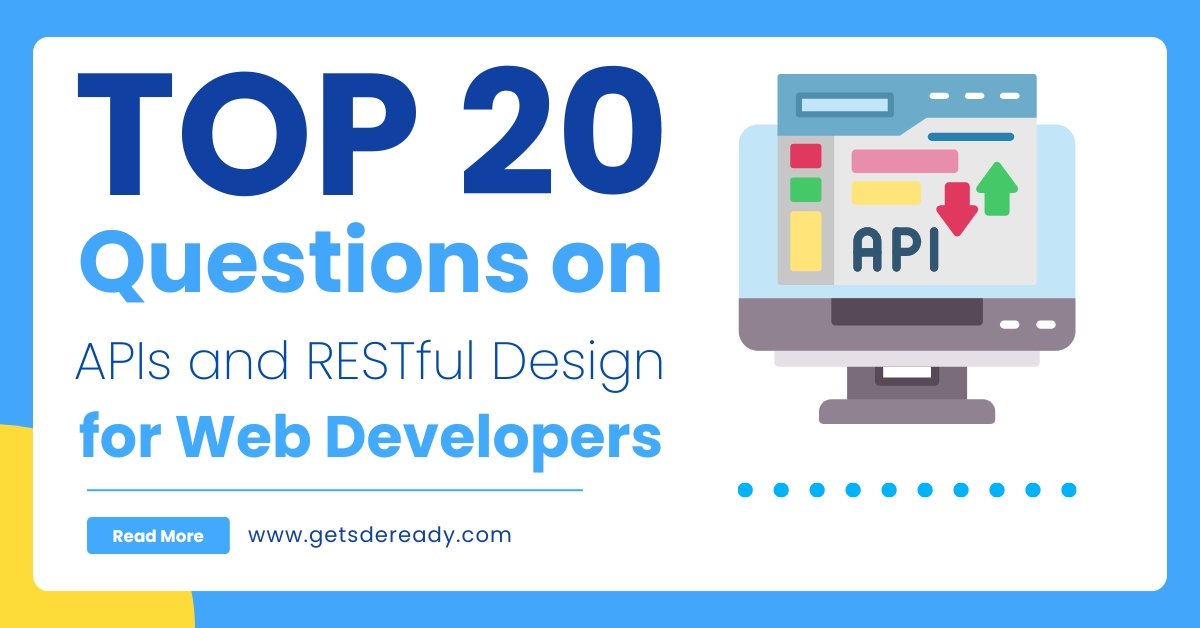
Top 10 System Design Interview Questions in 2025
System design interviews are an integral part of the hiring process at top tech companies such as Google, Amazon, Meta, and Microsoft. These interviews assess a candidate’s ability to architect scalable, reliable, and efficient systems that address real-world challenges.Â
Introduction
Over my decade-long experience as a Systems Engineer and Hiring Manager at Microsoft and Facebook, I have conducted hundreds of system design interviews. One common observation is that even highly skilled developers struggle with system design problems due to their open-ended nature. Such challenges require creative thinking and strong problem-solving abilities beyond typical coding tasks.
This comprehensive guide covers the top 10 system design interview questions for 2025, categorized by difficulty level. It also provides battle-tested strategies to approach these problems with confidence.For more free resources and course updates, check out this form.
Why System Design Interviews Are Challenging
1. Open-Ended Nature
- Unlike coding interviews, system design questions are often vague and open-ended, such as “Design a scalable chat application.”
- This lack of structure makes it difficult to know where to start or what the interviewer expects.
2. Broad Scope
- System design covers many domains: scalability, reliability, availability, consistency, fault tolerance, and more.
- You need knowledge of concepts like:
- Load balancing
- Database sharding
- Caching
- Message queues
- Distributed systems
3. Real-World Complexity
- These interviews simulate real-world challenges of designing systems for millions or billions of users.
- You must balance trade-offs, handle failures, and ensure performance while scaling systems.
4. Expecting Trade-Off Analysis
- There’s rarely a single “correct” answer. Instead, you’re judged on your ability to analyze trade-offs, such as:
- SQL vs. NoSQL databases
- Strong vs. eventual consistency
- Monolithic vs. microservices architecture
5. Focus on Communication
- Beyond technical skills, system design interviews assess your ability to:
- Communicate ideas clearly
- Articulate your thought process
- Justify decisions
- Respond effectively to clarifying questions
6. Time Constraints
- You’re often tasked with designing complex systems like Instagram or Uber within 45-60 minutes.
