs: A list of integers.
- Outputs: The length of the longest increasing subsequence.
- Constraints: The input array could have up to 10,000 elements, and elements can be negative or zero.
Choose the Right Data Structure
Selecting the right data structure is often the key to solving tricky DSA questions efficiently. For example, if the problem requires fast lookups, a hashmap or set might be ideal. If the problem involves maintaining a dynamic ordered collection, a tree or heap might be the solution.
Analyze the Problem Requirements
Carefully analyze the problem’s requirements. Are you working with large amounts of data? Is time complexity a critical factor? Does the problem involve sorting, searching, or traversing data?
Once you’ve answered these questions, choose the data structure that aligns best with your needs. For example:
- Use arrays or lists for simple collections.
- Use queues or stacks when you need a last-in, first-out (LIFO) or first-in, first-out (FIFO) approach.
- Use graphs for problems involving networks or nodes.
- Use heaps for priority-based tasks like scheduling.
Key Data Structures for DSA:
- Arrays/Lists
- Stacks
- Queues
- Hashmaps
- Trees (Binary Trees, AVL Trees)
- Graphs
- Heaps
Also Read: Top 20 API Design Interview Questions
Plan and Outline the Approach
Once you understand the problem and have selected the appropriate data structure, the next step is planning your approach. It’s essential to outline the steps before diving into coding. This not only helps you stay focused but also minimizes the chances of making logical mistakes.
Step-by-Step Approach
Here’s how you can structure your plan:
- Initial Analysis: Identify the type of problem (sorting, searching, traversal, etc.).
- Breakdown: Break down the problem into smaller sub-problems.
- Write a Pseudocode: Write pseudocode to map out your algorithm before implementing it.
- Edge Cases: Account for any edge cases such as empty inputs, large numbers, or special conditions.
For instance, if you’re solving a dynamic programming problem, visualize how the problem can be solved by storing intermediate results. If it’s a graph problem, sketch the graph and determine whether you need DFS or BFS.
Solve the Problem Step by Step
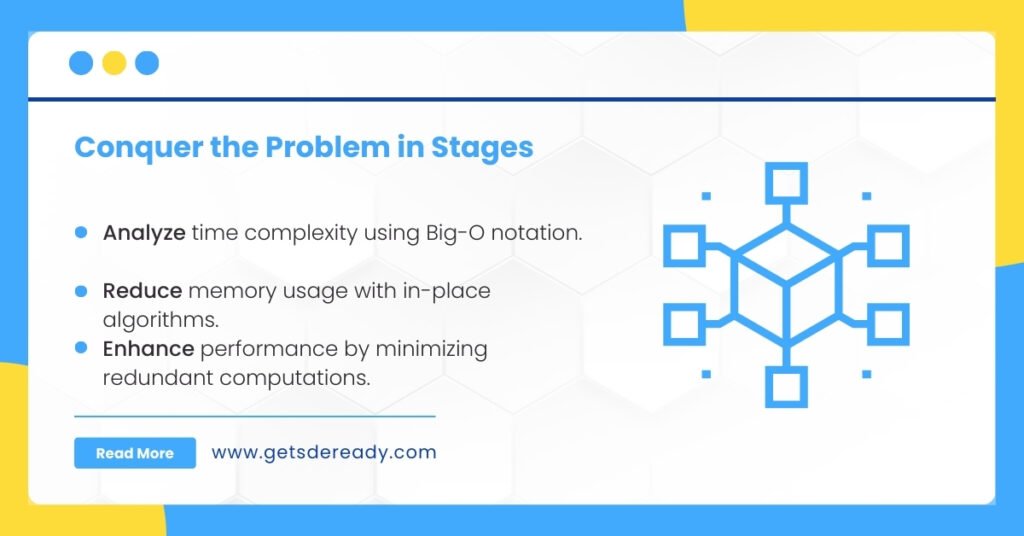
At this point, you should have a solid plan and a good understanding of how to implement the solution. Start coding but solve the problem incrementally.
Incremental Approach
- Start with Base Cases: Write code for simple cases first.
- Handle Edge Cases: Handle edge cases such as an empty array or null input.