- This requires sharp prioritization to cover critical aspects while skipping unnecessary details.
7. Dynamic Problem-Solving
- Interviewers may introduce new requirements or constraints midway through the session.
- Example: After designing a basic system, you may be asked, “How would you handle sudden traffic spikes?”
- This tests your adaptability to evolving challenges.
Many candidates find these interviews challenging due to their ambiguity and the need to balance multiple trade-offs.
Top 10 System Design Interview Questions
The questions are divided into three categories based on complexity:
1. Easy System Design Interview Questions
Question | Key Considerations |
Design an API rate limiter | Traffic control, error handling, scalability |
Design a pub/sub system like Kafka | Message persistence, scalability, reliability |
Design a URL-shortening service | Unique URL generation, efficient storage, redirection speed |
Design a content delivery network (CDN) | Caching strategies, load balancing, latency reduction |
Design a web crawler | Distributed crawling, politeness policies, URL deduplication |
Design a distributed cache | Consistency, eviction policies, latency |
2. Medium System Design Interview Questions
Question | Key Considerations |
Design a chat service like WhatsApp | Message delivery guarantees, real-time updates, data consistency |
Design a social media platform like Instagram | Media storage, scalability, newsfeed generation |
Design a proximity service like Yelp | Geolocation indexing, real-time updates, caching strategies |
Design a recommendation engine | Collaborative filtering, personalization, scalability |
Design a ride-sharing service like Uber | Matching algorithms, pricing, geolocation tracking |
3. Hard System Design Interview Questions
Question | Key Considerations |
Design a social media newsfeed | Personalization, ranking algorithms, real-time updates |
Design Google Maps | Spatial indexing, route optimization, scalability |
Design a payment gateway | Security, transaction processing, fraud detection |
Design a distributed storage system | Data partitioning, replication, consistency |
Design an online multiplayer game system | Low latency, synchronization, cheat prevention |
Effective Strategies to Approach System Design Interviews
1. Requirements Gathering
- Identify functional requirements (e.g., user authentication, notifications).
- List non-functional requirements (e.g., scalability, fault tolerance, performance).
- Clarify ambiguities by asking questions.
2. System Architecture Planning
- Choose the right architecture: monolithic vs. microservices.
- Discuss scalability using techniques such as load balancing, caching, and database sharding.
- Identify key components: databases, APIs, message queues.
3. Trade-off Analysis
- Compare different design choices based on trade-offs such as consistency vs. availability (CAP theorem).
- Evaluate storage options (SQL vs. NoSQL).
4. Scalability Considerations
- Load distribution strategies (horizontal vs. vertical scaling).
- Performance optimization using CDNs and caching layers.
- Distributed systems and fault tolerance.
5. Real-World Enhancements
- Consider emerging technologies such as machine learning for predictive analytics.
- Explore cloud services (AWS, Azure, GCP) to enhance scalability.
Recommended Topic: Top 10 Frontend Design Questions
1. API Rate Limiter Design
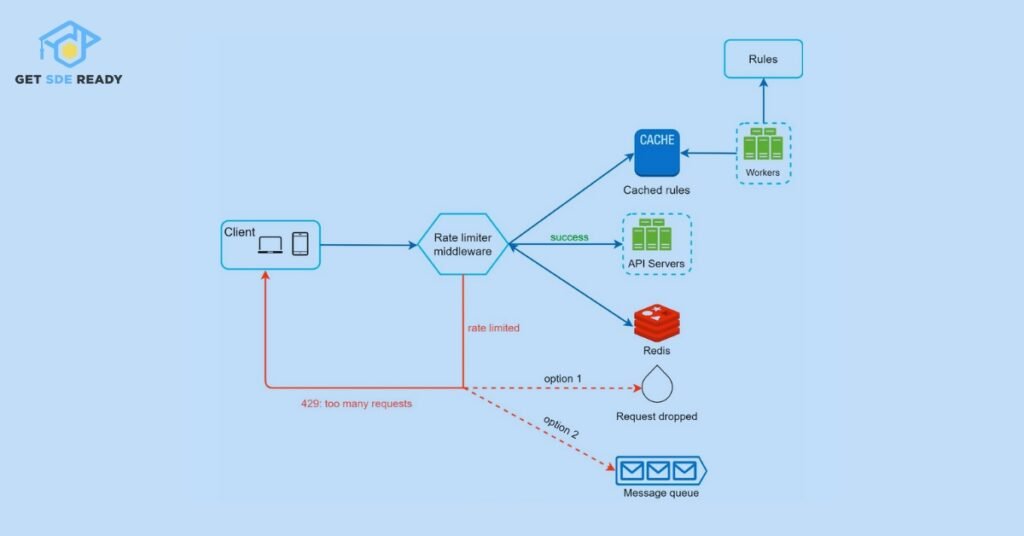
Problem Statement
Design an API rate limiter to cap the number of API calls a service can accept in a given period. This mechanism is crucial to prevent service overloads and ensure fair usage of resources.
Functional Requirements
- Limit Requests: The rate limiter should define a maximum number of requests allowed per client within a set period.
- Configurable: It must be possible to configure the rate limits per endpoint or per user.
- Error or Notification: When the limit is reached, the system must notify the user with an HTTP 429 response (“Too Many Requests”).
Nonfunctional Requirements
- Availability: The rate limiter must be available at all times without causing delays.
- Low Latency: The system should introduce minimal delay in request processing.
- Scalability: The solution must scale horizontally to handle high volumes of incoming requests.
System Design Workflow
The design follows a flow where each incoming request passes through an ID Builder, which identifies the client using a unique identifier (IP address, user ID, etc.). Then, a Decision Maker checks the Throttling Rules stored in the database. If the request exceeds the limit, the system returns a 429 error. If not, the request is forwarded to the Application Servers for processing.
In the event of a high request load, throttled requests are placed in a Queue for processing once the system is less congested.
Key Design Challenges
- Handling Requests Across Time Windows: It’s essential to measure requests within dynamic time windows. For example, if a user sends 20 requests over multiple time segments (e.g., 00:01:20 and 00:02:10), how should this be managed?
- Handling Failures: If the rate limiter fails, it may lead to either rejecting or allowing requests. This is critical for ensuring service reliability.
Improvement for Distributed Systems
In a distributed system, data consistency across nodes becomes a challenge. You may need to employ consistent hashing to balance requests across servers while ensuring cache consistency and handling session persistence.
Also Read: Top 15 DSA Questions on Arrays & Strings
2. Pub/Sub System Design
Problem Statement
Design a scalable and distributed Pub/Sub system like Kafka, capable of handling massive message throughput while ensuring reliable message delivery and supporting various messaging semantics.
Functional Requirements
- Create a Topic: Allow users to define topics for message distribution.
- Write and Read Messages: Producers write messages, and consumers read them.
- Retention and Deletion: Set retention policies for messages and delete them when necessary.
Nonfunctional Requirements
- Availability: Ensure high availability of message brokers.
- Scalability and Durability: The system should be able to scale horizontally and maintain durability even in failure scenarios.
- Concurrency: Efficiently handle multiple consumers reading and writing concurrently.
System Design Workflow
In this design, the Brokers store messages from Producers, while Consumers retrieve them. The Cluster Manager monitors the health of brokers and adds new brokers as needed to maintain availability. Consumer details, such as subscriptions and retention periods, are managed by the Consumer Manager.
Message Semantics
- At Least Once: Ensure that each message is delivered at least once, even if a failure occurs.
- Exactly Once: Guarantee that each message is delivered only once, avoiding duplicates.
- At Most Once: Ensure that messages are not repeated, even if there’s a failure.
Message Ordering
A key aspect is ensuring messages are delivered in order to specific consumers. This can be achieved using partitioning and ensuring that the messages within each partition are ordered.