- Test as You Go: After writing each section of the code, test it to ensure that it works as expected.
Key Steps in Incremental Coding:
- Solve the problem in smaller parts.
- Test your code frequently to catch bugs early.
Also Read: Top 10 Full-Stack Interview Questions
Optimize Your Solution
Optimizing your solution is crucial, especially when you are working with large datasets. For tricky DSA problems, inefficient algorithms can quickly become a bottleneck. It’s important to ensure that your solution is both time-efficient and space-efficient.
Analyze Time Complexity
Use Big-O notation to analyze the time complexity of your algorithm. Tricky DSA problems often require O(n log n) or O(log n) solutions for efficiency. If your initial solution has a time complexity of O(n²), try to optimize it by using better data structures or algorithms.
Space Optimization
Consider whether you can reduce the amount of memory used by your solution. Some problems allow for space optimization by using in-place algorithms or constant space solutions.
Optimization Tips:
- Use binary search for sorted arrays to reduce time complexity.
- Try to minimize space complexity by avoiding excessive use of extra data structures.
- Optimize loops and recursion to avoid unnecessary calculations.
Recommended Topic: Top 15 Blockchain Beginner Questions
Practice and Refine Your Skills
Solving tricky DSA problems requires consistent practice. The more problems you solve, the better your problem-solving skills will become. Make sure to practice on platforms like LeetCode, HackerRank, and CodeSignal to sharpen your skills.
Regular Practice
Set aside time each day to practice different DSA problems. Start with easy problems and gradually move to more complex ones. Focus on mastering common algorithms such as merge sort, quick sort, and binary search.
Top Practice Resources:
- LeetCode: Offers a wide range of problems categorized by difficulty.
- HackerRank: Provides problems with interactive coding environments.
- CodeForces: Regular contests with competitive DSA problems.
Recommended Topic: Top 15 Facebook Low-Level Design Questions
Debugging and Testing
When you’re stuck on a tricky problem or encountering bugs, it’s important to debug effectively. Many coding interviews require you to write bug-free code, and debugging skills are critical for solving complex problems quickly.
Debugging Tips
- Print Statements: Use print statements to check intermediate values and the state of variables.
- Use Debuggers: Modern IDEs come with built-in debuggers that can help you step through the code.
- Check for Off-by-One Errors: One of the most common bugs in DSA problems is off-by-one errors in loops or conditions.
Key Debugging Practices:
- Break down the code into smaller parts and isolate the issue.
- Test with various inputs, including edge cases and large data sets.
Conclusion
Tackling tricky Data Structures and Algorithms problems in coding interviews requires more than just knowledge of algorithms—it requires a methodical approach, strong problem-solving skills, and consistent practice. By breaking down the problem, selecting the right data structure, and optimizing your code, you will increase your chances of cracking the interview and securing the job you want.
FAQs
How do I start preparing for Data Structures and Algorithms (DSA) coding interviews?
Start by mastering basic data structures and algorithms. Practice solving problems regularly to improve your skills. For structured learning, consider courses like Master DSA, Web Dev & System Design.
What are the best resources for learning web development and DSA together?
The Design DSA Combined course offers a balanced approach to both web development and DSA, helping you build full-stack projects while mastering algorithms.
How can I improve my problem-solving skills in DSA efficiently?
Regular practice on coding platforms and understanding algorithms step by step will sharpen your problem-solving skills. For a focused approach, check out DSA Courses.
What should I focus on when learning web development for coding interviews?
Focus on core web technologies and practice building projects. If you want a structured path, explore Web Development Courses.
Is data science knowledge essential for DSA-related job interviews?
While not essential for all DSA interviews, knowledge of algorithms used in data science can enhance your problem-solving ability. Learn more through Data Science Courses.
Â
Accelerate your Path to a Product based Career
Boost your career or get hired at top product-based companies by joining our expertly crafted courses. Gain practical skills and real-world knowledge to help you succeed.
Reach Out Now
If you have any queries, please fill out this form. We will surely reach out to you.
Contact Email
Reach us at the following email address.
arun@getsdeready.com
Phone Number
You can reach us by phone as well.
+91-97737 28034
Our Location
Rohini, Sector-3, Delhi-110085