Recommended Topic: Top 20 DSA Questions for 2025 Interviews
3. URL-Shortening Service Design
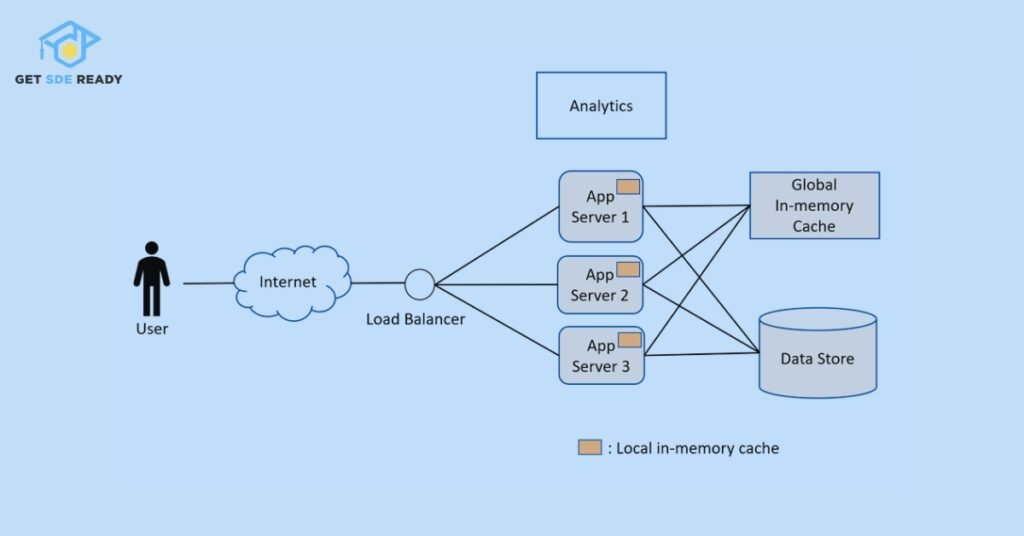
Problem Statement
Design a distributed and scalable URL-shortening service, like TinyURL or Bit.ly, which takes long URLs and generates short, unique versions.
Functional Requirements
- URL Generation: Create a unique short URL for every long URL.
- Redirection: Redirect to the original URL when the shortened version is accessed.
- Customization: Allow users to choose custom short URLs.
Nonfunctional Requirements
- Scalability: The system must handle millions of requests efficiently.
- Low Latency: Shortening and redirection should occur quickly.
- High Availability: Ensure system availability, even under heavy load.
System Design Workflow
Requests for URL shortening are first passed to a Load Balancer, which evenly distributes traffic to the available servers. The Sequencer generates unique IDs, which are encoded into short URLs by an Encoder. The URLs are stored in a Database, with frequently accessed URLs kept in a Cache to speed up retrieval.
Key Design Considerations
- Custom URL Conflicts: If two users choose the same custom URL, the system must handle conflicts appropriately by either rejecting or appending a suffix to the URL.
- Scalability Concerns: As user volume increases, additional caching and database partitioning strategies will be required to maintain performance.
Â
Also Read: Top 15 Companies Asking Advanced Design
4. Scalable Content Delivery Network (CDN) Design
Problem Statement
Design a scalable CDN that efficiently distributes content across global servers, minimizing latency and ensuring reliable content delivery.
Functional Requirements
- Content Retrieval: Fetch content from the origin server and cache it.
- Auto-content Delivery: Serve content from edge servers automatically if cached.
- Search and Update: Search for content and update it when necessary.
Nonfunctional Requirements
- Scalability: Efficiently scale to handle massive traffic.
- Low Latency: Deliver content quickly, even in high-traffic conditions.
- Security: Ensure the integrity of content and protect against DDoS attacks.
System Design Workflow
When a client requests content, the Request Routing System determines the nearest or fastest server. A Load Balancer directs the request to the optimal server. If the content is cached, it is returned directly. If not, the server fetches it from the origin and caches it for future use.
Content Caching Strategies
- Edge Caching: Frequently requested content is cached on edge servers to reduce latency.
- Cache Purging: Less popular content is periodically removed from caches to free up space for new content.
Key Design Questions
- Cache Optimization: How do you determine which content to cache?
- Scalability & Fault Tolerance: How does the CDN handle failures and scale globally?
Â
Recommended Topic: Solving Tricky DSA Interview Questions
5. Web Crawler Design
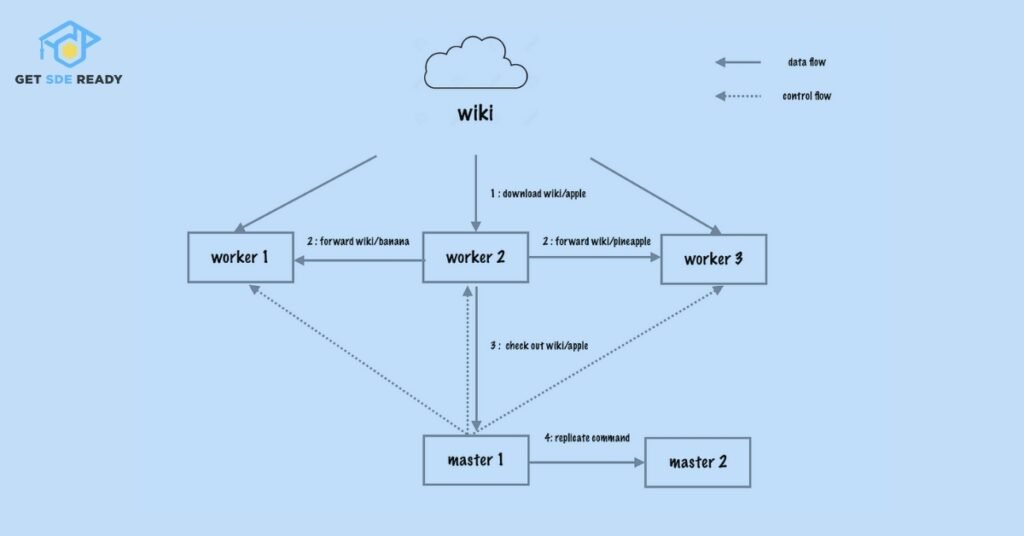
Problem Statement
Design a web crawler that efficiently indexes web pages by navigating through links and retrieving content.
Functional Requirements
- Crawling and Storing: Retrieve and store the content of web pages.
- Periodic Crawling: Schedule crawling at regular intervals to keep content up-to-date.
Nonfunctional Requirements
- Scalability: Ensure the crawler can scale to handle large volumes of websites.
- Reliability: The crawler must consistently navigate and index pages without failures.
System Design Workflow
The crawler uses workers to resolve DNS and connect to web pages. After extracting content, the Duplicate Eliminator ensures that no duplicate data is stored. The crawler follows links on each page and queues them for future crawling.
Handling Crawl Traps
The crawler must intelligently avoid infinite loops (crawl traps) by managing visited URLs and tracking the links it follows.
Also Read: 10 Tips for Mastering FAANG Design
6. Distributed Cache System Design
Problem Statement
Design a distributed caching system to provide fast data retrieval and handle large volumes of traffic.
Functional Requirements
- Data Insertion and Retrieval: Efficiently write and retrieve data across distributed cache nodes.
- Cache Eviction: Manage cache evictions to ensure space is available for new data.
Nonfunctional Requirements
- Consistency: Maintain data consistency across all cache nodes.
- High Availability and Low Latency: Ensure rapid access to data, even during high traffic.
System Design Workflow
Data is partitioned across multiple cache nodes using Consistent Hashing. When a client requests data, the system uses the appropriate cache node. Cache Misses are handled by retrieving the data from the primary data store and caching it.
Cache Eviction Strategies
- Least Recently Used (LRU): Evict the least recently accessed data when space is needed.
- Time-to-Live (TTL): Remove expired cache entries after a predefined period.
Key Design Considerations
- Data Consistency: Ensure that all cache nodes reflect the latest updates.
- Cache Miss Optimization: Implement strategies to avoid overwhelming the primary data store.
Â
Recommended Topic: Roadmap to Crack Startup Design Interviews
7. Designing a Scalable, Real-Time Chat Service Like WhatsApp or Facebook Messenger
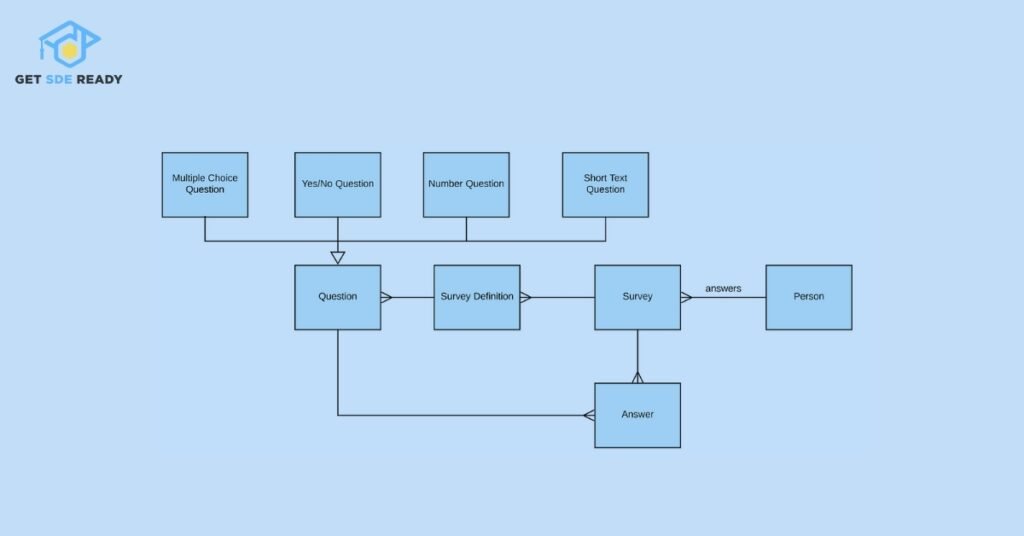
Designing a robust real-time messaging service that supports individual and group communication, media sharing, notifications, and message delivery acknowledgment is a complex task. Systems like WhatsApp and Facebook Messenger handle millions of users, requiring highly scalable, secure, and reliable architectures. This post explores the key design components needed to create such a system.
Functional Requirements for a Chat Service
To ensure that the messaging service meets users’ needs, the following functional requirements must be fulfilled:
- Real-Time Communication
The system must allow users to send and receive messages instantly, whether individually or in groups. - Message Delivery Acknowledgment
Each message sent must receive an acknowledgment once it has been successfully delivered or read, providing feedback to users about the status of their messages. - Media Sharing
The chat service must support sharing various types of media, including images, videos, voice messages, and documents. - Chat Storage
Messages and media should be stored in a database for history retrieval, allowing users to search their message history. - Notifications
Push notifications should be sent to users for new messages or updates, even when the app is running in the background or is closed.
Non-Functional Requirements
In addition to the functional requirements, the system should meet the following non-functional requirements to ensure it performs optimally:
- Availability
The system should be highly available, providing 24/7 access without downtime. - Low Latency
Real-time messaging demands low latency for both sending and receiving messages to ensure a seamless user experience. - Scalability
As the number of users grows, the system should scale horizontally to handle increased load while maintaining performance. - Consistency
The service must ensure that users receive consistent updates, particularly when multiple devices or platforms are in use simultaneously. - Security
End-to-end encryption should be implemented to protect the confidentiality and integrity of messages.
System Design and Workflow
To achieve the above requirements, a real-time chat service can be built using a combination of scalable architectures and protocols that ensure efficient delivery, storage, and retrieval of messages. Here’s a high-level design of how such a system works:
- Real-Time Communication
For real-time communication, protocols like WebSocket, XMPP, or MQTT can be used. These allow for continuous communication between clients and servers, ensuring messages are delivered instantly.
A WebSocket Manager can be responsible for managing connections between clients and servers, ensuring that each message is transmitted in real-time. - Message Delivery
Messages sent by the sender are placed in a message queue to be delivered to the recipient. Each message is tracked for delivery acknowledgment, and users are notified once the message is successfully sent and read. - Media Sharing
When users send media, the files are stored in a cloud storage service. Metadata and file references are then saved in the database for retrieval. - Database and Storage
Messages are stored in a relational database for fast retrieval and management. Media files are typically stored in blob storage, which is optimized for large files. - Notifications
Notifications are managed by a push notification service. These notifications inform users of new messages or updates, and they are pushed through the network to the client devices. - Scalability and Fault Tolerance
To handle scalability, load balancers are used to distribute incoming traffic across multiple servers. Servers are replicated to ensure the system remains available even in case of server failures. - Security
Messages are encrypted using end-to-end encryption, ensuring that only the sender and receiver can read the message content. The encryption keys are securely managed.
High-Level System Diagram
Here is a simplified diagram of a real-time chat system:
Knowledge Test and Key Considerations
- What happens if a message is sent when the user isn’t connected to the internet? Is it sent when the connection is restored?
If the user is offline, the message will be stored in a local message queue on the device. When the device reconnects to the internet, the message will be automatically sent to the server. - How will you encrypt and decrypt the message without increasing latency?
Encryption and decryption operations can be optimized by using efficient algorithms like AES and by offloading these operations to hardware acceleration if possible. This reduces the computational overhead and prevents latency issues. - How do users receive notifications?
Notifications are pushed via a push notification service. These notifications alert the user whenever a new message is received, ensuring prompt user engagement. - Are messages pulled from the device (the server periodically prompts the devices if they’re waiting to send a message) or are pushed to the server (the device prompts the server that it has a message to send)?
Messages are pushed to the server. When a user sends a message, the device triggers a push request to the server, ensuring the message is sent in real time.
Also Read: Top 10 Distributed System Design Challenges
8. Designing a Mass Social Media Service Like Facebook or Instagram
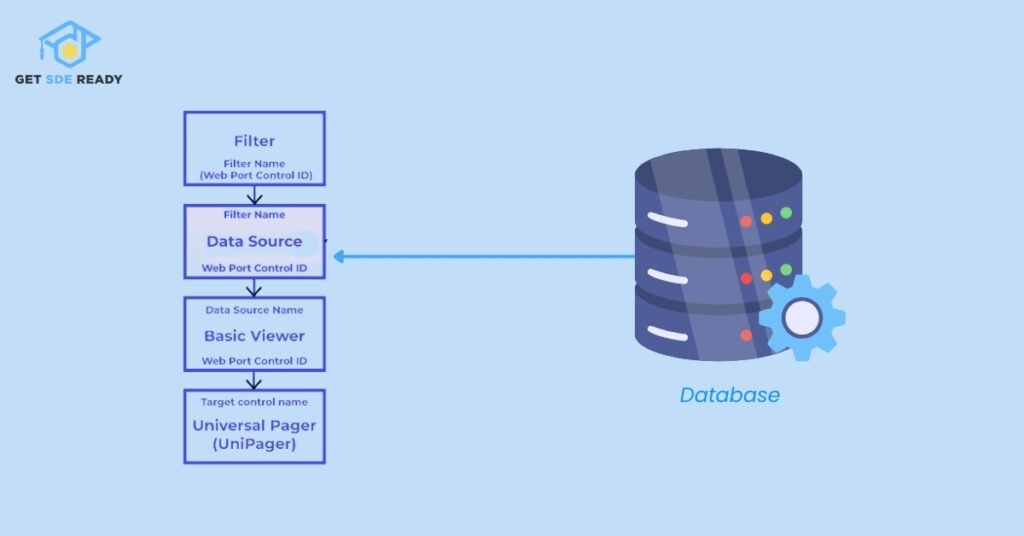
Building a scalable and efficient social media platform that can handle millions of users and their dynamic needs requires careful consideration of design, performance, and user interaction. Platforms like Facebook and Instagram are based on similar architectures but differ in specific features and technologies.
Functional Requirements for a Social Media Platform
The platform must support the following functional features to ensure a satisfying user experience:
- Create, Edit, Delete, and Share Posts
Users should be able to interact with their content in multiple ways, such as creating, editing, and deleting posts, as well as sharing them. - Follow and Unfollow Users
The platform must allow users to follow and unfollow other users to personalize their feed. - Search for Content
Users must be able to search for content, posts, and users easily. - Like and Dislike Posts
A simple mechanism for interacting with posts, through likes and dislikes, is essential.
Non-Functional Requirements
Key non-functional requirements for such a system include:
- Scalability
The system should be able to handle a growing number of users and posts. - Availability
The platform should be always available with minimal downtime. - Low Latency
Low latency is crucial for real-time interactions, such as comments and likes. - Reliability
The system must be robust enough to handle failures gracefully and maintain user data integrity. - Security
Data privacy is essential, with users’ personal data protected by encryption.
System Design and Workflow
To design a high-performance social media platform like Instagram, we need several critical components:
- Post Service
The post service handles content creation and management, ensuring that posts are stored efficiently and are quickly accessible to users. - Feed Generation and Ranking
The system’s timeline generation service is responsible for providing the user’s personalized feed. It pulls posts from the users they follow and ranks them based on relevance, using a feed ranking and recommendation engine. This engine analyzes the user’s interaction history, followed users, and interests to recommend the most relevant posts. - Content Delivery Network (CDN)
For video and image posts, the system uses a CDN to deliver content quickly with minimal latency. - Data Storage
Posts and interactions (likes, comments) are stored in a relational database, while images and videos are typically stored in cloud storage services. - Social Graph
A social graph stores relationships between users, enabling functionalities like following/unfollowing and determining the posts to display in a user’s feed.
Â
High-Level System Diagram
Here is a simplified diagram of a social media platform:
Knowledge Test and Key Considerations
- How do influencers or celebrities handle millions of followers?
Influencers typically require special handling within the system, such as dedicated servers or optimized routing, to manage the higher number of requests and ensure timely delivery of their posts. - How does the system weight posts by age?
Posts that are older may be given less weight in ranking algorithms, with fresher posts being prioritized in user feeds. - What’s the ratio of read and write-focused nodes?
For social media platforms, read-heavy architectures are common, as users are more likely to view posts than create them. Therefore, read-optimized nodes will be prevalent. - How do you store posts and images efficiently?
Efficient storage strategies, such as compressing images and using blob storage for media, are essential to optimize both space and retrieval times.
9. Design a proximity service like Yelp or nearby places/friends
Problem statement:
Design a proximity server that stores and reports the distance to places like restaurants. Users can search nearby places by distance or popularity. The database must store data for hundreds of millions of businesses across the globe.
Requirements
Follow these requirements for a System Design like Yelp:
Functional requirements
- User accounts
- Search
- Feedback
Nonfunctional requirements
- Scalability
- High availability
- Consistency
- Performance
System Design and workflow
The system handles search requests by using load balancers to distribute read requests to the read service, which then queries the quadtree service to identify relevant places within a specified radius. The quadtree service also refines the result before being sent to the clients. For adding places or feedback, write requests are similarly routed through load balancers to the writing service, which updates a relational database and stores images in blob storage. The system also involves segmenting the world map into smaller parts, storing places in a key-value store, and periodically updating these segments to include new places, although this update happens monthly due to the low probability of new additions.
A high-level design of proximity service like Yelp
![A high-level design of proximity service like Yelp]
Knowledge test!
- How do you store lots of data and retrieve search results quickly?
- How should the system handle different population densities? Rigid latitude/longitude grids will cause varied responsiveness based on density.
- Can we optimize commonly searched locations?
Â
Note: Look at the detailed design of Yelp to get answers to the above questions.
10. Design a search engine-related service like Typeahead
Problem statement:
Design a typeahead suggestion system that provides real-time, relevant autocomplete and autocorrect suggestions as users type, ensuring low latency and scalability to efficiently handle a large volume of queries.
Requirements
Follow these requirements for the system:
Functional requirements
- Autocomplete
- Autocorrect
Nonfunctional requirements
- Scalability
- Fault tolerance
- Performance
System Design and workflow
When a user starts typing a query, each individual character is sent to an application server. A suggestion service gathers the top N suggestions from a distributed cache, or Redis, and returns the list to the user. An alternate service called the data collector and aggregator takes the query, analytically ranks it, and stores it in a NoSQL database. The trie builder is a service that takes the aggregated data from the NoSQL database, builds tries, and stores them in the trie database.
A high-level design of Typeahead
![A high-level design of Typeahead]
Knowledge test!
- How strongly do you weigh spelling mistake corrections?
- How do you update selections without causing latency?
- How do you determine the most likely completed query? Does it adapt to the user’s searches?
- What happens if the user types very quickly? Do suggestions only appear after they’re done?
FAQs
What is DSA, and why is it important for software development?
Data Structures and Algorithms (DSA) are fundamental concepts that every software developer should master. They help improve problem-solving skills and optimize code. For an in-depth understanding, explore our DSA courses here.
Can I learn web development from scratch?Â
Yes, our Web Development course is designed for beginners and covers everything from HTML to advanced frameworks. Start your journey today at Get SDE Ready.
How does the combined DSA and System Design course help in my career?Â
This combined course is perfect for mastering both DSA and system design, two key areas required for top tech interviews. Find more details here.
What can I expect from the ‘Master DSA, Web Dev & System Design’ course?Â
This comprehensive course offers an integrated approach to DSA, Web Development, and System Design, preparing you for high-level software engineering roles. Check it out here.
Is there a course to get started with Data Science?Â
Our Data Science course covers everything from statistics to machine learning, perfect for beginners or those looking to upskill. Start learning here.
Accelerate your Path to a Product based Career
Boost your career or get hired at top product-based companies by joining our expertly crafted courses. Gain practical skills and real-world knowledge to help you succeed.
Reach Out Now
If you have any queries, please fill out this form. We will surely reach out to you.
Contact Email
Reach us at the following email address.
arun@getsdeready.com
Phone Number
You can reach us by phone as well.
+91-97737 28034
Our Location
Rohini, Sector-3, Delhi-110085